KCal Library
KCal::Journal Class Reference
#include <journal.h>
Inheritance diagram for KCal::Journal:
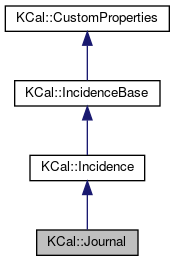
Public Types | |
typedef boost::shared_ptr < const Journal > | ConstPtr |
typedef ListBase< Journal > | List |
typedef boost::shared_ptr < Journal > | Ptr |
![]() | |
typedef boost::shared_ptr < const Incidence > | ConstPtr |
typedef ListBase< Incidence > | List |
typedef boost::shared_ptr < Incidence > | Ptr |
enum | Secrecy { SecrecyPublic =0, SecrecyPrivate =1, SecrecyConfidential =2 } |
enum | Status { StatusNone, StatusTentative, StatusConfirmed, StatusCompleted, StatusNeedsAction, StatusCanceled, StatusInProcess, StatusDraft, StatusFinal, StatusX } |
Public Member Functions | |
Journal () | |
~Journal () | |
Journal * | clone () |
Journal & | operator= (const Journal &other) |
bool | operator== (const Journal &journal) const |
QByteArray | type () const |
![]() | |
Incidence () | |
Incidence (const Incidence &other) | |
~Incidence () | |
void | addAlarm (Alarm *alarm) |
void | addAttachment (Attachment *attachment) |
void | addRelation (Incidence *incidence) |
const Alarm::List & | alarms () const |
Attachment::List | attachments () const |
Attachment::List | attachments (const QString &mime) const |
QStringList | categories () const |
QString | categoriesStr () const |
void | clearAlarms () |
void | clearAttachments () |
void | clearRecurrence () |
void | clearTempFiles () |
KDateTime | created () const |
void | deleteAttachment (Attachment *attachment) |
void | deleteAttachments (const QString &mime) |
QString | description () const |
bool | descriptionIsRich () const |
virtual KDateTime | dtEnd () const |
virtual KDateTime | endDateForStart (const KDateTime &startDt) const |
float & | geoLatitude () const |
float & | geoLongitude () const |
bool | hasGeo () const |
bool | isAlarmEnabled () const |
QString | location () const |
bool | locationIsRich () const |
Alarm * | newAlarm () |
Incidence & | operator= (const Incidence &other) |
bool | operator== (const Incidence &incidence) const |
int | priority () const |
void | recreate () |
Recurrence * | recurrence () const |
ushort | recurrenceType () const |
virtual void | recurrenceUpdated (Recurrence *recurrence) |
bool | recurs () const |
bool | recursAt (const KDateTime &dt) const |
virtual bool | recursOn (const QDate &date, const KDateTime::Spec &timeSpec) const |
Incidence * | relatedTo () const |
QString | relatedToUid () const |
Incidence::List | relations () const |
void | removeAlarm (Alarm *alarm) |
void | removeRelation (Incidence *incidence) |
QStringList | resources () const |
int | revision () const |
QString | richDescription () const |
QString | richLocation () const |
QString | richSummary () const |
QString | schedulingID () const |
Secrecy | secrecy () const |
QString | secrecyStr () const |
void | setAllDay (bool allDay) |
void | setCategories (const QStringList &categories) |
void | setCategories (const QString &catStr) |
void | setCreated (const KDateTime &dt) |
void | setCustomStatus (const QString &status) |
void | setDescription (const QString &description, bool isRich) |
void | setDescription (const QString &description) |
virtual void | setDtStart (const KDateTime &dt) |
void | setGeoLatitude (float geolatitude) |
void | setGeoLongitude (float geolongitude) |
void | setHasGeo (bool hasGeo) |
void | setLocation (const QString &location, bool isRich) |
void | setLocation (const QString &location) |
void | setPriority (int priority) |
void | setReadOnly (bool readonly) |
void | setRelatedTo (Incidence *incidence) |
void | setRelatedToUid (const QString &uid) |
void | setResources (const QStringList &resources) |
void | setRevision (int rev) |
void | setSchedulingID (const QString &sid) |
void | setSecrecy (Secrecy secrecy) |
void | setStatus (Status status) |
void | setSummary (const QString &summary, bool isRich) |
void | setSummary (const QString &summary) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
virtual QList< KDateTime > | startDateTimesForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::LocalZone) const |
virtual QList< KDateTime > | startDateTimesForDateTime (const KDateTime &datetime) const |
Status | status () const |
QString | statusStr () const |
QString | summary () const |
bool | summaryIsRich () const |
QString | writeAttachmentToTempFile (Attachment *attachment) const |
![]() | |
IncidenceBase () | |
IncidenceBase (const IncidenceBase &ib) | |
virtual | ~IncidenceBase () |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
bool | allDay () const |
Attendee * | attendeeByMail (const QString &email) const |
Attendee * | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee * | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
const Attendee::List & | attendees () const |
void | clearAttendees () |
void | clearComments () |
QStringList | comments () const |
virtual KDateTime | dtStart () const |
virtual KCAL_DEPRECATED QString | dtStartDateStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartTimeStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
void | setAllDay (bool allDay) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setLastModified (const KDateTime &lm) |
void | setOrganizer (const Person &organizer) |
void | setOrganizer (const QString &organizer) |
void | setUid (const QString &uid) |
void | startUpdates () |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | updated () |
KUrl | uri () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
Additional Inherited Members | |
![]() | |
static QStringList | secrecyList () |
static QString | secrecyName (Secrecy secrecy) |
static QString | statusName (Status status) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
![]() | |
virtual KDateTime | endDateRecurrenceBase () const |
![]() | |
virtual void | customPropertyUpdated () |
![]() | |
bool | mReadOnly |
Detailed Description
Member Typedef Documentation
typedef boost::shared_ptr<const Journal> KCal::Journal::ConstPtr |
typedef ListBase<Journal> KCal::Journal::List |
typedef boost::shared_ptr<Journal> KCal::Journal::Ptr |
Constructor & Destructor Documentation
Journal::Journal | ( | ) |
Constructs an empty journal.
Definition at line 37 of file journal.cpp.
Journal::~Journal | ( | ) |
Destroys a journal.
Definition at line 41 of file journal.cpp.
Member Function Documentation
|
virtual |
IncidenceBase::typeStr() Returns an exact copy of this journal. The returned object is owned by the caller.
Implements KCal::Incidence.
Definition at line 56 of file journal.cpp.
Assignment operator.
Definition at line 61 of file journal.cpp.
bool Journal::operator== | ( | const Journal & | journal | ) | const |
Compare this with journal
for equality.
- Parameters
-
journal is the journal to compare.
Definition at line 72 of file journal.cpp.
|
virtual |
The documentation for this class was generated from the following files:
This file is part of the KDE documentation.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:30 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:38:30 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.