umbrello/umbrello
#include <classifier.h>
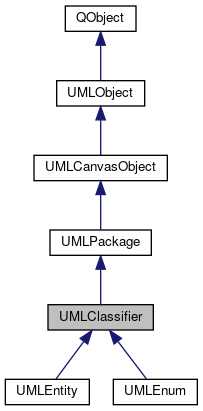
Public Types | |
enum | ClassifierType { ALL = 0, CLASS, INTERFACE, DATATYPE } |
![]() | |
enum | ObjectType { ot_Unknown = -1, ot_UMLObject = 100, ot_Actor, ot_UseCase, ot_Package, ot_Interface, ot_Datatype, ot_Enum, ot_Class, ot_Association, ot_Attribute, ot_Operation, ot_EnumLiteral, ot_Template, ot_Component, ot_Artifact, ot_Node, ot_Stereotype, ot_Role, ot_Entity, ot_EntityAttribute, ot_Folder, ot_EntityConstraint, ot_UniqueConstraint, ot_ForeignKeyConstraint, ot_CheckConstraint, ot_Category, ot_Port } |
Signals | |
void | attributeAdded (UMLClassifierListItem *) |
void | attributeRemoved (UMLClassifierListItem *) |
void | operationAdded (UMLClassifierListItem *) |
void | operationRemoved (UMLClassifierListItem *) |
void | templateAdded (UMLClassifierListItem *) |
void | templateRemoved (UMLClassifierListItem *) |
![]() | |
void | sigAssociationEndAdded (UMLAssociation *assoc) |
void | sigAssociationEndRemoved (UMLAssociation *assoc) |
![]() | |
void | modified () |
Protected Member Functions | |
virtual bool | load (QDomElement &element) |
void | saveToXMI (QDomDocument &qDoc, QDomElement &qElement) |
![]() | |
void | init () |
void | maybeSignalObjectCreated () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
void | emitModified () |
![]() | |
static QString | toString (ObjectType ot) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
UMLObjectList | m_objects |
![]() | |
UMLObjectList | m_List |
![]() | |
bool | m_bAbstract |
ObjectType | m_BaseType |
bool | m_bCreationWasSignalled |
bool | m_bInPaste |
bool | m_bStatic |
QString | m_Doc |
QString | m_name |
Uml::ID::Type | m_nId |
UMLObject * | m_pSecondary |
UMLStereotype * | m_pStereotype |
UMLPackage * | m_pUMLPackage |
QString | m_SecondaryFallback |
QString | m_SecondaryId |
Uml::Visibility::Enum | m_visibility |
![]() | |
objectName | |
Detailed Description
This class defines the non-graphical information required for a UML Classifier (ie a class or interface).
This class inherits from UMLPackage which allows classifiers to also act as namespaces, i.e. it allows classifiers to nest.
NOTE: There is a unit test available for this class. Please, use and adapt it when necessary.
Information for a non-graphical Concept/Class.
Definition at line 39 of file classifier.h.
Member Enumeration Documentation
Enumeration identifying the type of classifier.
Enumerator | |
---|---|
ALL | |
CLASS | |
INTERFACE | |
DATATYPE |
Definition at line 47 of file classifier.h.
Constructor & Destructor Documentation
|
explicit |
Sets up a Classifier.
- Parameters
-
name The name of the Concept. id The unique id of the Concept.
Definition at line 62 of file classifier.cpp.
|
virtual |
Standard deconstructor.
Definition at line 73 of file classifier.cpp.
Member Function Documentation
|
virtual |
Reimplemented from UMLObject.
Reimplemented from UMLObject.
Definition at line 734 of file classifier.cpp.
UMLAttribute * UMLClassifier::addAttribute | ( | const QString & | name, |
Uml::ID::Type | id = Uml::ID::None |
||
) |
Creates and adds an attribute for the class.
- Parameters
-
name an optional name, used by when creating through UMLListView id an optional id
- Returns
- the UMLAttribute created and added
Definition at line 818 of file classifier.cpp.
