Solid
#include <deviceinterface.h>
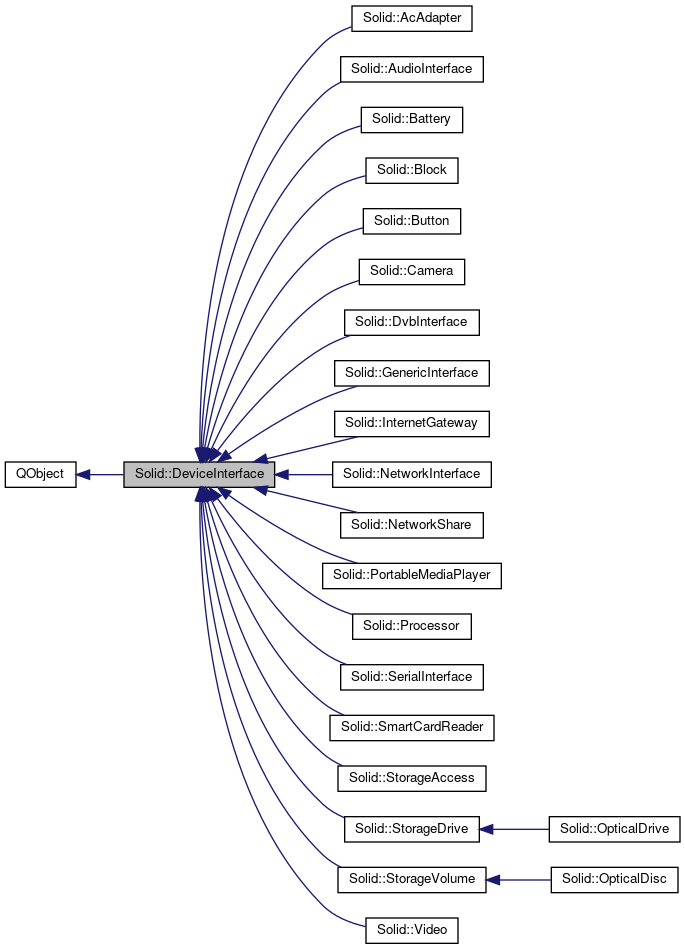
Public Types | |
enum | Type { Unknown = 0, GenericInterface = 1, Processor = 2, Block = 3, StorageAccess = 4, StorageDrive = 5, OpticalDrive = 6, StorageVolume = 7, OpticalDisc = 8, Camera = 9, PortableMediaPlayer = 10, NetworkInterface = 11, AcAdapter = 12, Battery = 13, Button = 14, AudioInterface = 15, DvbInterface = 16, Video = 17, SerialInterface = 18, SmartCardReader = 19, InternetGateway = 20, NetworkShare = 21, Last = 0xffff } |
Public Member Functions | |
virtual | ~DeviceInterface () |
bool | isValid () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
DeviceInterfacePrivate * | d_ptr |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Base class of all the device interfaces.
A device interface describes what a device can do. A device generally has a set of device interfaces.
Definition at line 42 of file deviceinterface.h.
Member Enumeration Documentation
This enum type defines the type of device interface that a Device can have.
- Unknown : An undetermined device interface
- Processor : A processor
- Block : A block device
- StorageAccess : A mechanism to access data on a storage device
- StorageDrive : A storage drive
- OpticalDrive : An optical drive (CD-ROM, DVD, ...)
- StorageVolume : A volume
- OpticalDisc : An optical disc
- Camera : A digital camera
- PortableMediaPlayer: A portable media player
- NetworkInterface: A network interface
- SerialInterface: A serial interface
- SmartCardReader: A smart card reader interface
- NetworkShare: A network share interface
Definition at line 67 of file deviceinterface.h.
Constructor & Destructor Documentation
|
virtual |
Destroys a DeviceInterface object.
Definition at line 38 of file deviceinterface.cpp.
|
protected |
Creates a new DeviceInterface object.
- Parameters
-
dd the private d member. It will take care of deleting it upon destruction. backendObject the device interface object provided by the backend
Definition at line 29 of file deviceinterface.cpp.
Member Function Documentation
bool Solid::DeviceInterface::isValid | ( | ) | const |
Indicates if this device interface is valid.
A device interface is considered valid if the device it is referring is available in the system.
- Returns
- true if this device interface's device is available, false otherwise
Definition at line 44 of file deviceinterface.cpp.
|
static |
- Returns
- the device interface type for the given class name
Definition at line 57 of file deviceinterface.cpp.
- Returns
- a description suitable to display in the UI of the device interface type
- Since
- 4.4
Definition at line 64 of file deviceinterface.cpp.
- Returns
- the class name of the device interface type
Definition at line 50 of file deviceinterface.cpp.
Member Data Documentation
|
protected |
Definition at line 118 of file deviceinterface.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:23:26 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.