KDEUI
#include <kglobalsettings.h>
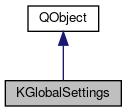
Classes | |
struct | KMouseSettings |
Public Types | |
enum | ActivateOption { ApplySettings = 0x1, ListenForChanges = 0x2 } |
enum | ChangeType { PaletteChanged = 0, FontChanged, StyleChanged, SettingsChanged, IconChanged, CursorChanged, ToolbarStyleChanged, ClipboardConfigChanged, BlockShortcuts, NaturalSortingChanged } |
enum | Completion { CompletionNone =1, CompletionAuto, CompletionMan, CompletionShell, CompletionPopup, CompletionPopupAuto } |
enum | GraphicEffect { NoEffects = 0x0000, GradientEffects = 0x0001, SimpleAnimationEffects = 0x0002, ComplexAnimationEffects = 0x0006 } |
enum | SettingsCategory { SETTINGS_MOUSE, SETTINGS_COMPLETION, SETTINGS_PATHS, SETTINGS_POPUPMENU, SETTINGS_QT, SETTINGS_SHORTCUTS, SETTINGS_LOCALE, SETTINGS_STYLE } |
enum | TearOffHandle { Disable = 0, ApplicationLevel, Enable } |
Signals | |
void | appearanceChanged () |
void | blockShortcuts (int data) |
void | cursorChanged () |
void | iconChanged (int group) |
void | kdisplayFontChanged () |
void | kdisplayPaletteChanged () |
void | kdisplayStyleChanged () |
void | naturalSortingChanged () |
void | settingsChanged (int category) |
void | toolbarAppearanceChanged (int) |
Public Member Functions | |
~KGlobalSettings () | |
void | activate () |
void | activate (ActivateOptions options) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Access the KDE global configuration.
Definition at line 58 of file kglobalsettings.h.
Member Enumeration Documentation
Specifies options passed to activate().
- Since
- 4.6
Enumerator | |
---|---|
ApplySettings |
Make all globally applicable settings take effect. |
ListenForChanges |
Listen for changes to the settings. |
Definition at line 567 of file kglobalsettings.h.
An identifier for change signals.
- See also
- emitChange
Enumerator | |
---|---|
PaletteChanged | |
FontChanged | |
StyleChanged | |
SettingsChanged | |
IconChanged | |
CursorChanged | |
ToolbarStyleChanged | |
ClipboardConfigChanged | |
BlockShortcuts | |
NaturalSortingChanged |
Definition at line 543 of file kglobalsettings.h.
This enum describes the completion mode used for by the KCompletion class.
See the styleguide.
Definition at line 179 of file kglobalsettings.h.
Definition at line 464 of file kglobalsettings.h.
Valid values for the settingsChanged signal.
Enumerator | |
---|---|
SETTINGS_MOUSE | |
SETTINGS_COMPLETION | |
SETTINGS_PATHS | |
SETTINGS_POPUPMENU | |
SETTINGS_QT | |
SETTINGS_SHORTCUTS | |
SETTINGS_LOCALE | |
SETTINGS_STYLE |
Definition at line 593 of file kglobalsettings.h.
This enum describes the return type for insertTearOffHandle() whether to insert a handle or not.
Applications who independently want to use handles in their popup menus should test for Application level before calling the appropriate function in KMenu.
Enumerator | |
---|---|
Disable |
disable tear-off handles |
ApplicationLevel |
enable on application level |
Enable |
enable tear-off handles |
Definition at line 130 of file kglobalsettings.h.
Constructor & Destructor Documentation
KGlobalSettings::~KGlobalSettings | ( | ) |
Definition at line 199 of file kglobalsettings.cpp.
Member Function Documentation
void KGlobalSettings::activate | ( | ) |
Makes all globally applicable settings take effect and starts listening for changes to these settings.
This is usually called only by the KApplication constructor.
- Since
- 4.3.3
Definition at line 204 of file kglobalsettings.cpp.
void KGlobalSettings::activate | ( | ActivateOptions | options | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 4.6
Definition at line 209 of file kglobalsettings.cpp.
|
static |
The default color to use for active texts.
