umbrello/umbrello
#include <umllistview.h>
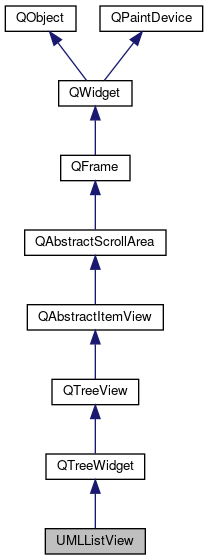
Public Slots | |
void | childObjectAdded (UMLClassifierListItem *obj) |
void | childObjectRemoved (UMLClassifierListItem *obj) |
void | collapseAll (UMLListViewItem *item) |
void | connectNewObjectsSlots (UMLObject *object) |
void | expandAll (UMLListViewItem *item) |
void | slotCutSuccessful () |
void | slotDeleteSelectedItems () |
void | slotDiagramCreated (Uml::ID::Type id) |
void | slotDiagramRemoved (Uml::ID::Type id) |
void | slotDiagramRenamed (Uml::ID::Type id) |
void | slotDropped (QDropEvent *de, UMLListViewItem *target) |
void | slotMenuSelection (QAction *action, const QPoint &position=QPoint()) |
void | slotObjectChanged () |
void | slotObjectCreated (UMLObject *object) |
void | slotObjectRemoved (UMLObject *object) |
Public Member Functions | |
UMLListView (QWidget *parent=0) | |
~UMLListView () | |
void | addNewItem (UMLListViewItem *parent, UMLListViewItem::ListViewType type) |
void | changeIconOf (UMLObject *o, Icon_Utils::IconType to) |
void | clean () |
void | closeDatatypesFolder () |
UMLListViewItem * | createDiagramItem (UMLView *view) |
bool | createItem (UMLListViewItem *item) |
UMLListViewItem * | determineParentItem (UMLObject *object) const |
UMLListViewItem * | determineParentItem (UMLListViewItem::ListViewType lvt) const |
UMLDoc * | document () const |
UMLListViewItem * | findFolderForDiagram (Uml::DiagramType::Enum dt) |
UMLListViewItem * | findItem (Uml::ID::Type id) |
UMLListViewItem * | findUMLObject (const UMLObject *p) const |
UMLListViewItem * | findView (UMLView *v) |
void | init () |
bool | isUnique (UMLListViewItem *item, const QString &name) |
bool | loadChildrenFromXMI (UMLListViewItem *parent, QDomElement &element) |
bool | loadFromXMI (QDomElement &element) |
UMLListViewItem * | moveObject (Uml::ID::Type srcId, UMLListViewItem::ListViewType srcType, UMLListViewItem *newParent) |
UMLListViewItem * | rootView (UMLListViewItem::ListViewType type) |
UMLListViewItem::ListViewType | rootViewType (UMLListViewItem *item) |
void | saveToXMI (QDomDocument &qDoc, QDomElement &qElement) |
UMLListViewItemList | selectedItems () |
int | selectedItemsCount () |
UMLListViewItemList | selectedItemsRoot () |
void | setDocument (UMLDoc *doc) |
void | setStartedCopy (bool startedCopy) |
void | setStartedCut (bool startedCut) |
void | setTitle (int column, const QString &text) |
void | setView (UMLView *view) |
bool | startedCopy () const |
UMLListViewItem * | theComponentView () |
UMLListViewItem * | theDatatypeFolder () |
UMLListViewItem * | theDeploymentView () |
UMLListViewItem * | theLogicalView () |
UMLListViewItem * | theRootView () |
UMLListViewItem * | theUseCaseView () |
![]() | |
QTreeWidget (QWidget *parent) | |
~QTreeWidget () | |
void | addTopLevelItem (QTreeWidgetItem *item) |
void | addTopLevelItems (const QList< QTreeWidgetItem * > &items) |
void | clear () |
void | closePersistentEditor (QTreeWidgetItem *item, int column) |
void | collapseItem (const QTreeWidgetItem *item) |
int | columnCount () const |
int | currentColumn () const |
QTreeWidgetItem * | currentItem () const |
void | currentItemChanged (QTreeWidgetItem *current, QTreeWidgetItem *previous) |
void | editItem (QTreeWidgetItem *item, int column) |
void | expandItem (const QTreeWidgetItem *item) |
QList< QTreeWidgetItem * > | findItems (const QString &text, QFlags< Qt::MatchFlag > flags, int column) const |
QTreeWidgetItem * | headerItem () const |
int | indexOfTopLevelItem (QTreeWidgetItem *item) const |
void | insertTopLevelItem (int index, QTreeWidgetItem *item) |
void | insertTopLevelItems (int index, const QList< QTreeWidgetItem * > &items) |
QTreeWidgetItem * | invisibleRootItem () const |
bool | isFirstItemColumnSpanned (const QTreeWidgetItem *item) const |
bool | isItemExpanded (const QTreeWidgetItem *item) const |
bool | isItemHidden (const QTreeWidgetItem *item) const |
bool | isItemSelected (const QTreeWidgetItem *item) const |
