KDEUI
#include <knotification.h>
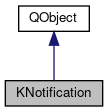
Public Types | |
typedef QPair< QString, QString > | Context |
typedef QList< Context > | ContextList |
enum | NotificationFlag { RaiseWidgetOnActivation =0x01, CloseOnTimeout =0x00, Persistent =0x02, CloseWhenWidgetActivated =0x04, Persistant = Persistent, DefaultEvent =0xF000 } |
enum | StandardEvent { Notification, Warning, Error, Catastrophe } |
Public Slots | |
void | activate (unsigned int action=0) |
void | close () |
void | deref () |
void | raiseWidget () |
void | ref () |
void | sendEvent () |
void | update () |
Signals | |
void | action1Activated () |
void | action2Activated () |
void | action3Activated () |
void | activated () |
void | activated (unsigned int action) |
void | closed () |
void | ignored () |
Public Member Functions | |
KNotification (const QString &eventId, QWidget *widget=0L, const NotificationFlags &flags=CloseOnTimeout) | |
KNotification (const QString &eventId, const NotificationFlags &flags, QObject *parent=NULL) | |
~KNotification () | |
QStringList | actions () const |
void | addContext (const Context &context) |
void | addContext (const QString &context_key, const QString &context_value) |
ContextList | contexts () const |
QString | eventId () const |
NotificationFlags | flags () const |
QPixmap | pixmap () const |
void | setActions (const QStringList &actions) |
void | setComponentData (const KComponentData &componentData) |
void | setContexts (const ContextList &contexts) |
void | setFlags (const NotificationFlags &flags) |
void | setPixmap (const QPixmap &pix) |
void | setText (const QString &text) |
void | setTitle (const QString &title) |
void | setWidget (QWidget *widget) |
QString | text () const |
QString | title () const |
QWidget * | widget () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static void | beep (const QString &reason=QString(), QWidget *widget=0L) |
static KNotification * | event (const QString &eventId, const QString &title, const QString &text, const QPixmap &pixmap=QPixmap(), QWidget *widget=0L, const NotificationFlags &flags=CloseOnTimeout, const KComponentData &componentData=KComponentData()) |
static KNotification * | event (const QString &eventId, const QString &text=QString(), const QPixmap &pixmap=QPixmap(), QWidget *widget=0L, const NotificationFlags &flags=CloseOnTimeout, const KComponentData &componentData=KComponentData()) |
static KNotification * | event (StandardEvent eventId, const QString &text=QString(), const QPixmap &pixmap=QPixmap(), QWidget *widget=0L, const NotificationFlags &flags=CloseOnTimeout) |
static KNotification * | event (StandardEvent eventId, const QString &title, const QString &text, const QPixmap &pixmap=QPixmap(), QWidget *widget=0L, const NotificationFlags &flags=CloseOnTimeout) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
KNotification is used to notify the user of an event.
introduction
There are two main kinds of notifications:
- Feedback events: For notifying the user that he/she just performed an operation, like maximizing a window. This allows us to play sounds when a dialog appears. This is an instant notification. It ends automatically after a small timeout.
- persistant notifications: Notify when the user received a new message, or when something else important happened the user has to know about. This notification has a start and a end. It begins when the event actually occurs, and finishes when the message is acknowledged or read.
Example of a persistent notification in an instant messaging application: The application emits the notification when the message is actually received, and closes it only when the user has read the message (when the message window has received the focus) using the close() slot Persistent notifications must have the Persistent flag.
By default a notification will use the application name as title, but you can also provide a brief text in the title and a more precise description in the body text. This is especially useful for notifications coming from applications which should be considered "part of the system", like a battery monitor or a network connection manager. For example a battery indicator could use "Low Battery" as a title and "Only 12 minutes left." as a body text.
