KCalCore Library
#include <incidence.h>
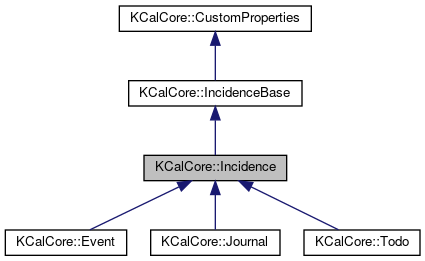
Static Public Member Functions | |
static QStringList | mimeTypes () |
![]() | |
static quint32 | magicSerializationIdentifier () |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
Incidence (const Incidence &other) | |
virtual IncidenceBase & | assign (const IncidenceBase &other) |
void | deserialize (QDataStream &in) |
virtual bool | equals (const IncidenceBase &incidence) const |
void | serialize (QDataStream &out) |
![]() | |
IncidenceBase (const IncidenceBase &ib) | |
virtual void | customPropertyUpdate () |
virtual void | customPropertyUpdated () |
void | setFieldDirty (IncidenceBase::Field field) |
virtual void | virtual_hook (int id, void *data)=0 |
Additional Inherited Members | |
![]() | |
enum | VirtualHook { SerializerHook, DeserializerHook } |
![]() | |
bool | mReadOnly |
Detailed Description
Provides the abstract base class common to non-FreeBusy (Events, To-dos, Journals) calendar components known as incidences.
Several properties are not allowed for VFREEBUSY objects (see rfc:2445), so they are not in IncidenceBase. The hierarchy is:
So IncidenceBase contains all properties that are common to all classes, and Incidence contains all additional properties that are common to Events, Todos and Journals, but are not allowed for FreeBusy entries.
Definition at line 68 of file incidence.h.
Member Typedef Documentation
typedef QVector<Ptr> KCalCore::Incidence::List |
List of incidences.
Definition at line 117 of file incidence.h.
A shared pointer to an Incidence.
Definition at line 112 of file incidence.h.
Member Enumeration Documentation
The different types of RELTYPE values specified by the RFC.
Only RelTypeParent is supported for now.
Enumerator | |
---|---|
RelTypeParent |
The related incidence is a parent. |
RelTypeChild |
The related incidence is a child. |
RelTypeSibling |
The related incidence is a peer. |
Definition at line 103 of file incidence.h.
The different types of incidence access classifications.
Enumerator | |
---|---|
SecrecyPublic |
Not secret (default) |
SecrecyPrivate |
Secret to the owner. |
SecrecyConfidential |
Secret to the owner and some others. |
Definition at line 93 of file incidence.h.
The different types of overall incidence status or confirmation.
The meaning is specific to the incidence type in context.
Definition at line 77 of file incidence.h.
Constructor & Destructor Documentation
Incidence::Incidence | ( | ) |
Constructs an empty incidence.
Private class that helps to provide binary compatibility between releases.
Definition at line 182 of file incidence.cpp.
|
virtual |
Destroys an incidence.
Definition at line 198 of file incidence.cpp.
|
protected |
Copy constructor.
- Parameters
-
other is the incidence to copy.
Definition at line 189 of file incidence.cpp.
Member Function Documentation
void Incidence::addAlarm | ( | const Alarm::Ptr & | alarm | ) |
Adds an alarm to the incidence.
- Parameters
-
alarm is a pointer to a valid Alarm object.
- See also
- removeAlarm().
Definition at line 888 of file incidence.cpp.
void Incidence::addAttachment | ( | const Attachment::Ptr & | attachment | ) |
Adds an attachment to the incidence.
- Parameters
-
attachment is a pointer to a valid Attachment object.
- See also
- deleteAttachment().
Definition at line 694 of file incidence.cpp.
Alarm::List Incidence::alarms | ( | ) | const |
Returns a list of all incidence alarms.
Definition at line 876 of file incidence.cpp.
QString Incidence::altDescription | ( | ) | const |
Returns the incidence alternative (=text/html) description.
- See also
- setAltDescription().
Definition at line 1100 of file incidence.cpp.
|
protectedvirtual |
Provides polymorfic assignment.
- Parameters
-
other is the IncidenceBase to assign.
Reimplemented from KCalCore::IncidenceBase.
Reimplemented in KCalCore::Todo, KCalCore::Event, and KCalCore::Journal.