QTreeWidgetItem * | itemAbove (const QTreeWidgetItem *item) const |
void | itemActivated (QTreeWidgetItem *item, int column) |
QTreeWidgetItem * | itemAt (const QPoint &p) const |
QTreeWidgetItem * | itemAt (int x, int y) const |
QTreeWidgetItem * | itemBelow (const QTreeWidgetItem *item) const |
void | itemChanged (QTreeWidgetItem *item, int column) |
void | itemClicked (QTreeWidgetItem *item, int column) |
void | itemCollapsed (QTreeWidgetItem *item) |
void | itemDoubleClicked (QTreeWidgetItem *item, int column) |
void | itemEntered (QTreeWidgetItem *item, int column) |
void | itemExpanded (QTreeWidgetItem *item) |
void | itemPressed (QTreeWidgetItem *item, int column) |
void | itemSelectionChanged () |
QWidget * | itemWidget (QTreeWidgetItem *item, int column) const |
void | openPersistentEditor (QTreeWidgetItem *item, int column) |
void | removeItemWidget (QTreeWidgetItem *item, int column) |
void | scrollToItem (const QTreeWidgetItem *item, QAbstractItemView::ScrollHint hint) |
QList< QTreeWidgetItem * > | selectedItems () const |
void | setColumnCount (int columns) |
void | setCurrentItem (QTreeWidgetItem *item) |
void | setCurrentItem (QTreeWidgetItem *item, int column) |
void | setCurrentItem (QTreeWidgetItem *item, int column, QFlags< QItemSelectionModel::SelectionFlag > command) |
void | setFirstItemColumnSpanned (const QTreeWidgetItem *item, bool span) |
void | setHeaderItem (QTreeWidgetItem *item) |
void | setHeaderLabel (const QString &label) |
void | setHeaderLabels (const QStringList &labels) |
void | setItemExpanded (const QTreeWidgetItem *item, bool expand) |
void | setItemHidden (const QTreeWidgetItem *item, bool hide) |
void | setItemSelected (const QTreeWidgetItem *item, bool select) |
void | setItemWidget (QTreeWidgetItem *item, int column, QWidget *widget) |
virtual void | setSelectionModel (QItemSelectionModel *selectionModel) |
int | sortColumn () const |
void | sortItems (int column, Qt::SortOrder order) |
QTreeWidgetItem * | takeTopLevelItem (int index) |
QTreeWidgetItem * | topLevelItem (int index) const |
int | topLevelItemCount () const |
QRect | visualItemRect (const QTreeWidgetItem *item) const |
![]() | |
QTreeView (QWidget *parent) | |
~QTreeView () | |
bool | allColumnsShowFocus () const |
int | autoExpandDelay () const |
void | collapse (const QModelIndex &index) |
void | collapseAll () |
void | collapsed (const QModelIndex &index) |
int | columnAt (int x) const |
int | columnViewportPosition (int column) const |
int | columnWidth (int column) const |
virtual void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight) |
void | expand (const QModelIndex &index) |
void | expandAll () |
void | expanded (const QModelIndex &index) |
bool | expandsOnDoubleClick () const |
void | expandToDepth (int depth) |
QHeaderView * | header () const |
void | hideColumn (int column) |
int | indentation () const |
QModelIndex | indexAbove (const QModelIndex &index) const |
virtual QModelIndex | indexAt (const QPoint &point) const |
QModelIndex | indexBelow (const QModelIndex &index) const |
bool | isAnimated () const |
bool | isColumnHidden (int column) const |
bool | isExpanded (const QModelIndex &index) const |
bool | isFirstColumnSpanned (int row, const QModelIndex &parent) const |
bool | isHeaderHidden () const |
bool | isRowHidden (int row, const QModelIndex &parent) const |
bool | isSortingEnabled () const |
bool | itemsExpandable () const |
virtual void | keyboardSearch (const QString &search) |
virtual void | reset () |
void | resizeColumnToContents (int column) |
bool | rootIsDecorated () const |
virtual void | scrollTo (const QModelIndex &index, ScrollHint hint) |
virtual void | selectAll () |
void | setAllColumnsShowFocus (bool enable) |
void | setAnimated (bool enable) |
void | setAutoExpandDelay (int delay) |
void | setColumnHidden (int column, bool hide) |
void | setColumnWidth (int column, int width) |
void | setExpanded (const QModelIndex &index, bool expanded) |
void | setExpandsOnDoubleClick (bool enable) |
