Solid
#include <opticaldisc.h>
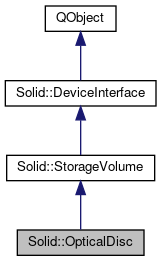
Public Member Functions | |
virtual | ~OpticalDisc () |
ContentTypes | availableContent () const |
qulonglong | capacity () const |
DiscType | discType () const |
bool | isAppendable () const |
bool | isBlank () const |
bool | isRewritable () const |
![]() | |
virtual | ~StorageVolume () |
Device | encryptedContainer () const |
QString | fsType () const |
bool | isIgnored () const |
QString | label () const |
qulonglong | size () const |
UsageType | usage () const |
QString | uuid () const |
![]() | |
virtual | ~DeviceInterface () |
bool | isValid () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static Type | deviceInterfaceType () |
![]() | |
static Type | deviceInterfaceType () |
![]() | |
static Type | stringToType (const QString &type) |
static QString | typeDescription (Type type) |
static QString | typeToString (Type type) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Properties | |
bool | appendable |
ContentTypes | availableContent |
bool | blank |
qulonglong | capacity |
DiscType | discType |
bool | rewritable |
![]() | |
QString | fsType |
bool | ignored |
QString | label |
qulonglong | size |
UsageType | usage |
QString | uuid |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
StorageVolume (StorageVolumePrivate &dd, QObject *backendObject) | |
![]() | |
DeviceInterface (DeviceInterfacePrivate &dd, QObject *backendObject) | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
DeviceInterfacePrivate * | d_ptr |
Detailed Description
This device interface is available on optical discs.
An optical disc is a volume that can be inserted in CD-R*,DVD*,Blu-Ray,HD-DVD drives.
Definition at line 38 of file opticaldisc.h.
Member Enumeration Documentation
This enum type defines the type of content available in an optical disc.
- Audio : A disc containing audio
- Data : A disc containing data
- VideoCd : A Video Compact Disc (VCD)
- SuperVideoCd : A Super Video Compact Disc (SVCD)
- VideoDvd : A Video Digital Versatile Disc (DVD-Video)
Enumerator | |
---|---|
NoContent | |
Audio | |
Data | |
VideoCd | |
SuperVideoCd | |
VideoDvd | |
VideoBluRay |
Definition at line 62 of file opticaldisc.h.
This type stores an OR combination of ContentType values.
This enum type defines the type of optical disc it can be.
- UnknownDiscType : An undetermined disc type
- CdRom : A Compact Disc Read-Only Memory (CD-ROM)
- CdRecordable : A Compact Disc Recordable (CD-R)
- CdRewritable : A Compact Disc ReWritable (CD-RW)
- DvdRom : A Digital Versatile Disc Read-Only Memory (DVD-ROM)
- DvdRam : A Digital Versatile Disc Random Access Memory (DVD-RAM)
- DvdRecordable : A Digital Versatile Disc Recordable (DVD-R)
- DvdRewritable : A Digital Versatile Disc ReWritable (DVD-RW)
- DvdPlusRecordable : A Digital Versatile Disc Recordable (DVD+R)
- DvdPlusRewritable : A Digital Versatile Disc ReWritable (DVD+RW)
- DvdPlusRecordableDuallayer : A Digital Versatile Disc Recordable Dual-Layer (DVD+R DL)
- DvdPlusRewritableDuallayer : A Digital Versatile Disc ReWritable Dual-Layer (DVD+RW DL)
- BluRayRom : A Blu-ray Disc (BD)
- BluRayRecordable : A Blu-ray Disc Recordable (BD-R)
- BluRayRewritable : A Blu-ray Disc (BD-RE)
- HdDvdRom: A High Density Digital Versatile Disc (HD DVD)
- HdDvdRecordable : A High Density Digital Versatile Disc Recordable (HD DVD-R)
- HdDvdRewritable : A High Density Digital Versatile Disc ReWritable (HD DVD-RW)
Definition at line 99 of file opticaldisc.h.
Constructor & Destructor Documentation
|
virtual |
Destroys an OpticalDisc object.
Definition at line 32 of file opticaldisc.cpp.
Member Function Documentation
ContentTypes Solid::OpticalDisc::availableContent | ( | ) | const |
Retrieves the content types this disc contains (audio, video, data...).
- Returns
- the flag set indicating the available contents
- See also
- Solid::OpticalDisc::ContentType
qulonglong Solid::OpticalDisc::capacity | ( | ) | const |
Retrieves the disc capacity (that is the maximum size of a volume could have on this disc).
- Returns
- the capacity of the disc in bytes
|
inlinestatic |
Get the Solid::DeviceInterface::Type of the OpticalDisc device interface.
- Returns
- the OpticalDisc device interface type
- See also
- Solid::Ifaces::Enums::DeviceInterface::Type
Definition at line 132 of file opticaldisc.h.
DiscType Solid::OpticalDisc::discType | ( | ) | const |
Retrieves the disc type (cdr, cdrw...).
- Returns
- the disc type
bool Solid::OpticalDisc::isAppendable | ( | ) | const |
Indicates if it's possible to write additional data to the disc.
- Returns
- true if the disc is appendable, false otherwise
Definition at line 49 of file opticaldisc.cpp.
bool Solid::OpticalDisc::isBlank | ( | ) | const |
Indicates if the disc is blank.
- Returns
- true if the disc is blank, false otherwise
Definition at line 55 of file opticaldisc.cpp.
bool Solid::OpticalDisc::isRewritable | ( | ) | const |
Indicates if the disc is rewritable.
A disc is rewritable if you can write on it several times.
- Returns
- true if the disc is rewritable, false otherwise
Definition at line 61 of file opticaldisc.cpp.
Property Documentation
|
read |
Definition at line 45 of file opticaldisc.h.
|
read |
Definition at line 43 of file opticaldisc.h.
|
read |
Definition at line 46 of file opticaldisc.h.
|
read |
Definition at line 48 of file opticaldisc.h.
|
read |
Definition at line 44 of file opticaldisc.h.
|
read |
Definition at line 47 of file opticaldisc.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:23:26 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.