KDEUI
#include <kcompletion.h>
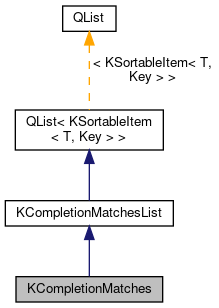
Public Member Functions | |
KCompletionMatches (bool sort) | |
KCompletionMatches (const KCompletionMatches &) | |
KCompletionMatches (const KCompletionMatchesWrapper &matches) | |
~KCompletionMatches () | |
QStringList | list (bool sort=true) const |
KCompletionMatches & | operator= (const KCompletionMatches &) |
void | removeDuplicates () |
bool | sorting () const |
![]() | |
void | insert (Key i, const T &t) |
T & | operator[] (Key i) |
const T & | operator[] (Key i) const |
void | sort () |
![]() | |
QList () | |
QList (const QList< T > &other) | |
QList (std::initializer_list< T > args) | |
~QList () | |
void | append (const T &value) |
void | append (const QList< T > &value) |
const T & | at (int i) const |
T & | back () |
const T & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const T &value) const |
int | count (const T &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const T &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const T &t) |
iterator | find (const T &t) |
const_iterator | find (const T &t) const |
const_iterator | find (const_iterator from, const T &t) const |
int | findIndex (const T &t) const |
T & | first () |
const T & | first () const |
T & | front () |
const T & | front () const |
int | indexOf (const T &value, int from) const |
void | insert (int i, const T &value) |
iterator | insert (iterator before, const T &value) |
bool | isEmpty () const |
T & | last () |
const T & | last () const |
int | lastIndexOf (const T &value, int from) const |
int | length () const |
QList< T > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< T > &other) const |
QList< T > | operator+ (const QList< T > &other) const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (const T &value) |
QList< T > & | operator<< (const T &value) |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator= (const QList< T > &other) |
bool | operator== (const QList< T > &other) const |
T & | operator[] (int i) |
const T & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const T &value) |
void | push_back (const T &value) |
void | push_front (const T &value) |
iterator | remove (iterator pos) |
int | remove (const T &t) |
int | removeAll (const T &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const T &value) |
void | replace (int i, const T &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const T &value) const |
void | swap (int i, int j) |
void | swap (QList< T > &other) |
T | takeAt (int i) |
T | takeFirst () |
T | takeLast () |
QSet< T > | toSet () const |
std::list< T > | toStdList () const |
QVector< T > | toVector () const |
T | value (int i, const T &defaultValue) const |
T | value (int i) const |
Additional Inherited Members | |
![]() | |
QList< T > | fromSet (const QSet< T > &set) |
QList< T > | fromStdList (const std::list< T > &list) |
QList< T > | fromVector (const QVector< T > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
This structure is returned by KCompletion::allWeightedMatches .
It also keeps the weight of the matches, allowing you to modify some matches or merge them with matches from another call to allWeightedMatches(), and sort the matches after that in order to have the matches ordered correctly
Example (a simplified example of what Konqueror's completion does):
List for keeping matches returned from KCompletion
Definition at line 579 of file kcompletion.h.
Constructor & Destructor Documentation
KCompletionMatches::KCompletionMatches | ( | bool | sort | ) |
Default constructor.
- Parameters
-
sort if false, the matches won't be sorted before the conversion, use only if you're sure the sorting is not needed
Definition at line 862 of file kcompletion.cpp.
KCompletionMatches::KCompletionMatches | ( | const KCompletionMatches & | o | ) |
copy constructor.
Definition at line 845 of file kcompletion.cpp.
KCompletionMatches::KCompletionMatches | ( | const KCompletionMatchesWrapper & | matches | ) |
Definition at line 867 of file kcompletion.cpp.
KCompletionMatches::~KCompletionMatches | ( | ) |
default destructor.
Definition at line 881 of file kcompletion.cpp.
Member Function Documentation
QStringList KCompletionMatches::list | ( | bool | sort = true | ) | const |
Returns the matches as a QStringList.
- Parameters
-
sort if false, the matches won't be sorted before the conversion, use only if you're sure the sorting is not needed
- Returns
- the list of matches
Definition at line 886 of file kcompletion.cpp.
KCompletionMatches & KCompletionMatches::operator= | ( | const KCompletionMatches & | o | ) |
assignment operator.
Definition at line 852 of file kcompletion.cpp.
void KCompletionMatches::removeDuplicates | ( | ) |
Removes duplicate matches.
Needed only when you merged several matches results and there's a possibility of duplicates.
Definition at line 902 of file kcompletion.cpp.
bool KCompletionMatches::sorting | ( | ) | const |
If sorting() returns false, the matches aren't sorted by their weight, even if true is passed to list().
- Returns
- true if the matches won't be sorted
Definition at line 897 of file kcompletion.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.