KCal Library
#include <incidence.h>
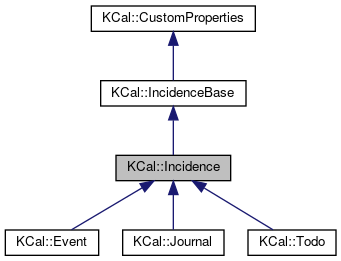
Public Types | |
typedef boost::shared_ptr < const Incidence > | ConstPtr |
typedef ListBase< Incidence > | List |
typedef boost::shared_ptr < Incidence > | Ptr |
enum | Secrecy { SecrecyPublic =0, SecrecyPrivate =1, SecrecyConfidential =2 } |
enum | Status { StatusNone, StatusTentative, StatusConfirmed, StatusCompleted, StatusNeedsAction, StatusCanceled, StatusInProcess, StatusDraft, StatusFinal, StatusX } |
Public Member Functions | |
Incidence () | |
Incidence (const Incidence &other) | |
~Incidence () | |
void | addAlarm (Alarm *alarm) |
void | addAttachment (Attachment *attachment) |
void | addRelation (Incidence *incidence) |
const Alarm::List & | alarms () const |
Attachment::List | attachments () const |
Attachment::List | attachments (const QString &mime) const |
QStringList | categories () const |
QString | categoriesStr () const |
void | clearAlarms () |
void | clearAttachments () |
void | clearRecurrence () |
void | clearTempFiles () |
virtual Incidence * | clone ()=0 |
KDateTime | created () const |
void | deleteAttachment (Attachment *attachment) |
void | deleteAttachments (const QString &mime) |
QString | description () const |
bool | descriptionIsRich () const |
virtual KDateTime | dtEnd () const |
virtual KDateTime | endDateForStart (const KDateTime &startDt) const |
float & | geoLatitude () const |
float & | geoLongitude () const |
bool | hasGeo () const |
bool | isAlarmEnabled () const |
QString | location () const |
bool | locationIsRich () const |
Alarm * | newAlarm () |
Incidence & | operator= (const Incidence &other) |
bool | operator== (const Incidence &incidence) const |
int | priority () const |
void | recreate () |
Recurrence * | recurrence () const |
ushort | recurrenceType () const |
virtual void | recurrenceUpdated (Recurrence *recurrence) |
bool | recurs () const |
bool | recursAt (const KDateTime &dt) const |
virtual bool | recursOn (const QDate &date, const KDateTime::Spec &timeSpec) const |
Incidence * | relatedTo () const |
QString | relatedToUid () const |
Incidence::List | relations () const |
void | removeAlarm (Alarm *alarm) |
void | removeRelation (Incidence *incidence) |
QStringList | resources () const |
int | revision () const |
QString | richDescription () const |
QString | richLocation () const |
QString | richSummary () const |
QString | schedulingID () const |
Secrecy | secrecy () const |
QString | secrecyStr () const |
void | setAllDay (bool allDay) |
void | setCategories (const QStringList &categories) |
void | setCategories (const QString &catStr) |
void | setCreated (const KDateTime &dt) |
void | setCustomStatus (const QString &status) |
void | setDescription (const QString &description, bool isRich) |
void | setDescription (const QString &description) |
virtual void | setDtStart (const KDateTime &dt) |
void | setGeoLatitude (float geolatitude) |
void | setGeoLongitude (float geolongitude) |
void | setHasGeo (bool hasGeo) |
void | setLocation (const QString &location, bool isRich) |
void | setLocation (const QString &location) |
void | setPriority (int priority) |
void | setReadOnly (bool readonly) |
void | setRelatedTo (Incidence *incidence) |
void | setRelatedToUid (const QString &uid) |
void | setResources (const QStringList &resources) |
void | setRevision (int rev) |
void | setSchedulingID (const QString &sid) |
void | setSecrecy (Secrecy secrecy) |
void | setStatus (Status status) |
void | setSummary (const QString &summary, bool isRich) |
void | setSummary (const QString &summary) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
virtual QList< KDateTime > | startDateTimesForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::LocalZone) const |
virtual QList< KDateTime > | startDateTimesForDateTime (const KDateTime &datetime) const |
Status | status () const |
QString | statusStr () const |
QString | summary () const |