- Returns
- the active text color
Definition at line 350 of file kglobalsettings.cpp.
|
static |
The default color to use for active titles.
- Returns
- the active title color
Definition at line 339 of file kglobalsettings.cpp.
|
static |
Returns if default background images are allowed by the color scheme.
A "default" background image is just that, i.e. the user has not actively selected a background image to use.
- Returns
- true if default background images may be used
Definition at line 381 of file kglobalsettings.cpp.
|
signal |
Emitted when the application has changed either its GUI style, its font or its palette in response to a kdisplay request.
Normally, widgets will update their styles automatically, but you should connect to this to program special behavior.
|
static |
Returns the KDE setting for the auto-select option.
- Returns
- the auto-select delay or -1 if auto-select is disabled.
Definition at line 261 of file kglobalsettings.cpp.
|
static |
The path to the autostart directory of the current user.
- Returns
- the path of the autostart directory
Definition at line 633 of file kglobalsettings.cpp.
|
signal |
Emitted by BlockShortcuts.
|
static |
The layout scheme to use for dialog buttons.
- Returns
- Returns the number of the scheme to use.
Definition at line 819 of file kglobalsettings.cpp.
|
static |
Checks whether the cursor changes over icons.
- Returns
- the KDE setting for "change cursor over icon"
Definition at line 255 of file kglobalsettings.cpp.
|
static |
Returns the preferred completion mode setting.
- Returns
- Completion. Default is
CompletionPopup
.
Definition at line 267 of file kglobalsettings.cpp.
|
static |
Returns the KDE setting for the shortcut key to open context menus.
- Returns
- the key that pops up context menus.
- Deprecated:
- Simply reimplement QWidget::contextMenuEvent() instead.
Definition at line 287 of file kglobalsettings.cpp.
|
static |
Returns the contrast for borders.
- Returns
- the contrast (between 0 for minimum and 10 for maximum contrast)
Definition at line 360 of file kglobalsettings.cpp.
|
static |
Returns the contrast for borders as a floating point value.
- Parameters
-
config pointer to the config from which to read the contrast setting (the default is to use KGlobal::config())
- Returns
- the contrast (between 0.0 for minimum and 1.0 for maximum contrast)
Definition at line 366 of file kglobalsettings.cpp.
|
static |
Used to obtain the QPalette that will be used to set the application palette.
This is only useful for configuration modules such as krdb and should not be used in normal circumstances.
- Parameters
-
config KConfig from which to load the colors (passed as-is to KColorScheme).
- Returns
- the QPalette
Definition at line 975 of file kglobalsettings.cpp.
|
static |
Used to obtain the QPalette that will be used to set the application palette.
This is only useful for configuration modules such as krdb and should not be used in normal circumstances.
- Parameters
-
config KConfig from which to load the colors (passed as-is to KColorScheme).
- Note
- The difference between this and the previous is that this never caches.
- Since
- 4.6.3
- Returns
- the QPalette
Definition at line 980 of file kglobalsettings.cpp.
|
signal |
Emitted when the cursor theme has been changed.
This function returns the desktop geometry for an application that needs to set the geometry of a widget on the screen manually.
It takes into account the user's display settings (number of screens, Xinerama, etc), and the user's preferences (if KDE should be Xinerama aware).
Note that this can break in multi-head (not Xinerama) mode because this point could be on multiple screens. Use with care.
- Parameters
-
point a reference point for the widget, for instance one that the widget should be adjacent or on top of.
- Returns
- the geometry to use for the desktop. Note that it might not start at (0,0).
Definition at line 732 of file kglobalsettings.cpp.
This function returns the desktop geometry for an application that needs to set the geometry of a widget on the screen manually.
It takes into account the user's display settings (number of screens, Xinerama, etc), and the user's preferences (if KDE should be Xinerama aware).
- Parameters
-
w the widget in question. This is used to determine which screen to use in Xinerama or multi-head mode.
- Returns
- the geometry to use for the desktop. Note that it might not start at (0,0).
Definition at line 749 of file kglobalsettings.cpp.
|
static |
The path to the desktop directory of the current user.
