akonadi
#include <monitor.h>
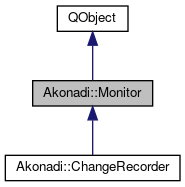
Public Types | |
enum | Type { Collections = 1, Items, Tags } |
Signals | |
void | allMonitored (bool monitored) |
void | collectionAdded (const Akonadi::Collection &collection, const Akonadi::Collection &parent) |
void | collectionChanged (const Akonadi::Collection &collection) |
void | collectionChanged (const Akonadi::Collection &collection, const QSet< QByteArray > &attributeNames) |
void | collectionMonitored (const Akonadi::Collection &collection, bool monitored) |
void | collectionMoved (const Akonadi::Collection &collection, const Akonadi::Collection &source, const Akonadi::Collection &destination) |
void | collectionRemoved (const Akonadi::Collection &collection) |
void | collectionStatisticsChanged (Akonadi::Collection::Id id, const Akonadi::CollectionStatistics &statistics) |
void | collectionSubscribed (const Akonadi::Collection &collection, const Akonadi::Collection &parent) |
void | collectionUnsubscribed (const Akonadi::Collection &collection) |
void | itemAdded (const Akonadi::Item &item, const Akonadi::Collection &collection) |
void | itemChanged (const Akonadi::Item &item, const QSet< QByteArray > &partIdentifiers) |
void | itemLinked (const Akonadi::Item &item, const Akonadi::Collection &collection) |
void | itemMonitored (const Akonadi::Item &item, bool monitored) |
void | itemMoved (const Akonadi::Item &item, const Akonadi::Collection &collectionSource, const Akonadi::Collection &collectionDestination) |
void | itemRemoved (const Akonadi::Item &item) |
void | itemsFlagsChanged (const Akonadi::Item::List &items, const QSet< QByteArray > &addedFlags, const QSet< QByteArray > &removedFlags) |
void | itemsLinked (const Akonadi::Item::List &items, const Akonadi::Collection &collection) |
void | itemsMoved (const Akonadi::Item::List &items, const Akonadi::Collection &collectionSource, const Akonadi::Collection &collectionDestination) |
void | itemsRemoved (const Akonadi::Item::List &items) |
void | itemsTagsChanged (const Akonadi::Item::List &items, const QSet< Akonadi::Tag > &addedTags, const QSet< Akonadi::Tag > &removedTags) |
void | itemsUnlinked (const Akonadi::Item::List &items, const Akonadi::Collection &collection) |
void | itemUnlinked (const Akonadi::Item &item, const Akonadi::Collection &collection) |
void | mimeTypeMonitored (const QString &mimeType, bool monitored) |
void | resourceMonitored (const QByteArray &identifier, bool monitored) |
void | tagAdded (const Akonadi::Tag &tag) |
void | tagChanged (const Akonadi::Tag &tag) |
void | tagMonitored (const Akonadi::Tag &tag, bool monitored) |
void | tagRemoved (const Akonadi::Tag &tag) |
void | typeMonitored (const Akonadi::Monitor::Type type, bool monitored) |
Public Member Functions | |
Monitor (QObject *parent=0) | |
virtual | ~Monitor () |
CollectionFetchScope & | collectionFetchScope () |
Collection::List | collectionsMonitored () const |
void | fetchChangedOnly (bool enable) |
void | fetchCollection (bool enable) |
void | fetchCollectionStatistics (bool enable) |
void | ignoreSession (Session *session) |
bool | isAllMonitored () const |
ItemFetchScope & | itemFetchScope () |
AKONADI_DEPRECATED QList < Item::Id > | itemsMonitored () const |
QVector< Item::Id > | itemsMonitoredEx () const |
QStringList | mimeTypesMonitored () const |
int | numItemsMonitored () const |
int | numMimeTypesMonitored () const |
int | numResourcesMonitored () const |
QList< QByteArray > | resourcesMonitored () const |
Session * | session () const |
void | setAllMonitored (bool monitored=true) |
void | setCollectionFetchScope (const CollectionFetchScope &fetchScope) |
void | setCollectionMonitored (const Collection &collection, bool monitored=true) |
void | setCollectionMoveTranslationEnabled (bool enabled) |
void | setItemFetchScope (const ItemFetchScope &fetchScope) |
void | setItemMonitored (const Item &item, bool monitored=true) |
void | setMimeTypeMonitored (const QString &mimetype, bool monitored=true) |
void | setResourceMonitored (const QByteArray &resource, bool monitored=true) |
void | setSession (Akonadi::Session *session) |
void | setTagFetchScope (const TagFetchScope &fetchScope) |
void | setTagMonitored (const Tag &tag, bool monitored=true) |
void | setTypeMonitored (Type type, bool monitored=true) |
TagFetchScope & | tagFetchScope () |
QVector< Tag::Id > | tagsMonitored () const |
QVector< Type > | typesMonitored () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Monitors an item or collection for changes.
