kcachegrind
#include <treemap.h>
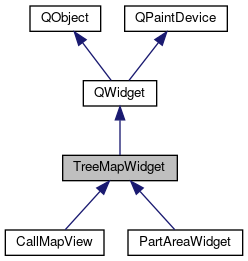
Public Types | |
enum | SelectionMode { Single, Multi, Extended, NoSelection } |
Signals | |
void | clicked (TreeMapItem *) |
void | contextMenuRequested (TreeMapItem *, const QPoint &) |
void | currentChanged (TreeMapItem *, bool keyboard) |
void | doubleClicked (TreeMapItem *) |
void | returnPressed (TreeMapItem *) |
void | rightButtonPressed (TreeMapItem *, const QPoint &) |
void | selectionChanged () |
void | selectionChanged (TreeMapItem *) |
Public Member Functions | |
TreeMapWidget (TreeMapItem *base, QWidget *parent=0) | |
~TreeMapWidget () | |
void | addSplitDirectionItems (QMenu *) |
bool | allowRotation () const |
TreeMapItem * | base () const |
int | borderWidth () const |
bool | clearSelection (TreeMapItem *parent=0) |
TreeMapItem * | current () const |
const QFont & | currentFont () const |
bool | defaultFieldForced (int) const |
DrawParams::Position | defaultFieldPosition (int) const |
QString | defaultFieldStop (int) const |
QString | defaultFieldType (int) const |
bool | defaultFieldVisible (int) const |
void | deletingItem (TreeMapItem *) |
void | drawFrame (int d, bool b) |
bool | drawFrame (int d) const |
void | drawTreeMap () |
bool | fieldForced (int) const |
DrawParams::Position | fieldPosition (int) const |
QString | fieldPositionString (int) const |
QString | fieldStop (int) const |
QString | fieldType (int) const |
bool | fieldVisible (int) const |
bool | isSelected (TreeMapItem *i) const |
bool | isShadingEnabled () const |
bool | isTransparent (int d) const |
TreeMapItem * | item (int x, int y) const |
int | maxDrawingDepth () const |
int | maxSelectDepth () const |
int | minimalArea () const |
TreeMapItem * | possibleSelection (TreeMapItem *) const |
void | redraw (TreeMapItem *) |
void | redraw () |
void | resort () |
TreeMapItemList | selection () const |
SelectionMode | selectionMode () const |
void | setAllowRotation (bool) |
void | setBorderWidth (int w) |
void | setCurrent (TreeMapItem *, bool kbd=false) |
void | setFieldForced (int, bool) |
void | setFieldPosition (int, DrawParams::Position) |
void | setFieldPosition (int, const QString &) |
void | setFieldStop (int, const QString &) |
void | setFieldType (int, const QString &) |
void | setFieldVisible (int, bool) |
void | setMarked (int markNo=1, bool redraw=true) |
void | setMaxDrawingDepth (int d) |
void | setMaxSelectDepth (int d) |
void | setMinimalArea (int area) |
void | setRangeSelection (TreeMapItem *i1, TreeMapItem *i2, bool selected) |
void | setSelected (TreeMapItem *, bool selected=true) |
void | setSelectionMode (SelectionMode m) |
void | setShadingEnabled (bool s) |
void | setSkipIncorrectBorder (bool enable=true) |
void | setSplitMode (TreeMapItem::SplitMode m) |
bool | setSplitMode (const QString &) |
void | setTransparent (int d, bool b) |
void | setVisibleWidth (int width, bool reuseSpace=false) |
bool | skipIncorrectBorder () const |
TreeMapItem::SplitMode | splitMode () const |
QString | splitModeString () const |
virtual QString | tipString (TreeMapItem *i) const |
TreeMapItem * | visibleItem (TreeMapItem *) const |
TreeMapWidget * | widget () |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
virtual QSize | minimumSizeHint () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
virtual QSize | sizeHint () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Slots | |
void | splitActivated (QAction *) |
Protected Member Functions | |
void | contextMenuEvent (QContextMenuEvent *) |
bool | event (QEvent *event) |
void | fontChange (const QFont &) |
void | keyPressEvent (QKeyEvent *) |
void | mouseDoubleClickEvent (QMouseEvent *) |
void | mouseMoveEvent (QMouseEvent *) |
void | mousePressEvent (QMouseEvent *) |
void | mouseReleaseEvent (QMouseEvent *) |
void | paintEvent (QPaintEvent *) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
acceptDrops | |
accessibleDescription | |
accessibleName | |
autoFillBackground | |
baseSize | |
childrenRect | |
childrenRegion | |
contextMenuPolicy | |
cursor | |
enabled | |
focus | |
focusPolicy | |
font | |
frameGeometry | |
frameSize | |
fullScreen | |
geometry | |
height | |
inputMethodHints | |
isActiveWindow | |
layoutDirection | |
locale | |
maximized | |
maximumHeight | |
maximumSize | |
maximumWidth | |
minimized | |
minimumHeight | |
minimumSize | |
minimumSizeHint | |
minimumWidth | |
modal | |
mouseTracking | |
normalGeometry | |
palette | |
pos | |
rect | |
size | |
sizeHint | |
sizeIncrement | |
sizePolicy | |
statusTip | |
styleSheet | |
toolTip | |
updatesEnabled | |
visible | |
whatsThis | |
width | |
windowFilePath | |
windowFlags | |
windowIcon | |
windowIconText | |
windowModality | |
windowModified | |
windowOpacity | |
windowTitle | |
x | |
y | |
![]() | |
objectName | |
Detailed Description
Class for visualization of a metric of hierarchically nested items as 2D areas.