bool | summaryIsRich () const |
QString | writeAttachmentToTempFile (Attachment *attachment) const |
![]() | |
IncidenceBase () | |
IncidenceBase (const IncidenceBase &ib) | |
virtual | ~IncidenceBase () |
virtual bool | accept (Visitor &v) |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
bool | allDay () const |
Attendee * | attendeeByMail (const QString &email) const |
Attendee * | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee * | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
const Attendee::List & | attendees () const |
void | clearAttendees () |
void | clearComments () |
QStringList | comments () const |
virtual KDateTime | dtStart () const |
virtual KCAL_DEPRECATED QString | dtStartDateStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartTimeStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
void | setAllDay (bool allDay) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setLastModified (const KDateTime &lm) |
void | setOrganizer (const Person &organizer) |
void | setOrganizer (const QString &organizer) |
void | setUid (const QString &uid) |
void | startUpdates () |
virtual QByteArray | type () const =0 |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | updated () |
KUrl | uri () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
Static Public Member Functions | |
static QStringList | secrecyList () |
static QString | secrecyName (Secrecy secrecy) |
static QString | statusName (Status status) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
virtual KDateTime | endDateRecurrenceBase () const |
![]() | |
virtual void | customPropertyUpdated () |
Additional Inherited Members | |
![]() | |
bool | mReadOnly |
Detailed Description
Provides the abstract base class common to non-FreeBusy (Events, To-dos, Journals) calendar components known as incidences.
Several properties are not allowed for VFREEBUSY objects (see rfc:2445), so they are not in IncidenceBase. The hierarchy is:
So IncidenceBase contains all properties that are common to all classes, and Incidence contains all additional properties that are common to Events, Todos and Journals, but are not allowed for FreeBusy entries.
Definition at line 68 of file incidence.h.
Member Typedef Documentation
typedef boost::shared_ptr<const Incidence> KCal::Incidence::ConstPtr |
A shared pointer to a non-mutable Incidence.
Definition at line 181 of file incidence.h.
typedef ListBase<Incidence> KCal::Incidence::List |
List of incidences.
Definition at line 171 of file incidence.h.
typedef boost::shared_ptr<Incidence> KCal::Incidence::Ptr |
A shared pointer to an Incidence.
Definition at line 176 of file incidence.h.
Member Enumeration Documentation
The different types of incidence access classifications.
Enumerator | |
---|---|
SecrecyPublic |
Not secret (default) |
SecrecyPrivate |
Secret to the owner. |
SecrecyConfidential |
Secret to the owner and some others. |
Definition at line 162 of file incidence.h.
Template for a class that implements a visitor for adding an Incidence to a resource supporting addEvent(), addTodo() and addJournal() calls.
Template for a class that implements a visitor for deleting an Incidence from a resource supporting deleteEvent(), deleteTodo() and deleteJournal() calls. The different types of overall incidence status or confirmation. The meaning is specific to the incidence type in context.
Definition at line 146 of file incidence.h.
Constructor & Destructor Documentation
Incidence::Incidence | ( | ) |
Constructs an empty incidence.
Private class that helps to provide binary compatibility between releases.
Definition at line 141 of file incidence.cpp.
Incidence::Incidence | ( | const Incidence & | other | ) |
Copy constructor.
- Parameters
-
other is the incidence to copy.
Definition at line 147 of file incidence.cpp.
Incidence::~Incidence | ( | ) |
Destroys an incidence.
Definition at line 197 of file incidence.cpp.