The Monitor emits signals if some of these objects are changed or removed or new ones are added to the Akonadi storage.
There are various ways to filter these notifications. There are three types of filter evaluation:
- (-) removal-only filter, ie. if the filter matches the notification is dropped, if not filter evaluation continues with the next one
- (+) pass-exit filter, ie. if the filter matches the notification is delivered, if not evaluation is continued
- (f) final filter, ie. evaluation ends here if the corresponding filter criteria is set, the notification is delievered depending on the result, evaluation is only continued if no filter criteria is defined
The following filter are available, listed in evaluation order: (1) ignored sessions (-) (2) monitor everything (+) (3a) resource and mimetype filters (f) (items only) (3b) resource filters (f) (collections only) (4) item is monitored (+) (5) collection is monitored (+)
Optionally, the changed objects can be fetched automatically from the server. To enable this, see itemFetchScope() and collectionFetchScope().
Note that as a consequence of rule 3a, it is not possible to monitor (more than zero resources OR more than zero mimetypes) AND more than zero collections.
- Todo:
Distinguish between monitoring collection properties and collection content.
Special case for collection content counts changed
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
Creates a new monitor.
- Parameters
-
parent The parent object.
Definition at line 39 of file monitor.cpp.
|
virtual |
Destroys the monitor.
Definition at line 59 of file monitor.cpp.
Member Function Documentation
|
signal |
This signal is emitted if the Monitor starts or stops monitoring everything.
- Parameters
-
monitored Whether everything is now being monitored or not.
- Since
- 4.3
|
signal |
This signal is emitted if a new collection has been added to a monitored collection in the Akonadi storage.
- Parameters
-
collection The new collection. parent The parent collection.
|
signal |
This signal is emitted if a monitored collection has been changed (properties or content).
- Parameters
-
collection The changed collection.
|
signal |
This signal is emitted if a monitored collection has been changed (properties or attributes).
- Parameters
-
collection The changed collection. attributeNames The names of the collection attributes that have been changed.
- Since
- 4.4
CollectionFetchScope & Monitor::collectionFetchScope | ( | ) |
Returns the collection fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the CollectionFetchScope documentation for an example.
- Returns
- a reference to the current collection fetch scope
- See also
- setCollectionFetchScope() for replacing the current collection fetch scope
- Since
- 4.4
Definition at line 255 of file monitor.cpp.
|
signal |
This signal is emitted if the Monitor starts or stops monitoring collection
explicitly.
- Parameters
-
collection The collection monitored Whether the collection is now being monitored or not.
- Since
- 4.3
|
signal |
This signals is emitted if a monitored collection has been moved.
- Parameters
-
collection The moved collection. source The previous parent collection. destination The new parent collection.
- Since
- 4.4
|
signal |
This signal is emitted if a monitored collection has been removed from the Akonadi storage.
- Parameters
-
collection The removed collection.
Akonadi::Collection::List Monitor::collectionsMonitored | ( | ) | const |
Returns the list of collections being monitored.
- Since
- 4.3
Definition at line 273 of file monitor.cpp.
|
signal |
This signal is emitted if the statistics information of a monitored collection has changed.
- Parameters
-
id The collection identifier of the changed collection. statistics The updated collection statistics, invalid if automatic fetching of statistics changes is disabled.
|
signal |
This signal is emitted if a collection has been subscribed to by the user.
It will be emitted even for unmonitored collections as the check for whether to monitor it has not been applied yet.
