KDEUI
#include <kdatecombobox.h>
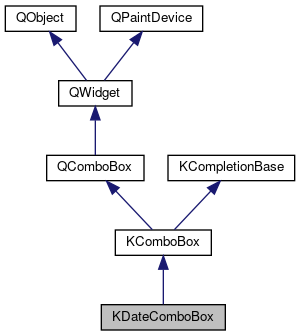
Public Types | |
enum | Option { EditDate = 0x0001, SelectDate = 0x0002, DatePicker = 0x0004, DateKeywords = 0x0008, WarnOnInvalid = 0x0010 } |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType { TextCompletion, PrevCompletionMatch, NextCompletionMatch, SubstringCompletion } |
Public Slots | |
void | resetDateRange () |
void | resetMaximumDate () |
void | resetMinimumDate () |
void | setCalendar (KCalendarSystem *calendar=0) |
void | setCalendarSystem (KLocale::CalendarSystem calendarSystem) |
void | setDate (const QDate &date) |
void | setDateMap (QMap< QDate, QString > dateMap) |
void | setDateRange (const QDate &minDate, const QDate &maxDate, const QString &minWarnMsg=QString(), const QString &maxWarnMsg=QString()) |
void | setDisplayFormat (KLocale::DateFormat format) |
void | setMaximumDate (const QDate &maxDate, const QString &maxWarnMsg=QString()) |
void | setMinimumDate (const QDate &minTime, const QString &minWarnMsg=QString()) |
void | setOptions (Options options) |
![]() | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autosubject=true) |
virtual void | setCompletedText (const QString &) |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
Signals | |
void | dateChanged (const QDate &date) |
void | dateEdited (const QDate &date) |
void | dateEntered (const QDate &date) |
![]() | |
void | aboutToShowContextMenu (QMenu *p) |
void | completion (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed () |
void | returnPressed (const QString &) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Member Functions | |
KDateComboBox (QWidget *parent=0) | |
virtual | ~KDateComboBox () |
const KCalendarSystem * | calendar () const |
KLocale::CalendarSystem | calendarSystem () const |
QDate | date () const |
QMap< QDate, QString > | dateMap () const |
KLocale::DateFormat | displayFormat () const |
bool | isNull () const |
bool | isValid () const |
QDate | maximumDate () const |
QDate | minimumDate () const |
Options | options () const |
![]() | |
KComboBox (QWidget *parent=0) | |
KComboBox (bool rw, QWidget *parent=0) | |
virtual | ~KComboBox () |
void | addUrl (const KUrl &url) |
void | addUrl (const QIcon &icon, const KUrl &url) |
bool | autoCompletion () const |
void | changeURL (const KUrl &url, int index) |
void | changeURL (const QPixmap &pixmap, const KUrl &url, int index) |
void | changeUrl (int index, const KUrl &url) |
void | changeUrl (int index, const QIcon &icon, const KUrl &url) |
KCompletionBox * | completionBox (bool create=true) |
bool | contains (const QString &text) const |
int | cursorPosition () const |
void | insertURL (const KUrl &url, int index=-1) |
void | insertURL (const QPixmap &pixmap, const KUrl &url, int index=-1) |
void | insertUrl (int index, const KUrl &url) |
void | insertUrl (int index, const QIcon &icon, const KUrl &url) |
virtual void | setAutoCompletion (bool autocomplete) |
virtual void | setContextMenuEnabled (bool showMenu) |
void | setEditable (bool editable) |
void | setEditUrl (const KUrl &url) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
void | setUrlDropsEnabled (bool enable) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
![]() | |
QComboBox (QWidget *parent) | |
QComboBox (QWidget *parent, const char *name) | |
QComboBox (bool rw, QWidget *parent, const char *name) | |
~QComboBox () | |
void | activated (const QString &text) |
void | activated (int index) |
void | addItem (const QString &text, const QVariant &userData) |
void | addItem (const QIcon &icon, const QString &text, const QVariant &userData) |
void | addItems (const QStringList &texts) |
bool | autoCompletion () const |
Qt::CaseSensitivity | autoCompletionCaseSensitivity () const |
void | changeItem (const QString &text, int index) |
void | changeItem (const QPixmap &pixmap, int index) |
void | changeItem (const QPixmap &pixmap, const QString &text, int index) |
void | clear () |
void | clearEdit () |
void | clearEditText () |
void | clearValidator () |
QCompleter * | completer () const |
int | count () const |
int | currentIndex () const |
void | currentIndexChanged (const QString &text) |
void | currentIndexChanged (int index) |
int | currentItem () const |
QString | currentText () const |
bool | duplicatesEnabled () const |
bool | editable () const |
void | editTextChanged (const QString &text) |
virtual bool | event (QEvent *event) |
int | findData (const QVariant &data, int role, QFlags< Qt::MatchFlag > flags) const |
int | findText (const QString &text, QFlags< Qt::MatchFlag > flags) const |
bool | hasFrame () const |
void | highlighted (int index) |