Member Function Documentation
void Incidence::addAlarm | ( | Alarm * | alarm | ) |
Adds an alarm to the incidence.
- Parameters
-
alarm is a pointer to a valid Alarm object.
- See also
- removeAlarm().
Definition at line 918 of file incidence.cpp.
void Incidence::addAttachment | ( | Attachment * | attachment | ) |
Adds an attachment to the incidence.
- Parameters
-
attachment is a pointer to a valid Attachment object.
- See also
- deleteAttachment().
Definition at line 688 of file incidence.cpp.
void Incidence::addRelation | ( | Incidence * | incidence | ) |
Adds an incidence that is related to this one.
- Parameters
-
incidence is a pointer to an Incidence object.
- See also
- removeRelation(), relations().
Definition at line 526 of file incidence.cpp.
const Alarm::List & Incidence::alarms | ( | ) | const |
Returns a list of all incidence alarms.
Definition at line 906 of file incidence.cpp.
Attachment::List Incidence::attachments | ( | ) | const |
Returns a list of all incidence attachments.
- See also
- attachments( const QString &).
Definition at line 715 of file incidence.cpp.
Attachment::List Incidence::attachments | ( | const QString & | mime | ) | const |
Returns a list of all incidence attachments with the specified MIME type.
- Parameters
-
mime is a QString containing the MIME type.
- See also
- attachments().
Definition at line 720 of file incidence.cpp.
QStringList Incidence::categories | ( | ) | const |
Returns the incidence categories as a list of strings.
- See also
- setCategories( const QStringList &), setCategories( Const QString &).
Definition at line 473 of file incidence.cpp.
QString Incidence::categoriesStr | ( | ) | const |
Returns the incidence categories as a comma separated string.
- See also
- categories().
Definition at line 478 of file incidence.cpp.
void Incidence::clearAlarms | ( | ) |
void Incidence::clearAttachments | ( | ) |
Removes all attachments and frees the memory used by them.
Definition at line 732 of file incidence.cpp.
void Incidence::clearRecurrence | ( | ) |
Removes all recurrence and exception rules and dates.
Definition at line 558 of file incidence.cpp.
|
pure virtual |
Returns an exact copy of this incidence.
The returned object is owned by the caller.
Implemented in KCal::Event, KCal::Todo, and KCal::Journal.
KDateTime Incidence::created | ( | ) | const |
Returns the incidence creation date/time.
- See also
- setCreated().
Definition at line 328 of file incidence.cpp.
void Incidence::deleteAttachment | ( | Attachment * | attachment | ) |
Removes the specified attachment from the incidence.
Additionally, the memory used by the attachment is freed.
- Parameters
-
attachment is a pointer to a valid Attachment object.
- See also
- addAttachment(), deleteAttachments().
Definition at line 698 of file incidence.cpp.
void Incidence::deleteAttachments | ( | const QString & | mime | ) |
Removes all attachments of the specified MIME type from the incidence.
The memory used by all the removed attachments is freed.
- Parameters
-
mime is a QString containing the MIME type.
- See also
- deleteAttachment().
Definition at line 703 of file incidence.cpp.
QString Incidence::description | ( | ) | const |
Returns the incidence description.
- See also
- setDescription().
- richDescription().
Definition at line 390 of file incidence.cpp.
bool Incidence::descriptionIsRich | ( | ) | const |
Returns true if incidence description contains RichText; false otherwise.
- See also
- setDescription(), description().
Definition at line 404 of file incidence.cpp.
|
virtual |
Returns the incidence ending date/time.
- See also
- IncidenceBase::dtStart().
Reimplemented in KCal::Event.
Definition at line 358 of file incidence.cpp.
|
virtual |
Returns the end date/time of the incidence occurrence if it starts at specified date/time.
- Parameters
-
startDt is the specified starting date/time.
- Returns
- the corresponding end date/time for the occurrence; or the start date/time if the end date/time is invalid; or the end date/time if the start date/time is invalid.
