kcachegrind
#include <treemap.h>
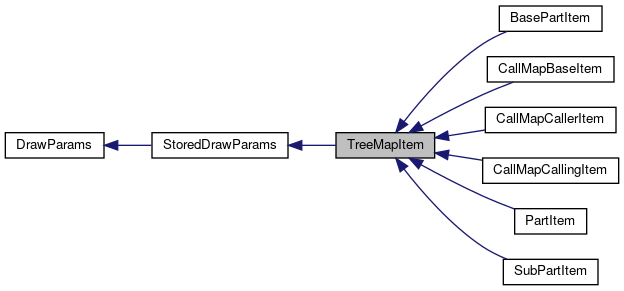
Public Types | |
enum | SplitMode { Bisection, Columns, Rows, AlwaysBest, Best, HAlternate, VAlternate, Horizontal, Vertical } |
![]() | |
enum | Position { TopLeft, TopCenter, TopRight, BottomLeft, BottomCenter, BottomRight, Default, Unknown } |
Public Member Functions | |
TreeMapItem (TreeMapItem *parent=0, double value=1.0) | |
TreeMapItem (TreeMapItem *parent, double value, const QString &text1, const QString &text2=QString(), const QString &text3=QString(), const QString &text4=QString()) | |
virtual | ~TreeMapItem () |
void | addFreeRect (const QRect &r) |
void | addItem (TreeMapItem *) |
virtual int | borderWidth () const |
virtual TreeMapItemList * | children () |
void | clear () |
void | clearFreeRects () |
void | clearItemRect () |
TreeMapItem * | commonParent (TreeMapItem *item) |
int | depth () const |
virtual const QFont & | font () const |
const QList< QRect > & | freeRects () const |
int | height () const |
int | index () const |
bool | initialized () |
bool | isChildOf (TreeMapItem *) |
virtual bool | isMarked (int) const |
const QRect & | itemRect () const |
TreeMapItem * | parent () const |
QStringList | path (int) const |
virtual Position | position (int) const |
void | redraw () |
void | refresh () |
void | resort (bool recursive=true) |
virtual int | rtti () const |
void | setIndex (int i) |
void | setItemRect (const QRect &r) |
void | setParent (TreeMapItem *p) |
void | setSorting (int textNo, bool ascending=true) |
void | setSum (double s) |
void | setValue (double s) |
void | setWidget (TreeMapWidget *w) |
virtual int | sorting (bool *ascending) const |
virtual SplitMode | splitMode () const |
virtual double | sum () const |
virtual double | value () const |
TreeMapWidget * | widget () const |
int | width () const |
![]() | |
StoredDrawParams () | |
StoredDrawParams (const QColor &c, bool selected=false, bool current=false) | |
QColor | backColor () const |
bool | current () const |
bool | drawFrame () const |
void | drawFrame (bool b) |
int | fieldCount () const |
int | maxLines (int) const |
QPixmap | pixmap (int) const |
bool | rotated () const |
bool | selected () const |
void | setBackColor (const QColor &c) |
void | setCurrent (bool b) |
void | setField (int f, const QString &t, const QPixmap &pm=QPixmap(), Position p=Default, int maxLines=0) |
void | setMaxLines (int f, int) |
void | setPixmap (int f, const QPixmap &) |
void | setPosition (int f, Position) |
void | setRotated (bool b) |
void | setSelected (bool b) |
void | setShaded (bool b) |
void | setText (int f, const QString &) |
bool | shaded () const |
QString | text (int) const |
![]() | |
virtual | ~DrawParams () |
Protected Attributes | |
TreeMapItemList * | _children |
double | _sum |
double | _value |
![]() | |
QColor | _backColor |
bool | _current:1 |
bool | _drawFrame:1 |
bool | _rotated:1 |
bool | _selected:1 |
bool | _shaded:1 |
Detailed Description
Base class of items in TreeMap.
This class supports an arbitrary number of text() strings positioned counterclock-wise starting at TopLeft. Each item has its own static value(), sum() and sorting(). The splitMode() and borderWidth() is taken from a TreeMapWidget.
If you want more flexibility, reimplement TreeMapItem and override the corresponding methods. For dynamic creation of child items on demand, reimplement children().
Member Enumeration Documentation
Split direction for nested areas: AlwaysBest: Choose split direction for every subitem according to longest side of rectangle left for drawing Best: Choose split direction for all subitems of an area depending on longest side HAlternate: Horizontal at top; alternate direction on depth step VAlternate: Vertical at top; alternate direction on depth step Horizontal: Always horizontal split direction Vertical: Always vertical split direction.
