KGameSound
#include <KGameSound>
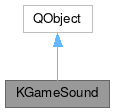
Public Types | |
enum | PlaybackType { AmbientPlayback = 1 , AbsolutePlayback , RelativePlayback } |
Properties | |
KGameSound::PlaybackType | playbackType |
QPointF | pos |
qreal | volume |
![]() | |
objectName | |
Signals | |
void | playbackTypeChanged (KGameSound::PlaybackType type) |
void | posChanged (QPointF pos) |
void | volumeChanged (qreal volume) |
Public Slots | |
void | start () |
void | start (QPointF pos) |
void | stop () |
Public Member Functions | |
KGameSound (const QString &file, QObject *parent=nullptr) | |
~KGameSound () override | |
bool | hasError () const |
bool | isValid () const |
KGameSound::PlaybackType | playbackType () const |
QPointF | pos () const |
void | setPlaybackType (KGameSound::PlaybackType type) |
void | setPos (QPointF pos) |
void | setVolume (qreal volume) |
qreal | volume () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class models a sound file.
Because it is implicitly added to this application's KGameAudioScene, it can be played at different positions (if positional playback is supported, see KGameAudioScene::capabilities()).
Compared to many other media playback classes, the notable difference of KGameSound is that one sound instance can be played multiple times at the same point in time, by calling start() multiple times (possibly with different playback positions). This behavior can be suppressed by calling stop() before start().
- Note
- WAV files and Ogg/Vorbis files are guaranteed to work. Other audio files may also work, depending on the KGameAudio backend and its configuration.
Definition at line 37 of file kgamesound.h.
Member Enumeration Documentation
◆ PlaybackType
This enumeration describes how a sound can be played back.
Definition at line 47 of file kgamesound.h.
Property Documentation
◆ playbackType
|
readwrite |
Definition at line 41 of file kgamesound.h.
◆ pos
|
readwrite |
Definition at line 42 of file kgamesound.h.
◆ volume
|
readwrite |
Definition at line 43 of file kgamesound.h.
Constructor & Destructor Documentation
◆ KGameSound()
Loads a new sound from the given file.
Note that this is an expensive operation which you might want to do during application startup. However, you can reuse the same Sound instance for multiple playback events.
Since version 7.2.0 this constructor supports reading files from Qt Resource System, file can be for example ":/sound.ogg".
Definition at line 32 of file kgamesound-openal.cpp.
◆ ~KGameSound()
|
override |
Destroys this KGameSound instance.
Definition at line 95 of file kgamesound-openal.cpp.
Member Function Documentation
◆ hasError()
bool KGameSound::hasError | ( | ) | const |
- Returns
- whether loading or playing this sound failed
See KGameAudioScene::hasError() for why you typically do not need to use this method.
Definition at line 164 of file kgamesound-openal.cpp.
◆ isValid()
bool KGameSound::isValid | ( | ) | const |
- Returns
- whether the sound file could be loaded successfully
Definition at line 106 of file kgamesound-openal.cpp.
◆ playbackType()
KGameSound::PlaybackType KGameSound::playbackType | ( | ) | const |
- Returns
- the playback type for this sound
Definition at line 113 of file kgamesound-openal.cpp.
◆ pos()
QPointF KGameSound::pos | ( | ) | const |
- Returns
- the position of this sound
Definition at line 130 of file kgamesound-openal.cpp.
◆ setPlaybackType()
void KGameSound::setPlaybackType | ( | KGameSound::PlaybackType | type | ) |
Sets the playback type for this sound.
This affects how the sound will be perceived by the listener. The default is AmbientPlayback.
- Note
- Changes to this property will not be propagated to running playbacks of this sound.
- Effective only if positional playback is supported.
Definition at line 120 of file kgamesound-openal.cpp.
◆ setPos()
void KGameSound::setPos | ( | QPointF | pos | ) |
Sets the position of this sound.
It depends on the playbackType() how this is position interpreted. See the KGameSound::PlaybackType enumeration documentation for details.
- Note
- Changes to this property will not be propagated to running playbacks of this sound.
- Effective only if positional playback is supported.
Definition at line 137 of file kgamesound-openal.cpp.
◆ setVolume()
void KGameSound::setVolume | ( | qreal | volume | ) |
Sets the volume of this sound.
The default is 1.0, which means no volume change, compared to the original sound file. 0.0 means that the sound is inaudible.
If you think of the KGameSound as a loudspeaker, the volume which is controlled by this method is what you regulate at its volume control. If positional playback is enabled (see playbackType()), this will not be the actual volume which the listener will perceive, because the playback volume decreases with increasing playback-listener distances.
- Note
- Changes to this property will not be propagated to running playbacks of this sound.
Definition at line 154 of file kgamesound-openal.cpp.
◆ start [1/2]
|
slot |
Starts a new playback instance of this sound.
This will not interrupt running playbacks of the same sound or any other sounds.
Definition at line 171 of file kgamesound-openal.cpp.
◆ start [2/2]
|
slot |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. This overload takes an additional position argument which overrides the sound's pos() property.
- Note
- pos is respected only if positional playback is supported.
Definition at line 178 of file kgamesound-openal.cpp.
◆ stop
|
slot |
Stops any playbacks of this sounds.
Definition at line 194 of file kgamesound-openal.cpp.
◆ volume()
qreal KGameSound::volume | ( | ) | const |
- Returns
- the volume of this sound
Definition at line 147 of file kgamesound-openal.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:56 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.