KDEUI
#include <krichtextedit.h>
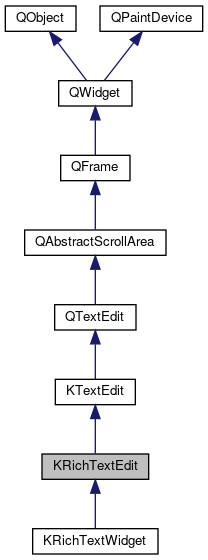
Public Types | |
enum | Mode { Plain, Rich } |
Signals | |
void | selectionFinished () |
void | textModeChanged (KRichTextEdit::Mode mode) |
![]() | |
void | aboutToShowContextMenu (QMenu *menu) |
void | checkSpellingChanged (bool) |
void | languageChanged (const QString &language) |
void | spellCheckerAutoCorrect (const QString ¤tWord, const QString &autoCorrectWord) |
void | spellCheckingCanceled () |
void | spellCheckingFinished () |
void | spellCheckStatus (const QString &) |
Public Member Functions | |
KRichTextEdit (const QString &text, QWidget *parent=0) | |
KRichTextEdit (QWidget *parent=0) | |
virtual | ~KRichTextEdit () |
bool | canDedentList () const |
bool | canIndentList () const |
QString | currentLinkText () const |
QString | currentLinkUrl () const |
void | enableRichTextMode () |
void | selectLinkText (QTextCursor *cursor) const |
void | selectLinkText () const |
void | setTextOrHtml (const QString &text) |
Mode | textMode () const |
QString | textOrHtml () const |
void | updateLink (const QString &linkUrl, const QString &linkText) |
![]() | |
KTextEdit (const QString &text, QWidget *parent=0) | |
KTextEdit (QWidget *parent=0) | |
~KTextEdit () | |
bool | checkSpellingEnabled () const |
QString | clickMessage () const |
virtual void | createHighlighter () |
void | enableFindReplace (bool enabled) |
void | forceSpellChecking () |
Sonnet::Highlighter * | highlighter () const |
void | highlightWord (int length, int pos) |
QMenu * | mousePopupMenu () |
void | setCheckSpellingEnabled (bool check) |
void | setClickMessage (const QString &msg) |
void | setHighlighter (Sonnet::Highlighter *_highLighter) |
virtual void | setReadOnly (bool readOnly) |
void | setSpellCheckingConfigFileName (const QString &fileName) |
void | setSpellInterface (KTextEditSpellInterface *spellInterface) |
void | showAutoCorrectButton (bool show) |
void | showTabAction (bool show) |
const QString & | spellCheckingLanguage () const |
![]() | |
QTextEdit (QWidget *parent) | |
QTextEdit (const QString &text, QWidget *parent) | |
QTextEdit (QWidget *parent, const char *name) | |
virtual | ~QTextEdit () |
bool | acceptRichText () const |
Qt::Alignment | alignment () const |
QString | anchorAt (const QPoint &pos) const |
void | append (const QString &text) |
AutoFormatting | autoFormatting () const |
bool | bold () const |
bool | canPaste () const |
void | clear () |
QColor | color () const |
void | copy () |
void | copyAvailable (bool yes) |
QMenu * | createStandardContextMenu () |
QMenu * | createStandardContextMenu (const QPoint &position) |
QTextCharFormat | currentCharFormat () const |
void | currentCharFormatChanged (const QTextCharFormat &f) |
void | currentColorChanged (const QColor &color) |
QFont | currentFont () const |
void | currentFontChanged (const QFont &font) |
QTextCursor | cursorForPosition (const QPoint &pos) const |
void | cursorPositionChanged () |
QRect | cursorRect (const QTextCursor &cursor) const |
QRect | cursorRect () const |
int | cursorWidth () const |
void | cut () |
QTextDocument * | document () const |
QString | documentTitle () const |
void | doKeyboardAction (KeyboardAction action) |
void | ensureCursorVisible () |
QList< ExtraSelection > | extraSelections () const |
QString | family () const |
bool | find (const QString &exp, QFlags< QTextDocument::FindFlag > options) |
bool | find (const QString &exp, bool cs, bool wo) |
QString | fontFamily () const |
bool | fontItalic () const |
qreal | fontPointSize () const |
bool | fontUnderline () const |
int | fontWeight () const |
bool | hasSelectedText () const |
void | insert (const QString &text) |
void | insertHtml (const QString &text) |
void | insertPlainText (const QString &text) |
bool | isModified () const |
bool | isReadOnly () const |
bool | isRedoAvailable () const |
bool | isUndoAvailable () const |
bool | isUndoRedoEnabled () const |
bool | italic () const |
int | lineWrapColumnOrWidth () const |
LineWrapMode | lineWrapMode () const |
virtual QVariant | loadResource (int type, const QUrl &name) |
void | mergeCurrentCharFormat (const QTextCharFormat &modifier) |
void | moveCursor (CursorAction action, QTextCursor::MoveMode mode) |
void | moveCursor (CursorAction action, bool select) |
void | moveCursor (QTextCursor::MoveOperation operation, QTextCursor::MoveMode mode) |
bool | overwriteMode () const |
void | paste () |
int | pointSize () const |
void | print (QPrinter *printer) const |
void | redo () |
void | redo () const |
void | redoAvailable (bool available) |
void | scrollToAnchor (const QString &name) |
void | selectAll () |
QString | selectedText () const |
void | selectionChanged () |
void | setAcceptRichText (bool accept) |
void | setAlignment (QFlags< Qt::AlignmentFlag > a) |
void | setAutoFormatting (QFlags< QTextEdit::AutoFormattingFlag > features) |
