KDEUI
#include <kcombobox.h>
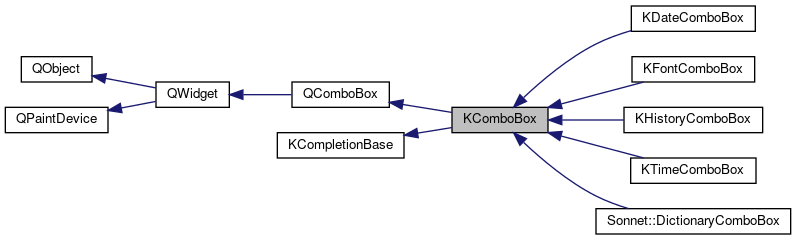
Public Slots | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autosubject=true) |
virtual void | setCompletedText (const QString &) |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
Signals | |
void | aboutToShowContextMenu (QMenu *p) |
void | completion (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed () |
void | returnPressed (const QString &) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Member Functions | |
KComboBox (QWidget *parent=0) | |
KComboBox (bool rw, QWidget *parent=0) | |
virtual | ~KComboBox () |
void | addUrl (const KUrl &url) |
void | addUrl (const QIcon &icon, const KUrl &url) |
bool | autoCompletion () const |
void | changeURL (const KUrl &url, int index) |
void | changeURL (const QPixmap &pixmap, const KUrl &url, int index) |
void | changeUrl (int index, const KUrl &url) |
void | changeUrl (int index, const QIcon &icon, const KUrl &url) |
KCompletionBox * | completionBox (bool create=true) |
bool | contains (const QString &text) const |
int | cursorPosition () const |
virtual bool | eventFilter (QObject *, QEvent *) |
void | insertURL (const KUrl &url, int index=-1) |
void | insertURL (const QPixmap &pixmap, const KUrl &url, int index=-1) |
void | insertUrl (int index, const KUrl &url) |
void | insertUrl (int index, const QIcon &icon, const KUrl &url) |
virtual void | setAutoCompletion (bool autocomplete) |
virtual void | setContextMenuEnabled (bool showMenu) |
void | setEditable (bool editable) |
void | setEditUrl (const KUrl &url) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
void | setUrlDropsEnabled (bool enable) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
![]() | |
QComboBox (QWidget *parent) | |
QComboBox (QWidget *parent, const char *name) | |
QComboBox (bool rw, QWidget *parent, const char *name) | |
~QComboBox () | |
void | activated (const QString &text) |
void | activated (int index) |
void | addItem (const QString &text, const QVariant &userData) |
void | addItem (const QIcon &icon, const QString &text, const QVariant &userData) |
void | addItems (const QStringList &texts) |
bool | autoCompletion () const |
Qt::CaseSensitivity | autoCompletionCaseSensitivity () const |
void | changeItem (const QString &text, int index) |
void | changeItem (const QPixmap &pixmap, int index) |
void | changeItem (const QPixmap &pixmap, const QString &text, int index) |
void | clear () |
void | clearEdit () |
void | clearEditText () |
void | clearValidator () |
QCompleter * | completer () const |
int | count () const |
int | currentIndex () const |
void | currentIndexChanged (const QString &text) |
void | currentIndexChanged (int index) |
int | currentItem () const |
QString | currentText () const |
bool | duplicatesEnabled () const |
bool | editable () const |
void | editTextChanged (const QString &text) |
virtual bool | event (QEvent *event) |
int | findData (const QVariant &data, int role, QFlags< Qt::MatchFlag > flags) const |
int | findText (const QString &text, QFlags< Qt::MatchFlag > flags) const |
bool | hasFrame () const |
virtual void | hidePopup () |
void | highlighted (int index) |
void | highlighted (const QString &text) |
QSize | iconSize () const |
InsertPolicy | insertionPolicy () const |
void | insertItem (const QPixmap &pixmap, const QString &text, int index) |
void | insertItem (const QPixmap &pixmap, int index) |
void | insertItem (const QString &text, int index) |
void | insertItem (int index, const QString &text, const QVariant &userData) |
void | insertItem (int index, const QIcon &icon, const QString &text, const QVariant &userData) |
void | insertItems (int index, const QStringList &list) |
InsertPolicy | insertPolicy () const |
void | insertSeparator (int index) |
void | insertStringList (const QStringList &list, int index) |
bool | isEditable () const |
QVariant | itemData (int index, int role) const |
QAbstractItemDelegate * | itemDelegate () const |
QIcon | itemIcon (int index) const |
QString | itemText (int index) const |
QLineEdit * | lineEdit () const |
int | maxCount () const |
int | maxVisibleItems () const |
