Tagging
#include <tagging.h>
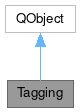
Signals | |
void | tagged (QVariantMap tag) |
void | tagRemoved (QString tag) |
void | urlRemoved (QString url) |
void | urlTagged (QString url, QString tag) |
void | urlTagRemoved (QString tag, QString url) |
Public Slots | |
bool | addTagToUrl (const QString tag, const QUrl &url) |
bool | fav (const QUrl &url) |
const QVariantList | get (const QString &query, std::function< bool(QVariantMap &item)> modifier=nullptr) |
QVariantList | getAllTags (const bool &strict=false) |
FMH::MODEL_LIST | getTags (const int &limit=5) |
QList< QUrl > | getTagUrls (const QString &tag, const QStringList &filters, const bool &strict=false, const int &limit=9999, const QString &mime=QStringLiteral("")) |
QVariantList | getUrls (const QString &tag, const bool &strict=false, const int &limit=MAX_LIMIT, const QString &mimeType=QStringLiteral(""), std::function< bool(QVariantMap &item)> modifier=nullptr) |
QVariantList | getUrlsTags (const bool &strict=false) |
QVariantList | getUrlTags (const QString &url, const bool &strict=false) |
FMH::MODEL_LIST | getUrlTags (const QUrl &url) |
bool | isFav (const QUrl &url, const bool &strict=false) |
bool | removeTag (const QString &tag, const bool &strict=false) |
bool | removeTagToUrl (const QString tag, const QUrl &url) |
bool | removeUrl (const QString &url) |
bool | removeUrlTag (const QString &url, const QString &tag) |
bool | removeUrlTags (const QString &url, const bool &strict=false) |
bool | tag (const QString &tag, const QString &color=QString(), const QString &comment=QString()) |
bool | tagExists (const QString &tag, const bool &strict=false) |
bool | tagUrl (const QString &url, const QString &tag, const QString &color=QString(), const QString &comment=QString()) |
bool | toggleFav (const QUrl &url) |
bool | unFav (const QUrl &url) |
bool | updateUrl (const QString &url, const QString &newUrl) |
bool | updateUrlTags (const QString &url, const QStringList &tags, const bool &strict=false) |
bool | urlTagExists (const QString &url, const QString &tag) |
Static Public Member Functions | |
static Tagging * | getInstance () |
static QObject * | qmlInstance (QQmlEngine *engine, QJSEngine *scriptEngine) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Static Protected Member Functions | |
static bool | setTagIconName (QVariantMap &item) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Tagging class provides quick methods to access and modify the tags associated to the files.
- Note
- This class follows a singleton pattern and it is thread safe, by creating a new instance for each new thread that request access to the singleton. All of the internal instances are self-handled and destroyed when the application quits.
Graphical interfaces are provided which implement most of this class functionality and can be quickly used:
Member Function Documentation
◆ addTagToUrl
Adds a tag to a given file URL.
- Parameters
-
tag the wanted tag, if the tag doesn't exists it is created url the file URL
- Returns
- whether the operation has been successful
Definition at line 400 of file tagging.cpp.
◆ fav
|
slot |
Marks a file URL as favorite.
This works if the tagging component has been enabled, otherwise returns false as default.
- Parameters
-
url the file URL
- Returns
- whether the operation has been successful
Definition at line 418 of file tagging.cpp.
◆ get
|
slot |
Retrieve the information into a model, optionally you can pass a modifier callback function to manipulate or discard items in the model.
- Parameters
-
query the query to be retrieved modifier a callback function that sends as an argument a reference to the current item being retrieved, which can be modified in-place, and expects a boolean value to be returned to decide if such item should de added to the model or not
- Returns
- the resulting model
Definition at line 75 of file tagging.cpp.
◆ getAllTags
|
slot |
Returns a list model of all the tags.
The model can be strictly enforced to only tags that were created by the application making the call
- Parameters
-
strict if true returns only tags created by the application making the request
- Returns
- the model with the info of all the requested tags
Definition at line 224 of file tagging.cpp.
◆ getInstance()
|
static |
◆ getTags
|
slot |
Get all the tags available with detailed information packaged as a FMH::MODEL_LIST.