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
Definition at line 1153 of file treemap.cpp.
TreeMapWidget::~TreeMapWidget | ( | ) |
Definition at line 1199 of file treemap.cpp.
Member Function Documentation
void TreeMapWidget::addSplitDirectionItems | ( | QMenu * | m | ) |
Populate given menu with option items.
The added items are automatically connected to handlers.
Definition at line 2848 of file treemap.cpp.
|
inline |
Returns the TreeMapItem filling out the widget space.
bool TreeMapWidget::clearSelection | ( | TreeMapItem * | parent = 0 | ) |
Clear selection of all selected items which are children of parent.
When parent == 0, clears whole selection Returns true if selection changed.
Definition at line 1705 of file treemap.cpp.
|
signal |
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1820 of file treemap.cpp.
|
signal |
|
inline |
|
signal |
This signal is emitted if the current item changes.
If the change is done because of keyboard navigation, the is set to true
const QFont & TreeMapWidget::currentFont | ( | ) | const |
Returns a reference to the current widget font.
Definition at line 1204 of file treemap.cpp.
bool TreeMapWidget::defaultFieldForced | ( | int | ) | const |
Definition at line 1337 of file treemap.cpp.
DrawParams::Position TreeMapWidget::defaultFieldPosition | ( | int | f | ) | const |
Definition at line 1342 of file treemap.cpp.
QString TreeMapWidget::defaultFieldStop | ( | int | ) | const |
Definition at line 1327 of file treemap.cpp.
QString TreeMapWidget::defaultFieldType | ( | int | f | ) | const |
Definition at line 1322 of file treemap.cpp.
bool TreeMapWidget::defaultFieldVisible | ( | int | f | ) | const |
Definition at line 1332 of file treemap.cpp.
void TreeMapWidget::deletingItem | ( | TreeMapItem * | i | ) |
Definition at line 1501 of file treemap.cpp.
|
signal |
void TreeMapWidget::drawFrame | ( | int | d, |
bool | b | ||
) |
Definition at line 1265 of file treemap.cpp.
void TreeMapWidget::drawTreeMap | ( | ) |
Definition at line 2178 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 2148 of file treemap.cpp.
bool TreeMapWidget::fieldForced | ( | int | f | ) | const |
Definition at line 1434 of file treemap.cpp.
DrawParams::Position TreeMapWidget::fieldPosition | ( | int | f | ) | const |
Definition at line 1453 of file treemap.cpp.
QString TreeMapWidget::fieldPositionString | ( | int | f | ) | const |
Definition at line 1479 of file treemap.cpp.
QString TreeMapWidget::fieldStop | ( | int | f | ) | const |
Definition at line 1398 of file treemap.cpp.
QString TreeMapWidget::fieldType | ( | int | f | ) | const |
Definition at line 1382 of file treemap.cpp.
bool TreeMapWidget::fieldVisible | ( | int | f | ) | const |
Definition at line 1415 of file treemap.cpp.
|
protected |
Definition at line 2142 of file treemap.cpp.
bool TreeMapWidget::isSelected | ( | TreeMapItem * | i | ) | const |
Definition at line 1722 of file treemap.cpp.
TreeMapItem * TreeMapWidget::item | ( | int | x, |
int | y | ||
) | const |
Returns the area item at position x/y, independent from any maxSelectDepth setting.
Definition at line 1541 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 2027 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1980 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1907 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1839 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1950 of file treemap.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 2171 of file treemap.cpp.
TreeMapItem * TreeMapWidget::possibleSelection | ( | TreeMapItem * | i | ) | const |
Returns the item possible for selection.
this returns the given item itself or a parent thereof, depending on setting of maxSelectDepth().
Definition at line 1593 of file treemap.cpp.
void TreeMapWidget::redraw | ( | TreeMapItem * | i | ) |
Redraws an item with all children.
This takes changed values(), sums(), colors() and text() into account.
Definition at line 2231 of file treemap.cpp.
|
inline |
|
signal |
|
signal |
|
inline |
|
signal |
|
signal |
|
inline |
void TreeMapWidget::setAllowRotation | ( | bool | enable | ) |
Do we allow the texts to be rotated by 90 degrees for better fitting?
Definition at line 1281 of file treemap.cpp.
void TreeMapWidget::setBorderWidth | ( | int | w | ) |
Definition at line 1306 of file treemap.cpp.
void TreeMapWidget::setCurrent | ( | TreeMapItem * | i, |
bool | kbd = false |
||
) |
Sets the current item.
The current item is mainly used for keyboard navigation.