Definition at line 218 of file incidence.cpp.
Attachment::List Incidence::attachments | ( | ) | const |
Returns a list of all incidence attachments.
- See also
- attachments( const QString &).
Definition at line 731 of file incidence.cpp.
Attachment::List Incidence::attachments | ( | const QString & | mime | ) | const |
Returns a list of all incidence attachments with the specified MIME type.
- Parameters
-
mime is a QString containing the MIME type.
- See also
- attachments().
Definition at line 736 of file incidence.cpp.
QStringList Incidence::categories | ( | ) | const |
Returns the incidence categories as a list of strings.
- See also
- setCategories( const QStringList &), setCategories( Const QString &).
Definition at line 521 of file incidence.cpp.
QString Incidence::categoriesStr | ( | ) | const |
Returns the incidence categories as a comma separated string.
- See also
- categories().
Definition at line 526 of file incidence.cpp.
void Incidence::clearAlarms | ( | ) |
void Incidence::clearAttachments | ( | ) |
Removes all attachments and frees the memory used by them.
- See also
- deleteAttachment( Attachment::Ptr), deleteAttachments( const QString &).
Definition at line 747 of file incidence.cpp.
void Incidence::clearRecurrence | ( | ) |
Removes all recurrence and exception rules and dates.
Definition at line 564 of file incidence.cpp.
void Incidence::clearTempFiles | ( | ) |
Deletes all temporary files used by attachments and frees any memory in use by them.
- See also
- writeAttachmentToTempFile().
Definition at line 775 of file incidence.cpp.
|
pure virtual |
Returns an exact copy of this incidence.
The returned object is owned by the caller.
Dirty fields are cleared.
Implemented in KCalCore::Event, KCalCore::Todo, and KCalCore::Journal.
KDateTime Incidence::created | ( | ) | const |
Returns the incidence creation date/time.
- See also
- setCreated().
Definition at line 370 of file incidence.cpp.
QString Incidence::customStatus | ( | ) | const |
Returns the non-standard status value.
- See also
- setCustomStatus().
Definition at line 850 of file incidence.cpp.
void Incidence::deleteAttachment | ( | const Attachment::Ptr & | attachment | ) |
Removes the specified attachment from the incidence.
Additionally, the memory used by the attachment is freed.
- Parameters
-
attachment is a pointer to a valid Attachment object.
- See also
- addAttachment(), deleteAttachments().
Definition at line 708 of file incidence.cpp.
void Incidence::deleteAttachments | ( | const QString & | mime | ) |
Removes all attachments of the specified MIME type from the incidence.
The memory used by all the removed attachments is freed.
- Parameters
-
mime is a QString containing the MIME type.
- See also
- deleteAttachment().
Definition at line 717 of file incidence.cpp.
QString Incidence::description | ( | ) | const |
Returns the incidence description.
- See also
- setDescription().
- richDescription().
Definition at line 430 of file incidence.cpp.
bool Incidence::descriptionIsRich | ( | ) | const |
Returns true if incidence description contains RichText; false otherwise.
- See also
- setDescription(), description().
Definition at line 444 of file incidence.cpp.
|
virtual |
Returns the end date/time of the incidence occurrence if it starts at specified date/time.
- Parameters
-
startDt is the specified starting date/time.
- Returns
- the corresponding end date/time for the occurrence; or the start date/time if the end date/time is invalid; or the end date/time if the start date/time is invalid.
Definition at line 680 of file incidence.cpp.
|
protectedvirtual |
Compares this with Incidence incidence
for equality.
- Parameters
-
incidence is the Incidence to compare against.
- Returns
- true if the incidences are equal; false otherwise.
Reimplemented from KCalCore::IncidenceBase.
Reimplemented in KCalCore::Todo, KCalCore::Event, and KCalCore::Journal.
Definition at line 231 of file incidence.cpp.
float Incidence::geoLatitude | ( | ) | const |
Returns the incidence geoLatidude.
- Returns
- incidences geolatitude value
- See also
- setGeoLatitude().
Definition at line 1002 of file incidence.cpp.
float Incidence::geoLongitude | ( | ) | const |
Returns the incidence geoLongitude.
- Returns
- incidences geolongitude value
- See also
- setGeoLongitude().
