umbrello/umbrello
#include <codeaccessormethod.h>
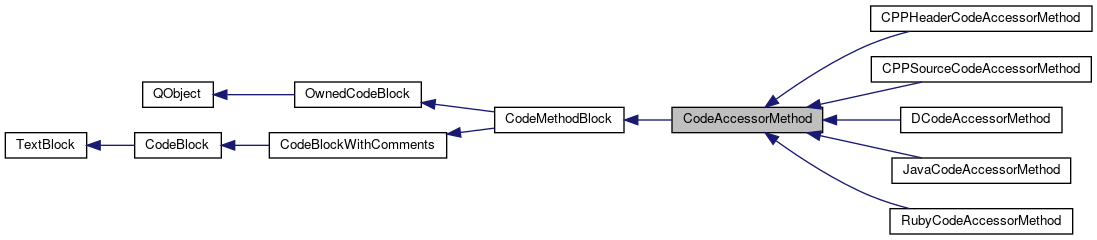
Public Types | |
enum | AccessorType { GET =0, SET, ADD, REMOVE, LIST } |
![]() | |
enum | ContentType { AutoGenerated =0, UserGenerated } |
Public Member Functions | |
CodeAccessorMethod (CodeClassField *field) | |
virtual | ~CodeAccessorMethod () |
CodeClassField * | getParentClassField () |
AccessorType | getType () |
virtual void | loadFromXMI (QDomElement &root) |
bool | parentIsAttribute () |
virtual void | saveToXMI (QDomDocument &doc, QDomElement &root) |
virtual void | setAttributesFromObject (TextBlock *obj) |
void | setType (AccessorType type) |
virtual void | updateContent ()=0 |
![]() | |
CodeMethodBlock (ClassifierCodeDocument *doc, UMLObject *parentObj, const QString &body=QString(), const QString &comment=QString()) | |
virtual | ~CodeMethodBlock () |
QString | getEndMethodText () const |
CodeDocument * | getParentDocument () |
QString | getStartMethodText () const |
virtual QString | toString () const |
![]() | |
OwnedCodeBlock (UMLObject *parent) | |
virtual | ~OwnedCodeBlock () |
UMLObject * | getParentObject () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
CodeBlockWithComments (CodeDocument *parent, const QString &body=QString(), const QString &comment=QString()) | |
virtual | ~CodeBlockWithComments () |
CodeComment * | getComment () const |
void | setComment (CodeComment *object) |
void | setOverallIndentationLevel (int level) |
![]() | |
CodeBlock (CodeDocument *parent, const QString &body=QString()) | |
virtual | ~CodeBlock () |
ContentType | contentType () const |
void | setContentType (ContentType new_var) |
![]() | |
TextBlock (CodeDocument *parent, const QString &text=QString()) | |
virtual | ~TextBlock () |
void | appendText (const QString &text) |
bool | canDelete () const |
virtual int | firstEditableLine () |
int | getIndentationLevel () const |
QString | getIndentationString (int level=0) const |
virtual QString | getNewEditorLine (int amount=0) |
CodeDocument * | getParentDocument () const |
QString | getTag () const |
QString | getText () const |
bool | getWriteOutText () const |
virtual int | lastEditableLine () |
void | setIndentationLevel (int level) |
void | setTag (const QString &value) |
void | setText (const QString &text) |
void | setWriteOutText (bool write) |
virtual QString | unformatText (const QString &text, const QString &indent=QString()) |
Protected Member Functions | |
void | forceRelease () |
virtual void | release () |
virtual void | setAttributesFromNode (QDomElement &element) |
virtual void | setAttributesOnNode (QDomDocument &doc, QDomElement &blockElement) |
virtual void | updateMethodDeclaration ()=0 |
![]() | |
void | setEndMethodText (const QString &value) |
void | setStartMethodText (const QString &value) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
void | setCanDelete (bool canDelete) |
Additional Inherited Members | |
![]() | |
virtual void | syncToParent () |
![]() | |
virtual void | syncToParent () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | enumToString (const ContentType &val) |
![]() | |
static QString | decodeText (const QString &text, const QString &endLine) |
static QString | encodeText (const QString &text, const QString &endLine) |
static QString | formatMultiLineText (const QString &work, const QString &linePrefix, const QString &breakStr, bool addBreak=true, bool lastLineHasBreak=true) |
static QString | getIndentation () |
static QString | getNewLineEndingChars () |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Definition at line 20 of file codeaccessormethod.h.
