KDEUI
#include <kpushbutton.h>
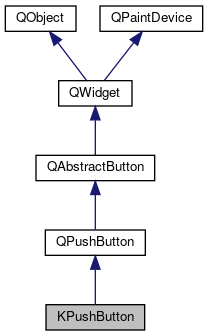
Signals | |
void | authorized (KAuth::Action *action) |
Public Member Functions | |
KPushButton (QWidget *parent=0) | |
KPushButton (const QString &text, QWidget *parent=0) | |
KPushButton (const KIcon &icon, const QString &text, QWidget *parent=0) | |
KPushButton (const KGuiItem &item, QWidget *parent=0) | |
~KPushButton () | |
KAuth::Action * | authAction () const |
QMenu * | delayedMenu () |
KStandardGuiItem::StandardItem | guiItem () const |
bool | isDragEnabled () const |
void | setAuthAction (KAuth::Action *action) |
void | setAuthAction (const QString &actionName) |
void | setDelayedMenu (QMenu *delayed_menu) |
void | setDragEnabled (bool enable) |
void | setGuiItem (const KGuiItem &item) |
void | setGuiItem (KStandardGuiItem::StandardItem item) |
void | setIcon (const KIcon &icon) |
void | setIcon (const QIcon &pix) |
void | setText (const QString &text) |
virtual QSize | sizeHint () const |
![]() | |
QPushButton (QWidget *parent) | |
QPushButton (const QString &text, QWidget *parent) | |
QPushButton (QWidget *parent, const char *name) | |
QPushButton (const QString &text, QWidget *parent, const char *name) | |
QPushButton (const QIcon &icon, const QString &text, QWidget *parent, const char *name) | |
QPushButton (const QIcon &icon, const QString &text, QWidget *parent) | |
~QPushButton () | |
bool | autoDefault () const |
bool | isDefault () const |
bool | isFlat () const |
bool | isMenuButton () const |
QMenu * | menu () const |
virtual QSize | minimumSizeHint () const |
void | openPopup () |
QMenu * | popup () const |
void | setAutoDefault (bool) |
void | setDefault (bool) |
void | setFlat (bool) |
void | setMenu (QMenu *menu) |
void | setPopup (QMenu *popup) |
void | showMenu () |
![]() | |
QAbstractButton (QWidget *parent) | |
QAbstractButton (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QAbstractButton () | |
QKeySequence | accel () const |
void | animateClick (int msec) |
bool | autoExclusive () const |
bool | autoRepeat () const |
int | autoRepeatDelay () const |
int | autoRepeatInterval () const |
void | click () |
void | clicked (bool checked) |
QButtonGroup * | group () const |
QIcon | icon () const |
QIcon * | iconSet () const |
QSize | iconSize () const |
bool | isCheckable () const |
bool | isChecked () const |
bool | isDown () const |
bool | isOn () const |
bool | isToggleButton () const |
const QPixmap * | pixmap () const |
void | pressed () |
void | released () |
void | setAccel (const QKeySequence &key) |
void | setAutoExclusive (bool) |
void | setAutoRepeat (bool) |
void | setAutoRepeatDelay (int) |
void | setAutoRepeatInterval (int) |
void | setCheckable (bool) |
void | setChecked (bool) |
void | setDown (bool) |
void | setIcon (const QIcon &icon) |
void | setIconSet (const QIcon &icon) |
void | setIconSize (const QSize &size) |
void | setOn (bool b) |
void | setPixmap (const QPixmap &p) |
void | setShortcut (const QKeySequence &key) |
void | setText (const QString &text) |
void | setToggleButton (bool b) |
QKeySequence | shortcut () const |
QString | text () const |
void | toggle () |
void | toggled (bool checked) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual QDrag * | dragObject () |
virtual void | mouseMoveEvent (QMouseEvent *) |
virtual void | mousePressEvent (QMouseEvent *) |
virtual void | paintEvent (QPaintEvent *) |
virtual void | startDrag () |
![]() | |
virtual bool | event (QEvent *e) |
virtual void | focusInEvent (QFocusEvent *e) |
virtual void | focusOutEvent (QFocusEvent *e) |
virtual bool | hitButton (const QPoint &pos) const |
void | initStyleOption (QStyleOptionButton *option) const |
virtual void | keyPressEvent (QKeyEvent *e) |
![]() | |
virtual void | changeEvent (QEvent *e) |
virtual void | checkStateSet () |
virtual void | keyReleaseEvent (QKeyEvent *e) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
virtual void | nextCheckState () |
virtual void | timerEvent (QTimerEvent *e) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A QPushButton with drag-support and KGuiItem support.
This is nothing but a QPushButton with drag-support and KGuiItem support. You must call setDragEnabled (true) and override the virtual method dragObject() to specify the QDragObject to be used.
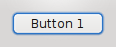
Definition at line 46 of file kpushbutton.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor.
