Marble::PopupItem
#include <PopupItem.h>
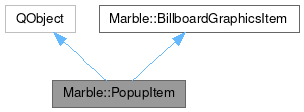
Signals | |
void | hide () |
void | repaintNeeded () |
Public Member Functions | |
PopupItem (QObject *parent=nullptr) | |
void | clearHistory () |
bool | eventFilter (QObject *, QEvent *e) override |
bool | isPrintButtonVisible () const |
void | setBackgroundColor (const QColor &color) |
void | setContent (const QString &html, const QUrl &baseUrl=QUrl()) |
void | setPrintButtonVisible (bool display) |
void | setTextColor (const QColor &color) |
void | setUrl (const QUrl &url) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
Qt::Alignment | alignment () const |
QList< QRectF > | boundingRects () const |
QRectF | containsRect (const QPointF &point) const |
GeoDataCoordinates | coordinate () const |
QList< QPointF > | positions () const |
void | setAlignment (Qt::Alignment alignment) |
void | setCoordinate (const GeoDataCoordinates &coordinates) |
Protected Member Functions | |
void | paint (QPainter *painter) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
The PopupItem Class.
This class represents graphics item for information bubble. Mostly used by
- See also
- MapInfoDialog.
It has nice API for QWebEngineView and methods for styling it.
Definition at line 36 of file PopupItem.h.
Constructor & Destructor Documentation
◆ PopupItem()
|
explicit |
Definition at line 35 of file PopupItem.cpp.
◆ ~PopupItem()
|
override |
Definition at line 82 of file PopupItem.cpp.
Member Function Documentation
◆ clearHistory()
void Marble::PopupItem::clearHistory | ( | ) |
Definition at line 315 of file PopupItem.cpp.
◆ eventFilter()
Reimplemented from QObject.
Definition at line 215 of file PopupItem.cpp.
◆ isPrintButtonVisible()
bool Marble::PopupItem::isPrintButtonVisible | ( | ) | const |
Print button visibility indicator.
There is a button in the header of item with print icon. It used to print the content of QWebEngineView inside. This method indicates visibility of this button.
- See also
- setPrintButtonVisible();
- Returns
- visibility of the print button
Definition at line 87 of file PopupItem.cpp.
◆ paint()
|
overrideprotected |
Definition at line 160 of file PopupItem.cpp.
◆ setBackgroundColor()
void Marble::PopupItem::setBackgroundColor | ( | const QColor & | color | ) |
Sets background color of the bubble.
Frame of the web browser is called bubble. This method sets background color
of this bubble.
- Parameters
-
color background color of the bubble
Definition at line 134 of file PopupItem.cpp.
◆ setContent()
Set content of the popup.
There is a small web browser inside. It can show custom HTML. This method sets custom html
for its window
- Parameters
-
html custom html for popup baseUrl base URL for popup
Definition at line 111 of file PopupItem.cpp.
◆ setPrintButtonVisible()
void Marble::PopupItem::setPrintButtonVisible | ( | bool | display | ) |
Sets visibility of the print button.
There is a button in the header of item with print icon. It used to print the content of QWebEngineView inside
This method sets visibility of this button.
If display
is true
, button will be displayed, otherwise - button won't be displayed
- Parameters
-
display visibility of the print button
Definition at line 92 of file PopupItem.cpp.
◆ setTextColor()
void Marble::PopupItem::setTextColor | ( | const QColor & | color | ) |
Sets text color of the header.
Frame of the web browser is called bubble. Bubble has a header - part of the bubble at the top. Usually it contains the name of the page which can be set via TITLE html tag in HTML document loaded. This method sets text color
of the header.
- Parameters
-
color text color of the header
Definition at line 122 of file PopupItem.cpp.
◆ setUrl()
void Marble::PopupItem::setUrl | ( | const QUrl & | url | ) |
Set URL for web window.
There is a small web browser inside. It can show open websites.
This method sets url
for its window.
- Parameters
-
url new url for web window
Definition at line 97 of file PopupItem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 11 2025 11:47:55 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.