Definition at line 674 of file incidence.cpp.
|
inlineprotectedvirtual |
Returns the end date/time of the base incidence (e.g.
due date/time for to-dos, end date/time for events). This method must be reimplemented by derived classes.
Reimplemented in KCal::Todo, and KCal::Event.
Definition at line 880 of file incidence.h.
float & Incidence::geoLatitude | ( | ) | const |
Returns the incidence geoLatidude.
- Returns
- incidences geolatitude value
- See also
- setGeoLatitude().
- Since
- 4.3
Definition at line 1010 of file incidence.cpp.
float & Incidence::geoLongitude | ( | ) | const |
Returns the incidence geoLongitude.
- Returns
- incidences geolongitude value
- See also
- setGeoLongitude().
- Since
- 4.3
Definition at line 1025 of file incidence.cpp.
bool Incidence::hasGeo | ( | ) | const |
Returns true if the incidence has geo data, otherwise return false.
- Since
- 4.3
Definition at line 995 of file incidence.cpp.
bool Incidence::isAlarmEnabled | ( | ) | const |
Returns true if any of the incidence alarms are enabled; false otherwise.
Definition at line 936 of file incidence.cpp.
QString Incidence::location | ( | ) | const |
Returns the incidence location.
Do not use with journals.
- See also
- setLocation().
- richLocation().
Definition at line 962 of file incidence.cpp.
bool Incidence::locationIsRich | ( | ) | const |
Returns true if incidence location contains RichText; false otherwise.
- See also
- setLocation(), location().
Definition at line 976 of file incidence.cpp.
Alarm * Incidence::newAlarm | ( | ) |
Create a new incidence alarm.
Definition at line 911 of file incidence.cpp.
Assignment operator.
- Warning
- Not polymorphic. Use AssignmentVisitor for correct assignment of an instance of type IncidenceBase to another instance of type Incidence.
- Parameters
-
other is the Incidence to assign.
- See also
- AssignmentVisitor
Definition at line 221 of file incidence.cpp.
bool Incidence::operator== | ( | const Incidence & | incidence | ) | const |
Compares this with Incidence ib
for equality.
- Warning
- Not polymorphic. Use ComparisonVisitor for correct comparison of two instances of type Incidence.
- Parameters
-
incidence is the Incidence to compare.
- See also
- ComparisonVisitor
Definition at line 235 of file incidence.cpp.
int Incidence::priority | ( | ) | const |
Returns the incidence priority.
- See also
- setPriority().
Definition at line 795 of file incidence.cpp.
void Incidence::recreate | ( | ) |
Recreate event.
The event is made a new unique event, but already stored event information is preserved. Sets uniquie id, creation date, last modification date and revision number.
Definition at line 284 of file incidence.cpp.
Recurrence * Incidence::recurrence | ( | ) | const |
Returns the recurrence rule associated with this incidence.
If there is none, returns an appropriate (non-0) object.
Definition at line 545 of file incidence.cpp.
ushort Incidence::recurrenceType | ( | ) | const |
Definition at line 564 of file incidence.cpp.
|
virtual |
Observer interface for the recurrence class.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
- Parameters
-
recurrence is a pointer to a valid Recurrence object.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
Definition at line 1043 of file incidence.cpp.
bool Incidence::recurs | ( | ) | const |
Definition at line 573 of file incidence.cpp.
bool Incidence::recursAt | ( | const KDateTime & | dt | ) | const |
Definition at line 588 of file incidence.cpp.
|
virtual |
Incidence * Incidence::relatedTo | ( | ) | const |
Returns a pointer for the incidence that is related to this one.
This function should only be used when constructing a calendar before the related incidence exists.
- See also
- setRelatedTo(), relatedToUid().
Definition at line 516 of file incidence.cpp.
QString Incidence::relatedToUid | ( | ) | const |
Returns a UID string for the incidence that is related to this one.