Definition at line 152 of file kpushbutton.cpp.
Constructor, that sets the button-text to text
.
Definition at line 158 of file kpushbutton.cpp.
Constructor, that sets an icon and the button-text to text
.
Definition at line 164 of file kpushbutton.cpp.
Constructor that takes a KGuiItem for the text, the icon, the tooltip and the what's this help.
Definition at line 171 of file kpushbutton.cpp.
KPushButton::~KPushButton | ( | ) |
Destructs the button.
Definition at line 177 of file kpushbutton.cpp.
Member Function Documentation
KAuth::Action * KPushButton::authAction | ( | ) | const |
Returns the action object associated with this button, or 0 if it does not have one.
- Returns
- the KAuth::Action associated with this button.
Definition at line 317 of file kpushbutton.cpp.
|
signal |
Signal emitted when the button is triggered and authorized.
If the button needs authorization, whenever the user triggers it, the authorization process automatically begins. If it succeeds, this signal is emitted. The KAuth::Action object is provided for convenience if you have multiple Action objects, but of course it's always the same set with setAuthAction().
WARNING: If your button needs authorization you should connect eventual slots processing stuff to this signal, and NOT clicked. Clicked will be emitted even if the user has not been authorized
- Parameters
-
action The object set with setAuthAction()
QMenu * KPushButton::delayedMenu | ( | ) |
returns a delayed popup menu since menu() isn't virtual
Definition at line 312 of file kpushbutton.cpp.
|
protectedvirtual |
Reimplement this and return the QDrag object that should be used for the drag.
Remember to give it "this" as parent.
Default implementation returns 0, so that no drag is initiated.
Definition at line 295 of file kpushbutton.cpp.
KStandardGuiItem::StandardItem KPushButton::guiItem | ( | ) | const |
Reads the standard KGuiItem for this button.
Definition at line 234 of file kpushbutton.cpp.
bool KPushButton::isDragEnabled | ( | ) | const |
- Returns
- if drag support is enabled or not.
|
protectedvirtual |
Reimplemented to add drag-support.
Reimplemented from QAbstractButton.
Definition at line 278 of file kpushbutton.cpp.
|
protectedvirtual |
Reimplemented to add drag-support.
Reimplemented from QAbstractButton.
Definition at line 271 of file kpushbutton.cpp.
|
protectedvirtual |
Reimplemented to add arrow for delayed menu.
- Since
- 4.4
Reimplemented from QPushButton.
Definition at line 371 of file kpushbutton.cpp.
void KPushButton::setAuthAction | ( | KAuth::Action * | action | ) |
Sets the action object associated with this button.
By setting a KAuth::Action, this button will become associated with it, and whenever it gets clicked, it will trigger the authorization and execution process for the action. The signal activated will also be emitted whenever the button gets clicked and the action gets authorized. Pass 0 to this function to disassociate the button
- Parameters
-
action the KAuth::Action to associate with this button.
Definition at line 331 of file kpushbutton.cpp.
void KPushButton::setAuthAction | ( | const QString & | actionName | ) |
Sets the action object associated with this button.
Overloaded member to allow creating the action by name
- Parameters
-
actionName the name of the action to associate
Definition at line 322 of file kpushbutton.cpp.
void KPushButton::setDelayedMenu | ( | QMenu * | delayed_menu | ) |
Sets a delayed popup menu for consistency, since menu() isn't virtual.
Definition at line 307 of file kpushbutton.cpp.
void KPushButton::setDragEnabled | ( | bool | enable | ) |
void KPushButton::setGuiItem | ( | const KGuiItem & | item | ) |
Sets the KGuiItem for this button.
Definition at line 215 of file kpushbutton.cpp.
void KPushButton::setGuiItem | ( | KStandardGuiItem::StandardItem | item | ) |
Sets the standard KGuiItem for this button.
Definition at line 228 of file kpushbutton.cpp.
void KPushButton::setIcon | ( | const KIcon & | icon | ) |
Sets the Icon Set for this button.
It also takes into account the KGlobalSettings::showIconsOnPushButtons() setting.
Definition at line 251 of file kpushbutton.cpp.
void KPushButton::setIcon | ( | const QIcon & | pix | ) |
Sets the pixmap for this button.
This one exists mostly for usage in Qt designer.
Definition at line 261 of file kpushbutton.cpp.
void KPushButton::setText | ( | const QString & | text | ) |
Sets the text of the button.
Definition at line 239 of file kpushbutton.cpp.
|
virtual |
Reimplemented to add arrow for delayed menu.
- Since
- 4.4
Reimplemented from QPushButton.
Definition at line 360 of file kpushbutton.cpp.
|
protectedvirtual |
Starts a drag (dragCopy() by default) using dragObject()
Definition at line 300 of file kpushbutton.cpp.
Property Documentation
|
readwrite |
Definition at line 49 of file kpushbutton.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.