KCal Library
#include <todo.h>
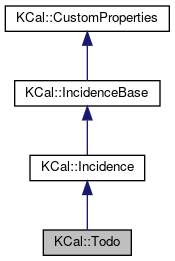
Public Types | |
typedef boost::shared_ptr < const Todo > | ConstPtr |
typedef ListBase< Todo > | List |
typedef boost::shared_ptr< Todo > | Ptr |
![]() | |
typedef boost::shared_ptr < const Incidence > | ConstPtr |
typedef ListBase< Incidence > | List |
typedef boost::shared_ptr < Incidence > | Ptr |
enum | Secrecy { SecrecyPublic =0, SecrecyPrivate =1, SecrecyConfidential =2 } |
enum | Status { StatusNone, StatusTentative, StatusConfirmed, StatusCompleted, StatusNeedsAction, StatusCanceled, StatusInProcess, StatusDraft, StatusFinal, StatusX } |
Public Member Functions | |
Todo () | |
Todo (const Todo &other) | |
~Todo () | |
Todo * | clone () |
KDateTime | completed () const |
QString | completedStr (bool shortfmt=false) const |
KDateTime | dtDue (bool first=false) const |
KCAL_DEPRECATED QString | dtDueDateStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
KCAL_DEPRECATED QString | dtDueStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
KCAL_DEPRECATED QString | dtDueTimeStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
KDateTime | dtRecurrence () const |
virtual KDateTime | dtStart () const |
KDateTime | dtStart (bool first) const |
KCAL_DEPRECATED QString | dtStartDateStr (bool shortfmt, bool first, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartDateStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
KCAL_DEPRECATED QString | dtStartStr (bool shortfmt, bool first, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
KCAL_DEPRECATED QString | dtStartTimeStr (bool shortfmt, bool first, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartTimeStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
bool | hasCompletedDate () const |
bool | hasDueDate () const |
bool | hasStartDate () const |
bool | isCompleted () const |
bool | isInProgress (bool first) const |
bool | isNotStarted (bool first) const |
bool | isOpenEnded () const |
bool | isOverdue () const |
Todo & | operator= (const Todo &other) |
bool | operator== (const Todo &todo) const |
int | percentComplete () const |
virtual bool | recursOn (const QDate &date, const KDateTime::Spec &timeSpec) const |
void | setCompleted (bool completed) |
void | setCompleted (const KDateTime &completeDate) |
void | setDtDue (const KDateTime &dtDue, bool first=false) |
void | setDtRecurrence (const KDateTime &dt) |
void | setDtStart (const KDateTime &dtStart) |
void | setHasDueDate (bool hasDueDate) |
void | setHasStartDate (bool hasStartDate) |
void | setPercentComplete (int percent) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
QByteArray | type () const |
![]() | |
Incidence () | |
Incidence (const Incidence &other) | |
~Incidence () | |
void | addAlarm (Alarm *alarm) |
void | addAttachment (Attachment *attachment) |
void | addRelation (Incidence *incidence) |
const Alarm::List & | alarms () const |
Attachment::List | attachments () const |
Attachment::List | attachments (const QString &mime) const |
QStringList | categories () const |
QString | categoriesStr () const |
void | clearAlarms () |
void | clearAttachments () |
void | clearRecurrence () |
void | clearTempFiles () |
KDateTime | created () const |
void | deleteAttachment (Attachment *attachment) |
void | deleteAttachments (const QString &mime) |
QString | description () const |
bool | descriptionIsRich () const |
virtual KDateTime | dtEnd () const |
virtual KDateTime | endDateForStart (const KDateTime &startDt) const |
float & | geoLatitude () const |
float & | geoLongitude () const |
bool | hasGeo () const |
bool | isAlarmEnabled () const |
QString | location () const |
bool | locationIsRich () const |
Alarm * | newAlarm () |
Incidence & | operator= (const Incidence &other) |
bool | operator== (const Incidence &incidence) const |
int | priority () const |
void | recreate () |
Recurrence * | recurrence () const |
ushort | recurrenceType () const |
virtual void | recurrenceUpdated (Recurrence *recurrence) |
bool | recurs () const |
bool | recursAt (const KDateTime &dt) const |
Incidence * | relatedTo () const |
QString | relatedToUid () const |
Incidence::List | relations () const |
void | removeAlarm (Alarm *alarm) |
void | removeRelation (Incidence *incidence) |
QStringList | resources () const |
int | revision () const |
QString | richDescription () const |
QString | richLocation () const |
QString | richSummary () const |
QString | schedulingID () const |
Secrecy | secrecy () const |
QString | secrecyStr () const |
void | setAllDay (bool allDay) |
void | setCategories (const QStringList &categories) |
void | setCategories (const QString &catStr) |
void | setCreated (const KDateTime &dt) |
void | setCustomStatus (const QString &status) |
void | setDescription (const QString &description, bool isRich) |
void | setDescription (const QString &description) |
void | setGeoLatitude (float geolatitude) |
void | setGeoLongitude (float geolongitude) |
void | setHasGeo (bool hasGeo) |
void | setLocation (const QString &location, bool isRich) |
void | setLocation (const QString &location) |
void | setPriority (int priority) |
void | setReadOnly (bool readonly) |
void | setRelatedTo (Incidence *incidence) |
void | setRelatedToUid (const QString &uid) |
void | setResources (const QStringList &resources) |