Definition at line 1019 of file incidence.cpp.
bool Incidence::hasAltDescription | ( | ) | const |
Returns true if the alternative (=text/html) description is available.
- See also
- setAltDescription(), altDescription()
Definition at line 1081 of file incidence.cpp.
bool Incidence::hasEnabledAlarms | ( | ) | const |
Returns true if any of the incidence alarms are enabled; false otherwise.
Definition at line 915 of file incidence.cpp.
bool Incidence::hasGeo | ( | ) | const |
Returns true if the incidence has geo data, otherwise return false.
Definition at line 980 of file incidence.cpp.
bool Incidence::hasRecurrenceId | ( | ) | const |
Returns true if the incidence has recurrenceId, otherwise return false.
- See also
- setRecurrenceId(KDateTime)
Definition at line 1034 of file incidence.cpp.
|
pure virtual |
Returns the name of the icon that best represents this incidence.
- Parameters
-
recurrenceId Some recurring incidences might use a different icon, for example, completed to-do occurrences. Use this parameter to identify the specific occurrence in a recurring serie.
Implemented in KCalCore::Todo, KCalCore::Event, and KCalCore::Journal.
QString Incidence::instanceIdentifier | ( | ) | const |
Returns a unique identifier for a specific instance of an incidence.
Due to the recurrence-id, the uid is not unique for a KCalCore::Incidence.
- Since
- 4.11
Definition at line 300 of file incidence.cpp.
bool Incidence::localOnly | ( | ) | const |
Get the localOnly status.
- Returns
- true if Local only, false otherwise.
- See also
- setLocalOnly()
Definition at line 341 of file incidence.cpp.
QString Incidence::location | ( | ) | const |
Returns the incidence location.
Do not use with journals.
- See also
- setLocation().
- richLocation().
Definition at line 943 of file incidence.cpp.
bool Incidence::locationIsRich | ( | ) | const |
Returns true if incidence location contains RichText; false otherwise.
- See also
- setLocation(), location().
Definition at line 957 of file incidence.cpp.
|
static |
Returns the list of possible mime types in an Incidence object: "text/calendar" "application/x-vnd.akonadi.calendar.event" "application/x-vnd.akonadi.calendar.todo" "application/x-vnd.akonadi.calendar.journal".
static
- Since
- 4.12
Definition at line 1115 of file incidence.cpp.
Alarm::Ptr Incidence::newAlarm | ( | ) |
Create a new incidence alarm.
Definition at line 881 of file incidence.cpp.
int Incidence::priority | ( | ) | const |
Returns the incidence priority.
- See also
- setPriority().
Definition at line 814 of file incidence.cpp.
void Incidence::recreate | ( | ) |
Recreate incidence.
The incidence is made a new unique incidence, but already stored information is preserved. Sets unique id, creation date, last modification date and revision number.
Definition at line 308 of file incidence.cpp.
Recurrence * Incidence::recurrence | ( | ) | const |
Returns the recurrence rule associated with this incidence.
If there is none, returns an appropriate (non-0) object.
Definition at line 551 of file incidence.cpp.
|
virtual |
Returns the incidence recurrenceId.
- Returns
- incidences recurrenceId value
- See also
- setRecurrenceId().
Reimplemented from KCalCore::IncidenceBase.
Definition at line 1039 of file incidence.cpp.
ushort Incidence::recurrenceType | ( | ) | const |
Returns the event's recurrence status.
See the enumeration at the top of this file for possible values.
Definition at line 570 of file incidence.cpp.
|
virtual |
Observer interface for the recurrence class.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
- Parameters
-
recurrence is a pointer to a valid Recurrence object.
If the recurrence is changed, this method will be called for the incidence the recurrence object belongs to.
Definition at line 1067 of file incidence.cpp.
bool Incidence::recurs | ( | ) | const |
Returns whether the event recurs at all.
Definition at line 579 of file incidence.cpp.
bool Incidence::recursAt | ( | const KDateTime & | dt | ) | const |
Returns true if the date/time specified is one at which the event will recur.
Times are rounded down to the nearest minute to determine the result.
- Parameters
-
dt is the date/time to check.
Definition at line 594 of file incidence.cpp.
|
virtual |
Returns true if the date specified is one on which the event will recur.
- Parameters
-
date date to check. timeSpec time specification for date
.
Reimplemented in KCalCore::Todo.