void | highlighted (const QString &text) |
QSize | iconSize () const |
InsertPolicy | insertionPolicy () const |
void | insertItem (const QPixmap &pixmap, const QString &text, int index) |
void | insertItem (const QPixmap &pixmap, int index) |
void | insertItem (const QString &text, int index) |
void | insertItem (int index, const QString &text, const QVariant &userData) |
void | insertItem (int index, const QIcon &icon, const QString &text, const QVariant &userData) |
void | insertItems (int index, const QStringList &list) |
InsertPolicy | insertPolicy () const |
void | insertSeparator (int index) |
void | insertStringList (const QStringList &list, int index) |
bool | isEditable () const |
QVariant | itemData (int index, int role) const |
QAbstractItemDelegate * | itemDelegate () const |
QIcon | itemIcon (int index) const |
QString | itemText (int index) const |
QLineEdit * | lineEdit () const |
int | maxCount () const |
int | maxVisibleItems () const |
int | minimumContentsLength () const |
QAbstractItemModel * | model () const |
int | modelColumn () const |
QPixmap | pixmap (int index) const |
void | popup () |
void | removeItem (int index) |
QModelIndex | rootModelIndex () const |
void | setAutoCompletion (bool enable) |
void | setAutoCompletionCaseSensitivity (Qt::CaseSensitivity sensitivity) |
void | setCompleter (QCompleter *completer) |
void | setCurrentIndex (int index) |
void | setCurrentItem (int index) |
void | setCurrentText (const QString &text) |
void | setDuplicatesEnabled (bool enable) |
void | setEditable (bool editable) |
void | setEditText (const QString &text) |
void | setFrame (bool) |
void | setIconSize (const QSize &size) |
void | setInsertionPolicy (InsertPolicy policy) |
void | setInsertPolicy (InsertPolicy policy) |
void | setItemData (int index, const QVariant &value, int role) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemIcon (int index, const QIcon &icon) |
void | setItemText (int index, const QString &text) |
void | setLineEdit (QLineEdit *edit) |
void | setMaxCount (int max) |
void | setMaxVisibleItems (int maxItems) |
void | setMinimumContentsLength (int characters) |
void | setModel (QAbstractItemModel *model) |
void | setModelColumn (int visibleColumn) |
void | setRootModelIndex (const QModelIndex &index) |
void | setSizeAdjustPolicy (SizeAdjustPolicy policy) |
void | setValidator (const QValidator *validator) |
void | setView (QAbstractItemView *itemView) |
SizeAdjustPolicy | sizeAdjustPolicy () const |
virtual QSize | sizeHint () const |
QString | text (int index) const |
void | textChanged (const QString &text) |
const QValidator * | validator () const |
QAbstractItemView * | view () const |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
![]() | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KGlobalSettings::Completion | completionMode () const |
KCompletion * | completionObject (bool hsig=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
KShortcut | getKeyBinding (KeyBindingType item) const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setCompletionObject (KCompletion *compObj, bool hsig=true) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const KShortcut &key) |
void | useGlobalKeyBindings () |
Protected Member Functions | |
virtual void | assignCalendarSystem (KLocale::CalendarSystem calendarSystem) |
virtual void | assignDate (const QDate &date) |
virtual bool | eventFilter (QObject *object, QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
virtual void | focusOutEvent (QFocusEvent *event) |
virtual void | hidePopup () |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showPopup () |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
virtual QSize | minimumSizeHint () const |
virtual void | setCompletedText (const QString &, bool) |
![]() | |
virtual void | changeEvent (QEvent *e) |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
virtual void | hideEvent (QHideEvent *e) |
void | initStyleOption (QStyleOptionComboBox *option) const |
virtual void | inputMethodEvent (QInputMethodEvent *e) |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
virtual void | keyReleaseEvent (QKeyEvent *e) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
virtual void | paintEvent (QPaintEvent *e) |
virtual void | showEvent (QShowEvent *e) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
bool | focusPreviousChild () |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | Policy |
![]() | |
typedef | RenderFlags |
![]() | |
virtual void | makeCompletion (const QString &) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
Definition at line 34 of file kdatecombobox.h.