UMLAttribute * UMLClassifier::addAttribute | ( | const QString & | name, |
UMLObject * | type, | ||
Uml::Visibility::Enum | scope | ||
) |
Adds an already created attribute.
The attribute object must not belong to any other concept.
- Parameters
-
name the name of the attribute type the type of the attribute scope the visibility of the attribute
- Returns
- the just created and added attribute
Definition at line 843 of file classifier.cpp.
bool UMLClassifier::addAttribute | ( | UMLAttribute * | att, |
IDChangeLog * | log = 0 , |
||
int | position = -1 |
||
) |
Adds an already created attribute.
The attribute object must not belong to any other concept.
- Parameters
-
att Pointer to the UMLAttribute. log Pointer to the IDChangeLog (optional.) position Position index for the insertion (optional.) If the position is omitted, or if it is negative or too large, the attribute is added to the end of the list.
- Returns
- True if the attribute was successfully added.
Definition at line 871 of file classifier.cpp.
bool UMLClassifier::addOperation | ( | UMLOperation * | op, |
int | position = -1 |
||
) |
Appends an operation to the classifier.
This function is mainly intended for the clipboard.
- Parameters
-
op Pointer to the UMLOperation to add. position Inserted at the given position.
- Returns
- True if the operation was added successfully.
Definition at line 312 of file classifier.cpp.
bool UMLClassifier::addOperation | ( | UMLOperation * | op, |
IDChangeLog * | log | ||
) |
Appends an operation to the classifier.
- See also
- bool addOperation(UMLOperation* Op, int position = -1) This function is mainly intended for the clipboard.
- Parameters
-
op Pointer to the UMLOperation to add. log Pointer to the IDChangeLog.
- Returns
- True if the operation was added successfully.
Definition at line 352 of file classifier.cpp.
UMLTemplate * UMLClassifier::addTemplate | ( | const QString & | name, |
Uml::ID::Type | id = Uml::ID::None |
||
) |
Adds an already created template.
The template object must not belong to any other concept.
- Parameters
-
name the name of the template id the id of the template
- Returns
- the added template
Definition at line 1051 of file classifier.cpp.
bool UMLClassifier::addTemplate | ( | UMLTemplate * | newTemplate, |
IDChangeLog * | log = 0 |
||
) |
Adds an already created template.
The template object must not belong to any other concept.
- Parameters
-
newTemplate Pointer to the UMLTemplate object to add. log Pointer to the IDChangeLog.
- Returns
- True if the template was successfully added.
Definition at line 1073 of file classifier.cpp.
bool UMLClassifier::addTemplate | ( | UMLTemplate * | templt, |
int | position | ||
) |
Adds an template to the class.
The template object must not belong to any other class. TODO: If the param IDChangeLog from the method above is not being used, give position a default value of -1 and the method can replace the above one.
- Parameters
-
templt Pointer to the UMLTemplate to add. position The position of the template in the list. A value of -1 will add the template at the end.
- Returns
- True if the template was successfully added.
Definition at line 1101 of file classifier.cpp.
|
signal |
|
signal |
int UMLClassifier::attributes | ( | ) |
Returns the number of attributes for the class.
- Returns
- The number of attributes for the class.
Definition at line 439 of file classifier.cpp.
UMLOperation * UMLClassifier::checkOperationSignature | ( | const QString & | name, |
UMLAttributeList | opParams, | ||
UMLOperation * | exemptOp = NULL |
||
) |
Checks whether an operation is valid based on its signature - An operation is "valid" if the operation's name and parameter list are unique in the classifier.
- Parameters
-
name Name of the operation to check. opParams The operation's argument list. exemptOp Pointer to the exempt method (optional.)
- Returns
- NULL if the signature is valid (ok), else return a pointer to the existing UMLOperation that causes the conflict.
Definition at line 141 of file classifier.cpp.
|
virtual |
Make a clone of this object.
Reimplemented from UMLPackage.
Reimplemented in UMLEntity, and UMLEnum.
Definition at line 685 of file classifier.cpp.
|
virtual |
Copy the internal presentation of this object into the new object.
