KCal Library
#include <attendee.h>
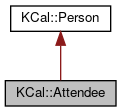
Public Types | |
typedef ListBase< Attendee > | List |
enum | PartStat { NeedsAction, Accepted, Declined, Tentative, Delegated, Completed, InProcess, None } |
enum | Role { ReqParticipant, OptParticipant, NonParticipant, Chair } |
Public Member Functions | |
Attendee (const QString &name, const QString &email, bool rsvp=false, PartStat status=None, Role role=ReqParticipant, const QString &uid=QString()) | |
Attendee (const Attendee &attendee) | |
~Attendee () | |
CustomProperties & | customProperties () |
const CustomProperties & | customProperties () const |
QString | delegate () const |
QString | delegator () const |
Attendee & | operator= (const Attendee &attendee) |
bool | operator== (const Attendee &attendee) |
Role | role () const |
QString | roleStr () const |
bool | RSVP () const |
void | setCustomProperty (const QByteArray &xname, const QString &xvalue) |
void | setDelegate (const QString &delegate) |
void | setDelegator (const QString &delegator) |
void | setRole (Role role) |
void | setRSVP (bool rsvp) |
void | setStatus (PartStat status) |
void | setUid (const QString &uid) |
PartStat | status () const |
QString | statusStr () const |
QString | uid () const |
Static Public Member Functions | |
static QStringList | roleList () |
static QString | roleName (Role role) |
static QStringList | statusList () |
static QString | statusName (PartStat status) |
Detailed Description
Represents information related to an attendee of an Calendar Incidence, typically a meeting or task (to-do).
Attendees are people with a name and (optional) email address who are invited to participate in some way in a meeting or task. This class also tracks that status of the invitation: accepted; tentatively accepted; declined; delegated to another person; in-progress; completed.
Attendees may optionally be asked to RSVP ("Respond Please") to the invitation.
Note that each attendee be can optionally associated with a UID (unique identifier) derived from a Calendar Incidence, Email Message, or any other thing you want.
Definition at line 58 of file attendee.h.
Member Typedef Documentation
typedef ListBase<Attendee> KCal::Attendee::List |
List of attendees.
Definition at line 95 of file attendee.h.
Member Enumeration Documentation
The different types of participant status.
The meaning is specific to the incidence type in context.
Enumerator | |
---|---|
NeedsAction |
Event, to-do or journal needs action (default) |
Accepted |
Event, to-do or journal accepted. |
Declined |
Event, to-do or journal declined. |
Tentative |
Event or to-do tentatively accepted. |
Delegated |
Event or to-do delegated. |
Completed |
To-do completed. |
InProcess |
To-do in process of being completed. |
Definition at line 71 of file attendee.h.
enum KCal::Attendee::Role |
The different types of participation roles.
Enumerator | |
---|---|
ReqParticipant |
Participation is required (default) |
OptParticipant |
Participation is optional. |
NonParticipant |
Non-Participant; copied for information purposes. |
Chair |
Chairperson. |
Definition at line 85 of file attendee.h.
Constructor & Destructor Documentation
Attendee::Attendee | ( | const QString & | name, |
const QString & | email, | ||
bool | rsvp = false , |
||
Attendee::PartStat | status = None , |
||
Attendee::Role | role = ReqParticipant , |
||
const QString & | uid = QString() |
||
) |
Constructs an attendee consisting of a Person name (name
) and email address (email
); invitation status and Role; an optional RSVP flag and UID.
Private class that helps to provide binary compatibility between releases.
- Parameters
-
name is person name of the attendee. email is person email address of the attendee. rsvp if true, the attendee is requested to reply to invitations. status is the PartStat status of the attendee. role is the Role of the attendee. uid is the UID of the attendee.
Definition at line 62 of file attendee.cpp.
Attendee::Attendee | ( | const Attendee & | attendee | ) |
Constructs an attendee by copying another attendee.
- Parameters
-
attendee is the attendee to be copied.