Member Enumeration Documentation
Options provided by the widget.
- See also
- options()
- setOptions()
Definition at line 51 of file kdatecombobox.h.
Constructor & Destructor Documentation
|
explicit |
Create a new KDateComboBox widget.
By default the EditDate, SelectDate, DatePicker and DateKeywords options are enabled, the ShortDate format is used and the date is set to the current date.
Definition at line 278 of file kdatecombobox.cpp.
|
virtual |
Destroy the widget.
Definition at line 299 of file kdatecombobox.cpp.
Member Function Documentation
|
protectedvirtual |
Assign the calendar system for the widget.
Virtual to allow sub-classes to apply extra validation rules.
- Parameters
-
time the new time
Definition at line 338 of file kdatecombobox.cpp.
|
protectedvirtual |
Assign the date for the widget.
Virtual to allow sub-classes to apply extra validation rules.
- Parameters
-
date the new date
Definition at line 321 of file kdatecombobox.cpp.
const KCalendarSystem * KDateComboBox::calendar | ( | ) | const |
Returns a pointer to the Calendar System object used by this widget.
Usually this will be the Global Calendar System using the Global Locale, but this may have been changed to a custom Calendar System possibly using a custom Locale.
Normally you will not need to access this object.
- See also
- KCalendarSystem
- setCalendar
- Returns
- the current calendar system instance
Definition at line 343 of file kdatecombobox.cpp.
KLocale::CalendarSystem KDateComboBox::calendarSystem | ( | ) | const |
Returns the Calendar System type used by the widget.
- Returns
- the Calendar System currently used
Definition at line 326 of file kdatecombobox.cpp.
QDate KDateComboBox::date | ( | ) | const |
Return the currently selected date.
- Returns
- the currently selected date
|
signal |
Signal if the date has been changed either manually by the user or programatically.
The returned date may be invalid.
- Parameters
-
date the new date
|
signal |
Signal if the date is being manually edited by the user.
The returned date may be invalid.
- Parameters
-
date the new date
|
signal |
Signal if the date has been manually entered or selected by the user.
The returned date may be invalid.
- Parameters
-
date the new date
Return the map of dates listed in the drop-down and their displayed string forms.
- See also
- setDateMap()
- Returns
- the select date map
Definition at line 448 of file kdatecombobox.cpp.
KLocale::DateFormat KDateComboBox::displayFormat | ( | ) | const |
Return the currently set date display format.
By default this is the Short Date
- Returns
- the currently set date format
Definition at line 434 of file kdatecombobox.cpp.
Re-implemented for internal reasons.
API not affected.
Reimplemented from KComboBox.
Definition at line 462 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 553 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 492 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 538 of file kdatecombobox.cpp.
bool KDateComboBox::isNull | ( | ) | const |
Return if the current user input is null.
