KDEUI
#include <kcompletion.h>
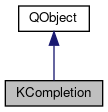
Public Types | |
enum | CompOrder { Sorted, Insertion, Weighted } |
Public Slots | |
void | addItem (const QString &item) |
void | addItem (const QString &item, uint weight) |
virtual void | clear () |
void | insertItems (const QStringList &items) |
void | removeItem (const QString &item) |
virtual void | setItems (const QStringList &list) |
void | slotMakeCompletion (const QString &string) |
void | slotNextMatch () |
void | slotPreviousMatch () |
Signals | |
void | match (const QString &item) |
void | matches (const QStringList &matchlist) |
void | multipleMatches () |
Public Member Functions | |
KCompletion () | |
virtual | ~KCompletion () |
QStringList | allMatches () |
QStringList | allMatches (const QString &string) |
KCompletionMatches | allWeightedMatches () |
KCompletionMatches | allWeightedMatches (const QString &string) |
KGlobalSettings::Completion | completionMode () const |
bool | hasMultipleMatches () const |
bool | ignoreCase () const |
bool | isEmpty () const |
QStringList | items () const |
virtual const QString & | lastMatch () const |
virtual QString | makeCompletion (const QString &string) |
QString | nextMatch () |
CompOrder | order () const |
QString | previousMatch () |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setIgnoreCase (bool ignoreCase) |
virtual void | setOrder (CompOrder order) |
virtual void | setSoundsEnabled (bool enable) |
bool | soundsEnabled () const |
QStringList | substringCompletion (const QString &string) const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual void | postProcessMatch (QString *pMatch) const |
virtual void | postProcessMatches (QStringList *pMatches) const |
virtual void | postProcessMatches (KCompletionMatches *pMatches) const |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Properties | |
bool | ignoreCase |
QStringList | items |
CompOrder | order |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A generic class for completing QStrings.
This class offers easy use of "auto-completion", "manual-completion" or "shell completion" on QString objects. A common use is completing filenames or URLs (see KUrlCompletion()). But it is not limited to URL-completion – everything should be completable! The user should be able to complete email-addresses, telephone-numbers, commands, SQL queries, ... Every time your program knows what the user can type into an edit-field, you should offer completion. With KCompletion, this is very easy, and if you are using a line edit widget ( KLineEdit), it is even more easy. Basically, you tell a KCompletion object what strings should be completable and whenever completion should be invoked, you call makeCompletion(). KLineEdit and (an editable) KComboBox even do this automatically for you.
KCompletion offers the completed string via the signal match() and all matching strings (when the result is ambiguous) via the method allMatches().
Notice: auto-completion, shell completion and manual completion work slightly differently:
- auto-completion always returns a complete item as match. When more than one matching items are available, it will deliver just the first (depending on sorting order) item. Iterating over all matches is possible via nextMatch() and previousMatch().
- popup-completion works in the same way, the only difference being that the completed items are not put into the edit-widget, but into a separate popup-box.
- manual completion works the same way as auto-completion, the subtle difference is, that it isn't invoked automatically while the user is typing, but only when the user presses a special key. The difference of manual and auto-completion is therefore only visible in UI classes, KCompletion needs to know whether to deliver partial matches (shell completion) or whole matches (auto/manual completion), therefore KGlobalSettings::CompletionMan and KGlobalSettings::CompletionAuto have the exact same effect in KCompletion.
- shell completion works like how shells complete filenames: when multiple matches are available, the longest possible string of all matches is returned (i.e. only a partial item). Iterating over all matching items (complete, not partial) is possible via nextMatch() and previousMatch().
You don't have to worry much about that though, KCompletion handles that for you, according to the setting setCompletionMode(). The default setting is globally configured by the user and read from KGlobalSettings::completionMode().
A short example:
In shell-completion-mode, this will be "carp"; in auto-completion- mode it will be "carp\@cs.tu-berlin.de", as that is alphabetically smaller. If setOrder was set to Insertion, "carpdjih\@sp.zrz.tu-berlin.de" would be completed in auto-completion-mode, as that was inserted before "carp\@cs.tu-berlin.de".