Reimplemented from UMLPackage.
Reimplemented in UMLEntity, and UMLEnum.
Definition at line 673 of file classifier.cpp.
|
virtual |
Creates an attribute for the class.
- Parameters
-
name An optional name, used by when creating through UMLListView type An optional type, used by when creating through UMLListView vis An optional visibility, used by when creating through UMLListView init An optional initial value, used by when creating through UMLListView
- Returns
- The UMLAttribute created
Reimplemented in UMLEntity.
Definition at line 764 of file classifier.cpp.
UMLOperation * UMLClassifier::createOperation | ( | const QString & | name = QString() , |
bool * | isExistingOp = NULL , |
||
Model_Utils::NameAndType_List * | params = NULL |
||
) |
Creates an operation in the current document.
The new operation is initialized with name, id, etc. If a method with the given profile already exists in the classifier, no new method is created and the existing operation is returned. If no name is provided, or if the params are NULL, an Operation Dialog is shown to ask the user for a name and parameters. The operation's signature is checked for validity within the parent classifier.
- Parameters
-
name The operation name (will be chosen internally if none given.) isExistingOp Optional pointer to bool. If supplied, the bool is set to true if an existing operation is returned. params Optional list of parameter names and types. If supplied, new operation parameters are constructed using this list.
- Returns
- The new operation, or NULL if the operation could not be created because for example, the user canceled the dialog or no appropriate name can be found.
Definition at line 243 of file classifier.cpp.
Create and add a just created template.
- Parameters
-
currentName the name of the template
- Returns
- the template or NULL
Definition at line 395 of file classifier.cpp.
|
virtual |
Find the child object by the given id.
- Parameters
-
id the id of the child object considerAncestors flag whether the ancestors should be considered during search
- Returns
- the found child object or NULL
Reimplemented from UMLCanvasObject.
Definition at line 561 of file classifier.cpp.
UMLOperation * UMLClassifier::findOperation | ( | const QString & | name, |
Model_Utils::NameAndType_List | params | ||
) |
Find an operation of the given name and parameter signature.
- Parameters
-
name The name of the operation to find. params The parameter descriptors of the operation to find.
- Returns
- The operation found. Will return 0 if none found.
Definition at line 186 of file classifier.cpp.
UMLOperationList UMLClassifier::findOperations | ( | const QString & | n | ) |
Find a list of operations with the given name.
- Parameters
-
n The name of the operation to find.
- Returns
- The list of objects found; will be empty if none found.
Definition at line 536 of file classifier.cpp.
UMLClassifierList UMLClassifier::findSubClassConcepts | ( | ClassifierType | type = ALL | ) |
Returns a list of concepts which inherit from this concept.
- Parameters
-
type The ClassifierType to seek.
- Returns
- List of UMLClassifiers that inherit from us.
Definition at line 585 of file classifier.cpp.
UMLClassifierList UMLClassifier::findSuperClassConcepts | ( | ClassifierType | type = ALL | ) |
Returns a list of concepts which this concept inherits from.
- Parameters
-
type The ClassifierType to seek.
- Returns
- List of UMLClassifiers we inherit from.
Definition at line 624 of file classifier.cpp.
UMLTemplate * UMLClassifier::findTemplate | ( | const QString & | name | ) |
Seeks the template parameter of the given name.
- Parameters
-
name the template name
- Returns
- the found template or 0
Definition at line 1145 of file classifier.cpp.
UMLAttributeList UMLClassifier::getAttributeList | ( | ) | const |
Returns the attributes for the specified scope.
- Returns
- List of true attributes for the class.
Definition at line 449 of file classifier.cpp.
UMLAttributeList UMLClassifier::getAttributeList | ( | Uml::Visibility::Enum | scope | ) | const |
Returns the attributes for the specified scope.
- Parameters
-
scope The scope of the attribute.
- Returns
- List of true attributes for the class.
Definition at line 466 of file classifier.cpp.
UMLAttributeList UMLClassifier::getAttributeListStatic | ( | Uml::Visibility::Enum | scope | ) | const |
Returns the static attributes for the specified scope.
