FM
#include <fm.h>
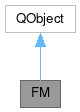
Signals | |
void | cloudItemReady (FMH::MODEL item, QUrl path) |
void | cloudServerContentReady (FMStatic::PATH_CONTENT list) |
void | dirCreated (FMH::MODEL dir) |
void | loadProgress (int percent) |
void | newItem (FMH::MODEL item, QUrl path) |
void | pathContentChanged (QUrl path) |
void | pathContentItemsChanged (QVector< QPair< FMH::MODEL, FMH::MODEL > > items) |
void | pathContentItemsReady (FMStatic::PATH_CONTENT list) |
void | pathContentItemsRemoved (FMStatic::PATH_CONTENT list) |
void | pathContentReady (QUrl path) |
void | warningMessage (QString message) |
Public Slots | |
bool | copy (const QList< QUrl > &urls, const QUrl &where) |
bool | cut (const QList< QUrl > &urls, const QUrl &where) |
void | getCloudItem (const QVariantMap &item) |
void | openCloudItem (const QVariantMap &item) |
Public Member Functions | |
FM (QObject *parent=nullptr) | |
Q_INVOKABLE void | createCloudDir (const QString &path, const QString &name) |
bool | getCloudServerContent (const QUrl &server, const QStringList &filters=QStringList(), const int &depth=0) |
void | getPathContent (const QUrl &path, const bool &hidden=false, const bool &onlyDirs=false, const QStringList &filters=QStringList(), const QDirIterator::IteratorFlags &iteratorFlags=QDirIterator::NoIteratorFlags) |
QString | resolveLocalCloudPath (const QString &path) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | resolveUserCloudCachePath (const QString &server, const QString &user) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The FM class stands for File Management, and exposes methods for file listing, browsing and handling, with syncing and tagging integration if such components were enabled with the build flags COMPONENT_SYNCING
and COMPONENT_TAGGING
.
- Warning
- File syncing support with webDAV cloud providers, such as NextCloud, is still work in progress.
Constructor & Destructor Documentation
◆ FM()
Member Function Documentation
◆ cloudItemReady
|
signal |
Emitted for every single item that becomes available, from the requested remote server location.
- Parameters
-
item the item data path the URL of the remote location initially requested
◆ cloudServerContentReady
|
signal |
Emitted once the requested contents of the server are ready.
- Parameters
-
list the contents packaged in a list, with the file information
◆ copy
◆ createCloudDir()
◆ cut
◆ dirCreated
|
signal |
Emitted when a directory has been created in the remote server in the current location.
- Parameters
-
dir the information of the newly created directory
◆ getCloudItem
|
slot |
◆ getCloudServerContent()
bool FM::getCloudServerContent | ( | const QUrl & | server, |
const QStringList & | filters = QStringList(), | ||
const int & | depth = 0 ) |
Given a server URL address retrieve its contents.
This only works if the syncing component has been enabled with COMPONENT_SYNCING
- See also
- cloudServerContentReady
- Parameters
-
server the server URL filters the list of string filters to be applied depth how deep in the directory three to go, for example, 1
keeps the retrieval in the first level or current directory.
- Returns
- whether the operation was successful.
◆ getPathContent()
void FM::getPathContent | ( | const QUrl & | path, |
const bool & | hidden = false, | ||
const bool & | onlyDirs = false, | ||
const QStringList & | filters = QStringList(), | ||
const QDirIterator::IteratorFlags & | iteratorFlags = QDirIterator::NoIteratorFlags ) |
Given a path URL retrieve the contents information packaged as a model.
This method is asynchronous and once items become ready the signals will be emitted, such as, pathContentItemsReady
or pathContentReady
- Parameters
-
path the directory path hidden whether to pack hidden files onlyDirs whether to only pack directories filters the list of string filters to be applied iteratorFlags the directory iterator flags, for reference check QDirIterator documentation
◆ loadProgress
|
signal |
Emitted with the progress of the listing.
- Parameters
-
percent the progress percent form 0 to 100
◆ newItem
|
signal |
Emitted when a new item is available in the remote server in the current location.
- Parameters
-
item the new item information path the path location of the new item
◆ openCloudItem
|
slot |
Open a given remote item in an external application.
If the item does not exists in the system local cache, then it is downloaded first.
- Parameters
-
item the item data. This is exposed this way for convenience of usage from QML, where the item entry from the model will become a QVariantMap.
◆ pathContentChanged
|
signal |
Emitted when the contents of the current location has changed, either by some new entries being added or removed.
- Parameters
-
path the requested location which has changed
◆ pathContentItemsChanged
|
signal |
Emitted when the current location entries have changed.
- Parameters
-
items the list of pair of entries that have changed, where first is the old version and second is the new version.
◆ pathContentItemsReady
|
signal |
Emitted when a set of entries for the current location are ready.
- Parameters
-
list the contents packaged in a list, with the file information
◆ pathContentItemsRemoved
|
signal |
Emitted when a set of entries in the current location have been removed.
- Parameters
-
list the removed contents packaged in a list, with the file information
◆ pathContentReady
|
signal |
Emitted once the contents of the current location are ready and the listing has finished.
- Parameters
-
path the requested location path
◆ resolveLocalCloudPath()
◆ resolveUserCloudCachePath()
◆ warningMessage
|
signal |
Emitted when there is an error.
- Parameters
-
message the error message
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:53 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.