You can dynamically update the completable items by removing and adding them whenever you want. For advanced usage, you could even use multiple KCompletion objects. E.g. imagine an editor like kwrite with multiple open files. You could store items of each file in a different KCompletion object, so that you know (and tell the user) where a completion comes from.
Note: KCompletion does not work with strings that contain 0x0 characters (unicode nul), as this is used internally as a delimiter.
You may inherit from KCompletion and override makeCompletion() in special cases (like reading directories/urls and then supplying the contents to KCompletion, as KUrlCompletion does), but generally, this is not necessary.
Definition at line 130 of file kcompletion.h.
Member Enumeration Documentation
Constants that represent the order in which KCompletion performs completion-lookups.
Enumerator | |
---|---|
Sorted |
Use alphabetically sorted order. |
Insertion |
Use order of insertion. |
Weighted |
Use weighted order. |
Definition at line 143 of file kcompletion.h.
Constructor & Destructor Documentation
KCompletion::KCompletion | ( | ) |
Constructor, nothing special here :)
Definition at line 67 of file kcompletion.cpp.
|
virtual |
Destructor, nothing special here, either.
Definition at line 73 of file kcompletion.cpp.
Member Function Documentation
|
slot |
Adds an item to the list of available completions.
Resets the current item-state ( previousMatch() and nextMatch() won't work anymore).
- Parameters
-
item the item to add
Definition at line 146 of file kcompletion.cpp.
|
slot |
Adds an item to the list of available completions.
Resets the current item-state ( previousMatch() and nextMatch() won't work anymore).
Sets the weighting of the item to weight
or adds it to the current weighting if the item is already available. The weight has to be greater than 1 to take effect (default weight is 1).
- Parameters
-
item the item to add weight the weight of the item, default is 1
Definition at line 155 of file kcompletion.cpp.
QStringList KCompletion::allMatches | ( | ) |
Returns a list of all items matching the last completed string.
Might take some time, when you have LOTS of items.
- Returns
- a list of all matches for the last completed string.
- See also
- substringCompletion
Definition at line 339 of file kcompletion.cpp.
QStringList KCompletion::allMatches | ( | const QString & | string | ) |
Returns a list of all items matching string
.
- Parameters
-
string the string to match
- Returns
- the list of all matches
Definition at line 365 of file kcompletion.cpp.
KCompletionMatches KCompletion::allWeightedMatches | ( | ) |
Returns a list of all items matching the last completed string.
Might take some time, when you have LOTS of items. The matches are returned as KCompletionMatches, which also keeps the weight of the matches, allowing you to modify some matches or merge them with matches from another call to allWeightedMatches(), and sort the matches after that in order to have the matches ordered correctly.
- Returns
- a list of all completion matches
- See also
- substringCompletion
Definition at line 352 of file kcompletion.cpp.
KCompletionMatches KCompletion::allWeightedMatches | ( | const QString & | string | ) |
Returns a list of all items matching string
.
- Parameters
-
string the string to match
- Returns
- a list of all matches
Definition at line 375 of file kcompletion.cpp.
|
virtualslot |
Removes all inserted items.
Definition at line 218 of file kcompletion.cpp.
KGlobalSettings::Completion KCompletion::completionMode | ( | ) | const |
Return the current completion mode.
May be different from KGlobalSettings::completionMode(), if you explicitly called setCompletionMode().
- Returns
- the current completion mode
- See also
- setCompletionMode
Definition at line 335 of file kcompletion.cpp.
bool KCompletion::hasMultipleMatches | ( | ) | const |
Returns true when more than one match is found.
- Returns
- true if there are more than one match
- See also
- multipleMatches
Definition at line 395 of file kcompletion.cpp.
bool KCompletion::ignoreCase | ( | ) | const |
Return whether KCompletion acts case insensitively or not.
Default is false (case sensitive).