void | setFirstColumnSpanned (int row, const QModelIndex &parent, bool span) |
void | setHeader (QHeaderView *header) |
void | setHeaderHidden (bool hide) |
void | setIndentation (int i) |
void | setItemsExpandable (bool enable) |
virtual void | setModel (QAbstractItemModel *model) |
virtual void | setRootIndex (const QModelIndex &index) |
void | setRootIsDecorated (bool show) |
void | setRowHidden (int row, const QModelIndex &parent, bool hide) |
void | setSortingEnabled (bool enable) |
void | setUniformRowHeights (bool uniform) |
void | setWordWrap (bool on) |
void | showColumn (int column) |
void | sortByColumn (int column) |
void | sortByColumn (int column, Qt::SortOrder order) |
bool | uniformRowHeights () const |
virtual QRect | visualRect (const QModelIndex &index) const |
bool | wordWrap () const |
![]() | |
QAbstractItemView (QWidget *parent) | |
~QAbstractItemView () | |
void | activated (const QModelIndex &index) |
bool | alternatingRowColors () const |
int | autoScrollMargin () const |
void | clearSelection () |
void | clicked (const QModelIndex &index) |
void | closePersistentEditor (const QModelIndex &index) |
QModelIndex | currentIndex () const |
Qt::DropAction | defaultDropAction () const |
void | doubleClicked (const QModelIndex &index) |
DragDropMode | dragDropMode () const |
bool | dragDropOverwriteMode () const |
bool | dragEnabled () const |
void | edit (const QModelIndex &index) |
EditTriggers | editTriggers () const |
void | entered (const QModelIndex &index) |
bool | hasAutoScroll () const |
ScrollMode | horizontalScrollMode () const |
QSize | iconSize () const |
virtual QModelIndex | indexAt (const QPoint &point) const =0 |
QWidget * | indexWidget (const QModelIndex &index) const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
QAbstractItemDelegate * | itemDelegate () const |
QAbstractItemDelegate * | itemDelegate (const QModelIndex &index) const |
QAbstractItemDelegate * | itemDelegateForColumn (int column) const |
QAbstractItemDelegate * | itemDelegateForRow (int row) const |
QAbstractItemModel * | model () const |
void | openPersistentEditor (const QModelIndex &index) |
void | pressed (const QModelIndex &index) |
QModelIndex | rootIndex () const |
virtual void | scrollTo (const QModelIndex &index, ScrollHint hint)=0 |
void | scrollToBottom () |
void | scrollToTop () |
QAbstractItemView::SelectionBehavior | selectionBehavior () const |
QAbstractItemView::SelectionMode | selectionMode () const |
QItemSelectionModel * | selectionModel () const |
void | setAlternatingRowColors (bool enable) |
void | setAutoScroll (bool enable) |
void | setAutoScrollMargin (int margin) |
void | setCurrentIndex (const QModelIndex &index) |
void | setDefaultDropAction (Qt::DropAction dropAction) |
void | setDragDropMode (DragDropMode behavior) |
void | setDragDropOverwriteMode (bool overwrite) |
void | setDragEnabled (bool enable) |
void | setDropIndicatorShown (bool enable) |
void | setEditTriggers (QFlags< QAbstractItemView::EditTrigger > triggers) |
void | setHorizontalScrollMode (ScrollMode mode) |
void | setIconSize (const QSize &size) |
void | setIndexWidget (const QModelIndex &index, QWidget *widget) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemDelegateForColumn (int column, QAbstractItemDelegate *delegate) |
void | setItemDelegateForRow (int row, QAbstractItemDelegate *delegate) |
void | setSelectionBehavior (QAbstractItemView::SelectionBehavior behavior) |
void | setSelectionMode (QAbstractItemView::SelectionMode mode) |
void | setTabKeyNavigation (bool enable) |
void | setTextElideMode (Qt::TextElideMode mode) |
void | setVerticalScrollMode (ScrollMode mode) |
bool | showDropIndicator () const |
QSize | sizeHintForIndex (const QModelIndex &index) const |
virtual int | sizeHintForRow (int row) const |
bool | tabKeyNavigation () const |
Qt::TextElideMode | textElideMode () const |
void | update (const QModelIndex &index) |
ScrollMode | verticalScrollMode () const |
void | viewportEntered () |
virtual QRect | visualRect (const QModelIndex &index) const =0 |
