KDEUI
#include <kactioncollection.h>
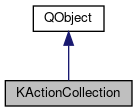
Signals | |
QT_MOC_COMPAT void | actionHighlighted (QAction *action) |
void | actionHovered (QAction *action) |
void | actionTriggered (QAction *action) |
void | inserted (QAction *action) |
QT_MOC_COMPAT void | removed (QAction *action) |
Public Member Functions | |
KActionCollection (QObject *parent, const KComponentData &cData=KComponentData()) | |
virtual | ~KActionCollection () |
QAction * | action (int index) const |
QAction * | action (const QString &name) const |
const QList< QActionGroup * > | actionGroups () const |
QList< QAction * > | actions () const |
const QList< QAction * > | actionsWithoutGroup () const |
template<class ActionType > | |
ActionType * | add (const QString &name, const QObject *receiver=0, const char *member=0) |
QAction * | addAction (const QString &name, QAction *action) |
KAction * | addAction (const QString &name, KAction *action) |
KAction * | addAction (KStandardAction::StandardAction actionType, const QObject *receiver=0, const char *member=0) |
KAction * | addAction (KStandardAction::StandardAction actionType, const QString &name, const QObject *receiver=0, const char *member=0) |
KAction * | addAction (const QString &name, const QObject *receiver=0, const char *member=0) |
void | addAssociatedWidget (QWidget *widget) |
QList< QWidget * > | associatedWidgets () const |
void | associateWidget (QWidget *widget) const |
void | clear () |
void | clearAssociatedWidgets () |
KComponentData | componentData () const |
QString | configGroup () const |
bool | configIsGlobal () const |
int | count () const |
void | exportGlobalShortcuts (KConfigGroup *config, bool writeDefaults=false) const |
void | importGlobalShortcuts (KConfigGroup *config) |
bool | isEmpty () const |
const KXMLGUIClient * | parentGUIClient () const |
void | readSettings (KConfigGroup *config=0) |
void | removeAction (QAction *action) |
void | removeAssociatedWidget (QWidget *widget) |
void | setComponentData (const KComponentData &componentData) |
void | setConfigGlobal (bool global) |
void | setConfigGroup (const QString &group) |
QAction * | takeAction (QAction *action) |
void | writeSettings (KConfigGroup *config=0, bool writeDefaults=false, QAction *oneAction=0) const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static const QList < KActionCollection * > & | allCollections () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
virtual void | connectNotify (const char *signal) |
virtual QT_MOC_COMPAT void | slotActionHighlighted () |
virtual void | slotActionTriggered () |
Properties | |
QString | configGroup |
bool | configIsGlobal |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A container for a set of QAction objects.
KActionCollection manages a set of QAction objects. It allows them to be grouped for organized presentation of configuration to the user, saving + loading of configuration, and optionally for automatic plugging into specified widget(s).
Additionally, KActionCollection provides several convenience functions for locating named actions, and actions grouped by QActionGroup.
- Note
- If you create your own action collection and need to assign shortcuts to the actions within, you have to call associateWidget() or addAssociatedWidget() to have them working.
Definition at line 56 of file kactioncollection.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Allows specification of a KComponentData other than the default global KComponentData, where needed.
Definition at line 96 of file kactioncollection.cpp.
|
virtual |
Destructor.
Definition at line 117 of file kactioncollection.cpp.
Member Function Documentation
QAction * KActionCollection::action | ( | int | index | ) | const |
Return the QAction* at position "index" in the action collection.
This is equivalent to actions().value(index);
Definition at line 141 of file kactioncollection.cpp.
Get the action with the given name from the action collection.
- Parameters
-
name Name of the KAction
- Returns
- A pointer to the KAction in the collection which matches the parameters or null if nothing matches.
Definition at line 131 of file kactioncollection.cpp.
const QList< QActionGroup * > KActionCollection::actionGroups | ( | ) | const |
Returns the list of all QActionGroups associated with actions in this action collection.
Definition at line 200 of file kactioncollection.cpp.
|
signal |
Indicates that action was highlighted (hovered over).
- Deprecated:
- Replaced by actionHovered(QAction* action);
|
signal |
Indicates that action was hovered.
Returns the list of KActions which belong to this action collection.
The list is guaranteed to be in the same order the action were put into the collection.
Definition at line 186 of file kactioncollection.cpp.
Returns the list of KActions without an QAction::actionGroup() which belong to this action collection.
Definition at line 191 of file kactioncollection.cpp.
|
signal |
Indicates that action was triggered.
|
inline |
Creates a new action under the given name, adds it to the collection and connects the action's triggered(bool) signal to the specified receiver/member.
The receiver slot may accept either a bool or no parameters at all (i.e. slotTriggered(bool) or slotTriggered() ). The type of the action is specified by the template parameter ActionType.