This function should only be used when constructing a calendar before the related incidence exists.
- See also
- setRelatedToUid(), relatedTo().
Definition at line 492 of file incidence.cpp.
Incidence::List Incidence::relations | ( | ) | const |
Returns a list of all incidences related to this one.
- See also
- addRelation, removeRelation().
Definition at line 521 of file incidence.cpp.
void Incidence::removeAlarm | ( | Alarm * | alarm | ) |
Removes the specified alarm from the incidence.
- Parameters
-
alarm is a pointer to a valid Alarm object.
- See also
- addAlarm().
Definition at line 924 of file incidence.cpp.
void Incidence::removeRelation | ( | Incidence * | incidence | ) |
Removes an incidence that is related to this one.
- Parameters
-
incidence is a pointer to an Incidence object.
- See also
- addRelation(), relations().
Definition at line 533 of file incidence.cpp.
QStringList Incidence::resources | ( | ) | const |
Returns the incidence resources as a list of strings.
- See also
- setResources().
Definition at line 780 of file incidence.cpp.
int Incidence::revision | ( | ) | const |
Returns the number of revisions this incidence has seen.
- See also
- setRevision().
Definition at line 344 of file incidence.cpp.
QString Incidence::richDescription | ( | ) | const |
Returns the incidence description in rich text format.
- See also
- setDescription().
- description().
- Since
- 4.1
Definition at line 395 of file incidence.cpp.
QString Incidence::richLocation | ( | ) | const |
Returns the incidence location in rich text format.
- See also
- setLocation().
- location().
- Since
- 4.1
Definition at line 967 of file incidence.cpp.
QString Incidence::richSummary | ( | ) | const |
Returns the incidence summary in rich text format.
- See also
- setSummary().
- summary().
- Since
- 4.1
Definition at line 429 of file incidence.cpp.
QString Incidence::schedulingID | ( | ) | const |
Returns the incidence scheduling ID.
Do not use with journals. If a scheduling ID is not set, then return the incidence UID.
- See also
- setSchedulingID().
Definition at line 986 of file incidence.cpp.
Incidence::Secrecy Incidence::secrecy | ( | ) | const |
Returns the incidence Secrecy.
- See also
- setSecrecy(), secrecyStr().
Definition at line 872 of file incidence.cpp.
|
static |
Returns a list of all available Secrecy types as a list of translated strings.
- See also
- secrecyName().
Definition at line 896 of file incidence.cpp.
|
static |
Returns the translated string form of a specified Secrecy.
- Parameters
-
secrecy is a Secrecy type.
- See also
- secrecyList().
Definition at line 882 of file incidence.cpp.
QString Incidence::secrecyStr | ( | ) | const |
Returns the incidence Secrecy as translated string.
- See also
- secrecy().
Definition at line 877 of file incidence.cpp.
void Incidence::setAllDay | ( | bool | allDay | ) |
Definition at line 305 of file incidence.cpp.
void Incidence::setCategories | ( | const QStringList & | categories | ) |
Sets the incidence category list.
- Parameters
-
categories is a list of category strings.
- See also
- setCategories( const QString &), categories().
Definition at line 443 of file incidence.cpp.
void Incidence::setCategories | ( | const QString & | catStr | ) |
Sets the incidence category list based on a comma delimited string.
- Parameters
-
catStr is a QString containing a list of categories which are delimited by a comma character.
Definition at line 452 of file incidence.cpp.
void Incidence::setCreated | ( | const KDateTime & | dt | ) |
Sets the incidence creation date/time.
It is stored as a UTC date/time.
- Parameters
-
dt is the creation date/time.
- See also
- created().
Definition at line 316 of file incidence.cpp.
void Incidence::setCustomStatus | ( | const QString & | status | ) |
Sets the incidence Status to a non-standard status value.
- Parameters
-
status is a non-standard status string. If empty, the incidence Status will be set to StatusNone.