In order to perform a notification, you need to create a description file, which contains default parameters of the notification, and use KNotification::event at the place in the application code where the notification occurs. The returned KNotification pointer may be used to connect signals or slots
The global config file
Your application should install a file called: $KDEDIR/share/apps/appname/appname.notifyrc
You can do this with the following CMake command: install( FILES appname.notifyrc DESTINATION ${DATA_INSTALL_DIR}/appname))
This file contains mainly 3 parts
Global information
The global part looks like that
[Global] IconName=Filename Comment=Friendly Name of app Name=Name of app
The icon filename is just the name, without extension, it's found with the KIconLoader. The Comment field will be used in KControl to describe the application. The Name field is optional and may be used as the application name for popup, if Name is not present, Comment is used instead
Context information
This part consists of hints for the configuration widget
[Context/group] Name=Group name Comment=The name of the group for contacts
[Context/folder] Name=Group name
The second part of the groupname is the context identifier. It should not contain special characters. The Name field is the one the user will see (and which is translated)
Definition of Events
The definition of the events forms the most important part of the config file
[Event/newmail] Name=New email Comment=You have got a new email Contexts=folder,group Action=Sound|Popup
[Event/contactOnline] Name=Contact goes online Comment=One of your contact has been connected Contexts=group Sound=filetoplay.ogg Action=None
These are the default settings for each notifiable event. Action is the string representing the action. Actions can be added to the KNotify daemon as plugins, by deriving from KNotifyPlugin. At the time of writing, the following actions are available: Taskbar, Sound, Popup, Logfile, KTTS, Execute. Actions can be combined by seperating them with '|'.
Contexts is a comma separated list of possible context for this event.
The user's config file
This is an implementation detail, and is described here for your information.
In the config file, there are two parts: the event configuration, and the context information
Context information
These are hints for the configuration dialog. They contain both the internal id of the context, and the user visible string.
[Context/group] Values=1:Friends,2:Work,3:Family
Events configuration
This contains the configuration of events for the user. It contains the same fields as the description file. The key of groups is in the form Event/<EventName>/<ContextName>/<ContextValue>
[Event/contactOnline] Action=Sound Sound=/usr/share/sounds/super.ogg
[Event/contactOnline/group/1] Action=Popup|Sound
Example of code
This portion of code will fire the event for the "contactOnline" event
Definition at line 180 of file knotification.h.
Member Typedef Documentation
Sometimes the user may want different notifications for the same event, depending the source of the event.
Example, you want to be notified for mails that arrive in your folder "personal inbox" but not for those in "spam" folder
A notification context is a pair of two strings. The first string is a key from what the context is. example "group" or "filter" (not translated). The second is the id of the context. In our example, the group id or the filter id in the applications. These strings are the ones present in the config file, and are in theory not shown in the user interface.
The order of contexts in the list is is important, the most important context should be placed first. They are processed in that order when the notification occurs.
- See also
- event
Definition at line 203 of file knotification.h.
Definition at line 204 of file knotification.h.
Member Enumeration Documentation
Enumerator | |
---|---|
RaiseWidgetOnActivation |
When the notification is activated, raise the notification's widget. This will change the desktop, raise the window, and switch to the tab.
|
CloseOnTimeout |
The notification will be automatically closed after a timeout. (this is the default) |
Persistent |
The notification will NOT be automatically closed after a timeout. You will have to track the notification, and close it with the close function manually when the event is done, otherwise there will be a memory leak |
CloseWhenWidgetActivated |
The notification will be automatically closed if the widget() becomes activated. If the widget is already activated when the notification occurs, the notification will be closed after a small timeout. This only works if the widget is the toplevel widget
|
Persistant |
DEPRECATED - Misspelling of 'Persistent'. Do not use in new code. |
DefaultEvent |
The event is a standard kde event, and not an event of the application |
Definition at line 206 of file knotification.h.
default events you can use in the event function
Enumerator | |
---|---|
Notification | |
Warning | |
Error | |
Catastrophe |
Definition at line 256 of file knotification.h.
Constructor & Destructor Documentation
|
explicit |
Create a new notification.