- Parameters
-
scope The scope of the attribute.
- Returns
- List of true attributes for the class.
Definition at line 501 of file classifier.cpp.
UMLAssociation * UMLClassifier::getClassAssoc | ( | ) | const |
Returns the UMLAssociation for which this class acts as an association class.
Returns NULL if this class does not act as an association class.
Definition at line 930 of file classifier.cpp.
|
virtual |
Returns the entries in m_List that are of the requested type.
If the requested type is UMLObject::ot_UMLObject then all entries are returned.
- Parameters
-
ot the requested object type
- Returns
- The list of true operations for the Concept.
Reimplemented in UMLEntity.
Definition at line 1027 of file classifier.cpp.
UMLOperationList UMLClassifier::getOpList | ( | bool | includeInherited = false , |
UMLClassifierSet * | alreadyTraversed = 0 |
||
) |
Return a list of operations for the Classifier.
- Parameters
-
includeInherited Includes operations from superclasses. alreadyTraversed internal used object to avoid recursive loops
- Returns
- The list of operations for the Classifier.
Definition at line 968 of file classifier.cpp.
UMLTemplateList UMLClassifier::getTemplateList | ( | ) | const |
Returns the templates.
Same as UMLClassifier::getFilteredList(ot_Template) but return type is a true UMLTemplateList.
- Returns
- Pointer to the list of true templates for the class.
Definition at line 1174 of file classifier.cpp.
|
virtual |
Return the list of unidirectional association that should show up in the code.
Definition at line 1377 of file classifier.cpp.
bool UMLClassifier::hasAbstractOps | ( | ) |
Return true if this classifier has abstract operations.
Definition at line 938 of file classifier.cpp.
bool UMLClassifier::hasAccessorMethods | ( | ) |
Return true if this classifier has accessor methods.
Definition at line 1341 of file classifier.cpp.
bool UMLClassifier::hasAssociations | ( | ) |
Return true if this classifier has associations.
- Returns
- true if classifier has associations
Definition at line 1307 of file classifier.cpp.
bool UMLClassifier::hasAttributes | ( | ) |
Return true if this classifier has attributes.
Definition at line 1318 of file classifier.cpp.
bool UMLClassifier::hasMethods | ( | ) |
Return true if this classifier has methods.
Definition at line 1357 of file classifier.cpp.
bool UMLClassifier::hasOperationMethods | ( | ) |
Return true if this classifier has operation methods.
Definition at line 1349 of file classifier.cpp.
bool UMLClassifier::hasStaticAttributes | ( | ) |
Return true if this classifier has static attributes.
Definition at line 1331 of file classifier.cpp.
bool UMLClassifier::hasVectorFields | ( | ) |
Return true if this classifier has vector fields.
Definition at line 1369 of file classifier.cpp.
bool UMLClassifier::isDatatype | ( | ) | const |
Returns true if this classifier represents a datatype.
Definition at line 125 of file classifier.cpp.
bool UMLClassifier::isInterface | ( | ) | const |
Returns true if this classifier represents an interface.
Definition at line 117 of file classifier.cpp.
bool UMLClassifier::isReference | ( | ) | const |
Get the m_isRef flag.
- Returns
- true if is reference, otherwise false
Definition at line 1298 of file classifier.cpp.
|
protectedvirtual |
Auxiliary to loadFromXMI: The loading of operations is implemented here.
Calls loadSpecialized() for any other tag. Child classes can override the loadSpecialized method to load its additional tags.
Reimplemented from UMLPackage.
Reimplemented in UMLEntity, and UMLEnum.
Definition at line 1525 of file classifier.cpp.
|
virtual |
utility functions to allow easy determination of what classifiers are "owned" by the current one via named association type (e.g.
Create a new ClassifierListObject (attribute, operation, template) according to the given XMI tag.
plain, aggregate or compositions).
Returns NULL if the string given does not contain one of the tags <UML:Attribute>, <UML:Operation>, or <UML:TemplateParameter>. Used by the clipboard for paste operation.