- Parameters
-
collection The subscribed collection parent The parent collection of the subscribed collection.
- Since
- 4.6
|
signal |
This signal is emitted if a user unsubscribes from a collection.
- Parameters
-
collection The unsubscribed collection
- Since
- 4.6
void Monitor::fetchChangedOnly | ( | bool | enable | ) |
Instructs the monitor to fetch only those parts that were changed and were requested in the fetch scope.
This is taken in account only for item modifications. Example usage:
In the example if an item was changed, but its payload was not, the full payload will not be retrieved. If the item's payload was changed, the monitor retrieves the changed payload as well.
The default is to fetch everything requested.
- Since
- 4.8
- Parameters
-
enable true
to enable the feature,false
means everything that was requested will be fetched.
- Returns
- void
Definition at line 243 of file monitor.cpp.
void Monitor::fetchCollection | ( | bool | enable | ) |
Enables automatic fetching of changed collections from the Akonadi storage.
- Parameters
-
enable true
enables automatic fetching,false
disables automatic fetching.
Definition at line 219 of file monitor.cpp.
void Monitor::fetchCollectionStatistics | ( | bool | enable | ) |
Enables automatic fetching of changed collection statistics information from the Akonadi storage.
- Parameters
-
enable true
to enables automatic fetching,false
disables automatic fetching.
Definition at line 225 of file monitor.cpp.
void Monitor::ignoreSession | ( | Session * | session | ) |
Ignores all change notifications caused by the given session.
This overrides all other settings on this session.
- Parameters
-
session The session you want to ignore.
Definition at line 206 of file monitor.cpp.
bool Monitor::isAllMonitored | ( | ) | const |
|
signal |
This signal is emitted if an item has been added to a monitored collection in the Akonadi storage.
- Parameters
-
item The new item. collection The collection the item has been added to.
|
signal |
This signal is emitted if a monitored item has changed, e.g.
item parts have been modified.
- Parameters
-
item The changed item. partIdentifiers The identifiers of the item parts that has been changed.
ItemFetchScope & Monitor::itemFetchScope | ( | ) |
Returns the item fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the ItemFetchScope documentation for an example.
- Returns
- a reference to the current item fetch scope
- See also
- setItemFetchScope() for replacing the current item fetch scope
Definition at line 237 of file monitor.cpp.
|
signal |
This signal is emitted if a reference to an item is added to a virtual collection.
- Parameters
-
item The linked item. collection The collection the item is linked to.
- Since
- 4.2
|
signal |
This signal is emitted if the Monitor starts or stops monitoring item
explicitly.
- Parameters
-
item The item monitored Whether the item is now being monitored or not.
- Since
- 4.3
|
signal |
This signal is emitted if a monitored item has been moved between two collections.
- Parameters
-
item The moved item. collectionSource The collection the item has been moved from. collectionDestination The collection the item has been moved to.
|
signal |
This signal is emitted if.
- a monitored item has been removed from the Akonadi storage or
- a item has been removed from a monitored collection.
- Parameters
-
item The removed item.
|
signal |
This signal is emitted if flags of monitored items have changed.
- Parameters
-
items Items that were changed addedFlags Flags that have been added to each item in items
removedFlags Flags that have been removed from each item in items
- Since
- 4.11
|
signal |
This signal is emitted if a reference to multiple items is added to a virtual collection.
- Parameters
-
items The linked items collection The collections the items are linked to
- Since
- 4.11
QList< Item::Id > Monitor::itemsMonitored | ( | ) | const |
Returns the set of items being monitored.
- Since
- 4.3
- Deprecated:
- Use itemsMonitoredEx() instead.
Definition at line 279 of file monitor.cpp.
QVector< Item::Id > Monitor::itemsMonitoredEx | ( | ) | const |
Returns the set of items being monitored.
Faster version (at least on 32-bit systems) of itemsMonitored().
- Since
- 4.6
Definition at line 285 of file monitor.cpp.
|
signal |
This is signal is emitted when multiple monitored items have been moved between two collections.
- Parameters
-
items Moved items collectionSource The collection the items have been moved from. collectionDestination The collection the items have been moved to.