- Returns
- true if the case will be ignored
- See also
- setIgnoreCase
|
slot |
Inserts items
into the list of possible completions.
Does the same as setItems(), but does not call clear() before.
- Parameters
-
items the items to insert
Definition at line 106 of file kcompletion.cpp.
bool KCompletion::isEmpty | ( | ) | const |
Returns true when the completion object contains no entries.
Definition at line 129 of file kcompletion.cpp.
QStringList KCompletion::items | ( | ) | const |
Returns a list of all items inserted into KCompletion.
This is useful if you need to save the state of a KCompletion object and restore it later.
Important note: when order() == Weighted, then every item in the stringlist has its weight appended, delimited by a colon. E.g. an item "www.kde.org" might look like "www.kde.org:4", where 4 is the weight.
This is necessary so that you can save the items along with its weighting on disk and load them back with setItems(), restoring its weight as well. If you really don't want the appended weightings, call setOrder( KCompletion::Insertion ) before calling items().
- Returns
- a list of all items
- See also
- setItems
|
virtual |
Returns the last match.
Might be useful if you need to check whether a completion is different from the last one.
- Returns
- the last match. QString() is returned when there is no last match.
Definition at line 436 of file kcompletion.cpp.
Attempts to find an item in the list of available completions, that begins with string
.
Will either return the first matching item (if there is more than one match) or QString(), if no match was found.
In the latter case, a sound will be issued, depending on soundsEnabled(). If a match was found, it will also be emitted via the signal match().
If this is called twice or more often with the same string while no items were added or removed in the meantime, all available completions will be emitted via the signal matches(). This happens only in shell-completion-mode.
- Parameters
-
string the string to complete
- Returns
- the matching item, or QString() if there is no matching item.
Definition at line 229 of file kcompletion.cpp.
|
signal |
The matching item.
Will be emitted by makeCompletion(), previousMatch() or nextMatch(). May be QString() if there is no matching item.
- Parameters
-
item the match, or QString() if there is none
|
signal |
All matching items.
Will be emitted by makeCompletion() in shell- completion-mode, when the same string is passed to makeCompletion twice or more often.
- Parameters
-
matchlist the list of matches
|
signal |
This signal is emitted, when calling makeCompletion() and more than one matching item is found.
- See also
- hasMultipleMatches
QString KCompletion::nextMatch | ( | ) |
Returns the next item from the matching-items-list.
When reaching the last item, the list is rotated, so it will return the first match and a sound is issued (depending on soundsEnabled()).
- Returns
- the next item from the matching-items-list. When there is no match, QString() is returned and a sound is issued
- See also
- slotNextMatch
Definition at line 404 of file kcompletion.cpp.
CompOrder KCompletion::order | ( | ) | const |
|
protectedvirtual |
This method is called after a completion is found and before the matching string is emitted.
You can override this method to modify the string that will be emitted. This is necessary e.g. in KUrlCompletion(), where files with spaces in their names are shown escaped ("filename\ with\ spaces"), but stored unescaped inside KCompletion. Never delete that pointer!
Default implementation does nothing.
- Parameters
-
pMatch the match to process
- See also
- postProcessMatches
Definition at line 134 of file kcompletion.cpp.
|
protectedvirtual |
This method is called before a list of all available completions is emitted via matches.
You can override this method to modify the found items before match() or matches are emitted. Never delete that pointer!
Default implementation does nothing.
- Parameters
-
pMatches the matches to process
- See also
- postProcessMatch
Definition at line 138 of file kcompletion.cpp.
|
protectedvirtual |
This method is called before a list of all available completions is emitted via matches.
You can override this method to modify the found items before match() or matches() are emitted. Never delete that pointer!
Default implementation does nothing.
- Parameters
-
pMatches the matches to process
- See also
- postProcessMatch
Definition at line 142 of file kcompletion.cpp.
QString KCompletion::previousMatch | ( | ) |
Returns the next item from the matching-items-list.
When reaching the beginning, the list is rotated so it will return the last match and a sound is issued (depending on soundsEnabled()).