- Returns
- the user's desktop directory
Definition at line 626 of file kglobalsettings.cpp.
|
static |
Returns a threshold in pixels for drag & drop operations.
As long as the mouse movement has not exceeded this number of pixels in either X or Y direction no drag operation may be started. This prevents spurious drags when the user intended to click on something but moved the mouse a bit while doing so.
For this to work you must save the position of the mouse (oldPos) in the QWidget::mousePressEvent(). When the position of the mouse (newPos) in a QWidget::mouseMoveEvent() exceeds this threshold you may start a drag which should originate from oldPos.
Example code:
- Returns
- the threshold for drag & drop in pixels
Definition at line 227 of file kglobalsettings.cpp.
|
static |
The path where documents are stored of the current user.
- Returns
- the path of the document directory
Definition at line 646 of file kglobalsettings.cpp.
|
static |
The path where download are stored of the current user.
- Returns
- the path of the download directory
Definition at line 652 of file kglobalsettings.cpp.
|
static |
Notifies all KDE applications on the current display of a change.
This is typically called by kcontrol modules after changing the corresponding config file. Do not call this from a normal KDE application.
Definition at line 825 of file kglobalsettings.cpp.
|
static |
Returns the default fixed font.
- Returns
- the default fixed font.
Definition at line 450 of file kglobalsettings.cpp.
|
static |
Returns the default general font.
- Returns
- the default general font.
Definition at line 446 of file kglobalsettings.cpp.
|
static |
This function determines the desired level of effects on the GUI.
- Since
- 4.1
Definition at line 782 of file kglobalsettings.cpp.
|
static |
This function determines the default level of effects on the GUI depending on the system capabilities.
- Since
- 4.1
Definition at line 797 of file kglobalsettings.cpp.
|
signal |
Emitted when the global icon settings have been changed.
- Parameters
-
group the new group
|
static |
The default color to use for inactive texts.
- Returns
- the inactive text color
Definition at line 328 of file kglobalsettings.cpp.
|
static |
The default color to use for inactive titles.
- Returns
- the inactive title color
Definition at line 317 of file kglobalsettings.cpp.
|
static |
Returns whether tear-off handles are inserted in KMenus.
- Returns
- whether tear-off handles are inserted in KMenus.
Definition at line 245 of file kglobalsettings.cpp.
|
static |
Returns if the user specified multihead.
In case the display has multiple screens, the return value of this function specifies if the user wants KDE to run on all of them or just on the primary On Windows, settings are retrieved from the system.
- Returns
- true if the user chose multi head
Definition at line 694 of file kglobalsettings.cpp.
|
signal |
Emitted when the application has changed its font in response to a KControl request.
Normally widgets will update their fonts automatically, but you should connect to this to monitor global font changes, especially if you are using explicit fonts.
Note: If you derive from a QWidget-based class, a faster method is to reimplement QWidget::changeEvent() and catch QEvent::FontChange. This is the preferred way to get informed about font updates.
|
signal |
Emitted when the application has changed its palette due to a KControl request.
Normally, widgets will update their palette automatically, but you should connect to this to program special behavior.
Note: If you derive from a QWidget-based class, a faster method is to reimplement QWidget::changeEvent() and catch QEvent::PaletteChange. This is the preferred way to get informed about palette updates.
|
signal |
Emitted when the application has changed its GUI style in response to a KControl request.
Normally, widgets will update their styles automatically (as they would respond to an explicit setGUIStyle() call), but you should connect to this to program special behavior.
Note: If you derive from a QWidget-based class, a faster method is to reimplement QWidget::changeEvent() and catch QEvent::StyleChange. This is the preferred way to get informed about style updates.
Returns a font of approx.
48 pt. capable of showing text
.
- Parameters
-
text the text to test
- Returns
- the font that is capable to show the text with 48 pt
Definition at line 540 of file kglobalsettings.cpp.
|
static |
Returns the default menu font.
- Returns
- the default menu font.
Definition at line 458 of file kglobalsettings.cpp.
|
static |
This returns the current mouse settings.
On Windows, settings are retrieved from the system.