![]() | |
QAbstractScrollArea (QWidget *parent) | |
~QAbstractScrollArea () | |
void | addScrollBarWidget (QWidget *widget, QFlags< Qt::AlignmentFlag > alignment) |
QWidget * | cornerWidget () const |
QScrollBar * | horizontalScrollBar () const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const |
QSize | maximumViewportSize () const |
virtual QSize | minimumSizeHint () const |
QWidgetList | scrollBarWidgets (QFlags< Qt::AlignmentFlag > alignment) |
void | setCornerWidget (QWidget *widget) |
void | setHorizontalScrollBar (QScrollBar *scrollBar) |
void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setVerticalScrollBar (QScrollBar *scrollBar) |
void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setViewport (QWidget *widget) |
virtual QSize | sizeHint () const |
QScrollBar * | verticalScrollBar () const |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const |
QWidget * | viewport () const |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Static Public Member Functions | |
static bool | mayHaveChildItems (UMLObject::ObjectType type) |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
Protected Slots | |
void | slotCollapsed (QTreeWidgetItem *item) |
void | slotExpanded (QTreeWidgetItem *item) |
void | slotItemSelectionChanged () |
Protected Member Functions | |
bool | acceptDrag (QDropEvent *event) const |
void | addAtContainer (UMLListViewItem *item, UMLListViewItem *parent) |
void | childObjectAdded (UMLClassifierListItem *child, UMLClassifier *parent) |
void | commitData (QWidget *editor) |
void | contextMenuEvent (QContextMenuEvent *event) |
void | deleteChildrenOf (UMLListViewItem *parent) |
bool | deleteItem (UMLListViewItem *temp) |
void | dragEnterEvent (QDragEnterEvent *event) |
void | dragMoveEvent (QDragMoveEvent *event) |
void | dropEvent (QDropEvent *event) |
bool | event (QEvent *e) |
UMLListViewItem * | findUMLObjectInFolder (UMLListViewItem *folder, UMLObject *obj) |
void | focusOutEvent (QFocusEvent *fe) |
UMLDragData * | getDragData () |
void | keyPressEvent (QKeyEvent *ke) |
void | mouseDoubleClickEvent (QMouseEvent *me) |
void | mouseMoveEvent (QMouseEvent *me) |
void | mousePressEvent (QMouseEvent *me) |
void | mouseReleaseEvent (QMouseEvent *me) |
![]() | |
virtual bool | dropMimeData (QTreeWidgetItem *parent, int index, const QMimeData *data, Qt::DropAction action) |
QModelIndex | indexFromItem (QTreeWidgetItem *item, int column) const |
QTreeWidgetItem * | itemFromIndex (const QModelIndex &index) const |
QList< QTreeWidgetItem * > | items (const QMimeData *data) const |
virtual QMimeData * | mimeData (const QList< QTreeWidgetItem * > items) const |
virtual QStringList | mimeTypes () const |
virtual Qt::DropActions | supportedDropActions () const |
![]() | |
void | columnCountChanged (int oldCount, int newCount) |
void | columnMoved () |
void | columnResized (int column, int oldSize, int newSize) |
virtual void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) |
virtual void | drawBranches (QPainter *painter, const QRect &rect, const QModelIndex &index) const |
virtual void | drawRow (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const |
void | drawTree (QPainter *painter, const QRegion ®ion) const |
virtual int | horizontalOffset () const |
int | indexRowSizeHint (const QModelIndex &index) const |
virtual bool | isIndexHidden (const QModelIndex &index) const |
virtual QModelIndex | moveCursor (CursorAction cursorAction, QFlags< Qt::KeyboardModifier > modifiers) |
virtual void | paintEvent (QPaintEvent *event) |
int | rowHeight (const QModelIndex &index) const |
virtual void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
virtual void | rowsInserted (const QModelIndex &parent, int start, int end) |
void | rowsRemoved (const QModelIndex &parent, int start, int end) |
virtual void | scrollContentsBy (int dx, int dy) |
virtual QModelIndexList | selectedIndexes () const |
virtual void | selectionChanged (const QItemSelection &selected, const QItemSelection &deselected) |