void | setBold (bool b) |
void | setColor (const QColor &color) |
void | setCurrentCharFormat (const QTextCharFormat &format) |
void | setCurrentFont (const QFont &f) |
void | setCursorWidth (int width) |
void | setDocument (QTextDocument *document) |
void | setDocumentTitle (const QString &title) |
void | setExtraSelections (const QList< ExtraSelection > &selections) |
void | setFamily (const QString &family) |
void | setFontFamily (const QString &fontFamily) |
void | setFontItalic (bool italic) |
void | setFontPointSize (qreal s) |
void | setFontUnderline (bool underline) |
void | setFontWeight (int weight) |
void | setHtml (const QString &text) |
void | setItalic (bool i) |
void | setLineWrapColumnOrWidth (int w) |
void | setLineWrapMode (LineWrapMode mode) |
void | setModified (bool m) |
void | setOverwriteMode (bool overwrite) |
void | setPlainText (const QString &text) |
void | setPointSize (int size) |
void | setReadOnly (bool ro) |
void | setTabChangesFocus (bool b) |
void | setTabStopWidth (int width) |
void | setText (const QString &text) |
void | setTextBackgroundColor (const QColor &c) |
void | setTextColor (const QColor &c) |
void | setTextCursor (const QTextCursor &cursor) |
void | setTextFormat (Qt::TextFormat f) |
void | setTextInteractionFlags (QFlags< Qt::TextInteractionFlag > flags) |
void | setUnderline (bool b) |
void | setUndoRedoEnabled (bool enable) |
void | setWordWrapMode (QTextOption::WrapMode policy) |
void | sync () |
bool | tabChangesFocus () const |
int | tabStopWidth () const |
QString | text () const |
QColor | textBackgroundColor () const |
void | textChanged () |
QColor | textColor () const |
QTextCursor | textCursor () const |
Qt::TextFormat | textFormat () const |
Qt::TextInteractionFlags | textInteractionFlags () const |
QString | toHtml () const |
QString | toPlainText () const |
bool | underline () const |
void | undo () const |
void | undo () |
void | undoAvailable (bool available) |
QTextOption::WrapMode | wordWrapMode () const |
void | zoomIn (int range) |
void | zoomOut (int range) |
![]() | |
QAbstractScrollArea (QWidget *parent) | |
~QAbstractScrollArea () | |
void | addScrollBarWidget (QWidget *widget, QFlags< Qt::AlignmentFlag > alignment) |
QWidget * | cornerWidget () const |
QScrollBar * | horizontalScrollBar () const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const |
QSize | maximumViewportSize () const |
virtual QSize | minimumSizeHint () const |
QWidgetList | scrollBarWidgets (QFlags< Qt::AlignmentFlag > alignment) |
void | setCornerWidget (QWidget *widget) |
void | setHorizontalScrollBar (QScrollBar *scrollBar) |
void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setVerticalScrollBar (QScrollBar *scrollBar) |
void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setViewport (QWidget *widget) |
virtual QSize | sizeHint () const |
QScrollBar * | verticalScrollBar () const |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const |
QWidget * | viewport () const |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual void | keyPressEvent (QKeyEvent *event) |
![]() | |
bool | checkSpellingEnabledInternal () const |
virtual void | contextMenuEvent (QContextMenuEvent *) |
virtual void | deleteWordBack () |
virtual void | deleteWordForward () |
virtual bool | event (QEvent *) |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | paintEvent (QPaintEvent *) |
void | setCheckSpellingEnabledInternal (bool check) |
virtual void | wheelEvent (QWheelEvent *) |
![]() | |
virtual bool | canInsertFromMimeData (const QMimeData *source) const |
virtual void | changeEvent (QEvent *e) |
virtual QMimeData * | createMimeDataFromSelection () const |
virtual void | dragEnterEvent (QDragEnterEvent *e) |
virtual void | dragLeaveEvent (QDragLeaveEvent *e) |
virtual void | dragMoveEvent (QDragMoveEvent *e) |
virtual void | dropEvent (QDropEvent *e) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | inputMethodEvent (QInputMethodEvent *e) |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery property) const |
virtual void | insertFromMimeData (const QMimeData *source) |
virtual void | keyReleaseEvent (QKeyEvent *e) |
virtual void | mouseDoubleClickEvent (QMouseEvent *e) |
virtual void | mouseMoveEvent (QMouseEvent *e) |
virtual void | mousePressEvent (QMouseEvent *e) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
virtual void | resizeEvent (QResizeEvent *e) |
virtual void | scrollContentsBy (int dx, int dy) |
virtual void | showEvent (QShowEvent *) |
![]() | |
void | setupViewport (QWidget *viewport) |
void | setViewportMargins (const QMargins &margins) |
void | setViewportMargins (int left, int top, int right, int bottom) |
virtual bool | viewportEvent (QEvent *event) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Detailed Description
The KRichTextEdit class provides a widget to edit and display rich text.