- Returns
- the current mouse settings
Definition at line 613 of file kglobalsettings.cpp.
|
static |
The path where music are stored of the current user.
- Returns
- the path of the music directory
Definition at line 688 of file kglobalsettings.cpp.
|
static |
Returns true, if user visible strings should be sorted in a natural way: image 1.jpg image 2.jpg image 10.jpg image 11.jpg If false is returned, the strings are sorted by their unicode values: image 1.jpg image 10.jpg image 11.jpg image 2.jpg.
- Since
- 4.4
Definition at line 775 of file kglobalsettings.cpp.
|
signal |
Emitted when the natural sorting has been changed.
- Since
- 4.4
|
static |
Whether the user wishes to use opaque resizing.
Primarily intended for QSplitter::setOpaqueResize()
- Returns
- Returns true if user wants to use opaque resizing.
Definition at line 813 of file kglobalsettings.cpp.
|
static |
The path where pictures are stored of the current user.
- Returns
- the path of the pictures directory
Definition at line 682 of file kglobalsettings.cpp.
|
static |
Return the KGlobalSettings singleton.
This is used to connect to its signals, to be notified of changes.
Definition at line 188 of file kglobalsettings.cpp.
|
signal |
Emitted when the global settings have been changed.
KGlobalSettings takes care of calling reparseConfiguration on KGlobal::config() so that applications/classes using this only have to re-read the configuration
- Parameters
-
category the category among the SettingsCategory enum.
|
static |
Returns if the sorted column in a K3ListView shall be drawn with a shaded background color.
- Returns
- true if the sorted column shall be shaded
Definition at line 375 of file kglobalsettings.cpp.
|
static |
Returns the KDE setting for context menus.
- Returns
- whether context menus should be shown on button press or button release (click).
Definition at line 280 of file kglobalsettings.cpp.
This function determines if the user wishes to see previews for the selected url.
- Returns
- Returns true if user wants to show previews.
Definition at line 805 of file kglobalsettings.cpp.
|
static |
This function determines if the user wishes to see icons on the push buttons.
- Returns
- Returns true if user wants to show icons.
Definition at line 768 of file kglobalsettings.cpp.
|
static |
Returns whether KDE runs in single (default) or double click mode.
see http://developer.kde.org/documentation/standards/kde/style/mouse/index.html
- Returns
- true if single click mode, or false if double click mode.
Definition at line 233 of file kglobalsettings.cpp.
|
static |
Returns the smallest readable font.
This can be used in dockers, rulers and other places where space is at a premium.
Definition at line 470 of file kglobalsettings.cpp.
|
static |
Returns if item views should force smooth scrolling.
- Returns
- true if smooth scrolling is enabled for item view, false otherwise.
- Since
- 4.2
Definition at line 239 of file kglobalsettings.cpp.
|
static |
This function returns the desktop geometry for an application's splash screen.
It takes into account the user's display settings (number of screens, Xinerama, etc), and the user's preferences (if KDE should be Xinerama aware).
- Returns
- the geometry to use for the desktop. Note that it might not start at (0,0).
Definition at line 713 of file kglobalsettings.cpp.
|
static |
Returns the default taskbar font.
- Returns
- the default taskbar font.
Definition at line 466 of file kglobalsettings.cpp.
|
signal |
Emitted when the settings for toolbars have been changed.
KToolBar will know what to do.
|
static |
Returns the default toolbar font.
- Returns
- the default toolbar font.
Definition at line 454 of file kglobalsettings.cpp.
|
static |
The path where videos are stored of the current user.
- Returns
- the path of the video directory
Definition at line 676 of file kglobalsettings.cpp.
|
static |
Typically, QScrollView derived classes can be scrolled fast by holding down the Ctrl-button during wheel-scrolling.
But QTextEdit and derived classes perform zooming instead of fast scrolling.
This value determines whether the user wants to zoom or scroll fast with Ctrl-wheelscroll.
- Returns
- true if the user wishes to zoom with the mouse wheel, false for scrolling
Definition at line 707 of file kglobalsettings.cpp.
|
static |
Returns the default window title font.
- Returns
- the default window title font.
Definition at line 462 of file kglobalsettings.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.