Definition at line 74 of file attendee.cpp.
Attendee::~Attendee | ( | ) |
Destroys the attendee.
Definition at line 80 of file attendee.cpp.
Member Function Documentation
CustomProperties & Attendee::customProperties | ( | ) |
Returns a reference to the CustomProperties object.
- Since
- 4.4
Definition at line 260 of file attendee.cpp.
const CustomProperties & Attendee::customProperties | ( | ) | const |
Returns a const reference to the CustomProperties object.
- Since
- 4.4
Definition at line 265 of file attendee.cpp.
QString Attendee::delegate | ( | ) | const |
QString Attendee::delegator | ( | ) | const |
Sets this attendee equal to attendee
.
- Parameters
-
attendee is the attendee to copy.
Definition at line 97 of file attendee.cpp.
bool KCal::Attendee::operator== | ( | const Attendee & | attendee | ) |
Compares this with attendee
for equality.
- Parameters
-
attendee the attendee to compare.
Definition at line 85 of file attendee.cpp.
Attendee::Role Attendee::role | ( | ) | const |
|
static |
Returns a list of strings representing each Role.
Definition at line 224 of file attendee.cpp.
|
static |
QString Attendee::roleStr | ( | ) | const |
Returns the attendee Role as a text string.
- See also
- role(), roleName()
Definition at line 190 of file attendee.cpp.
bool Attendee::RSVP | ( | ) | const |
void Attendee::setCustomProperty | ( | const QByteArray & | xname, |
const QString & | xvalue | ||
) |
Adds a custom property.
If the property already exists it will be overwritten.
- Parameters
-
xname is the name of the property. xvalue is its value.
- Since
- 4.4
Definition at line 255 of file attendee.cpp.
void Attendee::setDelegate | ( | const QString & | delegate | ) |
Sets the delegate.
- Parameters
-
delegate is a string containing a MAILTO URI of those delegated to attend the meeting.
- See also
- delegate(), setDelegator().
Definition at line 235 of file attendee.cpp.
void Attendee::setDelegator | ( | const QString & | delegator | ) |
Sets the delegator.
- Parameters
-
delegator is a string containing a MAILTO URI of those who have delegated their meeting attendance.
- See also
- delegator(), setDelegate().
Definition at line 245 of file attendee.cpp.
void Attendee::setRole | ( | Attendee::Role | role | ) |
Sets the Role of the attendee to role
.
- Parameters
-
role is the Role to use for the attendee.
- See also
- role()
Definition at line 180 of file attendee.cpp.
void Attendee::setRSVP | ( | bool | rsvp | ) |
Sets the RSVP flag of the attendee to rsvp
.
- Parameters
-
rsvp if set (true), the attendee is requested to reply to invitations.
- See also
- RSVP()
Definition at line 110 of file attendee.cpp.
void Attendee::setStatus | ( | Attendee::PartStat | status | ) |
Sets the PartStat of the attendee to status
.
- Parameters
-
status is the PartStat to use for the attendee.
- See also
- status()
Definition at line 120 of file attendee.cpp.
void Attendee::setUid | ( | const QString & | uid | ) |
Sets the UID of the attendee to uid
.
- Parameters
-
uid is the UID to use for the attendee.
- See also
- uid()
Definition at line 195 of file attendee.cpp.
Attendee::PartStat Attendee::status | ( | ) | const |
Returns the PartStat of the attendee.
- See also
- setStatus()
Definition at line 125 of file attendee.cpp.
|
static |
Returns a list of strings representing each PartStat.
Definition at line 166 of file attendee.cpp.
|
static |
Returns the specified PartStat status
as a text string.
- Parameters
-
status is a PartStat value.
- See also
- status(), statusStr()
Definition at line 135 of file attendee.cpp.
QString Attendee::statusStr | ( | ) | const |
Returns the attendee PartStat as a text string.
- See also
- status(), statusName()
Definition at line 130 of file attendee.cpp.
QString Attendee::uid | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:30 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.