- Returns
- the next item from the matching-items-list. When there is no match, QString() is returned and a sound is be issued.
- See also
- slotPreviousMatch
Definition at line 442 of file kcompletion.cpp.
|
slot |
Removes an item from the list of available completions.
Resets the current item-state ( previousMatch() and nextMatch() won't work anymore).
- Parameters
-
item the item to remove
Definition at line 208 of file kcompletion.cpp.
|
virtual |
Sets the completion mode to Auto/Manual, Shell or None.
If you don't set the mode explicitly, the global default value KGlobalSettings::completionMode() is used. KGlobalSettings::CompletionNone disables completion.
- Parameters
-
mode the completion mode
Definition at line 330 of file kcompletion.cpp.
|
virtual |
Setting this to true makes KCompletion behave case insensitively.
E.g. makeCompletion( "CA" ); might return "carp\@cs.tu-berlin.de". Default is false (case sensitive).
- Parameters
-
ignoreCase true to ignore the case
- See also
- ignoreCase
Definition at line 89 of file kcompletion.cpp.
|
virtualslot |
Sets the list of items available for completion.
Removes all previous items.
Notice: when order() == Weighted, then the weighting is looked up for every item in the stringlist. Every item should have ":number" appended, where number is an unsigned integer, specifying the weighting.
If you don't like this, call setOrder( KCompletion::Insertion ) before calling setItems().
- Parameters
-
list the list of items that are available for completion
- See also
- items
Definition at line 99 of file kcompletion.cpp.
|
virtual |
KCompletion offers three different ways in which it offers its items:
- in the order of insertion
- sorted alphabetically
- weighted
Choosing weighted makes KCompletion perform an implicit weighting based on how often an item is inserted. Imagine a web browser with a location bar, where the user enters URLs. The more often a URL is entered, the higher priority it gets.
Note: Setting the order to sorted only affects new inserted items, already existing items will stay in the current order. So you probably want to call setOrder( Sorted ) before inserting items, when you want everything sorted.
Default is insertion order.
- Parameters
-
order the new order
- See also
- order
Definition at line 78 of file kcompletion.cpp.
|
virtual |
Enables/disables playing a sound when.
- makeCompletion() can't find a match
- there is a partial completion (= multiple matches in Shell-completion mode)
- nextMatch() or previousMatch() hit the last possible match -> rotation
For playing the sounds, KNotifyClient() is used.
- Parameters
-
enable true to enable sounds
- See also
- soundsEnabled
Definition at line 385 of file kcompletion.cpp.
|
inlineslot |
Attempts to complete "string" and emits the completion via match().
Same as makeCompletion() (just as a slot).
- Parameters
-
string the string to complete
- See also
- makeCompletion
Definition at line 386 of file kcompletion.h.
|
inlineslot |
Searches the next matching item and emits it via match().
Same as nextMatch() (just as a slot).
- See also
- nextMatch
Definition at line 404 of file kcompletion.h.
|
inlineslot |
Searches the previous matching item and emits it via match().
Same as previousMatch() (just as a slot).
- See also
- previousMatch
Definition at line 395 of file kcompletion.h.
bool KCompletion::soundsEnabled | ( | ) | const |
Tells you whether KCompletion will play sounds on certain occasions.
Default is enabled.
- Returns
- true if sounds are enabled
- See also
- setSoundsEnabled
Definition at line 390 of file kcompletion.cpp.
QStringList KCompletion::substringCompletion | ( | const QString & | string | ) | const |
Returns a list of all completion items that contain the given string
.
- Parameters
-
string the string to complete
- Returns
- a list of items which all contain
text
as a substring, i.e. not necessarily at the beginning.
- See also
- makeCompletion
Definition at line 292 of file kcompletion.cpp.
Property Documentation
|
readwrite |
Definition at line 134 of file kcompletion.h.
|
readwrite |
Definition at line 135 of file kcompletion.h.
|
readwrite |
Definition at line 133 of file kcompletion.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.