- Since
- 4.11
|
signal |
This signal is emitted if monitored items have been removed from Akonadi storage of items have been removed from a monitored collection.
- Parameters
-
items Removed items
- Since
- 4.11
|
signal |
This signal is emitted if tags of monitored items have changed.
- Parameters
-
items Items that were changed addedTags Tags that have been added to each item in items
.removedTags Tags that have been removed from each item in items
- Since
- 4.13
|
signal |
This signal is emitted if a refernece to items is removed from a virtual collection.
- Parameters
-
items The unlinked items collection The collections the items are unlinked from
- Since
- 4.11
|
signal |
This signal is emitted if a reference to an item is removed from a virtual collection.
- Parameters
-
item The unlinked item. collection The collection the item is unlinked from.
- Since
- 4.2
|
signal |
This signal is emitted if the Monitor starts or stops monitoring mimeType
explicitly.
- Parameters
-
mimeType The mimeType. monitored Whether the mimeType is now being monitored or not.
- Since
- 4.3
QStringList Monitor::mimeTypesMonitored | ( | ) | const |
int Monitor::numItemsMonitored | ( | ) | const |
Returns the number of items being monitored.
Optimization.
- Since
- 4.14.3
Definition at line 294 of file monitor.cpp.
int Monitor::numMimeTypesMonitored | ( | ) | const |
Returns the number of mimetypes being monitored.
Optimization.
- Since
- 4.14.3
Definition at line 324 of file monitor.cpp.
int Monitor::numResourcesMonitored | ( | ) | const |
Returns the number of resources being monitored.
Optimization.
- Since
- 4.14.3
Definition at line 336 of file monitor.cpp.
|
signal |
This signal is emitted if the Monitor starts or stops monitoring the resource with the identifier identifier
explicitly.
- Parameters
-
identifier The identifier of the resource. monitored Whether the resource is now being monitored or not.
- Since
- 4.3
QList< QByteArray > Monitor::resourcesMonitored | ( | ) | const |
Returns the set of identifiers for resources being monitored.
- Since
- 4.3
Definition at line 330 of file monitor.cpp.
Session * Monitor::session | ( | ) | const |
Returns the Session used by the monitor to communicate with Akonadi.
- Since
- 4.4
Definition at line 365 of file monitor.cpp.
void Akonadi::Monitor::setAllMonitored | ( | bool | monitored = true | ) |
Sets whether all items shall be monitored.
- Parameters
-
monitored sets all items as monitored if set as true
Note that if a session is being ignored, this takes precedence over setAllMonitored() on that session.
Definition at line 186 of file monitor.cpp.
void Monitor::setCollectionFetchScope | ( | const CollectionFetchScope & | fetchScope | ) |
Sets the collection fetch scope.
Controls which collections are monitored and how much of a collection's data is fetched from the server.
- Parameters
-
fetchScope The new scope for collection fetch operations.
- See also
- collectionFetchScope()
- Since
- 4.4
Definition at line 249 of file monitor.cpp.
void Monitor::setCollectionMonitored | ( | const Collection & | collection, |
bool | monitored = true |
||
) |
Sets whether the specified collection shall be monitored for changes.
If monitoring is turned on for the collection, all notifications for items in that collection will be emitted, and its child collections will also be monitored. Note that move notifications will be emitted if either one of the collections involved is being monitored.
Note that if a session is being ignored, this takes precedence over setCollectionMonitored() on that session.
- Parameters
-
collection The collection to monitor. If this collection is Collection::root(), all collections in the Akonadi storage will be monitored. monitored Whether to monitor the collection.
Definition at line 66 of file monitor.cpp.
void Monitor::setCollectionMoveTranslationEnabled | ( | bool | enabled | ) |
Allows to enable/disable collection move translation.
If enabled (the default), move notifications are automatically translated into add/remove notifications if the source/destination is outside of the monitored collection hierarchy.
- Parameters
-
enabled enables collection move translation if set as true
- Since
- 4.9
Definition at line 371 of file monitor.cpp.
void Monitor::setItemFetchScope | ( | const ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
Controls how much of an item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- itemFetchScope()
Definition at line 231 of file monitor.cpp.
void Monitor::setItemMonitored | ( | const Item & | item, |
bool | monitored = true |
||
) |
Sets whether the specified item shall be monitored for changes.