You have to use sendEvent to show the notification.
The pointer is automatically deleted when the event is closed.
Make sure you use one of the NotificationFlags CloseOnTimeOut or CloseWhenWidgetActivated, if not, you have to close the notification yourself.
- Parameters
-
eventId is the name of the event widget is a widget where the notification reports to flags is a bitmask of NotificationFlag
Definition at line 82 of file knotification.cpp.
|
explicit |
Create a new notification.
You have to use sendEvent to show the notification.
The pointer is automatically deleted when the event is closed.
Make sure you use one of the NotificationFlags CloseOnTimeOut or CloseWhenWidgetActivated, if not, you have to close the notification yourself.
- Since
- 4.4
- Parameters
-
eventId is the name of the event flags is a bitmask of NotificationFlag parent parent object
Definition at line 93 of file knotification.cpp.
KNotification::~KNotification | ( | ) |
Definition at line 108 of file knotification.cpp.
Member Function Documentation
|
signal |
Convenience signal that is emitted when the first action is activated.
|
signal |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
|
signal |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
QStringList KNotification::actions | ( | ) | const |
- Returns
- the list of actions
Definition at line 173 of file knotification.cpp.
|
slot |
Activate the action specified action If the action is zero, then the default action is activated.
Definition at line 222 of file knotification.cpp.
|
signal |
Emit only when the default activation has occurred.
|
signal |
Emit when an action has been activated.
- Parameters
-
action will be 0 is the default aciton was activated, or any action id
void KNotification::addContext | ( | const Context & | context | ) |
append a context at the list of contexts, see KNotificaiton::Context
- Parameters
-
context the context which is added
Definition at line 196 of file knotification.cpp.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
context_key is the key of the context context_value is the value of the context
Definition at line 201 of file knotification.cpp.
This is a simple substitution for QApplication::beep()
- Parameters
-
reason a short text explaining what has happened (may be empty) widget the widget the notification refers to
Definition at line 352 of file knotification.cpp.
|
slot |
Close the notification without activating it.
This will delete the notification.
Definition at line 246 of file knotification.cpp.
|
signal |
Emitted when the notification is closed.
Both when it is activated or if it is just ignored.
KNotification::ContextList KNotification::contexts | ( | ) | const |
- Returns
- the list of contexts, see KNotification::Context
Definition at line 186 of file knotification.cpp.
|
slot |
remove a reference made with ref() the notification may be closed when calling this.
- See also
- ref
Definition at line 345 of file knotification.cpp.
|
static |
emit an event
This method creates the KNotification, setting every parameter, and fire the event. You don't need to call sendEvent
A popup may be displayed or a sound may be played, depending the config.
- Returns
- a KNotification . You may use that pointer to connect some signals or slot. the pointer is automatically deleted when the event is closed.
Make sure you use one of the CloseOnTimeOut or CloseWhenWidgetActivated, if not, you have to close yourself the notification.
- Note
- the text is shown in a QLabel, you should escape HTML, if needed.
- Parameters
-
eventId is the name of the event title is title of the notification to show in the popup. text is the text of the notification to show in the popup. pixmap is a picture which may be shown in the popup. widget is a widget where the notification reports to flags is a bitmask of NotificationFlag componentData used to determine the location of the config file. by default, kapp is used
- Since
- 4.4
Definition at line 291 of file knotification.cpp.
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event text is the text of the notification to show in the popup. pixmap is a picture which may be shown in the popup. widget is a widget where the notification reports to flags is a bitmask of NotificationFlag componentData used to determine the location of the config file. by default, kapp is used
Definition at line 305 of file knotification.cpp.