int | minimumContentsLength () const |
QAbstractItemModel * | model () const |
int | modelColumn () const |
QPixmap | pixmap (int index) const |
void | popup () |
void | removeItem (int index) |
QModelIndex | rootModelIndex () const |
void | setAutoCompletion (bool enable) |
void | setAutoCompletionCaseSensitivity (Qt::CaseSensitivity sensitivity) |
void | setCompleter (QCompleter *completer) |
void | setCurrentIndex (int index) |
void | setCurrentItem (int index) |
void | setCurrentText (const QString &text) |
void | setDuplicatesEnabled (bool enable) |
void | setEditable (bool editable) |
void | setEditText (const QString &text) |
void | setFrame (bool) |
void | setIconSize (const QSize &size) |
void | setInsertionPolicy (InsertPolicy policy) |
void | setInsertPolicy (InsertPolicy policy) |
void | setItemData (int index, const QVariant &value, int role) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemIcon (int index, const QIcon &icon) |
void | setItemText (int index, const QString &text) |
void | setLineEdit (QLineEdit *edit) |
void | setMaxCount (int max) |
void | setMaxVisibleItems (int maxItems) |
void | setMinimumContentsLength (int characters) |
void | setModel (QAbstractItemModel *model) |
void | setModelColumn (int visibleColumn) |
void | setRootModelIndex (const QModelIndex &index) |
void | setSizeAdjustPolicy (SizeAdjustPolicy policy) |
void | setValidator (const QValidator *validator) |
void | setView (QAbstractItemView *itemView) |
virtual void | showPopup () |
SizeAdjustPolicy | sizeAdjustPolicy () const |
virtual QSize | sizeHint () const |
QString | text (int index) const |
void | textChanged (const QString &text) |
const QValidator * | validator () const |
QAbstractItemView * | view () const |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
![]() | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KGlobalSettings::Completion | completionMode () const |
KCompletion * | completionObject (bool hsig=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
KShortcut | getKeyBinding (KeyBindingType item) const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setCompletionObject (KCompletion *compObj, bool hsig=true) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const KShortcut &key) |
void | useGlobalKeyBindings () |
Protected Slots | |
virtual void | makeCompletion (const QString &) |
Protected Member Functions | |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
virtual QSize | minimumSizeHint () const |
virtual void | setCompletedText (const QString &, bool) |
virtual void | wheelEvent (QWheelEvent *ev) |
![]() | |
virtual void | changeEvent (QEvent *e) |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
virtual void | focusInEvent (QFocusEvent *e) |
virtual void | focusOutEvent (QFocusEvent *e) |
virtual void | hideEvent (QHideEvent *e) |
void | initStyleOption (QStyleOptionComboBox *option) const |
virtual void | inputMethodEvent (QInputMethodEvent *e) |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
virtual void | keyPressEvent (QKeyEvent *e) |
virtual void | keyReleaseEvent (QKeyEvent *e) |
virtual void | mousePressEvent (QMouseEvent *e) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
virtual void | paintEvent (QPaintEvent *e) |
virtual void | resizeEvent (QResizeEvent *e) |
virtual void | showEvent (QShowEvent *e) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
bool | focusPreviousChild () |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType { TextCompletion, PrevCompletionMatch, NextCompletionMatch, SubstringCompletion } |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | Policy |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
An enhanced combo box.
A combined button, line-edit and a popup list widget.
Detail
This widget inherits from QComboBox and implements the following additional functionalities: a completion object that provides both automatic and manual text completion as well as text rotation features, configurable key-bindings to activate these features, and a popup-menu item that can be used to allow the user to change the text completion mode on the fly.
