KHTML
#include <dom_text.h>
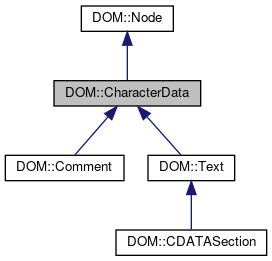
Public Member Functions | |
CharacterData () | |
CharacterData (const CharacterData &other) | |
CharacterData (const Node &other) | |
~CharacterData () | |
void | appendData (const DOMString &arg) |
DOMString | data () const |
void | deleteData (const unsigned long offset, const unsigned long count) |
void | insertData (const unsigned long offset, const DOMString &arg) |
unsigned long | length () const |
CharacterData & | operator= (const Node &other) |
CharacterData & | operator= (const CharacterData &other) |
void | replaceData (const unsigned long offset, const unsigned long count, const DOMString &arg) |
void | setData (const DOMString &) |
DOMString | substringData (const unsigned long offset, const unsigned long count) |
![]() | |
Node () | |
Node (const Node &other) | |
Node (NodeImpl *_impl) | |
virtual | ~Node () |
void | addEventListener (const DOMString &type, EventListener *listener, const bool useCapture) |
Node | appendChild (const Node &newChild) |
void | applyChanges () |
NamedNodeMap | attributes () const |
NodeList | childNodes () const |
Node | cloneNode (bool deep) |
unsigned | compareDocumentPosition (const DOM::Node &other) |
bool | dispatchEvent (const Event &evt) |
quint32 | elementId () const |
Node | firstChild () const |
void | getCursor (int offset, int &_x, int &_y, int &height) |
QRect | getRect () |
NodeImpl * | handle () const |
bool | hasAttributes () |
bool | hasChildNodes () |
unsigned long | index () const |
Node | insertBefore (const Node &newChild, const Node &refChild) |
bool | isNull () const |
bool | isSupported (const DOMString &feature, const DOMString &version) const |
Node | lastChild () const |
DOMString | localName () const |
DOMString | namespaceURI () const |
Node | nextSibling () const |
DOMString | nodeName () const |
unsigned short | nodeType () const |
DOMString | nodeValue () const |
void | normalize () |
bool | operator!= (const Node &other) const |
Node & | operator= (const Node &other) |
bool | operator== (const Node &other) const |
Document | ownerDocument () const |
Node | parentNode () const |
DOMString | prefix () const |
Node | previousSibling () const |
Node | removeChild (const Node &oldChild) |
void | removeEventListener (const DOMString &type, EventListener *listener, bool useCapture) |
Node | replaceChild (const Node &newChild, const Node &oldChild) |
void | setNodeValue (const DOMString &) |
void | setPrefix (const DOMString &prefix) |
void | setTextContent (const DOMString &text) |
DOMString | textContent () const |
QString | toHTML () |
Protected Member Functions | |
CharacterData (CharacterDataImpl *i) | |
Additional Inherited Members | |
![]() | |
enum | DocumentPosition { DOCUMENT_POSITION_DISCONNECTED = 0x01, DOCUMENT_POSITION_PRECEDING = 0x02, DOCUMENT_POSITION_FOLLOWING = 0x04, DOCUMENT_POSITION_CONTAINS = 0x08, DOCUMENT_POSITION_CONTAINED_BY = 0x10, DOCUMENT_POSITION_IMPLEMENTATION_SPECIFIC = 0x20 } |
enum | NodeType { ELEMENT_NODE = 1, ATTRIBUTE_NODE = 2, TEXT_NODE = 3, CDATA_SECTION_NODE = 4, ENTITY_REFERENCE_NODE = 5, ENTITY_NODE = 6, PROCESSING_INSTRUCTION_NODE = 7, COMMENT_NODE = 8, DOCUMENT_NODE = 9, DOCUMENT_TYPE_NODE = 10, DOCUMENT_FRAGMENT_NODE = 11, NOTATION_NODE = 12, XPATH_NAMESPACE_NODE = 13 } |
![]() | |
NodeImpl * | impl |
Detailed Description
The CharacterData
interface extends Node with a set of attributes and methods for accessing character data in the DOM.
For clarity this set is defined here rather than on each object that uses these attributes and methods. No DOM objects correspond directly to CharacterData
, though Text
and others do inherit the interface from it. All offset
s in this interface start from 0.
Definition at line 49 of file dom_text.h.
Constructor & Destructor Documentation
CharacterData::CharacterData | ( | ) |
Definition at line 30 of file dom_text.cpp.