Member Enumeration Documentation
Enumerator | |
---|---|
GET | |
SET | |
ADD | |
REMOVE | |
LIST |
Definition at line 32 of file codeaccessormethod.h.
Constructor & Destructor Documentation
|
explicit |
Constructors.
Definition at line 23 of file codeaccessormethod.cpp.
|
virtual |
Empty Destructor.
Definition at line 32 of file codeaccessormethod.cpp.
Member Function Documentation
|
protected |
A method so the parent code classfield can force code block to release.
Definition at line 91 of file codeaccessormethod.cpp.
CodeClassField * CodeAccessorMethod::getParentClassField | ( | ) |
Get the value of m_parentclassfield.
- Returns
- the value of m_parentclassfield
Definition at line 40 of file codeaccessormethod.cpp.
CodeAccessorMethod::AccessorType CodeAccessorMethod::getType | ( | ) |
Utility method to get the value of the parent object of the parent classifield.
- Returns
- the value of the parent of the parent classfield Return the type of accessor method this is.
Definition at line 64 of file codeaccessormethod.cpp.
|
virtual |
Load params from the appropriate XMI element node.
Reimplemented from CodeBlockWithComments.
Definition at line 102 of file codeaccessormethod.cpp.
bool CodeAccessorMethod::parentIsAttribute | ( | ) |
Definition at line 45 of file codeaccessormethod.cpp.
|
protectedvirtual |
This type of textblock is special we DON'T release it when resetTextBlocks is called because we re-use it over and over until the codeclassfield is released.
Reimplemented from CodeMethodBlock.
Definition at line 83 of file codeaccessormethod.cpp.
|
virtual |
Save the XMI representation of this object.
Reimplemented from CodeBlockWithComments.
Definition at line 110 of file codeaccessormethod.cpp.
|
protectedvirtual |
Set the class attributes of this object from the passed element node.
Reimplemented from CodeMethodBlock.
Reimplemented in DCodeAccessorMethod, JavaCodeAccessorMethod, and RubyCodeAccessorMethod.
Definition at line 137 of file codeaccessormethod.cpp.
|
virtual |
Set the class attributes from a passed object.
Reimplemented from CodeMethodBlock.
Definition at line 170 of file codeaccessormethod.cpp.
|
protectedvirtual |
Set attributes of the node that represents this class in the XMI document.
Reimplemented from CodeMethodBlock.
Reimplemented in DCodeAccessorMethod, JavaCodeAccessorMethod, and RubyCodeAccessorMethod.
Definition at line 123 of file codeaccessormethod.cpp.
void CodeAccessorMethod::setType | ( | CodeAccessorMethod::AccessorType | atype | ) |
Set the type of accessor method this is.
Definition at line 72 of file codeaccessormethod.cpp.
|
pure virtual |
This is the method called from within syncToparent() to update the body of the method.
It is only called if the method is Auto-generated.
Implements CodeMethodBlock.
Implemented in CPPHeaderCodeAccessorMethod, CPPSourceCodeAccessorMethod, DCodeAccessorMethod, JavaCodeAccessorMethod, and RubyCodeAccessorMethod.
|
protectedpure virtual |
This is the method called from within syncToparent().
To update the start and end Method text. It is called whether or not the method is Auto or User generated.
Implements CodeMethodBlock.
Implemented in CPPHeaderCodeAccessorMethod, CPPSourceCodeAccessorMethod, DCodeAccessorMethod, JavaCodeAccessorMethod, and RubyCodeAccessorMethod.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.