To support these new features KComboBox emits a few additional signals such as completion( const QString& ) and textRotation( KeyBindingType ). The completion signal can be connected to a slot that will assist the user in filling out the remaining text while the rotation signal can be used to traverse through all possible matches whenever text completion results in multiple matches. Additionally, a returnPressed() and a returnPressed( const QString& ) signals are emitted when the user presses the Enter/Return key.
KCombobox by default creates a completion object when you invoke the completionObject( bool ) member function for the first time or explicitly use setCompletionObject( KCompletion*, bool ) to assign your own completion object. Additionally, to make this widget more functional, KComboBox will by default handle text rotation and completion events internally whenever a completion object is created through either one of the methods mentioned above. If you do not need this functionality, simply use KCompletionBase::setHandleSignals(bool) or alternatively set the boolean parameter in the setCompletionObject
call to false.
Beware: The completion object can be deleted on you, especially if a call such as setEditable(false) is made. Store the pointer at your own risk, and consider using QPointer<KCompletion>.
The default key-bindings for completion and rotation is determined from the global settings in KStandardShortcut. These values, however, can be overridden locally by invoking KCompletionBase::setKeyBinding(). The values can easily be reverted back to the default setting, by simply calling useGlobalSettings(). An alternate method would be to default individual key-bindings by usning setKeyBinding() with the default second argument.
A non-editable combobox only has one completion mode, CompletionAuto
. Unlike an editable combobox the CompletionAuto mode, works by matching any typed key with the first letter of entries in the combobox. Please note that if you call setEditable( false ) to change an editable combobox to a non-editable one, the text completion object associated with the combobox will no longer exist unless you created the completion object yourself and assigned it to this widget or you called setAutoDeleteCompletionObject( false ). In other words do not do the following:
A read-only KComboBox will have the same background color as a disabled KComboBox, but its foreground color will be the one used for the read-write mode. This differs from QComboBox's implementation and is done to give visual distinction between the three different modes: disabled, read-only, and read-write.
Usage
To enable the basic completion feature:
To use your own completion object:
Note that you have to either delete the allocated completion object when you don't need it anymore, or call setAutoDeleteCompletionObject( true );
Miscellaneous function calls:
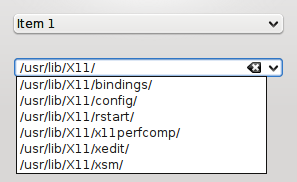
Definition at line 148 of file kcombobox.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a read-only or rather select-only combo box with a parent object and a name.
- Parameters
-
parent The parent object of this widget
Definition at line 57 of file kcombobox.cpp.
Constructs a "read-write" or "read-only" combo box depending on the value of the first argument( rw
) with a parent, a name.
- Parameters
-
rw When true
, widget will be editable.parent The parent object of this widget.
Definition at line 63 of file kcombobox.cpp.
|
virtual |
Destructor.
Definition at line 70 of file kcombobox.cpp.
Member Function Documentation
|
signal |
Emitted before the context menu is displayed.
The signal allows you to add your own entries into the context menu. Note that you MUST NOT store the pointer to the QPopupMenu since it is created and deleted on demand. Otherwise, you can crash your app.
- Parameters
-
p the context menu about to be displayed
void KComboBox::addUrl | ( | const KUrl & | url | ) |
Appends url
to the combobox.
KUrl::prettyUrl() is used so that the url is properly decoded for displaying.
Definition at line 209 of file kcombobox.cpp.
Appends url
with the icon &p icon to the combobox.
KUrl::prettyUrl() is used so that the url is properly decoded for displaying.
Definition at line 214 of file kcombobox.cpp.
bool KComboBox::autoCompletion | ( | ) | const |
Re-implemented from QComboBox.
Returns true
if the current completion mode is set to automatic. See its more comprehensive replacement completionMode().
- Returns
true
when completion mode is automatic.
|
inline |
Definition at line 189 of file kcombobox.h.