void | setRevision (int rev) |
void | setSchedulingID (const QString &sid) |
void | setSecrecy (Secrecy secrecy) |
void | setStatus (Status status) |
void | setSummary (const QString &summary, bool isRich) |
void | setSummary (const QString &summary) |
virtual QList< KDateTime > | startDateTimesForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::LocalZone) const |
virtual QList< KDateTime > | startDateTimesForDateTime (const KDateTime &datetime) const |
Status | status () const |
QString | statusStr () const |
QString | summary () const |
bool | summaryIsRich () const |
QString | writeAttachmentToTempFile (Attachment *attachment) const |
![]() | |
IncidenceBase () | |
IncidenceBase (const IncidenceBase &ib) | |
virtual | ~IncidenceBase () |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
bool | allDay () const |
Attendee * | attendeeByMail (const QString &email) const |
Attendee * | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee * | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
const Attendee::List & | attendees () const |
void | clearAttendees () |
void | clearComments () |
QStringList | comments () const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
void | setAllDay (bool allDay) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setLastModified (const KDateTime &lm) |
void | setOrganizer (const Person &organizer) |
void | setOrganizer (const QString &organizer) |
void | setUid (const QString &uid) |
void | startUpdates () |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | updated () |
KUrl | uri () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
Protected Member Functions | |
virtual KDateTime | endDateRecurrenceBase () const |
![]() | |
virtual void | customPropertyUpdated () |
Additional Inherited Members | |
![]() | |
static QStringList | secrecyList () |
static QString | secrecyName (Secrecy secrecy) |
static QString | statusName (Status status) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
![]() | |
bool | mReadOnly |
Detailed Description
Member Typedef Documentation
typedef boost::shared_ptr<const Todo> KCal::Todo::ConstPtr |
typedef ListBase<Todo> KCal::Todo::List |
typedef boost::shared_ptr<Todo> KCal::Todo::Ptr |
Constructor & Destructor Documentation
KCal::Todo::Todo | ( | ) |
Constructs an empty to-do.
KCal::Todo::Todo | ( | const Todo & | other | ) |
Copy constructor.
- Parameters
-
other is the to-do to copy.
KCal::Todo::~Todo | ( | ) |
Destroys a to-do.
Member Function Documentation
|
virtual |
IncidenceBase::typeStr() Returns an exact copy of this todo. The returned object is owned by the caller.
Implements KCal::Incidence.
KDateTime Todo::completed | ( | ) | const |
QString Todo::completedStr | ( | bool | shortfmt = false | ) | const |
KDateTime Todo::dtDue | ( | bool | first = false | ) | const |
Returns due date and time.
- Parameters
-
first If true and the todo recurs, the due date of the first occurrence will be returned. If false and recurrent, the date of the current occurrence will be returned. If non-recurrent, the normal due date will be returned.
QString Todo::dtDueDateStr | ( | bool | shortfmt = true , |
const KDateTime::Spec & | spec = KDateTime::Spec() |
||
) | const |
Returns due date as string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set, use short date format; else use long format. spec If set, return the date in the given spec, else use the todo's current spec.
QString Todo::dtDueStr | ( | bool | shortfmt = true , |
const KDateTime::Spec & | spec = KDateTime::Spec() |
||
) | const |
Returns due date and time as string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set, use short date format; else use long format. spec If set, return the date/time in the given spec, else use the todo's current spec.
QString Todo::dtDueTimeStr | ( | bool | shortfmt = true , |
const KDateTime::Spec & | spec = KDateTime::Spec() |
||
) | const |
Returns due time as string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set, use short date format; else use long format. spec If set, return the time in the given spec, else use the todo's current spec.
KDateTime Todo::dtRecurrence | ( | ) | const |
|
virtual |
Reimplemented from KCal::IncidenceBase.
KDateTime Todo::dtStart | ( | bool | first | ) | const |
Returns the start date of the todo.
- Parameters
-
first If true, the start date of the todo will be returned; also, if the todo recurs, the start date of the first occurrence will be returned. If false and the todo recurs, the relative start date will be returned, based on the date returned by dtRecurrence().
QString Todo::dtStartDateStr | ( | bool | shortfmt, |
bool | first, | ||
const KDateTime::Spec & | spec = KDateTime::Spec() |
||
) | const |
Returns a todo's starting date as a string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set, use short date format; else use long format. first If true, the start date of the todo will be returned; also, if the todo recurs, the start date of the first occurrence will be returned. If false and the todo recurs, the relative start date will be returned, based on the date returned by dtRecurrence(). spec If set, returns the date in the given spec, else use the todo's current spec.
|
virtual |
IncidenceBase::dtStartDateStr()
Reimplemented from KCal::IncidenceBase.