- See also
- isValid()
- Returns
- if the current user input is null
Definition at line 361 of file kdatecombobox.cpp.
bool KDateComboBox::isValid | ( | ) | const |
Return if the current user input is valid.
If the user input is null then it is not valid
- See also
- isNull()
- Returns
- if the current user input is valid
Definition at line 353 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 467 of file kdatecombobox.cpp.
QDate KDateComboBox::maximumDate | ( | ) | const |
Return the current maximum date.
- Returns
- the current maximum date
QDate KDateComboBox::minimumDate | ( | ) | const |
Return the current minimum date.
- Returns
- the current minimum date
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 543 of file kdatecombobox.cpp.
Options KDateComboBox::options | ( | ) | const |
Return the currently set widget options.
- Returns
- the currently set widget options
|
slot |
Reset the minimum and maximum date to the default values.
- See also
- setDateRange()
Definition at line 429 of file kdatecombobox.cpp.
|
slot |
Reset the maximum date to the default.
Definition at line 405 of file kdatecombobox.cpp.
|
slot |
Reset the minimum date to the default.
Definition at line 390 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 558 of file kdatecombobox.cpp.
|
slot |
Changes the calendar system to use.
Can use its own local locale if set.
You retain ownership of the calendar object, it will not be destroyed with the widget.
- Parameters
-
calendar the calendar system object to use, defaults to global
Definition at line 348 of file kdatecombobox.cpp.
|
slot |
Set the Calendar System used for this widget.
Uses the global locale.
- Parameters
-
calendarSystem the Calendar System to use
Definition at line 331 of file kdatecombobox.cpp.
|
slot |
Set the currently selected date.
You can set an invalid date or a date outside the valid range, validity checking is only done via isValid().
- Parameters
-
date the new date
Definition at line 310 of file kdatecombobox.cpp.
Set the list of dates able to be selected from the drop-down and the string form to display for those dates, e.g.
"2010-01-01" and "Yesterday".
Any invalid or duplicate dates will be used, the list will NOT be sorted, and the minimum and maximum date will not be affected.
The dateMap
is keyed by the date to be listed and the value is the string to be displayed. If you want the date to be displayed in the default date format then the string should be null. If you want a separator to be displayed then set the string to "seperator".
- See also
- dateMap()
- Parameters
-
dateMap the map of dates able to be selected
Definition at line 453 of file kdatecombobox.cpp.
|
slot |
Set the valid date range to be applied by isValid().
Both dates must be valid and the minimum date must be less than or equal to the maximum date, otherwise the date range will not be set.
- Parameters
-
minDate the minimum date maxDate the maximum date minWarnMsg the minimum warning message maxWarnMsg the maximum warning message
Definition at line 410 of file kdatecombobox.cpp.
|
slot |
Sets the date format to display.
By default is the Short Date format.
- Parameters
-
format the date format to use
Definition at line 439 of file kdatecombobox.cpp.
|
slot |
Set the maximum allowed date.
If the date is invalid, or less than current minimum, then the maximum will not be set.
- Parameters
-
maxDate the maximum date maxWarnMsg the maximum warning message
Definition at line 400 of file kdatecombobox.cpp.
|
slot |
Set the minimum allowed date.
If the date is invalid, or greater than current maximum, then the minimum will not be set.
- Parameters
-
minDate the minimum date minWarnMsg the minimum warning message
Definition at line 385 of file kdatecombobox.cpp.
|
slot |
Set the new widget options.
- Parameters
-
options the new widget options
Definition at line 371 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from QComboBox.
Definition at line 499 of file kdatecombobox.cpp.
|
protectedvirtual |
Reimplemented from KComboBox.
Definition at line 548 of file kdatecombobox.cpp.
Property Documentation
|
readwrite |
Definition at line 38 of file kdatecombobox.h.
|
readwrite |
Definition at line 40 of file kdatecombobox.h.
|
readwrite |
Definition at line 39 of file kdatecombobox.h.
|
readwrite |
Definition at line 41 of file kdatecombobox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.