Definition at line 588 of file incidence.cpp.
QString Incidence::relatedTo | ( | RelType | relType = RelTypeParent | ) | const |
Returns a UID string for the incidence that is related to this one.
This function should only be used when constructing a calendar before the related incidence exists.
- Warning
- KCalCore only supports one related-to field per reltype for now.
- Parameters
-
relType specifies the relation type.
- See also
- setRelatedTo().
Definition at line 544 of file incidence.cpp.
void Incidence::removeAlarm | ( | const Alarm::Ptr & | alarm | ) |
Removes the specified alarm from the incidence.
- Parameters
-
alarm is a pointer to a valid Alarm object.
- See also
- addAlarm().
Definition at line 896 of file incidence.cpp.
QStringList Incidence::resources | ( | ) | const |
Returns the incidence resources as a list of strings.
- See also
- setResources().
Definition at line 797 of file incidence.cpp.
int Incidence::revision | ( | ) | const |
Returns the number of revisions this incidence has seen.
- See also
- setRevision().
Definition at line 388 of file incidence.cpp.
QString Incidence::richDescription | ( | ) | const |
Returns the incidence description in rich text format.
- See also
- setDescription().
- description().
Definition at line 435 of file incidence.cpp.
QString Incidence::richLocation | ( | ) | const |
Returns the incidence location in rich text format.
- See also
- setLocation().
- location().
Definition at line 948 of file incidence.cpp.
QString Incidence::richSummary | ( | ) | const |
Returns the incidence summary in rich text format.
- See also
- setSummary().
- summary().
Definition at line 471 of file incidence.cpp.
QString Incidence::schedulingID | ( | ) | const |
Returns the incidence scheduling ID.
Do not use with journals. If a scheduling ID is not set, then return the incidence UID.
- See also
- setSchedulingID().
Definition at line 971 of file incidence.cpp.
Incidence::Secrecy Incidence::secrecy | ( | ) | const |
Returns the incidence Secrecy.
- See also
- setSecrecy(), secrecyStr().
Definition at line 871 of file incidence.cpp.
void Incidence::setAllDay | ( | bool | allDay | ) |
Definition at line 346 of file incidence.cpp.
void Incidence::setAltDescription | ( | const QString & | altdescription | ) |
Sets the incidence's alternative (=text/html) description.
If the text is empty, the property is removed.
- Parameters
-
altdescription is the incidence altdescription string.
- See also
- altAltdescription().
Definition at line 1089 of file incidence.cpp.
void Incidence::setCategories | ( | const QStringList & | categories | ) |
Sets the incidence category list.
- Parameters
-
categories is a list of category strings.
- See also
- setCategories( const QString &), categories().
Definition at line 485 of file incidence.cpp.
void Incidence::setCategories | ( | const QString & | catStr | ) |
Sets the incidence category list based on a comma delimited string.
- Parameters
-
catStr is a QString containing a list of categories which are delimited by a comma character.
Definition at line 496 of file incidence.cpp.
void Incidence::setCreated | ( | const KDateTime & | dt | ) |
Sets the incidence creation date/time.
It is stored as a UTC date/time.
- Parameters
-
dt is the creation date/time.
- See also
- created().
Definition at line 357 of file incidence.cpp.
void Incidence::setCustomStatus | ( | const QString & | status | ) |
Sets the incidence Status to a non-standard status value.
- Parameters
-
status is a non-standard status string. If empty, the incidence Status will be set to StatusNone.
- See also
- setStatus(), status() customStatus().
Definition at line 832 of file incidence.cpp.
void Incidence::setDescription | ( | const QString & | description, |
bool | isRich | ||
) |
Sets the incidence description.
- Parameters
-
description is the incidence description string. isRich if true indicates the description string contains richtext.
- See also
- description().
Definition at line 413 of file incidence.cpp.
void Incidence::setDescription | ( | const QString & | description | ) |
Sets the incidence description and tries to guess if the description is rich text.
- Parameters
-
description is the incidence description string.
- See also
- description().
Definition at line 425 of file incidence.cpp.
|
virtual |
Sets the incidence starting date/time.
- Parameters
-
dt is the starting date/time.
- See also
- IncidenceBase::dtStart().
Reimplemented from KCalCore::IncidenceBase.