CharacterData::CharacterData | ( | const CharacterData & | other | ) |
Definition at line 34 of file dom_text.cpp.
|
inline |
Definition at line 56 of file dom_text.h.
CharacterData::~CharacterData | ( | ) |
Definition at line 61 of file dom_text.cpp.
|
protected |
Definition at line 146 of file dom_text.cpp.
Member Function Documentation
void CharacterData::appendData | ( | const DOMString & | arg | ) |
Append the string to the end of the character data of the node.
Upon success, data
provides access to the concatenation of data
and the DOMString
specified.
- Parameters
-
arg The DOMString
to append.
- Returns
- Exceptions
-
DOMException NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
Definition at line 102 of file dom_text.cpp.
DOMString CharacterData::data | ( | ) | const |
The character data of the node that implements this interface.
The DOM implementation may not put arbitrary limits on the amount of data that may be stored in a CharacterData
node. However, implementation limits may mean that the entirety of a node's data may not fit into a single DOMString
. In such cases, the user may call substringData
to retrieve the data in appropriately sized pieces.
- Exceptions
-
DOMException DOMSTRING_SIZE_ERR: Raised when it would return more characters than fit in a DOMString
variable on the implementation platform.
Definition at line 65 of file dom_text.cpp.
void CharacterData::deleteData | ( | const unsigned long | offset, |
const unsigned long | count | ||
) |
Remove a range of characters from the node.
Upon success, data
and length
reflect the change.
- Parameters
-
offset The offset from which to remove characters. count The number of characters to delete. If the sum of offset
andcount
exceedslength
then all characters fromoffset
to the end of the data are deleted.
- Returns
- Exceptions
-
DOMException INDEX_SIZE_ERR: Raised if the specified offset is negative or greater than the number of characters in data
, or if the specifiedcount
is negative.
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
Definition at line 124 of file dom_text.cpp.
void CharacterData::insertData | ( | const unsigned long | offset, |
const DOMString & | arg | ||
) |
Insert a string at the specified character offset.
- Parameters
-
offset The character offset at which to insert. arg The DOMString
to insert.
- Returns
- Exceptions
-
DOMException INDEX_SIZE_ERR: Raised if the specified offset is negative or greater than the number of characters in data
.
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
Definition at line 113 of file dom_text.cpp.
unsigned long CharacterData::length | ( | ) | const |
The number of characters that are available through data
and the substringData
method below.
This may have the value zero, i.e., CharacterData
nodes may be empty.
Definition at line 83 of file dom_text.cpp.
CharacterData & CharacterData::operator= | ( | const Node & | other | ) |
Definition at line 38 of file dom_text.cpp.
CharacterData & CharacterData::operator= | ( | const CharacterData & | other | ) |
Definition at line 55 of file dom_text.cpp.
void CharacterData::replaceData | ( | const unsigned long | offset, |
const unsigned long | count, | ||
const DOMString & | arg | ||
) |
Replace the characters starting at the specified character offset with the specified string.
- Parameters
-
offset The offset from which to start replacing. count The number of characters to replace. If the sum of offset
andcount
exceedslength
, then all characters to the end of the data are replaced (i.e., the effect is the same as aremove
method call with the same range, followed by anappend
method invocation).arg The DOMString
with which the range must be replaced.
- Returns
- Exceptions
-
DOMException INDEX_SIZE_ERR: Raised if the specified offset is negative or greater than the number of characters in data
, or if the specifiedcount
is negative.
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
Definition at line 135 of file dom_text.cpp.
void CharacterData::setData | ( | const DOMString & | str | ) |
see data
- Exceptions
-
DOMException NO_MODIFICATION_ALLOWED_ERR: Raised when the node is readonly.
Definition at line 71 of file dom_text.cpp.
DOMString CharacterData::substringData | ( | const unsigned long | offset, |
const unsigned long | count | ||
) |
Extracts a range of data from the node.
- Parameters
-
offset Start offset of substring to extract. count The number of characters to extract.
- Returns
- The specified substring. If the sum of
offset
andcount
exceeds thelength
, then all characters to the end of the data are returned.
- Exceptions
-
DOMException INDEX_SIZE_ERR: Raised if the specified offset is negative or greater than the number of characters in data
, or if the specifiedcount
is negative.
DOMSTRING_SIZE_ERR: Raised if the specified range of text does not fit into a DOMString
.
Definition at line 90 of file dom_text.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.