KIO
#include <scheduler.h>
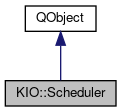
Signals | |
Q_SCRIPTABLE void | reparseSlaveConfiguration (const QString &) |
void | slaveConnected (KIO::Slave *slave) |
void | slaveError (KIO::Slave *slave, int error, const QString &errorMsg) |
Q_SCRIPTABLE void | slaveOnHoldListChanged () |
Public Member Functions | |
bool | connect (const QObject *sender, const char *signal, const char *member) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | assignJobToSlave (KIO::Slave *slave, KIO::SimpleJob *job) |
static void | cancelJob (SimpleJob *job) |
static void | checkSlaveOnHold (bool b) |
static bool | connect (const char *signal, const QObject *receiver, const char *member) |
static bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *member) |
static bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *member) |
static bool | disconnectSlave (KIO::Slave *slave) |
static void | doJob (SimpleJob *job) |
static void | emitReparseSlaveConfiguration () |
static KIO::Slave * | getConnectedSlave (const KUrl &url, const KIO::MetaData &config=MetaData()) |
static bool | isSlaveOnHoldFor (const KUrl &url) |
static void | jobFinished (KIO::SimpleJob *job, KIO::Slave *slave) |
static void | publishSlaveOnHold () |
static void | putSlaveOnHold (KIO::SimpleJob *job, const KUrl &url) |
static void | registerWindow (QWidget *wid) |
static void | removeSlaveOnHold () |
static void | scheduleJob (SimpleJob *job) |
static void | setJobPriority (SimpleJob *job, int priority) |
static void | unregisterWindow (QObject *wid) |
static void | updateInternalMetaData (SimpleJob *job) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The KIO::Scheduler manages io-slaves for the application.
It also queues jobs and assigns the job to a slave when one becomes available.
There are 3 possible ways for a job to get a slave:
1. Direct
This is the default. When you create a job the KIO::Scheduler will be notified and will find either an existing slave that is idle or it will create a new slave for the job.
Example:
2. Scheduled
If you create a lot of jobs, you might want not want to have a slave for each job. If you schedule a job, a maximum number of slaves will be created. When more jobs arrive, they will be queued. When a slave is finished with a job, it will be assigned a job from the queue.
Example:
3. Connection Oriented
For some operations it is important that multiple jobs use the same connection. This can only be ensured if all these jobs use the same slave.
You can ask the scheduler to open a slave for connection oriented operations. You can then use the scheduler to assign jobs to this slave. The jobs will be queued and the slave will handle these jobs one after the other.
Example:
Note that you need to explicitly disconnect the slave when the connection goes down, so your error handler should contain:
- See also
- KIO::Slave
- KIO::Job
Definition at line 109 of file scheduler.h.
Member Function Documentation
|
static |
Uses slave
to do job
.
This function should be called immediately after creating a Job.
- Parameters
-
slave The slave to use. The slave must have been obtained with a call to getConnectedSlave and must not be currently assigned to any other job. job The job to do.
- Returns
- true is successful, false otherwise.
Definition at line 851 of file scheduler.cpp.
|
static |
Stop the execution of a job.
- Parameters
-
job the job to cancel
Definition at line 810 of file scheduler.cpp.
|
static |
When true, the next job will check whether KLauncher has a slave on hold that is suitable for the job.
- Parameters
-
b true when KLauncher has a job on hold
Definition at line 895 of file scheduler.cpp.
|
static |
Function to connect signals emitted by the scheduler.
- See also
- slaveConnected()
- slaveError()
Definition at line 871 of file scheduler.cpp.
|
static |
Definition at line 877 of file scheduler.cpp.
Definition at line 889 of file scheduler.cpp.
|
static |
Definition at line 883 of file scheduler.cpp.
|
static |
Disconnects slave
.
- Parameters
-
slave The slave to disconnect. The slave must have been obtained with a call to getConnectedSlave and must not be assigned to any job.
- Returns
- true is successful, false otherwise.
- See also
- getConnectedSlave
- assignJobToSlave
Definition at line 856 of file scheduler.cpp.
|
static |
Register job
with the scheduler.
The default is to create a new slave for the job if no slave is available. This can be changed by calling setJobPriority.
- Parameters
-
job the job to register
Definition at line 793 of file scheduler.cpp.
|
static |
Definition at line 900 of file scheduler.cpp.
|
static |
Requests a slave for use in connection-oriented mode.
- Parameters
-
url This defines the username,password,host & port to connect with. config Configuration data for the slave.
- Returns
- A pointer to a connected slave or 0 if an error occurred.
- See also
- assignJobToSlave()
- disconnectSlave()
Definition at line 845 of file scheduler.cpp.
Returns true if there is a slave on hold for url
.
- Since
- 4.7
Definition at line 835 of file scheduler.cpp.
|
static |
Called when a job is done.
- Parameters
-
job the finished job slave the slave that executed the job
Definition at line 815 of file scheduler.cpp.
|
static |
Send the slave that was put on hold back to KLauncher.
This allows another process to take over the slave and resume the job that was started.
Definition at line 830 of file scheduler.cpp.
|
static |
Puts a slave on notice.
A next job may reuse this slave if it requests the same URL.
A job can be put on hold after it has emit'ed its mimetype. Based on the mimetype, the program can give control to another component in the same process which can then resume the job by simply asking for the same URL again.
- Parameters
-
job the job that should be stopped url the URL that is handled by the url
Definition at line 820 of file scheduler.cpp.
|
static |
Register the mainwindow wid
with the KIO subsystem Do not call this, it is called automatically from void KIO::Job::setWindow(QWidget*).
- Parameters
-
wid the window to register
Definition at line 861 of file scheduler.cpp.
|
static |
Removes any slave that might have been put on hold.
If a slave was put on hold it will be killed.
Definition at line 825 of file scheduler.cpp.
|
signal |
|
static |
Schedules job
scheduled for later execution.
This method is deprecated and just sets the job's priority to 1. It is recommended to replace calls to scheduleJob(job) with setJobPriority(job, 1).
- Parameters
-
job the job to schedule
Definition at line 799 of file scheduler.cpp.
|
static |
Changes the priority of job
; jobs of the same priority run in the order in which they were created.
Jobs of lower numeric priority always run before any waiting jobs of higher numeric priority. The range of priority is -10 to 10, the default priority of jobs is 0.
- Parameters
-
job the job to change priority new priority of job
, lower runs earlier
Definition at line 805 of file scheduler.cpp.
|
signal |
|
signal |
|
signal |
|
static |
Unregisters the window registered by registerWindow().
Definition at line 866 of file scheduler.cpp.
|
static |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:55 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.