KCalCore Library
#include <alarm.h>
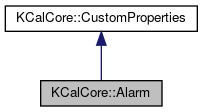
Public Types | |
typedef QVector< Ptr > | List |
typedef QSharedPointer< Alarm > | Ptr |
enum | Type { Invalid, Display, Procedure, Email, Audio } |
Public Member Functions | |
Alarm (Incidence *parent) | |
Alarm (const Alarm &other) | |
virtual | ~Alarm () |
void | addMailAddress (const Person::Ptr &mailAlarmAddress) |
void | addMailAttachment (const QString &mailAttachFile) |
QString | audioFile () const |
Duration | duration () const |
bool | enabled () const |
Duration | endOffset () const |
KDateTime | endTime () const |
bool | hasEndOffset () const |
bool | hasLocationRadius () const |
bool | hasStartOffset () const |
bool | hasTime () const |
int | locationRadius () const |
Person::List | mailAddresses () const |
QStringList | mailAttachments () const |
QString | mailSubject () const |
QString | mailText () const |
KDateTime | nextRepetition (const KDateTime &preTime) const |
KDateTime | nextTime (const KDateTime &preTime, bool ignoreRepetitions=false) const |
bool | operator!= (const Alarm &a) const |
Alarm & | operator= (const Alarm &) |
bool | operator== (const Alarm &a) const |
QString | parentUid () const |
KDateTime | previousRepetition (const KDateTime &afterTime) const |
QString | programArguments () const |
QString | programFile () const |
int | repeatCount () const |
void | setAudioAlarm (const QString &audioFile=QString()) |
void | setAudioFile (const QString &audioFile) |
void | setDisplayAlarm (const QString &text=QString()) |
void | setEmailAlarm (const QString &subject, const QString &text, const Person::List &addressees, const QStringList &attachments=QStringList()) |
void | setEnabled (bool enable) |
void | setEndOffset (const Duration &offset) |
void | setHasLocationRadius (bool hasLocationRadius) |
void | setLocationRadius (int locationRadius) |
void | setMailAddress (const Person::Ptr &mailAlarmAddress) |
void | setMailAddresses (const Person::List &mailAlarmAddresses) |
void | setMailAttachment (const QString &mailAttachFile) |
void | setMailAttachments (const QStringList &mailAttachFiles) |
void | setMailSubject (const QString &mailAlarmSubject) |
void | setMailText (const QString &text) |
void | setParent (Incidence *parent) |
void | setProcedureAlarm (const QString &programFile, const QString &arguments=QString()) |
void | setProgramArguments (const QString &arguments) |
void | setProgramFile (const QString &programFile) |
void | setRepeatCount (int alarmRepeatCount) |
void | setSnoozeTime (const Duration &alarmSnoozeTime) |
void | setStartOffset (const Duration &offset) |
void | setText (const QString &text) |
void | setTime (const KDateTime &alarmTime) |
void | setType (Type type) |
void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
Duration | snoozeTime () const |
Duration | startOffset () const |
QString | text () const |
KDateTime | time () const |
void | toggleAlarm () |
Type | type () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
QString | nonKDECustomPropertyParameters (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value, const QString ¶meters=QString()) |
Protected Member Functions | |
virtual void | customPropertyUpdated () |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | customPropertyUpdate () |
Friends | |
KCALCORE_EXPORT QDataStream & | operator<< (QDataStream &s, const KCalCore::Alarm::Ptr &) |
KCALCORE_EXPORT QDataStream & | operator>> (QDataStream &s, const KCalCore::Alarm::Ptr &) |
Additional Inherited Members | |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Detailed Description
Represents an alarm notification.
Alarms are user notifications that occur at specified times. Notifications can be on-screen pop-up dialogs, email messages, the playing of audio files, or the running of another program.
Alarms always belong to a parent Incidence.
Member Typedef Documentation
typedef QVector<Ptr> KCalCore::Alarm::List |
typedef QSharedPointer<Alarm> KCalCore::Alarm::Ptr |
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
Alarm::Alarm | ( | const Alarm & | other | ) |
Member Function Documentation
void Alarm::addMailAddress | ( | const Person::Ptr & | mailAlarmAddress | ) |
void Alarm::addMailAttachment | ( | const QString & | mailAttachFile | ) |
QString Alarm::audioFile | ( | ) | const |
Returns the audio file name for an Audio alarm type.
Returns an empty string if the alarm is not an Audio type.
- See also
- setAudioAlarm(), setAudioFile()
|
protectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCalCore::CustomProperties.
Duration Alarm::duration | ( | ) | const |
bool Alarm::enabled | ( | ) | const |
Returns the alarm enabled status: true (enabled) or false (disabled).
- See also
- setEnabled(), toggleAlarm()
Duration Alarm::endOffset | ( | ) | const |
Returns offset of alarm in time relative to the end of the event.
If the alarm's time is not defined in terms of an offset relative to the end of the event, returns zero.
