KDEUI
#include <kviewstatesaver.h>
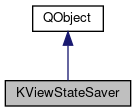
Public Member Functions | |
KViewStateSaver (QObject *parent=0) | |
~KViewStateSaver () | |
QString | currentIndexKey () const |
QStringList | expansionKeys () const |
void | restoreCurrentItem (const QString &indexString) |
void | restoreExpanded (const QStringList &indexStrings) |
void | restoreScrollState (int verticalScoll, int horizontalScroll) |
void | restoreSelection (const QStringList &indexStrings) |
void | restoreState (const KConfigGroup &configGroup) |
void | saveState (KConfigGroup &configGroup) |
QPair< int, int > | scrollState () const |
QStringList | selectionKeys () const |
QItemSelectionModel * | selectionModel () const |
void | setSelectionModel (QItemSelectionModel *selectionModel) |
void | setView (QAbstractItemView *view) |
QAbstractItemView * | view () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual QModelIndex | indexFromConfigString (const QAbstractItemModel *model, const QString &key) const =0 |
virtual QString | indexToConfigString (const QModelIndex &index) const =0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Object for saving and restoring state in QTreeViews and QItemSelectionModels.
Implement the indexFromConfigString and indexToConfigString methods to handle the model in the view whose state is being saved. These implementations can be quite trivial:
It is possible to restore the state of a QTreeView (that is, the expanded state and selected state of all indexes as well as the horizontal and vertical scroll state) by using setTreeView.
If there is no tree view state to restore (for example if using QML), the selection state of a QItemSelectionModel can be saved or restored instead.
The state of any QAbstractScrollArea can also be saved and restored.
A KViewStateSaver should be created on the stack when saving and on the heap when restoring. The model may be populated dynamically between several event loops, so it may not be immediate for the indexes that should be selected to be in the model. The saver should not be persisted as a member. The saver will destroy itself when it has completed the restoration specified in the config group, or a small amount of time has elapsed.
After creating a saver, the state can be saved using a KConfigGroup.
It is also possible to save and restore state directly by using the restoreSelection, restoreExpanded etc methods. Note that the implementation of these methods should return strings that the indexFromConfigString implementation can handle.
Note that a single instance of this class should be used with only one widget. That is don't do this:
To save the state of 3 different widgets, use three savers, even if they operate on the same root model.
- Note
- The KViewStateSaver does not take ownership of any widgets set on it.
It is recommended to restore the state on application startup and after the model has been reset, and to save the state on application close and before the model has been reset.
- Since
- 4.5
Definition at line 169 of file kviewstatesaver.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
- Parameters
-
parent standard parent object, but ignored since this object will delete itself
Definition at line 120 of file kviewstatesaver.cpp.
KViewStateSaver::~KViewStateSaver | ( | ) |
Destructor.
Definition at line 127 of file kviewstatesaver.cpp.
Member Function Documentation
QString KViewStateSaver::currentIndexKey | ( | ) | const |
Returns a QString describing the current index in the selection model.
Definition at line 370 of file kviewstatesaver.cpp.
QStringList KViewStateSaver::expansionKeys | ( | ) | const |
Returns a QStringList representing the expanded indexes in the QTreeView.
Definition at line 378 of file kviewstatesaver.cpp.
|
protectedpure virtual |
Reimplement to return an index in the model
described by the unique key key
.
|
protectedpure virtual |
Reimplement to return a unique string for the index
.
void KViewStateSaver::restoreCurrentItem | ( | const QString & | indexString | ) |
Make the index described by indexString
the currentIndex in the selectionModel.
Definition at line 273 of file kviewstatesaver.cpp.
void KViewStateSaver::restoreExpanded | ( | const QStringList & | indexStrings | ) |
Expand the indexes described by indexStrings
in the QTreeView.
Definition at line 308 of file kviewstatesaver.cpp.
void KViewStateSaver::restoreScrollState | ( | int | verticalScoll, |
int | horizontalScroll | ||
) |
Restores the scroll state of the QAbstractScrollArea to the verticalScoll
and horizontalScroll
.
Definition at line 323 of file kviewstatesaver.cpp.
void KViewStateSaver::restoreSelection | ( | const QStringList & | indexStrings | ) |
Select the indexes described by indexStrings
.
Definition at line 354 of file kviewstatesaver.cpp.
void KViewStateSaver::restoreState | ( | const KConfigGroup & | configGroup | ) |
Restores the state from the configGroup
.
Definition at line 196 of file kviewstatesaver.cpp.
void KViewStateSaver::saveState | ( | KConfigGroup & | configGroup | ) |
Saves the state to the configGroup
.
Definition at line 233 of file kviewstatesaver.cpp.
QPair< int, int > KViewStateSaver::scrollState | ( | ) | const |
Returns the vertical and horizontal scroll of the QAbstractScrollArea.
Definition at line 401 of file kviewstatesaver.cpp.
QStringList KViewStateSaver::selectionKeys | ( | ) | const |
Returns a QStringList describing the selection in the selectionModel.
Definition at line 387 of file kviewstatesaver.cpp.
QItemSelectionModel * KViewStateSaver::selectionModel | ( | ) | const |
The QItemSelectionModel whose state is persisted.
Definition at line 152 of file kviewstatesaver.cpp.
void KViewStateSaver::setSelectionModel | ( | QItemSelectionModel * | selectionModel | ) |
Sets the QItemSelectionModel whose state is persisted.
Definition at line 158 of file kviewstatesaver.cpp.
void KViewStateSaver::setView | ( | QAbstractItemView * | view | ) |
Sets the view whose state is persisted.
Definition at line 132 of file kviewstatesaver.cpp.
QAbstractItemView * KViewStateSaver::view | ( | ) | const |
The view whose state is persisted.
Definition at line 146 of file kviewstatesaver.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.