Definition at line 191 of file kcombobox.h.
void KComboBox::changeUrl | ( | int | index, |
const KUrl & | url | ||
) |
Replaces the item at position index
with url
.
KUrl::prettyUrl() is used so that the url is properly decoded for displaying.
Definition at line 229 of file kcombobox.cpp.
Replaces the item at position index
with url
and icon icon
.
KUrl::prettyUrl() is used so that the url is properly decoded for displaying.
Definition at line 234 of file kcombobox.cpp.
|
signal |
Emitted when the completion key is pressed.
The argument is the current text being edited.
Note that this signal is not available when the widget is non-editable or the completion mode is set to KGlobalSettings::CompletionNone
.
KCompletionBox * KComboBox::completionBox | ( | bool | create = true | ) |
- Returns
- the completion-box, that is used in completion mode KGlobalSettings::CompletionPopup and KGlobalSettings::CompletionPopupAuto. This method will create a completion-box by calling KLineEdit::completionBox, if none is there, yet.
- Parameters
-
create Set this to false if you don't want the box to be created i.e. to test if it is available.
Definition at line 246 of file kcombobox.cpp.
|
signal |
Emitted whenever the completion mode is changed by the user through the context menu.
Convenience method which iterates over all items and checks if any of them is equal to text
.
If text
is an empty string, false
is returned.
- Returns
true
if an item with the stringtext
is in the combobox.
Definition at line 88 of file kcombobox.cpp.
|
protectedvirtual |
Reimplemented for internal reasons, the API is not affected.
Definition at line 254 of file kcombobox.cpp.
int KComboBox::cursorPosition | ( | ) | const |
Returns the current cursor position.
This method always returns a -1 if the combo-box is not editable (read-write).
- Returns
- Current cursor position.
Definition at line 102 of file kcombobox.cpp.
Re-implemented for internal reasons.
API not affected.
Reimplemented from QObject.
Reimplemented in KDateComboBox, and KTimeComboBox.
Definition at line 183 of file kcombobox.cpp.
|
inline |
Definition at line 187 of file kcombobox.h.
void KComboBox::insertUrl | ( | int | index, |
const KUrl & | url | ||
) |
Inserts url
at position index
into the combobox.
KUrl::prettyUrl() is used so that the url is properly decoded for displaying.
Definition at line 219 of file kcombobox.cpp.
Inserts url
with the pixmap &p pixmap at position index
into the combobox.
KUrl::prettyUrl() is used so that the url is properly decoded for displaying.
Definition at line 224 of file kcombobox.cpp.
|
protectedvirtualslot |
Completes text according to the completion mode.
Note: this method is not
invoked if the completion mode is set to CompletionNone. Also if the mode is set to CompletionShell
and multiple matches are found, this method will complete the text to the first match with a beep to inidicate that there are more matches. Then any successive completion key event iterates through the remaining matches. This way the rotation functionality is left to iterate through the list as usual.
Definition at line 162 of file kcombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 266 of file kcombobox.cpp.
|
signal |
Emitted when the user presses the Enter key.
Note that this signal is only emitted when the widget is editable.
|
signal |
Emitted when the user presses the Enter key.
The argument is the current text being edited. This signal is just like returnPressed() except it contains the current text as its argument.
Note that this signal is only emitted when the widget is editable.
|
slot |
Iterates through all possible matches of the completed text or the history list.
Depending on the value of the argument, this function either iterates through the history list of this widget or the all possible matches in whenever multiple matches result from a text completion request. Note that the all-possible-match iteration will not work if there are no previous matches, i.e. no text has been completed and the *nix shell history list rotation is only available if the insertion policy for this widget is set either QComobBox::AtTop
or QComboBox::AtBottom
. For other insertion modes whatever has been typed by the user when the rotation event was initiated will be lost.
- Parameters
-
type The key-binding invoked.
Definition at line 176 of file kcombobox.cpp.
|
virtual |
Re-implemented from QComboBox.
If true
, the completion mode will be set to automatic. Otherwise, it is defaulted to the global setting. This method has been replaced by the more comprehensive setCompletionMode().