Reimplemented in KCalCore::Todo, and KCalCore::Event.
Definition at line 393 of file incidence.cpp.
void Incidence::setGeoLatitude | ( | float | geolatitude | ) |
Set the incidences geoLatitude.
- Parameters
-
geolatitude is the incidence geolatitude to set
- See also
- geoLatitude().
Definition at line 1007 of file incidence.cpp.
void Incidence::setGeoLongitude | ( | float | geolongitude | ) |
Set the incidencesgeoLongitude.
- Parameters
-
geolongitude is the incidence geolongitude to set
- See also
- geoLongitude().
Definition at line 1024 of file incidence.cpp.
void Incidence::setHasGeo | ( | bool | hasGeo | ) |
Sets if the incidence has geo data.
- Parameters
-
hasGeo true if incidence has geo data, otherwise false
- See also
- hasGeo(), geoLatitude(), geoLongitude().
Definition at line 985 of file incidence.cpp.
|
virtual |
Reimplemented from KCalCore::IncidenceBase.
Definition at line 318 of file incidence.cpp.
void Incidence::setLocalOnly | ( | bool | localonly | ) |
Set localOnly state of incidence.
A local only incidence can be updated but it will not increase the revision number neither the modified date.
- Parameters
-
localonly If true, the incidence is set to localonly, if false the incidence is set to normal stat.
Definition at line 333 of file incidence.cpp.
void Incidence::setLocation | ( | const QString & | location, |
bool | isRich | ||
) |
Sets the incidence location.
Do not use with journals.
- Parameters
-
location is the incidence location string. isRich if true indicates the location string contains richtext.
- See also
- location().
Definition at line 925 of file incidence.cpp.
void Incidence::setLocation | ( | const QString & | location | ) |
Sets the incidence location and tries to guess if the location is richtext.
Do not use with journals.
- Parameters
-
location is the incidence location string.
- See also
- location().
Definition at line 938 of file incidence.cpp.
void Incidence::setPriority | ( | int | priority | ) |
Sets the incidences priority.
The priority must be an integer value between 0 and 9, where 0 is undefined, 1 is the highest, and 9 is the lowest priority (decreasing order).
- Parameters
-
priority is the incidence priority to set.
- See also
- priority().
Definition at line 802 of file incidence.cpp.
|
virtual |
Set readonly state of incidence.
- Parameters
-
readonly If true, the incidence is set to readonly, if false the incidence is set to readwrite.
Reimplemented from KCalCore::IncidenceBase.
Definition at line 325 of file incidence.cpp.
void Incidence::setRecurrenceId | ( | const KDateTime & | recurrenceId | ) |
Set the incidences recurrenceId.
This field indicates that this is an exception to a recurring incidence. The uid of this incidence MUST be the same as the one of the recurring main incidence.
- Parameters
-
recurrenceId is the incidence recurrenceId to set
- See also
- recurrenceId().
Definition at line 1054 of file incidence.cpp.
void Incidence::setRelatedTo | ( | const QString & | uid, |
RelType | relType = RelTypeParent |
||
) |
Relates another incidence to this one, by UID.
This function should only be used when constructing a calendar before the related incidence exists.
- Parameters
-
uid is a QString containing a UID for another incidence. relType specifies the relation type.
- Warning
- KCalCore only supports one related-to field per reltype for now.
- See also
- relatedTo().
Definition at line 531 of file incidence.cpp.
void Incidence::setResources | ( | const QStringList & | resources | ) |
Sets a list of incidence resources.
(Note: resources in this context means items used by the incidence such as money, fuel, hours, etc).
- Parameters
-
resources is a list of resource strings.
- See also
- resources().
Definition at line 785 of file incidence.cpp.
void Incidence::setRevision | ( | int | rev | ) |
Sets the number of revisions this incidence has seen.
- Parameters
-
rev is the incidence revision number.
- See also
- revision().
Definition at line 375 of file incidence.cpp.
Set the incidence scheduling ID.
Do not use with journals. This is used for accepted invitations as the place to store the UID of the invitation. It is later used again if updates to the invitation comes in. If we did not set a new UID on incidences from invitations, we can end up with more than one resource having events with the same UID, if you have access to other peoples resources.
