umbrello/umbrello
#include <codeclassfield.h>
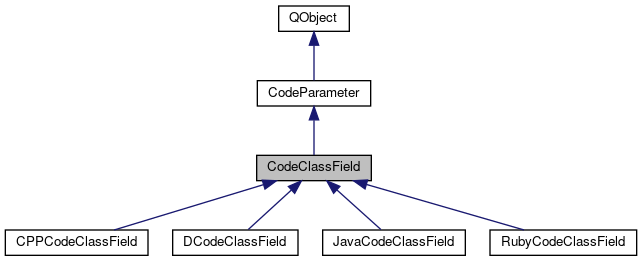
Public Types | |
enum | ClassFieldType { Attribute, PlainAssociation, Self, Aggregation, Composition, Unknown_Assoc } |
Signals | |
void | modified () |
Public Member Functions | |
CodeClassField (ClassifierCodeDocument *parentDoc, UMLAttribute *attrib) | |
CodeClassField (ClassifierCodeDocument *parentDoc, UMLRole *role) | |
virtual | ~CodeClassField () |
bool | fieldIsSingleValue () |
CodeAccessorMethod * | findMethodByType (CodeAccessorMethod::AccessorType type, int role_id=-1) |
void | finishInitialization () |
ClassFieldType | getClassFieldType () const |
CodeClassFieldDeclarationBlock * | getDeclarationCodeBlock () |
QString | getListObjectType () |
CodeAccessorMethodList | getMethodList () const |
QString | getTypeName () |
bool | getWriteOutMethods () const |
virtual void | loadFromXMI (QDomElement &root) |
int | maximumListOccurances () |
int | minimumListOccurances () |
bool | parentIsAttribute () const |
virtual void | saveToXMI (QDomDocument &doc, QDomElement &root) |
void | setWriteOutMethods (bool val) |
virtual void | synchronize () |
void | updateContent () |
![]() | |
CodeParameter (ClassifierCodeDocument *doc, UMLObject *parentObj) | |
virtual | ~CodeParameter () |
bool | getAbstract () |
CodeComment * | getComment () |
virtual QString | getInitialValue () |
QString | getName () const |
ClassifierCodeDocument * | getParentDocument () |
UMLObject * | getParentObject () |
bool | getStatic () |
Uml::Visibility::Enum | getVisibility () const |
QString | ID () |
void | setComment (CodeComment *comment) |
virtual void | setInitialValue (const QString &new_var) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
bool | addMethod (CodeAccessorMethod *add) |
QString | cleanName (const QString &name) |
QString | fixInitialStringDeclValue (const QString &val, const QString &type) |
QString | getUMLObjectName (UMLObject *obj) |
bool | removeMethod (CodeAccessorMethod *remove) |
void | setListClassName (const QString &className) |
void | setParentUMLObject (UMLObject *obj) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
void | syncToParent () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
class CodeClassField a special type of parameter.
. occurs on class declarations.
Definition at line 29 of file codeclassfield.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Attribute | |
PlainAssociation | |
Self | |
Aggregation | |
Composition | |
Unknown_Assoc |
Definition at line 34 of file codeclassfield.h.
Constructor & Destructor Documentation
CodeClassField::CodeClassField | ( | ClassifierCodeDocument * | parentDoc, |
UMLAttribute * | attrib | ||
) |
Constructor.
Definition at line 42 of file codeclassfield.cpp.
CodeClassField::CodeClassField | ( | ClassifierCodeDocument * | parentDoc, |
UMLRole * | role | ||
) |
Constructor.
Definition at line 32 of file codeclassfield.cpp.
|
virtual |
Empty Destructor.
Definition at line 52 of file codeclassfield.cpp.
Member Function Documentation
|
protected |
Add a Method object to the m_methodVector List.
Definition at line 156 of file codeclassfield.cpp.
A little utility method to make life easier for code document programmers.
Definition at line 376 of file codeclassfield.cpp.
bool CodeClassField::fieldIsSingleValue | ( | ) |
Determine whether the parent object in this classfield indicates that it is a single variable or a List (Vector).
One day this will be done correctly with special multiplicity object.