virtual void | setSelection (const QRect &rect, QFlags< QItemSelectionModel::SelectionFlag > command) |
virtual int | sizeHintForColumn (int column) const |
virtual void | timerEvent (QTimerEvent *event) |
virtual void | updateGeometries () |
virtual int | verticalOffset () const |
virtual bool | viewportEvent (QEvent *event) |
virtual QRegion | visualRegionForSelection (const QItemSelection &selection) const |
![]() | |
virtual void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
QPoint | dirtyRegionOffset () const |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
DropIndicatorPosition | dropIndicatorPosition () const |
virtual bool | edit (const QModelIndex &index, EditTrigger trigger, QEvent *event) |
virtual void | editorDestroyed (QObject *editor) |
void | executeDelayedItemsLayout () |
virtual void | focusInEvent (QFocusEvent *event) |
virtual bool | focusNextPrevChild (bool next) |
virtual int | horizontalOffset () const =0 |
int | horizontalStepsPerItem () const |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual bool | isIndexHidden (const QModelIndex &index) const =0 |
virtual QModelIndex | moveCursor (CursorAction cursorAction, QFlags< Qt::KeyboardModifier > modifiers)=0 |
virtual void | resizeEvent (QResizeEvent *event) |
void | scheduleDelayedItemsLayout () |
void | scrollDirtyRegion (int dx, int dy) |
virtual QItemSelectionModel::SelectionFlags | selectionCommand (const QModelIndex &index, const QEvent *event) const |
void | setDirtyRegion (const QRegion ®ion) |
void | setHorizontalStepsPerItem (int steps) |
virtual void | setSelection (const QRect &rect, QFlags< QItemSelectionModel::SelectionFlag > flags)=0 |
void | setState (State state) |
void | setVerticalStepsPerItem (int steps) |
virtual void | startDrag (QFlags< Qt::DropAction > supportedActions) |
State | state () const |
virtual int | verticalOffset () const =0 |
int | verticalStepsPerItem () const |
virtual QStyleOptionViewItem | viewOptions () const |
virtual QRegion | visualRegionForSelection (const QItemSelection &selection) const =0 |
![]() | |
void | setupViewport (QWidget *viewport) |
void | setViewportMargins (const QMargins &margins) |
void | setViewportMargins (int left, int top, int right, int bottom) |
virtual void | wheelEvent (QWheelEvent *e) |
![]() | |
virtual void | changeEvent (QEvent *ev) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
![]() | |
QPaintDevice () | |
Static Protected Member Functions | |
static bool | isExpandable (UMLListViewItem::ListViewType lvt) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Friends | |
QDebug | operator<< (QDebug out, const UMLListView &view) |
Detailed Description
This is one of the main classes used in this program.
Information is displayed here in a tree view. No objects are created here. A call to UMLDoc make any additions/deletion or updates to objects. This class will then wait for a signal before updating the tree view.
Displays the list view for the program.
Definition at line 46 of file umllistview.h.
Constructor & Destructor Documentation
|
explicit |
Constructs the tree view.
- Parameters
-
parent The parent to this.
Definition at line 89 of file umllistview.cpp.
UMLListView::~UMLListView | ( | ) |
Standard destructor.
Definition at line 133 of file umllistview.cpp.
Member Function Documentation
|
protected |
Event handler for accepting drag request.
- Parameters
-
event the drop event
- Returns
- success state
Definition at line 1499 of file umllistview.cpp.
|
protected |
Auxiliary method for moveObject(): Adds the model object at the proper new container (package if nested, UMLDoc if at global level), and updates the containment relationships in the model.
Definition at line 1568 of file umllistview.cpp.
void UMLListView::addNewItem | ( | UMLListViewItem * | parentItem, |
UMLListViewItem::ListViewType | type | ||
) |
Adds a new item to the tree of the given type under the given parent.
Method will take care of signalling anyone needed on creation of new item. e.g. UMLDoc if an UMLObject is created.