While constructing an incidence, when setting the scheduling ID, you will always want to set the incidence UID too. Instead of calling setUID() separately, you can pass the UID through uid
so both members are changed in one atomic operation ( don't forget that setUID() emits incidenceUpdated() and whoever catches that signal will have an half-initialized incidence, therefore, always set the schedulingID and UID at the same time, and never with two separate calls).
- Parameters
-
sid is a QString containing the scheduling ID. uid is a QString containing the incidence UID to set, if not specified, the current UID isn't changed, and this parameter is ignored.
- See also
- schedulingID().
Definition at line 962 of file incidence.cpp.
void Incidence::setSecrecy | ( | Incidence::Secrecy | secrecy | ) |
Sets the incidence Secrecy.
- Parameters
-
secrecy is the incidence Secrecy to set.
- See also
- secrecy(), secrecyStr().
Definition at line 859 of file incidence.cpp.
void Incidence::setStatus | ( | Incidence::Status | status | ) |
Sets the incidence status to a standard Status value.
Note that StatusX cannot be specified.
- Parameters
-
status is the incidence Status to set.
- See also
- status(), setCustomStatus().
Definition at line 819 of file incidence.cpp.
void Incidence::setSummary | ( | const QString & | summary, |
bool | isRich | ||
) |
Sets the incidence summary.
- Parameters
-
summary is the incidence summary string. isRich if true indicates the summary string contains richtext.
- See also
- summary().
Definition at line 449 of file incidence.cpp.
void Incidence::setSummary | ( | const QString & | summary | ) |
Sets the incidence summary and tries to guess if the summary is richtext.
- Parameters
-
summary is the incidence summary string.
- See also
- summary().
Definition at line 461 of file incidence.cpp.
void Incidence::setThisAndFuture | ( | bool | thisAndFuture | ) |
Set to true if the exception also applies to all future occurrences.
This option is only relevant if the incidence has a recurrenceId set.
- Parameters
-
thisAndFuture value
- See also
- thisAndFuture(), setRecurrenceId()
- Since
- 4.11
Definition at line 1044 of file incidence.cpp.
|
virtual |
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
Reimplemented from KCalCore::IncidenceBase.
Reimplemented in KCalCore::Todo, and KCalCore::Event.
Definition at line 401 of file incidence.cpp.
|
virtual |
Calculates the start date/time for all recurrences that happen at some time on the given date (might start before that date, but end on or after the given date).
- Parameters
-
date the date when the incidence should occur timeSpec time specification for date
.
- Returns
- the start date/time of all occurrences that overlap with the given date; an empty list if the incidence does not overlap with the date at all.
Definition at line 599 of file incidence.cpp.
|
virtual |
Calculates the start date/time for all recurrences that happen at the given time.
- Parameters
-
datetime the date/time when the incidence should occur.
- Returns
- the start date/time of all occurrences that overlap with the given date/time; an empty list if the incidence does not happen at the given time at all.
Definition at line 640 of file incidence.cpp.
Incidence::Status Incidence::status | ( | ) | const |
Returns the incidence Status.
- See also
- setStatus(), setCustomStatus(), statusStr().
Definition at line 845 of file incidence.cpp.
QString Incidence::summary | ( | ) | const |
Returns the incidence summary.
- See also
- setSummary().
- richSummary().
Definition at line 466 of file incidence.cpp.
bool Incidence::summaryIsRich | ( | ) | const |
Returns true if incidence summary contains RichText; false otherwise.
- See also
- setSummary(), summary().
Definition at line 480 of file incidence.cpp.
bool Incidence::supportsGroupwareCommunication | ( | ) | const |
Returns true if the incidence type supports groupware communication.
- Since
- 4.10
Definition at line 1109 of file incidence.cpp.
bool Incidence::thisAndFuture | ( | ) | const |
Returns true if the exception also applies to all future occurrences.
- Returns
- incidences thisAndFuture value
- See also
- setThisAndFuture()
- Since
- 4.11
Definition at line 1049 of file incidence.cpp.
QString Incidence::writeAttachmentToTempFile | ( | const Attachment::Ptr & | attachment | ) | const |
Writes the data in the attachment attachment
to a temporary file and returns the local name of the temporary file.
- Parameters
-
attachment is a pointer to a valid Attachment instance.
- Returns
- a string containing the name of the temporary file containing the attachment.
- See also
- clearTempFiles().
Definition at line 753 of file incidence.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:36:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.