It offers several additional rich text editing functions to KTextEdit and makes them easier to access including:
- Changing fonts, sizes.
- Font formatting, such as bold, underline, italic, foreground and background color.
- Paragraph alignment
- Ability to edit and remove hyperlinks
- Nested list handling
- Simple actions to insert tables. TODO
The KRichTextEdit can be in two modes: Rich text mode and plain text mode. Calling functions which modify the format/style of the text will automatically enable the rich text mode. Rich text mode is sometimes also referred to as HTML mode.
Do not call setAcceptRichText() or acceptRichText() yourself. Instead simply connect to the slots which insert the rich text, use switchToPlainText() or enableRichTextMode().
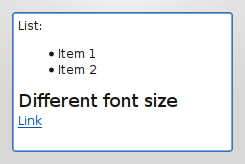
- Since
- 4.1
Definition at line 65 of file krichtextedit.h.
Member Enumeration Documentation
enum KRichTextEdit::Mode |
The mode the edit widget is in.
Enumerator | |
---|---|
Plain |
Plain text mode. |
Rich |
Rich text mode. |
Definition at line 74 of file krichtextedit.h.
Constructor & Destructor Documentation
Constructs a KRichTextEdit object.
Private class that helps to provide binary compatibility between releases.
- Parameters
-
text The initial text of the text edit, which is interpreted as HTML. parent The parent widget
Definition at line 118 of file krichtextedit.cpp.
|
explicit |
Constructs a KRichTextEdit object.
- Parameters
-
parent The parent widget
Definition at line 124 of file krichtextedit.cpp.
|
virtual |
Destructor.
Definition at line 130 of file krichtextedit.cpp.
Member Function Documentation
|
slot |
Sets the alignment of the current block to Centered.
Definition at line 182 of file krichtextedit.cpp.
|
slot |
Sets the alignment of the current block to Justified.
Definition at line 196 of file krichtextedit.cpp.
|
slot |
Sets the alignment of the current block to Left Aligned.
Definition at line 175 of file krichtextedit.cpp.
|
slot |
Sets the alignment of the current block to Right Aligned.
Definition at line 189 of file krichtextedit.cpp.
bool KRichTextEdit::canDedentList | ( | ) | const |
Returns true if the list item at the current position can be dedented.
- See also
- canIndentList
Definition at line 523 of file krichtextedit.cpp.
bool KRichTextEdit::canIndentList | ( | ) | const |
Returns true if the list item at the current position can be indented.
- See also
- canDedentList
Definition at line 518 of file krichtextedit.cpp.
QString KRichTextEdit::currentLinkText | ( | ) | const |
Returns the text of the link at the current position or an empty string if the cursor is not on a link.
- See also
- currentLinkUrl
- Returns
- The link text
Definition at line 371 of file krichtextedit.cpp.
QString KRichTextEdit::currentLinkUrl | ( | ) | const |
Returns the URL target (href) of the link at the current position or an empty string if the cursor is not on a link.
- See also
- currentLinkText
- Returns
- The link target URL
Definition at line 418 of file krichtextedit.cpp.
void KRichTextEdit::enableRichTextMode | ( | ) |
This enables rich text mode.
Nothing is done except changing the internal mode and allowing rich text pastes.
Definition at line 340 of file krichtextedit.cpp.
|
slot |
Decreases the nesting level of the current block or selected blocks.
- See also
- canDedentList
Definition at line 156 of file krichtextedit.cpp.
|
slot |
Increases the nesting level of the current block or selected blocks.
- See also
- canIndentList
Definition at line 150 of file krichtextedit.cpp.
|
slot |
Inserts a horizontal rule below the current block.
Definition at line 161 of file krichtextedit.cpp.
|
slot |
- Since
- 4.10 Because of binary compatibility constraints, insertPlainText is not virtual. Therefore it must dynamically detect and call this slot.