Definition at line 2156 of file umllistview.cpp.
void UMLListView::changeIconOf | ( | UMLObject * | o, |
Icon_Utils::IconType | to | ||
) |
Changes the icon for the given UMLObject to the given icon.
Definition at line 1284 of file umllistview.cpp.
|
protected |
Adds a new operation, attribute or template item to a classifier, identical to childObjectAdded(obj) but with an explicit parent.
- Parameters
-
child the child object parent the parent object
Definition at line 1092 of file umllistview.cpp.
|
slot |
Adds a new operation, attribute or template item to a classifier.
- Parameters
-
obj the child object
Definition at line 1080 of file umllistview.cpp.
|
slot |
Deletes the list view item.
- Parameters
-
obj the object to remove
Definition at line 1125 of file umllistview.cpp.
void UMLListView::clean | ( | ) |
Remove all items and subfolders of the main folders.
Special case: The datatype folder, child of the logical view, is not deleted.
Definition at line 1414 of file umllistview.cpp.
void UMLListView::closeDatatypesFolder | ( | ) |
Definition at line 2664 of file umllistview.cpp.
|
slot |
Close all items in the list view.
Definition at line 2572 of file umllistview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 2762 of file umllistview.cpp.
|
slot |
Connect some signals into slots in the list view for newly created UMLObjects.
Definition at line 986 of file umllistview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractScrollArea.
Definition at line 2041 of file umllistview.cpp.
UMLListViewItem * UMLListView::createDiagramItem | ( | UMLView * | view | ) |
Create a listview item for an existing diagram.
- Parameters
-
view The existing diagram.
Definition at line 1942 of file umllistview.cpp.
bool UMLListView::createItem | ( | UMLListViewItem * | item | ) |
|
protected |
Deletes all child-items of parent
.
Do it in reverse order, because of the index.
Definition at line 2648 of file umllistview.cpp.
|
protected |
Delete a listview item.
- Parameters
-
temp a non-null UMLListViewItem, for example: (UMLListViewItem*)currentItem()
- Returns
- true if correctly deleted
Definition at line 2674 of file umllistview.cpp.
UMLListViewItem * UMLListView::determineParentItem | ( | UMLObject * | object | ) | const |
Determine the parent ListViewItem given an UMLObject.
- Parameters
-
object Pointer to the UMLObject for which to look up the parent.
- Returns
- Pointer to the parent UMLListViewItem chosen. Returns NULL on error (no parent could be determined.)
Definition at line 849 of file umllistview.cpp.
UMLListViewItem * UMLListView::determineParentItem | ( | UMLListViewItem::ListViewType | lvt | ) | const |
Determine the parent ListViewItem given a ListViewType.
This parent is used for creating new UMLListViewItems.
- Parameters
-
lvt The ListViewType for which to lookup the parent.
- Returns
- Pointer to the parent UMLListViewItem chosen.
Definition at line 1972 of file umllistview.cpp.
UMLDoc * UMLListView::document | ( | ) | const |
Returns the document pointer.
Called by the UMLListViewItem class.
Definition at line 2020 of file umllistview.cpp.
|
protectedvirtual |
Always allow starting a drag.
Reimplemented from QAbstractItemView.
Definition at line 2724 of file umllistview.cpp.
|
protectedvirtual |
Check drag destination and update move/copy action.
Reimplemented from QTreeView.
Definition at line 2732 of file umllistview.cpp.
|
protectedvirtual |
Reimplemented from QTreeWidget.
Definition at line 2746 of file umllistview.cpp.
|
protectedvirtual |
Event handler for the tool tip event.
Works only for operations to show the signature.
Reimplemented from QTreeWidget.
Definition at line 173 of file umllistview.cpp.
|
slot |
Open all items in the list view.
Definition at line 2560 of file umllistview.cpp.
UMLListViewItem * UMLListView::findFolderForDiagram | ( | Uml::DiagramType::Enum | dt | ) |
Find the parent folder for a diagram.
If the currently selected item in the list view is a folder then that folder is returned as the parent.
- Parameters
-
dt The Diagram_Type of the diagram. The type will only be used if there is no currently selected item, or if the current item is not a folder. In that case the root folder which is suitable for the Diagram_Type is returned.
- Returns
- Pointer to the parent UMLListViewItem for the diagram.
Definition at line 790 of file umllistview.cpp.
UMLListViewItem * UMLListView::findItem | ( | Uml::ID::Type | id | ) |
Searches through the tree for the item with the given ID.