Definition at line 1735 of file treemap.cpp.
void TreeMapWidget::setFieldForced | ( | int | f, |
bool | enable | ||
) |
Should the drawing of the name into the rectangle be forced? This enables drawing of the name before drawing subitems, and thus destroys proportional constrains.
Definition at line 1423 of file treemap.cpp.
void TreeMapWidget::setFieldPosition | ( | int | f, |
DrawParams::Position | pos | ||
) |
Set the field position in the area.
Definition at line 1442 of file treemap.cpp.
void TreeMapWidget::setFieldPosition | ( | int | f, |
const QString & | pos | ||
) |
Definition at line 1461 of file treemap.cpp.
void TreeMapWidget::setFieldStop | ( | int | f, |
const QString & | stop | ||
) |
Stop drawing at item with name.
Definition at line 1388 of file treemap.cpp.
void TreeMapWidget::setFieldType | ( | int | f, |
const QString & | type | ||
) |
Set the type name of a field.
This is important for the visualization menu generated with visualizationMenu()
Definition at line 1373 of file treemap.cpp.
void TreeMapWidget::setFieldVisible | ( | int | f, |
bool | enable | ||
) |
Should the text with number textNo be visible? This is only done if remaining space is enough to allow for proportional size constrains.
Definition at line 1404 of file treemap.cpp.
void TreeMapWidget::setMarked | ( | int | markNo = 1 , |
bool | redraw = true |
||
) |
Switches on the marking <markNo>.
Marking 0 switches off marking. This is mutually exclusive to selection, and is automatically switched off when selection is changed (also by the user). Marking is visually the same as selection, and is based on TreeMapItem::isMarked(<markNo>). This enables to programmatically show multiple selected items at once even in single selection mode.
Definition at line 1647 of file treemap.cpp.
void TreeMapWidget::setMaxDrawingDepth | ( | int | d | ) |
Maximal nesting depth.
Definition at line 1314 of file treemap.cpp.
|
inline |
Set the maximal depth a selected item can have.
If you try to select a item with higher depth, the ancestor holding this condition is used.
See also possibleSelection().
void TreeMapWidget::setMinimalArea | ( | int | area | ) |
Minimal area for rectangles to draw.
Definition at line 1492 of file treemap.cpp.
void TreeMapWidget::setRangeSelection | ( | TreeMapItem * | i1, |
TreeMapItem * | i2, | ||
bool | selected | ||
) |
Selects or unselects items in a range.
This is needed internally for Shift-Click in Extended mode. Range means for a hierarchical widget:
- select/unselect i1 and i2 according selected
- search common parent of i1 and i2, and select/unselect the range of direct children between but excluding the child leading to i1 and the child leading to i2.
Definition at line 1764 of file treemap.cpp.
void TreeMapWidget::setSelected | ( | TreeMapItem * | item, |
bool | selected = true |
||
) |
Selects or unselects an item.
In multiselection mode, the constrain that a selected item has no selected children or parents stays true.
Definition at line 1626 of file treemap.cpp.
|
inline |
void TreeMapWidget::setShadingEnabled | ( | bool | s | ) |
Definition at line 1257 of file treemap.cpp.
void TreeMapWidget::setSkipIncorrectBorder | ( | bool | enable = true | ) |
If a children value() is almost the parents sum(), it can happen that the border to be drawn for visibilty of nesting relations takes to much space, and the parent/child size relation can not be mapped to a correct area size relation.
Either (1) Ignore the incorrect drawing, or (2) Skip drawing of the parent level alltogether.
Definition at line 1298 of file treemap.cpp.
void TreeMapWidget::setSplitMode | ( | TreeMapItem::SplitMode | m | ) |
for setting/getting global split direction
Definition at line 1209 of file treemap.cpp.
bool TreeMapWidget::setSplitMode | ( | const QString & | mode | ) |
Definition at line 1222 of file treemap.cpp.
void TreeMapWidget::setTransparent | ( | int | d, |
bool | b | ||
) |
Definition at line 1273 of file treemap.cpp.
void TreeMapWidget::setVisibleWidth | ( | int | width, |
bool | reuseSpace = false |
||
) |
Items usually have a size proportional to their value().
With <width>, you can give the minimum width of the resulting rectangle to still be drawn. For space not used because of to small items, you can specify with <reuseSpace> if the background should shine through or the space will be used to enlarge the next item to be drawn at this level.
Definition at line 1289 of file treemap.cpp.
|
protectedslot |
Definition at line 2835 of file treemap.cpp.
TreeMapItem::SplitMode TreeMapWidget::splitMode | ( | ) | const |
Definition at line 1217 of file treemap.cpp.
QString TreeMapWidget::splitModeString | ( | ) | const |
Definition at line 1238 of file treemap.cpp.
|
virtual |
Return tooltip string to show for a item (can be rich text) Default implementation gives lines with "text0 (text1)" going to root.
Reimplemented in PartAreaWidget, and CallMapView.
Definition at line 1521 of file treemap.cpp.
TreeMapItem * TreeMapWidget::visibleItem | ( | TreeMapItem * | i | ) | const |
Returns the nearest item with a visible area; this can be the given item itself.
Definition at line 1607 of file treemap.cpp.
|
inline |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:39:51 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.