Definition at line 607 of file codeclassfield.cpp.
CodeAccessorMethod * CodeClassField::findMethodByType | ( | CodeAccessorMethod::AccessorType | type, |
int | role_id = -1 |
||
) |
Utility method to allow finding particular accessor method of this code class field by its type identifier.
Definition at line 417 of file codeclassfield.cpp.
void CodeClassField::finishInitialization | ( | ) |
Finish off initializations of the object.
This is necessary as a separate method because we cannot call virtual methods that are reimplemented in a language specific class during our own construction (the own object is not finished being constructed and therefore the C++ dispatch mechanism does not yet work as expected.)
Definition at line 650 of file codeclassfield.cpp.
|
protected |
Another utility method to make life easier for code document programmers this one fixes the initial declared value of string attributes so that if it is empty or lacking quotations, it comes out as "".
Definition at line 386 of file codeclassfield.cpp.
CodeClassField::ClassFieldType CodeClassField::getClassFieldType | ( | ) | const |
Get the type of classfield this is.
Definition at line 135 of file codeclassfield.cpp.
CodeClassFieldDeclarationBlock * CodeClassField::getDeclarationCodeBlock | ( | ) |
Return the declaration statement for this class field object.
will be empty until this (abstract) class is inherited in elsewhere.
Definition at line 220 of file codeclassfield.cpp.
QString CodeClassField::getListObjectType | ( | ) |
Definition at line 112 of file codeclassfield.cpp.
CodeAccessorMethodList CodeClassField::getMethodList | ( | ) | const |
Get the list of Method objects held by m_methodVector.
- Returns
- QPtrList<CodeMethodBlock *> list of Method objects held by m_methodVector
Definition at line 190 of file codeclassfield.cpp.
|
virtual |
Get the value of m_dialog.
- Returns
- the value of m_dialog
Reimplemented from CodeParameter.
Reimplemented in RubyCodeClassField, DCodeClassField, and JavaCodeClassField.
Definition at line 94 of file codeclassfield.cpp.
Definition at line 148 of file codeclassfield.cpp.
bool CodeClassField::getWriteOutMethods | ( | ) | const |
Determine if we will allow methods to be viewable.
this flag is often used to toggle autogeneration of accessor methods in the code class field.
Definition at line 200 of file codeclassfield.cpp.
|
virtual |
Load params from the appropriate XMI element node.
Definition at line 228 of file codeclassfield.cpp.
int CodeClassField::maximumListOccurances | ( | ) |
Find the maximum number of things that can occur in an association If mistakenly called on attribute CF's the default value of is "1" is returned.
If the association (role) CF doesn't have a multiplicty or has a "*" specified then '-1' (unbounded) is returned.
Definition at line 352 of file codeclassfield.cpp.
int CodeClassField::minimumListOccurances | ( | ) |
Find the minimum number of things that can occur in an association If mistakenly called on attribute CF's the default value of is "0" is returned.
Similarly, if the association (role) CF doesn't have a multiplicty 0 is returned.
Definition at line 328 of file codeclassfield.cpp.
|
signal |
bool CodeClassField::parentIsAttribute | ( | ) | const |
Get the value of m_isAbstract.
- Returns
- the value of m_isAbstract
Definition at line 126 of file codeclassfield.cpp.
|
protected |
Remove a Method object from m_methodVector List.
Definition at line 177 of file codeclassfield.cpp.
|
virtual |
Save the XMI representation of this object.
Definition at line 313 of file codeclassfield.cpp.
|
protected |
|
protected |
Set the parent UMLobject appropriately.
Definition at line 74 of file codeclassfield.cpp.
void CodeClassField::setWriteOutMethods | ( | bool | val | ) |
Determine if we will allow methods to be viewable.
this flag is often used to toggle autogeneration of accessor methods in the code class field.
Definition at line 210 of file codeclassfield.cpp.
|
virtual |
Force the synchronization of the content (methods and declarations) of this class field.
Definition at line 403 of file codeclassfield.cpp.
|
virtual |
Updates the status of the accessor methods as to whether or not they should be written out.
Implements CodeParameter.
Definition at line 509 of file codeclassfield.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.