- Parameters
-
id The ID to search for.
- Returns
- The item with the given ID or 0 if not found.
Definition at line 1362 of file umllistview.cpp.
UMLListViewItem * UMLListView::findUMLObject | ( | const UMLObject * | p | ) | const |
Find an UMLObject in the listview.
- Parameters
-
p Pointer to the object to find in the list view.
- Returns
- Pointer to the UMLObject found or NULL if not found.
Definition at line 1270 of file umllistview.cpp.
|
protected |
This methods looks for a object in a folder an its subfolders recursive.
- Parameters
-
folder The folder entry of the list view. obj The object to be found in the folder.
- Returns
- The object if found else a NULL pointer.
Definition at line 1225 of file umllistview.cpp.
UMLListViewItem * UMLListView::findView | ( | UMLView * | v | ) |
Searches through the tree for the item which represents the diagram given.
- Parameters
-
v the diagram to search for
- Returns
- the item which represents the diagram
Definition at line 1296 of file umllistview.cpp.
|
protectedvirtual |
Event handler for lost focus.
- Parameters
-
fe the focus event
Reimplemented from QAbstractItemView.
Definition at line 2029 of file umllistview.cpp.
|
protected |
Definition at line 1200 of file umllistview.cpp.
void UMLListView::init | ( | ) |
Carries out initalisation of attributes in class.
This method is called more than once during an instance's lifetime (by UMLDoc)! So we must not allocate any memory before freeing the previously allocated one or do connect()s.
Definition at line 1377 of file umllistview.cpp.
|
staticprotected |
Return true if the given list view type can be expanded/collapsed.
Definition at line 2088 of file umllistview.cpp.
bool UMLListView::isUnique | ( | UMLListViewItem * | item, |
const QString & | name | ||
) |
Returns if the given name is unique for the given items type.
Definition at line 2214 of file umllistview.cpp.
|
protectedvirtual |
Handler for key press events.
- Parameters
-
ke the key event
Reimplemented from QTreeView.
Definition at line 294 of file umllistview.cpp.
bool UMLListView::loadChildrenFromXMI | ( | UMLListViewItem * | parent, |
QDomElement & | element | ||
) |
Definition at line 2350 of file umllistview.cpp.
bool UMLListView::loadFromXMI | ( | QDomElement & | element | ) |
Definition at line 2323 of file umllistview.cpp.
|
static |
Return true if the given ObjectType permits child items.
A "child item" is anything that qualifies as a UMLClassifierListItem, e.g. operations and attributes of classifiers.
Definition at line 906 of file umllistview.cpp.
|
protectedvirtual |
Event handler for mouse double click.
Reimplemented from QTreeView.
Definition at line 1439 of file umllistview.cpp.
|
protectedvirtual |
Handler for mouse move events.
- Parameters
-
me the mouse event
Reimplemented from QTreeView.
Definition at line 236 of file umllistview.cpp.
|
protectedvirtual |
Handler for mouse press events.
- Parameters
-
me the mouse event
Reimplemented from QTreeView.
Definition at line 193 of file umllistview.cpp.
|
protectedvirtual |
Handler for mouse release event.
- Parameters
-
me the mouse event
Reimplemented from QTreeView.
Definition at line 270 of file umllistview.cpp.
UMLListViewItem * UMLListView::moveObject | ( | Uml::ID::Type | srcId, |
UMLListViewItem::ListViewType | srcType, | ||
UMLListViewItem * | newParent | ||
) |
Moves an object given is unique ID and listview type to an other listview parent item.
Also takes care of the corresponding move in the model.
Definition at line 1597 of file umllistview.cpp.
UMLListViewItem * UMLListView::rootView | ( | UMLListViewItem::ListViewType | type | ) |
Returns the corresponding view if the listview type is one of the root views, Root/Logical/UseCase/Component/Deployment/EntityRelation View.
Definition at line 2613 of file umllistview.cpp.
UMLListViewItem::ListViewType UMLListView::rootViewType | ( | UMLListViewItem * | item | ) |
Determines the root listview type of the given UMLListViewItem.
Starts at the given item, compares it against each of the predefined root views (Root, Logical, UseCase, Component, Deployment, EntityRelationship.) Returns the ListViewType of the matching root view; if no match then continues the search using the item's parent, then grandparent, and so forth. Returns UMLListViewItem::lvt_Unknown if no match at all is found.