- See also
- setEndOffset(), hasEndOffset()
KDateTime Alarm::endTime | ( | ) | const |
bool Alarm::hasEndOffset | ( | ) | const |
Returns whether the alarm is defined in terms of an offset relative to the end of the event.
- See also
- endOffset(), setEndOffset()
bool Alarm::hasLocationRadius | ( | ) | const |
Returns true if alarm has location radius defined.
- See also
- setLocationRadius()
bool Alarm::hasStartOffset | ( | ) | const |
Returns whether the alarm is defined in terms of an offset relative to the start of the parent Incidence.
- See also
- startOffset(), setStartOffset()
bool Alarm::hasTime | ( | ) | const |
int Alarm::locationRadius | ( | ) | const |
Returns the location radius in meters.
- See also
- setLocationRadius()
Person::List Alarm::mailAddresses | ( | ) | const |
QStringList Alarm::mailAttachments | ( | ) | const |
QString Alarm::mailSubject | ( | ) | const |
Returns the subject line string for an Email alarm type.
Returns an empty string if the alarm is not an Email type.
- See also
- setMailSubject()
QString Alarm::mailText | ( | ) | const |
Returns the body text for an Email alarm type.
Returns an empty string if the alarm is not an Email type.
- See also
- setMailText()
KDateTime Alarm::nextRepetition | ( | const KDateTime & | preTime | ) | const |
Returns the date/time of the alarm's initial occurrence or its next repetition after a given time.
- Parameters
-
preTime the date/time after which to find the next repetition.
- Returns
- the date/time of the next repetition, or an invalid date/time if the specified time is at or after the alarm's last repetition.
- See also
- previousRepetition()
KDateTime Alarm::nextTime | ( | const KDateTime & | preTime, |
bool | ignoreRepetitions = false |
||
) | const |
Returns the next alarm trigger date/time after given date/time.
Takes recurrent incidences into account.
- Parameters
-
preTime date/time from where to start ignoreRepetitions don't take repetitions into account
- See also
- nextRepetition()
bool Alarm::operator!= | ( | const Alarm & | a | ) | const |
bool Alarm::operator== | ( | const Alarm & | a | ) | const |
QString Alarm::parentUid | ( | ) | const |
Returns the parent's incidence UID of the alarm.
- See also
- setParent()
KDateTime Alarm::previousRepetition | ( | const KDateTime & | afterTime | ) | const |
Returns the date/time of the alarm's latest repetition or, if none, its initial occurrence before a given time.
- Parameters
-
afterTime is the date/time before which to find the latest repetition.
- Returns
- the date and time of the latest repetition, or an invalid date/time if the specified time is at or before the alarm's initial occurrence.
- See also
- nextRepetition()
QString Alarm::programArguments | ( | ) | const |
QString Alarm::programFile | ( | ) | const |
int Alarm::repeatCount | ( | ) | const |
Returns how many times an alarm may repeats after its initial occurrence.
- See also
- setRepeatCount()
Sets the Audio type for this alarm and the name of the audio file to play when the alarm is triggered.
- Parameters
-
audioFile is the name of the audio file to play when the alarm is triggered.
- See also
- setAudioFile(), audioFile()
void Alarm::setAudioFile | ( | const QString & | audioFile | ) |
Sets the name of the audio file to play when the audio alarm is triggered.
Ignored if the alarm is not an Audio type.
- Parameters
-
audioFile is the name of the audio file to play when the alarm is triggered.
- See also
- setAudioAlarm(), audioFile()
void Alarm::setEmailAlarm | ( | const QString & | subject, |
const QString & | text, | ||
const Person::List & | addressees, | ||
const QStringList & | attachments = QStringList() |
||
) |
Sets the Email type for this alarm and the email subject
, text
, addresses
, and attachments
that make up an email message to be sent when the alarm is triggered.
- Parameters
-
subject is the email subject. text is a string containing the body of the email message. addressees is Person list of email addresses. attachments is a a QStringList of optional file names of email attachments.
void Alarm::setEnabled | ( | bool | enable | ) |
Sets the enabled status of the alarm.
- Parameters
-
enable if true, then enable the alarm; else disable the alarm.
- See also
- enabled(), toggleAlarm()
void Alarm::setEndOffset | ( | const Duration & | offset | ) |
Sets the alarm offset relative to the end of the parent Incidence.
- Parameters
-
offset is a Duration to be used as a time relative to the end of the parent Incidence to be used as the alarm trigger.
- See also
- setStartOffset(), startOffset(), endOffset()
void Alarm::setHasLocationRadius | ( | bool | hasLocationRadius | ) |
Set if the location radius for the alarm has been defined.
- Parameters
-
hasLocationRadius if true, then this alarm has a location radius.
- See also
- setLocationRadius()
void Alarm::setLocationRadius | ( | int | locationRadius | ) |
Set location radius for the alarm.
This means that alarm will be triggered when user approaches the location. Given value will be stored into custom properties as X-LOCATION-RADIUS.
