KIO
KFileItemList Class Reference
#include <kfileitem.h>
Inheritance diagram for KFileItemList:
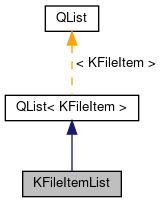
Public Member Functions | |
KFileItemList () | |
KFileItemList (const QList< KFileItem > &items) | |
KFileItem | findByName (const QString &fileName) const |
KFileItem | findByUrl (const KUrl &url) const |
KUrl::List | targetUrlList () const |
KUrl::List | urlList () const |
![]() | |
QList () | |
QList (const QList< T > &other) | |
QList (std::initializer_list< T > args) | |
~QList () | |
void | append (const T &value) |
void | append (const QList< T > &value) |
const T & | at (int i) const |
T & | back () |
const T & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const T &value) const |
int | count (const T &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const T &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const T &t) |
iterator | find (const T &t) |
const_iterator | find (const T &t) const |
const_iterator | find (const_iterator from, const T &t) const |
int | findIndex (const T &t) const |
T & | first () |
const T & | first () const |
T & | front () |
const T & | front () const |
int | indexOf (const T &value, int from) const |
void | insert (int i, const T &value) |
iterator | insert (iterator before, const T &value) |
bool | isEmpty () const |
T & | last () |
const T & | last () const |
int | lastIndexOf (const T &value, int from) const |
int | length () const |
QList< T > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< T > &other) const |
QList< T > | operator+ (const QList< T > &other) const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (const T &value) |
QList< T > & | operator<< (const T &value) |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator= (const QList< T > &other) |
bool | operator== (const QList< T > &other) const |
T & | operator[] (int i) |
const T & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const T &value) |
void | push_back (const T &value) |
void | push_front (const T &value) |
iterator | remove (iterator pos) |
int | remove (const T &t) |
int | removeAll (const T &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const T &value) |
void | replace (int i, const T &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const T &value) const |
void | swap (int i, int j) |
void | swap (QList< T > &other) |
T | takeAt (int i) |
T | takeFirst () |
T | takeLast () |
QSet< T > | toSet () const |
std::list< T > | toStdList () const |
QVector< T > | toVector () const |
T | value (int i, const T &defaultValue) const |
T | value (int i) const |
Additional Inherited Members | |
![]() | |
QList< T > | fromSet (const QSet< T > &set) |
QList< T > | fromStdList (const std::list< T > &list) |
QList< T > | fromVector (const QVector< T > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
List of KFileItems, which adds a few helper methods to QList<KFileItem>.
Definition at line 674 of file kfileitem.h.
Constructor & Destructor Documentation
KFileItemList::KFileItemList | ( | ) |
Creates an empty list of file items.
Definition at line 1719 of file kfileitem.cpp.
Creates a new KFileItemList from a QList of file items
.
Definition at line 1723 of file kfileitem.cpp.
Member Function Documentation
Find a KFileItem by name and return it.
- Returns
- the item with the given name, or a null-item if none was found (see KFileItem::isNull())
Definition at line 1728 of file kfileitem.cpp.
Find a KFileItem by URL and return it.
- Returns
- the item with the given URL, or a null-item if none was found (see KFileItem::isNull())
Definition at line 1740 of file kfileitem.cpp.
KUrl::List KFileItemList::targetUrlList | ( | ) | const |
- Returns
- the list of target URLs that those items represent
- Since
- 4.2
Definition at line 1761 of file kfileitem.cpp.
KUrl::List KFileItemList::urlList | ( | ) | const |
- Returns
- the list of URLs that those items represent
Definition at line 1751 of file kfileitem.cpp.
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.