Note that if a session is being ignored, this takes precedence over setItemMonitored() on that session.
- Parameters
-
item The item to monitor. monitored Whether to monitor the item.
Definition at line 86 of file monitor.cpp.
void Monitor::setMimeTypeMonitored | ( | const QString & | mimetype, |
bool | monitored = true |
||
) |
Sets whether items of the specified mime type shall be monitored for changes.
If monitoring is turned on for the mime type, all notifications for items matching that mime type will be emitted, but notifications for collections matching that mime type will only be emitted if this is otherwise specified, for example by setCollectionMonitored().
Note that if a session is being ignored, this takes precedence over setMimeTypeMonitored() on that session.
- Parameters
-
mimetype The mime type to monitor. monitored Whether to monitor the mime type.
Definition at line 126 of file monitor.cpp.
void Monitor::setResourceMonitored | ( | const QByteArray & | resource, |
bool | monitored = true |
||
) |
Sets whether the specified resource shall be monitored for changes.
If monitoring is turned on for the resource, all notifications for collections and items in that resource will be emitted.
Note that if a session is being ignored, this takes precedence over setResourceMonitored() on that session.
- Parameters
-
resource The resource identifier. monitored Whether to monitor the resource.
Definition at line 106 of file monitor.cpp.
void Monitor::setSession | ( | Akonadi::Session * | session | ) |
Sets the session used by the Monitor to communicate with the Akonadi server.
If not set, the Akonadi::Session::defaultSession is used.
- Parameters
-
session the session to be set
- Since
- 4.4
Definition at line 348 of file monitor.cpp.
void Monitor::setTagFetchScope | ( | const TagFetchScope & | fetchScope | ) |
Sets the tag fetch scope.
Controls how much of an tag's data is fetched from the server.
- Parameters
-
fetchScope The new scope for tag fetch operations.
- See also
- tagFetchScope()
Definition at line 261 of file monitor.cpp.
void Monitor::setTagMonitored | ( | const Tag & | tag, |
bool | monitored = true |
||
) |
Sets whether the specified tag shall be monitored for changes.
Same rules as for item monitoring apply.
- Parameters
-
tag Tag to monitor. monitored Whether to monitor the tag.
- Since
- 4.13
Definition at line 146 of file monitor.cpp.
void Monitor::setTypeMonitored | ( | Monitor::Type | type, |
bool | monitored = true |
||
) |
Sets whether given type (Collection, Item, Tag should be monitored).
By default all types are monitored, but once you change one, you have to explicitly enable all other types you want to monitor.
- Parameters
-
type Type to monitor. monitored Whether to monitor the type
- Since
- 4.13
Definition at line 166 of file monitor.cpp.
|
signal |
This signal is emitted if a tag has been added to Akonadi storage.
- Parameters
-
tag The added tag
- Since
- 4.13
|
signal |
This signal is emitted if a monitored tag is changed on the server.
- Parameters
-
tag The changed tag.
- Since
- 4.13
TagFetchScope & Monitor::tagFetchScope | ( | ) |
Returns the tag fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable.
- Returns
- a reference to the current tag fetch scope
- See also
- setTagFetchScope() for replacing the current tag fetch scope
Definition at line 267 of file monitor.cpp.
|
signal |
This signal is emitted if the Monitor starts or stops monitoring tag
explicitly.
- Parameters
-
tag The tag. monitored Whether the tag is now being monitored or not.
- Since
- 4.13
|
signal |
This signal is emitted if a monitored tag is removed from the server storage.
The monitor will also emit itemTagsChanged() signal for all monitored items (if any) that were tagged by tag
.
- Parameters
-
tag The removed tag.
- Since
- 4.13
QVector< Tag::Id > Monitor::tagsMonitored | ( | ) | const |
|
signal |
This signal is emitted if the Monitor starts or stops monitoring type
explicitly.
- Parameters
-
type The type. monitored Whether the type is now being monitored or not.
- Since
- 4.13
QVector< Monitor::Type > Monitor::typesMonitored | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.