NOTE: KDE prior to 4.2 connected the triggered() signal instead of the triggered(bool) signal.
- Parameters
-
name The internal name of the action (e.g. "file-open"). receiver The QObject to connect the triggered(bool) signal to. Leave 0 if no connection is desired. member The SLOT to connect the triggered(bool) signal to. Leave 0 if no connection is desired.
- Returns
- new action of the given type ActionType.
Definition at line 412 of file kactioncollection.h.
Add an action under the given name to the collection.
Inserting an action that was previously inserted under a different name will replace the old entry, i.e. the action will not be available under the old name anymore but only under the new one.
Inserting an action under a name that is already used for another action will replace the other action in the collection (but will not delete it).
- Parameters
-
name The name by which the action be retrieved again from the collection. action The action to add.
- Returns
- the same as the action given as parameter. This is just for convenience (chaining calls) and consistency with the other addAction methods, you can also simply ignore the return value.
Definition at line 217 of file kactioncollection.cpp.
Definition at line 209 of file kactioncollection.cpp.
KAction * KActionCollection::addAction | ( | KStandardAction::StandardAction | actionType, |
const QObject * | receiver = 0 , |
||
const char * | member = 0 |
||
) |
Creates a new standard action, adds it to the collection and connects the action's triggered(bool) signal to the specified receiver/member.
The newly created action is also returned.
Note: Using KStandardAction::OpenRecent will cause a different signal than triggered(bool) to be used, see KStandardAction for more information.
The action can be retrieved later from the collection by its standard name as per KStandardAction::stdName.
- Parameters
-
actionType The standard action type of the action to create. receiver The QObject to connect the triggered(bool) signal to. Leave 0 if no connection is desired. member The SLOT to connect the triggered(bool) signal to. Leave 0 if no connection is desired.
- Returns
- new action of the given type ActionType.
Definition at line 337 of file kactioncollection.cpp.
KAction * KActionCollection::addAction | ( | KStandardAction::StandardAction | actionType, |
const QString & | name, | ||
const QObject * | receiver = 0 , |
||
const char * | member = 0 |
||
) |
Creates a new standard action, adds to the collection under the given name and connects the action's triggered(bool) signal to the specified receiver/member.
The newly created action is also returned.
Note: Using KStandardAction::OpenRecent will cause a different signal than triggered(bool) to be used, see KStandardAction for more information.
The action can be retrieved later from the collection by the specified name.
- Parameters
-
actionType The standard action type of the action to create. name The name by which the action be retrieved again from the collection. receiver The QObject to connect the triggered(bool) signal to. Leave 0 if no connection is desired. member The SLOT to connect the triggered(bool) signal to. Leave 0 if no connection is desired.
- Returns
- new action of the given type ActionType.
Definition at line 343 of file kactioncollection.cpp.
KAction * KActionCollection::addAction | ( | const QString & | name, |
const QObject * | receiver = 0 , |
||
const char * | member = 0 |
||
) |
Creates a new action under the given name to the collection and connects the action's triggered(bool) signal to the specified receiver/member.
The newly created action is returned.
NOTE: KDE prior to 4.2 used the triggered() signal instead of the triggered(bool) signal.
Inserting an action that was previously inserted under a different name will replace the old entry, i.e. the action will not be available under the old name anymore but only under the new one.
Inserting an action under a name that is already used for another action will replace the other action in the collection.
- Parameters
-
name The name by which the action be retrieved again from the collection. receiver The QObject to connect the triggered(bool) signal to. Leave 0 if no connection is desired. member The SLOT to connect the triggered(bool) signal to. Leave 0 if no connection is desired.
- Returns
- new action of the given type ActionType.
Definition at line 358 of file kactioncollection.cpp.
void KActionCollection::addAssociatedWidget | ( | QWidget * | widget | ) |
Associate all actions in this collection to the given widget, including any actions added after this association is made.
This does not change the action's shortcut context, so if you need to have the actions only trigger when the widget has focus, you'll need to set the shortcut context on each action to Qt::WidgetShortcut (or better still, Qt::WidgetWithChildrenShortcut with Qt 4.4+)
Definition at line 708 of file kactioncollection.cpp.
|
static |
Access the list of all action collections in existence for this app.
Definition at line 695 of file kactioncollection.cpp.
Return a list of all associated widgets.
Definition at line 761 of file kactioncollection.cpp.
void KActionCollection::associateWidget | ( | QWidget * | widget | ) | const |
Associate all actions in this collection to the given widget.
Unlike addAssociatedWidget, this method only adds all current actions in the collection to the given widget. Any action added after this call will not be added to the given widget automatically. So this is just a shortcut for a foreach loop and a widget->addAction call.
Definition at line 700 of file kactioncollection.cpp.
void KActionCollection::clear | ( | ) |
Clears the entire action collection, deleting all actions.