Enumerator | |
---|---|
Bisection | |
Columns | |
Rows | |
AlwaysBest | |
Best | |
HAlternate | |
VAlternate | |
Horizontal | |
Vertical |
Constructor & Destructor Documentation
|
explicit |
Definition at line 836 of file treemap.cpp.
TreeMapItem::TreeMapItem | ( | TreeMapItem * | parent, |
double | value, | ||
const QString & | text1, | ||
const QString & | text2 = QString() , |
||
const QString & | text3 = QString() , |
||
const QString & | text4 = QString() |
||
) |
Definition at line 860 of file treemap.cpp.
|
virtual |
Definition at line 883 of file treemap.cpp.
Member Function Documentation
void TreeMapItem::addFreeRect | ( | const QRect & | r | ) |
Definition at line 1108 of file treemap.cpp.
void TreeMapItem::addItem | ( | TreeMapItem * | i | ) |
Adds an item to a parent.
When no sorting is used, the item is appended (drawn at bottom). This is only needed if the parent was not already specified in the construction of the item.
Definition at line 982 of file treemap.cpp.
|
virtual |
Reimplemented in CallMapCallerItem, CallMapCallingItem, PartItem, and BasePartItem.
Definition at line 1031 of file treemap.cpp.
|
virtual |
Reimplemented in CallMapCallerItem, SubPartItem, CallMapCallingItem, PartItem, CallMapBaseItem, and BasePartItem.
Definition at line 1089 of file treemap.cpp.
void TreeMapItem::clear | ( | ) |
Definition at line 927 of file treemap.cpp.
void TreeMapItem::clearFreeRects | ( | ) |
Temporary rectangle list of free space of this item.
Used internally to enable tooltip.
Definition at line 1103 of file treemap.cpp.
void TreeMapItem::clearItemRect | ( | ) |
Definition at line 1097 of file treemap.cpp.
TreeMapItem * TreeMapItem::commonParent | ( | TreeMapItem * | item | ) |
Definition at line 913 of file treemap.cpp.
int TreeMapItem::depth | ( | ) | const |
|
virtual |
Reimplemented from StoredDrawParams.
Definition at line 1019 of file treemap.cpp.
|
inline |
bool TreeMapItem::initialized | ( | ) |
Definition at line 973 of file treemap.cpp.
bool TreeMapItem::isChildOf | ( | TreeMapItem * | item | ) |
Definition at line 901 of file treemap.cpp.
|
virtual |
Reimplemented in CallMapCallerItem, CallMapCallingItem, and CallMapBaseItem.
Definition at line 1025 of file treemap.cpp.
|
inline |
QStringList TreeMapItem::path | ( | int | textNo | ) | const |
Returns a list of text strings of specified text number, from root up to this item.
Definition at line 949 of file treemap.cpp.
|
virtual |
Reimplemented from StoredDrawParams.
Definition at line 1009 of file treemap.cpp.
void TreeMapItem::redraw | ( | ) |
Definition at line 921 of file treemap.cpp.
void TreeMapItem::refresh | ( | ) |
Definition at line 942 of file treemap.cpp.
void TreeMapItem::resort | ( | bool | recursive = true | ) |
Resort according to the already set sorting.
This has to be done if the sorting base changes (e.g. text or values change). If this is only true for the children of this item, you can set the recursive parameter to false.
Definition at line 1063 of file treemap.cpp.
|
virtual |
Reimplemented in CallMapCallerItem, SubPartItem, CallMapCallingItem, PartItem, CallMapBaseItem, and BasePartItem.
Definition at line 1084 of file treemap.cpp.
|
inline |
void TreeMapItem::setParent | ( | TreeMapItem * | p | ) |
Definition at line 895 of file treemap.cpp.
void TreeMapItem::setSorting | ( | int | textNo, |
bool | ascending = true |
||
) |
Set the sorting for child drawing.
Default is no sorting: <textNo> = -1 For value() sorting, use <textNo> = -2
For fast sorting, set this to -1 before child insertions and call again after inserting all children.
Definition at line 1046 of file treemap.cpp.
|
inline |
|
virtual |
Returns the text number after that sorting is done or -1 for no sorting, -2 for value() sorting (default).
If ascending != 0, a bool value is written at that location to indicate if sorting should be ascending.
Definition at line 1039 of file treemap.cpp.
|
virtual |
Reimplemented in SubPartItem.
Definition at line 1076 of file treemap.cpp.
|
virtual |
Reimplemented in SubPartItem, CallMapCallingItem, PartItem, and CallMapBaseItem.
Definition at line 1004 of file treemap.cpp.
|
virtual |
Reimplemented in CallMapCallerItem, SubPartItem, CallMapCallingItem, PartItem, CallMapBaseItem, and BasePartItem.
Definition at line 999 of file treemap.cpp.
|
inline |
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:39:51 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.