Definition at line 317 of file krichtextedit.cpp.
|
protectedvirtual |
Reimplemented.
Catches key press events. Used to handle some key presses on lists.
Reimplemented from KTextEdit.
Definition at line 485 of file krichtextedit.cpp.
|
slot |
Sets the direction of the current block to Left-To-Right.
- Since
- 4.6
Definition at line 214 of file krichtextedit.cpp.
|
slot |
Sets the direction of the current block to Right-To-Left.
- Since
- 4.6
Definition at line 203 of file krichtextedit.cpp.
|
signal |
Emitted whenever the user has finished making a selection.
(on mouse up)
void KRichTextEdit::selectLinkText | ( | QTextCursor * | cursor | ) | const |
If the cursor is on a link, sets the cursor to a selection of the text of the link.
If the cursor is not on a link, selects the current word or existing selection.
- Parameters
-
cursor The cursor to use to select the text.
- See also
- updateLink
Definition at line 385 of file krichtextedit.cpp.
void KRichTextEdit::selectLinkText | ( | ) | const |
Convenience function to select the link text using the active cursor.
- See also
- selectLinkText
Definition at line 378 of file krichtextedit.cpp.
|
slot |
Sets the current word or selection to the font font.
- Parameters
-
font the font of the text will be set to this font
Definition at line 297 of file krichtextedit.cpp.
|
slot |
Sets the current word or selection to the font family fontFamily.
- Parameters
-
fontFamily The text's font family will be changed to this one
Definition at line 279 of file krichtextedit.cpp.
|
slot |
Sets the current word or selection to the font size size.
- Parameters
-
size The text's font will get this size
Definition at line 288 of file krichtextedit.cpp.
|
slot |
Sets the list style of the current list, or creates a new list using the current block.
The _styleindex corresponds to the QTextListFormat::Style
- Parameters
-
_styleIndex The list will get this style
Definition at line 143 of file krichtextedit.cpp.
|
slot |
Sets the background color of the current word or selection to color.
- Parameters
-
color The text will get this foreground color
Definition at line 270 of file krichtextedit.cpp.
|
slot |
Toggles the bold formatting of the current word or selection at the current cursor position.
- Parameters
-
bold If true, the text will be set to bold
Definition at line 225 of file krichtextedit.cpp.
|
slot |
Sets the foreground color of the current word or selection to color.
- Parameters
-
color The text will get this background color
Definition at line 261 of file krichtextedit.cpp.
|
slot |
Toggles the italic formatting of the current word or selection at the current cursor position.
- Parameters
-
italic If true, the text will be set to italic
Definition at line 234 of file krichtextedit.cpp.
void KRichTextEdit::setTextOrHtml | ( | const QString & | text | ) |
Replaces all the content of the text edit with the given string.
If the string is in rich text format, the text is inserted as rich text, otherwise it is inserted as plain text.
- Parameters
-
text The text to insert
Definition at line 358 of file krichtextedit.cpp.
|
slot |
Toggles the strikeout formatting of the current word or selection at the current cursor position.
- Parameters
-
strikeOut If true, the text will be struck out
Definition at line 252 of file krichtextedit.cpp.
|
slot |
Toggles the subscript formatting of the current word or selection at the current cursor position.
- Parameters
-
subscript If true, the text will be set to subscript
Definition at line 331 of file krichtextedit.cpp.
|
slot |
Toggles the superscript formatting of the current word or selection at the current cursor position.
- Parameters
-
superscript If true, the text will be set to superscript
Definition at line 322 of file krichtextedit.cpp.
|
slot |
Toggles the underline formatting of the current word or selection at the current cursor position.
- Parameters
-
underline If true, the text will be underlined
Definition at line 243 of file krichtextedit.cpp.
|
slot |
This will switch the editor to plain text mode.
All rich text formatting will be destroyed.
Definition at line 306 of file krichtextedit.cpp.
KRichTextEdit::Mode KRichTextEdit::textMode | ( | ) | const |
- Returns
- The current text mode
Definition at line 345 of file krichtextedit.cpp.
|
signal |
Emitted whenever the text mode is changed.
- Parameters
-
mode The new text mode
QString KRichTextEdit::textOrHtml | ( | ) | const |
- Returns
- The plain text string if in plain text mode or the HTML code if in rich text mode. The text is not word-wrapped.
Definition at line 350 of file krichtextedit.cpp.
|
slot |
This will clean some of the bad html produced by the underlying QTextEdit It walks over all lines and cleans up a bit.
Should be improved to produce our own Html.
Definition at line 528 of file krichtextedit.cpp.
Replaces the current selection with a hyperlink with the link URL linkUrl and the link text linkText.
- Parameters
-
linkUrl The link will get this URL as href (target) linkText The link will get this alternative text, which is the text displayed in the text edit.
Definition at line 423 of file krichtextedit.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.