- Parameters
-
locationRadius radius in meters
- See also
- locationRadius()
void Alarm::setMailAddress | ( | const Person::Ptr & | mailAlarmAddress | ) |
Sets the email address of an Email type alarm.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAlarmAddress is a Person to receive a mail message when an Email type alarm is triggered.
void Alarm::setMailAddresses | ( | const Person::List & | mailAlarmAddresses | ) |
Sets a list of email addresses of an Email type alarm.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAlarmAddresses is a Person list to receive a mail message when an Email type alarm is triggered.
void Alarm::setMailAttachment | ( | const QString & | mailAttachFile | ) |
Sets the filename to attach to a mail message for an Email alarm type.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAttachFile is a string containing a filename to be attached to an email message to send when the Email type alarm is triggered.
void Alarm::setMailAttachments | ( | const QStringList & | mailAttachFiles | ) |
void Alarm::setMailSubject | ( | const QString & | mailAlarmSubject | ) |
Sets the subject line of a mail message for an Email alarm type.
Ignored if the alarm is not an Email type.
- Parameters
-
mailAlarmSubject is a string to be used as a subject line of an email message to send when the Email type alarm is triggered.
void Alarm::setMailText | ( | const QString & | text | ) |
Sets the body text for an Email alarm type.
Ignored if the alarm is not an Email type.
- Parameters
-
text is a string containing the body text of a mail message when an Email type alarm is triggered.
void Alarm::setParent | ( | Incidence * | parent | ) |
Sets the parent
Incidence of the alarm.
- Parameters
-
parent is alarm parent Incidence to set.
- See also
- parentUid()
void Alarm::setProcedureAlarm | ( | const QString & | programFile, |
const QString & | arguments = QString() |
||
) |
Sets the Procedure type for this alarm and the program (with arguments) to execute when the alarm is triggered.
- Parameters
-
programFile is the name of the program file to execute when the alarm is triggered. arguments is a string of arguments to supply to programFile
.
void Alarm::setProgramArguments | ( | const QString & | arguments | ) |
Sets the program arguments string when the alarm is triggered.
Ignored if the alarm is not a Procedure type.
- Parameters
-
arguments is a string of arguments to supply to the program.
void Alarm::setProgramFile | ( | const QString & | programFile | ) |
Sets the program file to execute when the alarm is triggered.
Ignored if the alarm is not a Procedure type.
- Parameters
-
programFile is the name of the program file to execute when the alarm is triggered.
void Alarm::setRepeatCount | ( | int | alarmRepeatCount | ) |
Sets how many times an alarm is to repeat itself after its initial occurrence (w/snoozes).
- Parameters
-
alarmRepeatCount is the number of times an alarm may repeat, excluding the initial occurrence.
- See also
- repeatCount()
void Alarm::setSnoozeTime | ( | const Duration & | alarmSnoozeTime | ) |
Sets the snooze time interval for the alarm.
- Parameters
-
alarmSnoozeTime the time between snoozes.
- See also
- snoozeTime()
void Alarm::setStartOffset | ( | const Duration & | offset | ) |
Sets the alarm offset relative to the start of the parent Incidence.
- Parameters
-
offset is a Duration to be used as a time relative to the start of the parent Incidence to be used as the alarm trigger.
- See also
- setEndOffset(), startOffset(), endOffset()
void Alarm::setText | ( | const QString & | text | ) |
Sets the description text
to be displayed when the alarm is triggered.
Ignored if the alarm is not a display alarm.
- Parameters
-
text is the description to display when the alarm is triggered.
- See also
- setDisplayAlarm(), text()
void Alarm::setTime | ( | const KDateTime & | alarmTime | ) |
void Alarm::setType | ( | Alarm::Type | type | ) |
void Alarm::shiftTimes | ( | const KDateTime::Spec & | oldSpec, |
const KDateTime::Spec & | newSpec | ||
) |
Shift the times of the alarm so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual alarm time zone.
For example, shifting an alarm whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the alarm start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
Duration Alarm::snoozeTime | ( | ) | const |
Duration Alarm::startOffset | ( | ) | const |
Returns offset of alarm in time relative to the start of the parent Incidence.
If the alarm's time is not defined in terms of an offset relative to the start of the event, returns zero.
- See also
- setStartOffset(), hasStartOffset()
QString Alarm::text | ( | ) | const |
KDateTime Alarm::time | ( | ) | const |
void Alarm::toggleAlarm | ( | ) |
Toggles the alarm status, i.e, an enable alarm becomes disabled and a disabled alarm becomes enabled.
- See also
- enabled(), setEnabled()
Alarm::Type Alarm::type | ( | ) | const |
|
protectedvirtual |
Reimplemented from KCalCore::CustomProperties.
Friends And Related Function Documentation
|
friend |
Alarm serializer.
- Since
- 4.12
|
friend |
Alarm deserializer.
- Since
- 4.12
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.