KHTML
#include <dom_text.h>
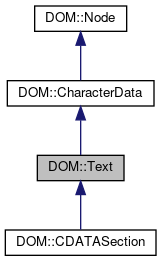
Public Member Functions | |
Text () | |
Text (const Text &other) | |
Text (const Node &other) | |
~Text () | |
Text & | operator= (const Node &other) |
Text & | operator= (const Text &other) |
Text | splitText (const unsigned long offset) |
![]() | |
CharacterData () | |
CharacterData (const CharacterData &other) | |
CharacterData (const Node &other) | |
~CharacterData () | |
void | appendData (const DOMString &arg) |
DOMString | data () const |
void | deleteData (const unsigned long offset, const unsigned long count) |
void | insertData (const unsigned long offset, const DOMString &arg) |
unsigned long | length () const |
CharacterData & | operator= (const Node &other) |
CharacterData & | operator= (const CharacterData &other) |
void | replaceData (const unsigned long offset, const unsigned long count, const DOMString &arg) |
void | setData (const DOMString &) |
DOMString | substringData (const unsigned long offset, const unsigned long count) |
![]() | |
Node () | |
Node (const Node &other) | |
Node (NodeImpl *_impl) | |
virtual | ~Node () |
void | addEventListener (const DOMString &type, EventListener *listener, const bool useCapture) |
Node | appendChild (const Node &newChild) |
void | applyChanges () |
NamedNodeMap | attributes () const |
NodeList | childNodes () const |
Node | cloneNode (bool deep) |
unsigned | compareDocumentPosition (const DOM::Node &other) |
bool | dispatchEvent (const Event &evt) |
quint32 | elementId () const |
Node | firstChild () const |
void | getCursor (int offset, int &_x, int &_y, int &height) |
QRect | getRect () |
NodeImpl * | handle () const |
bool | hasAttributes () |
bool | hasChildNodes () |
unsigned long | index () const |
Node | insertBefore (const Node &newChild, const Node &refChild) |
bool | isNull () const |
bool | isSupported (const DOMString &feature, const DOMString &version) const |
Node | lastChild () const |
DOMString | localName () const |
DOMString | namespaceURI () const |
Node | nextSibling () const |
DOMString | nodeName () const |
unsigned short | nodeType () const |
DOMString | nodeValue () const |
void | normalize () |
bool | operator!= (const Node &other) const |
Node & | operator= (const Node &other) |
bool | operator== (const Node &other) const |
Document | ownerDocument () const |
Node | parentNode () const |
DOMString | prefix () const |
Node | previousSibling () const |
Node | removeChild (const Node &oldChild) |
void | removeEventListener (const DOMString &type, EventListener *listener, bool useCapture) |
Node | replaceChild (const Node &newChild, const Node &oldChild) |
void | setNodeValue (const DOMString &) |
void | setPrefix (const DOMString &prefix) |
void | setTextContent (const DOMString &text) |
DOMString | textContent () const |
QString | toHTML () |
Protected Member Functions | |
Text (TextImpl *i) | |
![]() | |
CharacterData (CharacterDataImpl *i) | |
Additional Inherited Members | |
![]() | |
enum | DocumentPosition { DOCUMENT_POSITION_DISCONNECTED = 0x01, DOCUMENT_POSITION_PRECEDING = 0x02, DOCUMENT_POSITION_FOLLOWING = 0x04, DOCUMENT_POSITION_CONTAINS = 0x08, DOCUMENT_POSITION_CONTAINED_BY = 0x10, DOCUMENT_POSITION_IMPLEMENTATION_SPECIFIC = 0x20 } |
enum | NodeType { ELEMENT_NODE = 1, ATTRIBUTE_NODE = 2, TEXT_NODE = 3, CDATA_SECTION_NODE = 4, ENTITY_REFERENCE_NODE = 5, ENTITY_NODE = 6, PROCESSING_INSTRUCTION_NODE = 7, COMMENT_NODE = 8, DOCUMENT_NODE = 9, DOCUMENT_TYPE_NODE = 10, DOCUMENT_FRAGMENT_NODE = 11, NOTATION_NODE = 12, XPATH_NAMESPACE_NODE = 13 } |
![]() | |
NodeImpl * | impl |
Detailed Description
The Text
interface represents the textual content (termed character data in XML) of an Element
or Attr
.
If there is no markup inside an element's content, the text is contained in a single object implementing the Text
interface that is the only child of the element. If there is markup, it is parsed into a list of elements and Text
nodes that form the list of children of the element.
When a document is first made available via the DOM, there is only one Text
node for each block of text. Users may create adjacent Text
nodes that represent the contents of a given element without any intervening markup, but should be aware that there is no way to represent the separations between these nodes in XML or HTML, so they will not (in general) persist between DOM editing sessions. The normalize()
method on Element
merges any such adjacent Text
objects into a single node for each block of text; this is recommended before employing operations that depend on a particular document structure, such as navigation with XPointers
.
Definition at line 269 of file dom_text.h.
Constructor & Destructor Documentation
Text::Text | ( | ) |
Definition at line 190 of file dom_text.cpp.
Text::Text | ( | const Text & | other | ) |
Definition at line 194 of file dom_text.cpp.
|
inline |
Definition at line 277 of file dom_text.h.
Text::~Text | ( | ) |
Definition at line 220 of file dom_text.cpp.
|
protected |
Definition at line 236 of file dom_text.cpp.
Member Function Documentation
Definition at line 198 of file dom_text.cpp.
Definition at line 214 of file dom_text.cpp.
Text Text::splitText | ( | const unsigned long | offset | ) |
Breaks this Text
node into two Text nodes at the specified offset, keeping both in the tree as siblings.
This node then only contains all the content up to the offset
point. And a new Text
node, which is inserted as the next sibling of this node, contains all the content at and after the offset
point.
- Parameters
-
offset The offset at which to split, starting from 0.
- Returns
- The new
Text
node.
- Exceptions
-
DOMException INDEX_SIZE_ERR: Raised if the specified offset is negative or greater than the number of characters in data
.
NO_MODIFICATION_ALLOWED_ERR: Raised if this node is readonly.
Definition at line 224 of file dom_text.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.