umbrello/umbrello
#include <codeparameter.h>
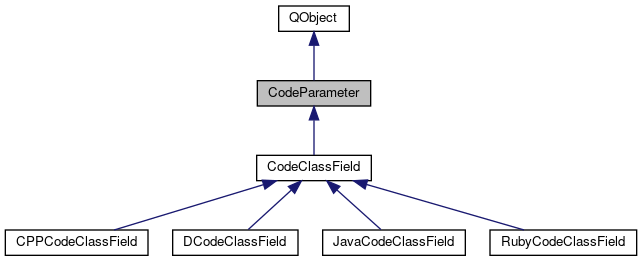
Public Slots | |
void | syncToParent () |
Public Member Functions | |
CodeParameter (ClassifierCodeDocument *doc, UMLObject *parentObj) | |
virtual | ~CodeParameter () |
bool | getAbstract () |
CodeComment * | getComment () |
virtual QString | getInitialValue () |
QString | getName () const |
ClassifierCodeDocument * | getParentDocument () |
UMLObject * | getParentObject () |
bool | getStatic () |
virtual QString | getTypeName () |
Uml::Visibility::Enum | getVisibility () const |
QString | ID () |
void | setComment (CodeComment *comment) |
virtual void | setInitialValue (const QString &new_var) |
virtual void | updateContent ()=0 |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual void | setAttributesFromNode (QDomElement &element) |
virtual void | setAttributesOnNode (QDomDocument &doc, QDomElement &blockElement) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
class CodeParameter A parameter on some type of code.
Definition at line 25 of file codeparameter.h.
Constructor & Destructor Documentation
CodeParameter::CodeParameter | ( | ClassifierCodeDocument * | doc, |
UMLObject * | parentObj | ||
) |
Constructor.
Definition at line 29 of file codeparameter.cpp.
|
virtual |
Destructor.
Definition at line 39 of file codeparameter.cpp.
Member Function Documentation
bool CodeParameter::getAbstract | ( | ) |
Utility method to get the value of parent object abstract value.
- Returns
- the value of parent object abstrtact
Definition at line 47 of file codeparameter.cpp.
CodeComment * CodeParameter::getComment | ( | ) |
Get the Comment on this object.
Definition at line 125 of file codeparameter.cpp.
|
virtual |
Get the value of m_initialValue The initial value of this code parameter.
- Returns
- the value of m_initialValue
Reimplemented in RubyCodeClassField, CPPCodeClassField, DCodeClassField, and JavaCodeClassField.
Definition at line 109 of file codeparameter.cpp.
QString CodeParameter::getName | ( | ) | const |
Utility method to get the value of parent object name The name of this code parameter.
- Returns
- the value
Definition at line 67 of file codeparameter.cpp.
ClassifierCodeDocument * CodeParameter::getParentDocument | ( | ) |
Get the parent Code Document.
Definition at line 133 of file codeparameter.cpp.
UMLObject * CodeParameter::getParentObject | ( | ) |
Get the ParentObject object.
Definition at line 141 of file codeparameter.cpp.
bool CodeParameter::getStatic | ( | ) |
Utility method to get the value of parent object static Whether or not this is static.
- Returns
- the value of static
Definition at line 57 of file codeparameter.cpp.
|
virtual |
Utility method to get the value of parent object type.
the typeName of this parameters (e.g. boolean, int, etc or perhaps Class name of an object)
- Returns
- the value of type
Reimplemented in CodeClassField, RubyCodeClassField, DCodeClassField, and JavaCodeClassField.
Definition at line 78 of file codeparameter.cpp.
Uml::Visibility::Enum CodeParameter::getVisibility | ( | ) | const |
Utility method to get the value of parent object scope.
The visibility of this code parameter.
- Returns
- the value of parent object scope
Definition at line 89 of file codeparameter.cpp.
QString CodeParameter::ID | ( | ) |
Definition at line 148 of file codeparameter.cpp.
|
protectedvirtual |
Set the class attributes of this object from the passed element node.
Definition at line 192 of file codeparameter.cpp.
|
protectedvirtual |
Set attributes of the node that represents this class in the XMI document.
Definition at line 166 of file codeparameter.cpp.
void CodeParameter::setComment | ( | CodeComment * | comment | ) |
Set a Comment object.
Definition at line 117 of file codeparameter.cpp.
|
virtual |
Set the value of m_initialValue.
The initial value of this code parameter.
- Parameters
-
new_var the new value of m_initialValue
Definition at line 99 of file codeparameter.cpp.
|
slot |
Create the string representation of this code parameter.
- Returns
- QString
Definition at line 268 of file codeparameter.cpp.
|
pure virtual |
Implemented in CodeClassField.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.