Definition at line 2065 of file umllistview.cpp.
void UMLListView::saveToXMI | ( | QDomDocument & | qDoc, |
QDomElement & | qElement | ||
) |
Definition at line 2313 of file umllistview.cpp.
UMLListViewItemList UMLListView::selectedItems | ( | ) |
Get selected items.
- Returns
- the list of selected items
Definition at line 1896 of file umllistview.cpp.
int UMLListView::selectedItemsCount | ( | ) |
Return the amount of items selected.
Definition at line 2011 of file umllistview.cpp.
UMLListViewItemList UMLListView::selectedItemsRoot | ( | ) |
Get selected items, but only root elements selected (without children).
- Returns
- the list of selected root items
Definition at line 1916 of file umllistview.cpp.
void UMLListView::setDocument | ( | UMLDoc * | doc | ) |
Sets the document this is associated with.
This is important as this is required as to set up the callbacks.
- Parameters
-
doc The document to associate with this class.
Definition at line 1157 of file umllistview.cpp.
void UMLListView::setStartedCopy | ( | bool | startedCopy | ) |
Set the variable m_bStartedCopy.
NB: While m_bStartedCut is reset as soon as the Cut operation is done, the variable m_bStartedCopy is reset much later - upon pasting.
Definition at line 2596 of file umllistview.cpp.
void UMLListView::setStartedCut | ( | bool | startedCut | ) |
Set the variable m_bStartedCut to indicate that selection should be deleted in slotCutSuccessful().
Definition at line 2586 of file umllistview.cpp.
void UMLListView::setTitle | ( | int | column, |
const QString & | text | ||
) |
Sets the title.
- Parameters
-
column column in which to write text the text to write
Definition at line 143 of file umllistview.cpp.
void UMLListView::setView | ( | UMLView * | view | ) |
Set the current view to the given view.
- Parameters
-
view The current view.
Definition at line 1427 of file umllistview.cpp.
|
protectedslot |
Calls updateFolder() on the item to update the icon to closed.
Definition at line 2118 of file umllistview.cpp.
|
slot |
Connects to the signal that UMLApp emits when a cut operation is successful.
Definition at line 2130 of file umllistview.cpp.
|
slot |
Delete every selected item.
Definition at line 2143 of file umllistview.cpp.
|
slot |
Creates a new item to represent a new diagram.
- Parameters
-
id the id of the new diagram
Definition at line 821 of file umllistview.cpp.
|
slot |
Removes the item representing a diagram.
- Parameters
-
id the id of the diagram
Definition at line 1190 of file umllistview.cpp.
|
slot |
Renames a diagram in the list view.
- Parameters
-
id the id of the renamed diagram
Definition at line 1140 of file umllistview.cpp.
|
slot |
Something has been dragged and dropped onto the list view.
Definition at line 1862 of file umllistview.cpp.
|
protectedslot |
Calls updateFolder() on the item to update the icon to open.
Definition at line 2107 of file umllistview.cpp.
|
protectedslot |
Handler for item selection changed signals.
Definition at line 151 of file umllistview.cpp.
Called when a right mouse button menu has an item selected.
- Parameters
-
action the selected action position the position of the menu on the diagram (only used for multi selection "Show")
Definition at line 314 of file umllistview.cpp.
|
slot |
Calls updateObject() on the item representing the sending object no parameters, uses sender() to work out which object called the slot.
Definition at line 1064 of file umllistview.cpp.
|
slot |
Creates a new list view item and connects the appropriate signals/slots.
- Parameters
-
object the newly created object
Definition at line 926 of file umllistview.cpp.
|
slot |
Disconnects signals and removes the list view item.
- Parameters
-
object the object about to be removed
Definition at line 1175 of file umllistview.cpp.
bool UMLListView::startedCopy | ( | ) | const |
Return the variable m_bStartedCopy.
Definition at line 2604 of file umllistview.cpp.
|
inline |
Definition at line 102 of file umllistview.h.
|
inline |
Definition at line 104 of file umllistview.h.
|
inline |
Definition at line 103 of file umllistview.h.
|
inline |
Definition at line 100 of file umllistview.h.
|
inline |
Definition at line 99 of file umllistview.h.
|
inline |
Definition at line 101 of file umllistview.h.
Friends And Related Function Documentation
|
friend |
Overloading operator for debugging output.
Definition at line 2804 of file umllistview.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.