umbrello/umbrello
#include <template.h>
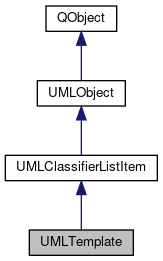
Public Member Functions | |
UMLTemplate (UMLObject *parent, const QString &name, Uml::ID::Type id=Uml::ID::None, const QString &type=QLatin1String("class")) | |
UMLTemplate (UMLObject *parent) | |
virtual | ~UMLTemplate () |
virtual UMLObject * | clone () const |
virtual void | copyInto (UMLObject *lhs) const |
virtual QString | getTypeName () const |
bool | operator== (const UMLTemplate &rhs) const |
void | saveToXMI (QDomDocument &qDoc, QDomElement &qElement) |
bool | showPropertiesDialog (QWidget *parent) |
QString | toString (Uml::SignatureType::Enum sig=Uml::SignatureType::NoSig) |
![]() | |
UMLClassifierListItem (UMLObject *parent, const QString &name, Uml::ID::Type id=Uml::ID::None) | |
UMLClassifierListItem (UMLObject *parent) | |
virtual | ~UMLClassifierListItem () |
UMLClassifier * | getType () const |
virtual void | setType (UMLObject *type) |
void | setTypeName (const QString &type) |
![]() | |
UMLObject (UMLObject *parent, const QString &name, Uml::ID::Type id=Uml::ID::None) | |
UMLObject (UMLObject *parent) | |
UMLObject (const QString &name=QString(), Uml::ID::Type id=Uml::ID::None) | |
virtual | ~UMLObject () |
virtual bool | acceptAssociationType (Uml::AssociationType::Enum) |
ObjectType | baseType () const |
QLatin1String | baseTypeStr () const |
QString | doc () const |
virtual QString | fullyQualifiedName (const QString &separator=QString(), bool includeRoot=false) const |
bool | hasDoc () const |
virtual Uml::ID::Type | id () const |
bool | isAbstract () const |
bool | isStatic () const |
virtual bool | loadFromXMI (QDomElement &element) |
bool | loadStereotype (QDomElement &element) |
QString | name () const |
bool | operator== (const UMLObject &rhs) const |
QString | package (const QString &separator=QString(), bool includeRoot=false) |
UMLPackageList | packages (bool includeRoot=false) const |
virtual bool | resolveRef () |
QDomElement | save (const QString &tag, QDomDocument &qDoc) |
QString | secondaryFallback () const |
QString | secondaryId () const |
void | setAbstract (bool bAbstract) |
virtual void | setBaseType (ObjectType ot) |
void | setDoc (const QString &d) |
virtual void | setID (Uml::ID::Type NewID) |
virtual void | setName (const QString &strName) |
void | setNameCmd (const QString &strName) |
void | setPackage (const QString &_name) |
void | setSecondaryFallback (const QString &id) |
void | setSecondaryId (const QString &id) |
void | setStatic (bool bStatic) |
void | setStereotype (const QString &_name) |
void | setStereotypeCmd (const QString &_name) |
bool | setUMLPackage (UMLPackage *pPkg) |
void | setUMLStereotype (UMLStereotype *stereo) |
void | setVisibility (Uml::Visibility::Enum visibility) |
void | setVisibilityCmd (Uml::Visibility::Enum visibility) |
virtual bool | showPropertiesPagedDialog (int page=0, bool assoc=false) |
QString | stereotype (bool includeAdornments=false) const |
UMLPackage * | umlPackage () |
const UMLStereotype * | umlStereotype () |
Uml::Visibility::Enum | visibility () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
bool | load (QDomElement &element) |
![]() | |
void | init () |
void | maybeSignalObjectCreated () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | ObjectType { ot_Unknown = -1, ot_UMLObject = 100, ot_Actor, ot_UseCase, ot_Package, ot_Interface, ot_Datatype, ot_Enum, ot_Class, ot_Association, ot_Attribute, ot_Operation, ot_EnumLiteral, ot_Template, ot_Component, ot_Artifact, ot_Node, ot_Stereotype, ot_Role, ot_Entity, ot_EntityAttribute, ot_Folder, ot_EntityConstraint, ot_UniqueConstraint, ot_ForeignKeyConstraint, ot_CheckConstraint, ot_Category, ot_Port } |
![]() | |
void | emitModified () |
![]() | |
void | modified () |
![]() | |
static QString | toString (ObjectType ot) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
bool | m_bAbstract |
ObjectType | m_BaseType |
bool | m_bCreationWasSignalled |
bool | m_bInPaste |
bool | m_bStatic |
QString | m_Doc |
QString | m_name |
Uml::ID::Type | m_nId |
UMLObject * | m_pSecondary |
UMLStereotype * | m_pStereotype |
UMLPackage * | m_pUMLPackage |
QString | m_SecondaryFallback |
QString | m_SecondaryId |
Uml::Visibility::Enum | m_visibility |
![]() | |
objectName | |
Detailed Description
This class holds information used by template classes, called paramaterised class in UML and a generic in Java.
It has a type (usually just "class") and name.
Sets up template information.
- See also
- UMLObject Bugs and comments to umbre or llo- devel @kde .orghttp://bugs.kde.org
Definition at line 26 of file template.h.
Constructor & Destructor Documentation
UMLTemplate::UMLTemplate | ( | UMLObject * | parent, |
const QString & | name, | ||
Uml::ID::Type | id = Uml::ID::None , |
||
const QString & | type = QLatin1String("class") |
||
) |
Sets up a template.
- Parameters
-
parent The parent of this UMLTemplate (i.e. its concept). name The name of this UMLTemplate. id The unique id given to this UMLTemplate. type The type of this UMLTemplate.
Definition at line 28 of file template.cpp.
|
explicit |
Sets up a template.
- Parameters
-
parent The parent of this UMLTemplate (i.e. its concept).
Definition at line 41 of file template.cpp.
|
virtual |
Destructor.
Definition at line 50 of file template.cpp.
Member Function Documentation
|
virtual |
Make a clone of this object.
Implements UMLClassifierListItem.
Definition at line 108 of file template.cpp.
|
virtual |
Copy the internal presentation of this object into the new object.
Reimplemented from UMLClassifierListItem.
Definition at line 100 of file template.cpp.
|
virtual |
Overrides method from UMLClassifierListItem.
Returns the type name of the UMLTemplate. If the template parameter is a class, there is no separate type object. In this case, getTypeName() returns "class".
- Returns
- The type name of the UMLClassifierListItem.
Reimplemented from UMLClassifierListItem.
Definition at line 72 of file template.cpp.
|
protectedvirtual |
Loads the <UML:TemplateParameter> XMI element.
Reimplemented from UMLObject.
Definition at line 131 of file template.cpp.
bool UMLTemplate::operator== | ( | const UMLTemplate & | rhs | ) | const |
Overloaded '==' operator.
Definition at line 82 of file template.cpp.
|
virtual |
Writes the <UML:TemplateParameter> XMI element.
Implements UMLObject.
Definition at line 119 of file template.cpp.
|
virtual |
Display the properties configuration dialog for the template.
- Returns
- Success status.
Implements UMLClassifierListItem.
Definition at line 142 of file template.cpp.
|
virtual |
Returns a string representation of the list item.
- Parameters
-
sig What type of operation string to show.
- Returns
- The string representation of the operation.
Reimplemented from UMLClassifierListItem.
Definition at line 54 of file template.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:40:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.