Reimplemented in UMLEntity, and UMLEnum.
Definition at line 1501 of file classifier.cpp.
|
signal |
|
signal |
int UMLClassifier::operations | ( | ) |
Counts the number of operations in the Classifier.
- Returns
- The number of operations for the Classifier.
Definition at line 955 of file classifier.cpp.
bool UMLClassifier::operator== | ( | const UMLClassifier & | rhs | ) | const |
Overloaded '==' operator.
Definition at line 656 of file classifier.cpp.
UMLClassifier * UMLClassifier::originType | ( | ) | const |
Get the origin type (in case of e.g.
typedef)
- Returns
- the origin type
Definition at line 1280 of file classifier.cpp.
int UMLClassifier::removeAttribute | ( | UMLAttribute * | att | ) |
Removes an attribute from the class.
- Parameters
-
att The attribute to remove.
- Returns
- Count of the remaining attributes after removal. Returns -1 if the given attribute was not found.
Definition at line 901 of file classifier.cpp.
int UMLClassifier::removeOperation | ( | UMLOperation * | op | ) |
Remove an operation from the Classifier.
The operation is not deleted so the caller is responsible for what happens to it after this.
- Parameters
-
op The operation to remove.
- Returns
- Count of the remaining operations after removal, or -1 if the given operation was not found.
Definition at line 372 of file classifier.cpp.
int UMLClassifier::removeTemplate | ( | UMLTemplate * | umltemplate | ) |
Removes a template from the class.
- Parameters
-
umltemplate The template to remove.
- Returns
- Count of the remaining templates after removal. Returns -1 if the given template was not found.
Definition at line 1128 of file classifier.cpp.
|
virtual |
Needs to be called after all UML objects are loaded from file.
Calls the parent resolveRef(), and calls resolveRef() on all UMLClassifierListItems. Overrides the method from UMLObject.
- Returns
- true for success.
Reimplemented from UMLPackage.
Reimplemented in UMLEntity.
Definition at line 700 of file classifier.cpp.
|
protectedvirtual |
Utility method called by "get*ChildClassfierList()" methods.
Creates XML tag <UML:Class>, <UML:Interface>, or <UML:DataType> depending on m_BaseType.
It basically finds all the classifiers named in each association in the given association list which aren't the current one. Useful for finding which classifiers are "owned" by the current one via declared associations such as in aggregations/compositions.
Saves possible template parameters, generalizations, attributes, operations, and contained objects to the given QDomElement.
Reimplemented from UMLPackage.
Reimplemented in UMLEntity, and UMLEnum.
Definition at line 1413 of file classifier.cpp.
|
virtual |
Reimplementation of method from class UMLObject for controlling the exact type of this classifier: class, interface, or datatype.
- Parameters
-
ot the base type to set
Reimplemented from UMLObject.
Definition at line 82 of file classifier.cpp.
void UMLClassifier::setClassAssoc | ( | UMLAssociation * | assoc | ) |
Sets the UMLAssociation for which this class shall act as an association class.
Definition at line 920 of file classifier.cpp.
void UMLClassifier::setIsReference | ( | bool | isRef = true | ) |
Set the m_isRef flag (true when dealing with a pointer type)
- Parameters
-
isRef the flag to set
Definition at line 1289 of file classifier.cpp.
void UMLClassifier::setOriginType | ( | UMLClassifier * | origType | ) |
Set the origin type (in case of e.g.
typedef)
- Parameters
-
origType the origin type to set
Definition at line 1271 of file classifier.cpp.
int UMLClassifier::takeItem | ( | UMLClassifierListItem * | item | ) |
Take and return a subordinate item from this classifier.
Ownership of the item is passed to the caller.
- Parameters
-
item Subordinate item to take.
- Returns
- Index in m_List of the item taken. Return -1 if the item is not found in m_List.
Definition at line 1194 of file classifier.cpp.
|
signal |
|
signal |
int UMLClassifier::templates | ( | ) |
Returns the number of templates for the class.
- Returns
- The number of templates for the class.
Definition at line 1161 of file classifier.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.