Definition at line 124 of file kactioncollection.cpp.
void KActionCollection::clearAssociatedWidgets | ( | ) |
Clear all associated widgets and remove the actions from those widgets.
Definition at line 766 of file kactioncollection.cpp.
KComponentData KActionCollection::componentData | ( | ) | const |
The KComponentData with which this class is associated.
Definition at line 176 of file kactioncollection.cpp.
QString KActionCollection::configGroup | ( | ) | const |
Returns the KConfig group with which settings will be loaded and saved.
bool KActionCollection::configIsGlobal | ( | ) | const |
Returns whether this action collection's configuration should be global to KDE ( true ), or specific to the application ( false ).
|
protectedvirtualslot |
Overridden to perform connections when someone wants to know whether an action was highlighted or triggered.
Definition at line 671 of file kactioncollection.cpp.
int KActionCollection::count | ( | ) | const |
Returns the number of actions in the collection.
This is equivalent to actions().count().
Definition at line 147 of file kactioncollection.cpp.
void KActionCollection::exportGlobalShortcuts | ( | KConfigGroup * | config, |
bool | writeDefaults = false |
||
) | const |
Export the current configurable global key associations to config
.
- Since
- 4.1
- Parameters
-
config Config object to save to writeDefaults set to true to write settings which are already at defaults.
Definition at line 444 of file kactioncollection.cpp.
void KActionCollection::importGlobalShortcuts | ( | KConfigGroup * | config | ) |
Import from config
all configurable global key associations.
- Since
- 4.1
- Parameters
-
config Config object to read from
Definition at line 386 of file kactioncollection.cpp.
|
signal |
Indicates that action was inserted into this action collection.
bool KActionCollection::isEmpty | ( | ) | const |
Returns whether the action collection is empty or not.
Definition at line 152 of file kactioncollection.cpp.
const KXMLGUIClient * KActionCollection::parentGUIClient | ( | ) | const |
The parent KXMLGUIClient, or null if not available.
Definition at line 181 of file kactioncollection.cpp.
void KActionCollection::readSettings | ( | KConfigGroup * | config = 0 | ) |
Read all key associations from config
.
If config
is zero, read all key associations from the application's configuration file KGlobal::config(), in the group set by setConfigGroup().
Definition at line 413 of file kactioncollection.cpp.
void KActionCollection::removeAction | ( | QAction * | action | ) |
Removes an action from the collection and deletes it.
- Parameters
-
action The action to remove.
Definition at line 316 of file kactioncollection.cpp.
void KActionCollection::removeAssociatedWidget | ( | QWidget * | widget | ) |
Remove an association between all actions in this collection and the given widget, i.e.
remove those actions from the widget, and stop associating newly added actions as well.
Definition at line 718 of file kactioncollection.cpp.
|
signal |
Indicates that action was removed from this action collection.
void KActionCollection::setComponentData | ( | const KComponentData & | componentData | ) |
Set the componentData associated with this action collection.
- Warning
- Don't call this method on a KActionCollection that contains actions. This is not supported.
- Parameters
-
componentData the KComponentData which is to be associated with this action collection, or an invalid KComponentData instance to indicate the default KComponentData.
Definition at line 157 of file kactioncollection.cpp.
void KActionCollection::setConfigGlobal | ( | bool | global | ) |
Set whether this action collection's configuration should be global to KDE ( true ), or specific to the application ( false ).
Definition at line 381 of file kactioncollection.cpp.
void KActionCollection::setConfigGroup | ( | const QString & | group | ) |
Sets group as the KConfig group with which settings will be loaded and saved.
Definition at line 371 of file kactioncollection.cpp.
|
protectedvirtualslot |
- Deprecated:
- Replaced by slotActionHovered();
Definition at line 643 of file kactioncollection.cpp.
|
protectedvirtualslot |
Definition at line 636 of file kactioncollection.cpp.
Removes an action from the collection.
- Parameters
-
action the action to remove.
Definition at line 321 of file kactioncollection.cpp.
void KActionCollection::writeSettings | ( | KConfigGroup * | config = 0 , |
bool | writeDefaults = false , |
||
QAction * | oneAction = 0 |
||
) | const |
Write the current configurable key associations to config.
What the function does if config is zero depends. If this action collection belongs to a KXMLGuiClient the setting are saved to the kxmlgui definition file. If not the settings are written to the applications config file.
- Note
- oneAction() and writeDefaults() have no meaning for the kxmlgui configuration file.
- Parameters
-
config Config object to save to, or null (see above) writeDefaults set to true to write settings which are already at defaults. oneAction pass an action here if you just want to save the values for one action, eg. if you know that action is the only one which has changed.
Definition at line 563 of file kactioncollection.cpp.
Property Documentation
|
readwrite |
Definition at line 62 of file kactioncollection.h.
|
readwrite |
Definition at line 63 of file kactioncollection.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.