- See also
- setStatus(), status().
Definition at line 811 of file incidence.cpp.
void Incidence::setDescription | ( | const QString & | description, |
bool | isRich | ||
) |
Sets the incidence description.
- Parameters
-
description is the incidence description string. isRich if true indicates the description string contains richtext.
- See also
- description().
Definition at line 375 of file incidence.cpp.
void Incidence::setDescription | ( | const QString & | description | ) |
Sets the incidence description and tries to guess if the description is rich text.
- Parameters
-
description is the incidence description string.
- See also
- description().
- Since
- 4.1
Definition at line 385 of file incidence.cpp.
|
virtual |
Sets the incidence starting date/time.
- Parameters
-
dt is the starting date/time.
- See also
- IncidenceBase::dtStart().
Reimplemented from KCal::IncidenceBase.
Reimplemented in KCal::Todo.
Definition at line 349 of file incidence.cpp.
void Incidence::setGeoLatitude | ( | float | geolatitude | ) |
Set the incidences geoLatitude.
- Parameters
-
geolatitude is the incidence geolatitude to set
- See also
- geoLatitude().
- Since
- 4.3
Definition at line 1015 of file incidence.cpp.
void Incidence::setGeoLongitude | ( | float | geolongitude | ) |
Set the incidencesgeoLongitude.
- Parameters
-
geolongitude is the incidence geolongitude to set
- See also
- geoLongitude().
- Since
- 4.3
Definition at line 1030 of file incidence.cpp.
void Incidence::setHasGeo | ( | bool | hasGeo | ) |
Sets if the incidence has geo data.
- Parameters
-
hasGeo true if incidence has geo data, otherwise false
- See also
- hasGeo(), geoLatitude(), geoLongitude().
- Since
- 4.3
Definition at line 1000 of file incidence.cpp.
void Incidence::setLocation | ( | const QString & | location, |
bool | isRich | ||
) |
Sets the incidence location.
Do not use with journals.
- Parameters
-
location is the incidence location string. isRich if true indicates the location string contains richtext.
- See also
- location().
Definition at line 946 of file incidence.cpp.
void Incidence::setLocation | ( | const QString & | location | ) |
Sets the incidence location and tries to guess if the location is richtext.
Do not use with journals.
- Parameters
-
location is the incidence location string.
- See also
- location().
- Since
- 4.1
Definition at line 957 of file incidence.cpp.
void Incidence::setPriority | ( | int | priority | ) |
Sets the incidences priority.
The priority must be an integer value between 0 and 9, where 0 is undefined, 1 is the highest, and 9 is the lowest priority (decreasing order).
- Parameters
-
priority is the incidence priority to set.
- See also
- priority().
Definition at line 785 of file incidence.cpp.
|
virtual |
Set readonly state of incidence.
- Parameters
-
readonly If true, the incidence is set to readonly, if false the incidence is set to readwrite.
Reimplemented from KCal::IncidenceBase.
Definition at line 297 of file incidence.cpp.
void Incidence::setRelatedTo | ( | Incidence * | incidence | ) |
Relates another incidence to this one.
This function should only be used when constructing a calendar before the related incidence exists.
- Parameters
-
incidence is a pointer to another incidence object.
- See also
- relatedTo(), setRelatedToUid().
Definition at line 497 of file incidence.cpp.
void Incidence::setRelatedToUid | ( | const QString & | uid | ) |
Relates another incidence to this one, by UID.
This function should only be used when constructing a calendar before the related incidence exists.
- Parameters
-
uid is a QString containing a UID for another incidence.
- See also
- relatedToUid(), setRelatedTo().
Definition at line 483 of file incidence.cpp.
void Incidence::setResources | ( | const QStringList & | resources | ) |
Sets a list of incidence resources.
(Note: resources in this context means items used by the incidence such as money, fuel, hours, etc).
- Parameters
-
resources is a list of resource strings.