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event text is the text of the notification to show in the popup pixmap is a picture which may be shown in the popup widget is a widget where the notification reports to flags is a bitmask of NotificationFlag
Definition at line 334 of file knotification.cpp.
|
static |
emit a standard event
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This will emit a standard event
- Parameters
-
eventId is the name of the event title is title of the notification to show in the popup. text is the text of the notification to show in the popup pixmap is a picture which may be shown in the popup widget is a widget where the notification reports to flags is a bitmask of NotificationFlag
- Since
- 4.4
Definition at line 312 of file knotification.cpp.
reimplemented for internal reasons
Reimplemented from QObject.
Definition at line 427 of file knotification.cpp.
QString KNotification::eventId | ( | ) | const |
- Returns
- the name of the event
Definition at line 115 of file knotification.cpp.
KNotification::NotificationFlags KNotification::flags | ( | ) | const |
- Returns
- the notification flags.
Definition at line 206 of file knotification.cpp.
|
signal |
The notification has been ignored.
QPixmap KNotification::pixmap | ( | ) | const |
- Returns
- the pixmap shown in the popup
- See also
- setPixmap
Definition at line 160 of file knotification.cpp.
|
slot |
Raise the widget.
This will change the desktop, activate the window, and the tab if needed.
Definition at line 257 of file knotification.cpp.
|
slot |
The notification will automatically be closed if all presentations are finished.
if you want to show your own presentation in your application, you should use this function, so it will not be automatically closed when there is nothing to show.
don't forgot to deref, or the notification may be never closed if there is no timeout.
- See also
- ref
Definition at line 340 of file knotification.cpp.
|
slot |
Emit or re-emit the event.
Definition at line 357 of file knotification.cpp.
void KNotification::setActions | ( | const QStringList & | actions | ) |
Set the list of actions link shown in the popup.
- Parameters
-
actions the list of actions
Definition at line 178 of file knotification.cpp.
void KNotification::setComponentData | ( | const KComponentData & | componentData | ) |
The componentData is used to determine the location of the config file.
By default, kapp is used
- Parameters
-
componentData the new componentData
Definition at line 217 of file knotification.cpp.
void KNotification::setContexts | ( | const ContextList & | contexts | ) |
set the list of contexts, see KNotification::Context
The list of contexts must be set before calling sendEvent;
Definition at line 191 of file knotification.cpp.
void KNotification::setFlags | ( | const NotificationFlags & | flags | ) |
Set the notification flags.
should be called before sendEvent().
Definition at line 211 of file knotification.cpp.
void KNotification::setPixmap | ( | const QPixmap & | pix | ) |
set the pixmap that will be shown in the popup.
- Parameters
-
pix the pixmap
Definition at line 165 of file knotification.cpp.
void KNotification::setText | ( | const QString & | text | ) |
Set the notification text that will appear in the popup.
The text is shown in a QLabel, you should make sure to escape any html that is needed. You can use some of the qt basic html tags.
- Parameters
-
text the text
Definition at line 152 of file knotification.cpp.
void KNotification::setTitle | ( | const QString & | title | ) |
Set the title of the notification popup.
If no title is set, the application name will be used.
- Parameters
-
title the title
- Since
- 4.3
Definition at line 144 of file knotification.cpp.
void KNotification::setWidget | ( | QWidget * | widget | ) |
Set the widget associated to the notification.
The notification is reparented to the new widget.
- See also
- widget()
- Parameters
-
widget the new widget
Definition at line 135 of file knotification.cpp.
QString KNotification::text | ( | ) | const |
QString KNotification::title | ( | ) | const |
- Returns
- the notification title
- See also
- setTitle
- Since
- 4.3
Definition at line 120 of file knotification.cpp.
|
slot |
update the texts, the icon, and the actions of one existing notification
Definition at line 422 of file knotification.cpp.
QWidget * KNotification::widget | ( | ) | const |
the widget associated to the notification
If the widget is destroyed, the notification will be automatically cancelled. If the widget is activated, the notification will be automatically closed if the NotificationFlags specify that
When the notification is activated, the widget might be raised. Depending on the configuration, the taskbar entry of the window containing the widget may blink.
Definition at line 130 of file knotification.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.