- Parameters
-
autocomplete Flag to enable/disable automatic completion mode.
Definition at line 107 of file kcombobox.cpp.
|
slot |
Sets items
into the completion-box if completionMode() is CompletionPopup.
The popup will be shown immediately.
Definition at line 240 of file kcombobox.cpp.
|
virtualslot |
Sets the completed text in the line-edit appropriately.
This function is an implementation for KCompletionBase::setCompletedText.
Definition at line 156 of file kcombobox.cpp.
Definition at line 150 of file kcombobox.cpp.
|
virtual |
Enables or disable the popup (context) menu.
This method only works if this widget is editable, i.e. read-write and allows you to enable/disable the context menu. It does nothing if invoked for a none-editable combo-box.
By default, the context menu is created if this widget is editable. Call this function with the argument set to false to disable the popup menu.
- Parameters
-
showMenu If true
, show the context menu.
- Deprecated:
- use setContextMenuPolicy
Definition at line 130 of file kcombobox.cpp.
Selects the first item that matches item
.
If there is no such item, it is inserted at position index
if insert
is true. Otherwise, no item is selected.
Definition at line 347 of file kcombobox.cpp.
void KComboBox::setEditable | ( | bool | editable | ) |
"Re-implemented" so that setEditable(true) creates a KLineEdit instead of QLineEdit.
Note that QComboBox::setEditable is not virtual, so do not use a KComboBox in a QComboBox pointer.
Definition at line 386 of file kcombobox.cpp.
void KComboBox::setEditUrl | ( | const KUrl & | url | ) |
Sets url
into the edit field of the combobox.
It uses KUrl::prettyUrl() so that the url is properly decoded for displaying.
Definition at line 204 of file kcombobox.cpp.
|
virtual |
Re-implemented for internal reasons.
API remains unaffected. Note that QComboBox::setLineEdit is not virtual in Qt4, do not use a KComboBox in a QComboBox pointer.
NOTE: Only editable comboboxes can have a line editor. As such any attempt to assign a line-edit to a non-editable combobox will simply be ignored.
Definition at line 282 of file kcombobox.cpp.
void KComboBox::setTrapReturnKey | ( | bool | trap | ) |
By default, KComboBox recognizes Key_Return and Key_Enter and emits the returnPressed() signals, but it also lets the event pass, for example causing a dialog's default-button to be called.
Call this method with trap
equal to true to make KComboBox stop these events. The signals will still be emitted of course.
Only affects read-writable comboboxes.
- See also
- setTrapReturnKey()
Definition at line 188 of file kcombobox.cpp.
void KComboBox::setUrlDropsEnabled | ( | bool | enable | ) |
Enables/Disables handling of URL drops.
If enabled and the user drops an URL, the decoded URL will be inserted. Otherwise the default behavior of QComboBox is used, which inserts the encoded URL.
- Parameters
-
enable If true
, insert decoded URLs
Definition at line 138 of file kcombobox.cpp.
|
signal |
Emitted when the shortcut for substring completion is pressed.
|
signal |
Emitted when the text rotation key-bindings are pressed.
The argument indicates which key-binding was pressed. In this case this can be either one of four values: PrevCompletionMatch
, NextCompletionMatch
, RotateUp
or RotateDown
. See KCompletionBase::setKeyBinding() for details.
Note that this signal is NOT emitted if the completion mode is set to CompletionNone.
bool KComboBox::trapReturnKey | ( | ) | const |
- Returns
true
if keyevents of Key_Return or Key_Enter will be stopped or if they will be propagated.
- See also
- setTrapReturnKey ()
bool KComboBox::urlDropsEnabled | ( | ) | const |
Returns true
when decoded URL drops are enabled.
|
protectedvirtual |
Reimplemented from QComboBox.
Reimplemented in KDateComboBox, KTimeComboBox, and KHistoryComboBox.
Definition at line 260 of file kcombobox.cpp.
Property Documentation
|
readwrite |
Definition at line 151 of file kcombobox.h.
|
readwrite |
Definition at line 153 of file kcombobox.h.
|
readwrite |
Definition at line 152 of file kcombobox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.