- See also
- resources().
Definition at line 770 of file incidence.cpp.
void Incidence::setRevision | ( | int | rev | ) |
Sets the number of revisions this incidence has seen.
- Parameters
-
rev is the incidence revision number.
- See also
- revision().
Definition at line 333 of file incidence.cpp.
void Incidence::setSchedulingID | ( | const QString & | sid | ) |
Set the incidence scheduling ID.
Do not use with journals. This is used for accepted invitations as the place to store the UID of the invitation. It is later used again if updates to the invitation comes in. If we did not set a new UID on incidences from invitations, we can end up with more than one resource having events with the same UID, if you have access to other peoples resources.
- Parameters
-
sid is a QString containing the scheduling ID.
- See also
- schedulingID().
Definition at line 981 of file incidence.cpp.
void Incidence::setSecrecy | ( | Incidence::Secrecy | secrecy | ) |
Sets the incidence Secrecy.
- Parameters
-
secrecy is the incidence Secrecy to set.
- See also
- secrecy(), secrecyStr().
Definition at line 862 of file incidence.cpp.
void Incidence::setStatus | ( | Incidence::Status | status | ) |
Sets the incidence status to a standard Status value.
Note that StatusX cannot be specified.
- Parameters
-
status is the incidence Status to set.
- See also
- status(), setCustomStatus().
Definition at line 800 of file incidence.cpp.
void Incidence::setSummary | ( | const QString & | summary, |
bool | isRich | ||
) |
Sets the incidence summary.
- Parameters
-
summary is the incidence summary string. isRich if true indicates the summary string contains richtext.
- See also
- summary().
Definition at line 409 of file incidence.cpp.
void Incidence::setSummary | ( | const QString & | summary | ) |
Sets the incidence summary and tries to guess if the summary is richtext.
- Parameters
-
summary is the incidence summary string.
- See also
- summary().
- Since
- 4.1
Definition at line 419 of file incidence.cpp.
|
virtual |
Reimplemented from KCal::IncidenceBase.
Reimplemented in KCal::Todo, and KCal::Event.
Definition at line 363 of file incidence.cpp.
|
virtual |
Calculates the start date/time for all recurrences that happen at some time on the given date (might start before that date, but end on or after the given date).
- Parameters
-
date the date when the incidence should occur timeSpec time specification for date
.
- Returns
- the start date/time of all occurrences that overlap with the given date; an empty list if the incidence does not overlap with the date at all.
Definition at line 593 of file incidence.cpp.
|
virtual |
Calculates the start date/time for all recurrences that happen at the given time.
- Parameters
-
datetime the date/time when the incidence should occur.
- Returns
- the start date/time of all occurrences that overlap with the given date/time; an empty list if the incidence does not happen at the given time at all.
Definition at line 634 of file incidence.cpp.
Incidence::Status Incidence::status | ( | ) | const |
Returns the incidence Status.
- See also
- setStatus(), setCustomStatus(), statusStr().
Definition at line 822 of file incidence.cpp.
|
static |
Returns the translated string form of a specified Status.
- Parameters
-
status is a Status type.
Definition at line 836 of file incidence.cpp.
QString Incidence::statusStr | ( | ) | const |
Returns the incidence Status as translated string.
- See also
- status().
Definition at line 827 of file incidence.cpp.
QString Incidence::summary | ( | ) | const |
Returns the incidence summary.
- See also
- setSummary().
- richSummary().
Definition at line 424 of file incidence.cpp.
bool Incidence::summaryIsRich | ( | ) | const |
Returns true if incidence summary contains RichText; false otherwise.
- See also
- setSummary(), summary().
Definition at line 438 of file incidence.cpp.
QString Incidence::writeAttachmentToTempFile | ( | Attachment * | attachment | ) | const |
Writes the data in the attachment attachment
to a temporary file and returns the local name of the temporary file.
Definition at line 737 of file incidence.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:30 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.