QString Todo::dtStartStr | ( | bool | shortfmt, |
bool | first, | ||
const KDateTime::Spec & | spec = KDateTime::Spec() |
||
) | const |
Returns a todo's starting date and time as a string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set, use short date format; else use long format. first If true, the start date of the todo will be returned; also, if the todo recurs, the start date of the first occurrence will be returned. If false and the todo recurs, the relative start date will be returned, based on the date returned by dtRecurrence(). spec If set, returns the date and time in the given spec, else use the todo's current spec.
|
virtual |
Reimplemented from KCal::IncidenceBase.
QString Todo::dtStartTimeStr | ( | bool | shortfmt, |
bool | first, | ||
const KDateTime::Spec & | spec = KDateTime::Spec() |
||
) | const |
Returns a todo's starting time as a string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set, use short date format; else use long format. first If true, the start date of the todo will be returned; also, if the todo recurs, the start date of the first occurrence will be returned. If false and the todo recurs, the relative start date will be returned, based on the date returned by dtRecurrence(). spec If set, returns the time in the given spec, else use the todo's current spec.
|
virtual |
IncidenceBase::dtStartTimeStr()
Reimplemented from KCal::IncidenceBase.
|
protectedvirtual |
Returns the end date/time of the base incidence.
Reimplemented from KCal::Incidence.
bool Todo::hasCompletedDate | ( | ) | const |
bool Todo::hasDueDate | ( | ) | const |
bool Todo::hasStartDate | ( | ) | const |
bool Todo::isCompleted | ( | ) | const |
Returns true if the todo is 100% completed, otherwise return false.
- See also
- isOverdue, isInProgress(), isOpenEnded(), isNotStarted(bool), setCompleted(), percentComplete()
bool Todo::isInProgress | ( | bool | first | ) | const |
Returns true, if the to-do is in-progress (started, or >0% completed); otherwise return false.
If the to-do is overdue, then it is not considered to be in-progress.
- Parameters
-
first If true, the start and due dates of the todo will be used; also, if the todo recurs, the start date and due date of the first occurrence will be used. If false and the todo recurs, the relative start and due dates will be used, based on the date returned by dtRecurrence().
- See also
- isOverdue(), isCompleted(), isOpenEnded(), isNotStarted(bool)
- Since
- 4.4
bool Todo::isNotStarted | ( | bool | first | ) | const |
Returns true, if the to-do has yet to be started (no start date and 0% completed); otherwise return false.
- Parameters
-
first If true, the start date of the todo will be used; also, if the todo recurs, the start date of the first occurrence will be used. If false and the todo recurs, the relative start date will be used, based on the date returned by dtRecurrence().
- See also
- isOverdue(), isCompleted(), isInProgress(), isOpenEnded()
- Since
- 4.4
bool Todo::isOpenEnded | ( | ) | const |
Returns true, if the to-do is open-ended (no due date); false otherwise.
- See also
- isOverdue(), isCompleted(), isInProgress(), isNotStarted(bool)
- Since
- 4.4
bool Todo::isOverdue | ( | ) | const |
Returns true if this todo is overdue (e.g.
due date is lower than today and not completed), else false.
- See also
- isCompleted(), isInProgress(), isOpenEnded(), isNotStarted(bool)
Assignment operator.
- Parameters
-
other is the to-do to assign.
bool KCal::Todo::operator== | ( | const Todo & | todo | ) | const |
Compare this with todo
for equality.
- Parameters
-
todo is the to-do to compare.
int Todo::percentComplete | ( | ) | const |
|
virtual |
Returns true if the date
specified is one on which the to-do will recur.
Todos are a special case, hence the overload. It adds an extra check, which make it return false if there's an occurrence between the recur start and today.
- Parameters
-
date is the date to check. timeSpec is the
Reimplemented from KCal::Incidence.
void Todo::setCompleted | ( | bool | completed | ) |
Sets completed state.
- Parameters
-
completed If true set completed state to 100%, if false set completed state to 0%.
- See also
- isCompleted(), percentComplete()
void Todo::setCompleted | ( | const KDateTime & | completeDate | ) |
void KCal::Todo::setDtDue | ( | const KDateTime & | dtDue, |
bool | first = false |
||
) |
Sets due date and time.
- Parameters
-
dtDue The due date/time. first If true and the todo recurs, the due date of the first occurrence will be returned. If false and recurrent, the date of the current occurrence will be returned. If non-recurrent, the normal due date will be returned.
void Todo::setDtRecurrence | ( | const KDateTime & | dt | ) |
|
virtual |
Sets the start date of the todo.
- Parameters
-
dtStart is the to-do start date.
Reimplemented from KCal::Incidence.
void Todo::setHasDueDate | ( | bool | hasDueDate | ) |
void Todo::setHasStartDate | ( | bool | hasStartDate | ) |
void Todo::setPercentComplete | ( | int | percent | ) |
Sets what percentage of the to-do is completed.
Valid values are in the range from 0 to 100.
- Parameters
-
percent is the completion percentage, which as integer value between 0 and 100, inclusive.
- See also
- isCompleted(), setCompleted()
|
virtual |
Reimplemented from KCal::Incidence.
|
virtual |
Implements KCal::IncidenceBase.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:30 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.