- Parameters
-
limit the maximum numbers of tags
- Returns
- the model of tags
Definition at line 374 of file tagging.cpp.
◆ getTagUrls
|
slot |
Shortcut for getting a list of file URLs associated to a tag, the resulting list of URLs can be filtered by regular expression or by mime-type and limited.
- Parameters
-
tag the tag to look up filters the regular expressions list strict if strict then the URLs returned are only associated to the application making the call, meaning, that such tag was added by such application only. limit the maximum limit number of URLs to be returned mime the mime-type filtering, for example, "image/\*"
or"image/png"
,"audio/mp4"
- Returns
- the list of file URLs
Definition at line 348 of file tagging.cpp.
◆ getUrls
|
slot |
Returns a model of all the file URLs associated to a tag, the result can be strictly enforced to only file URLs associated to a tag created by the application making the request, restrict it to a maximum limit, filter by the mime-type or just add a modifier function.
- Parameters
-
tag the tag name to perform the search strict strictly enforced to only file URLs associated to the given tag created by the application making the request limit maximum limit of results mimeType filter by mime-type, for example: "image/\*"
or"image/png"
modifier a callback function that sends as an argument a reference to the current item being retrieved, which can be modified, and expects a boolean value to be returned to decide if such item should be added to the model or not
- Returns
- the result model
Definition at line 231 of file tagging.cpp.
◆ getUrlsTags
|
slot |
Give a list of all tags associated to files.
- Parameters
-
strict
- Returns
- whether the operation was successful
Definition at line 210 of file tagging.cpp.
◆ getUrlTags [1/2]
|
slot |
Returns a model list of all the tags associated to a file URL.
The result can be strictly enforced to only tags created by the application making the call
- Parameters
-
url the file URL strict strictly enforced to only tags created by the application making the request
- Returns
- the result model
Definition at line 243 of file tagging.cpp.
◆ getUrlTags [2/2]
|
slot |
Returns a model of tags associated to a file URL.
- Parameters
-
url the file URL
- Returns
- model of the tags
Definition at line 395 of file tagging.cpp.
◆ isFav
|
slot |
Checks if a file URL has been marked as favorite.
This works if the tagging component has been enabled, otherwise returns false as default.
- Parameters
-
url the file URL to be checked strict strictly check if the file has been marked as favorite by the app making the request or not
- Returns
Definition at line 428 of file tagging.cpp.
◆ qmlInstance()
|
inlinestatic |
◆ removeTag
|
slot |
Remove a tag.
Definition at line 293 of file tagging.cpp.
◆ removeTagToUrl
Removes a tag from a file URL if the tags exists.
- Parameters
-
tag the lookup tag url the file URL
- Returns
- whether the operation has been successful
Definition at line 405 of file tagging.cpp.
◆ removeUrl
|
slot |
Removes a URL with its associated tags.
- Parameters
-
url the file URL
- Returns
- whether the operation was successful
Definition at line 270 of file tagging.cpp.
◆ removeUrlTag
Removes a given tag associated to a given file URL.
- Parameters
-
url file URL tag tag associated to file URL to be removed
- Returns
- whether the operation was successful
Definition at line 257 of file tagging.cpp.
◆ removeUrlTags
|
slot |
Given a file URL remove all the tags associated to it.
- Parameters
-
url the file URL
- Returns
- whether the operation was successful
Definition at line 251 of file tagging.cpp.
◆ setTagIconName()
|
staticprotected |
Definition at line 218 of file tagging.cpp.
◆ tag
|
slot |
Adds a new tag, the newly created tag gets associated to the app making the call.
If the tag already exists nothing is changed. If the tag exists the app making the request will get associated to the tag too
- Parameters
-
tag the name of the tag color optional color for the tag comment optional comment for the tag
- Returns
- whether the operation was successful, meaning the tag was created
Definition at line 131 of file tagging.cpp.
◆ tagExists
|
slot |
Checks if a given tag exists, it can be strictly enforced, meaning it is checked if the tag was created by the application making the request.
- Parameters
-
tag the tag to search strict whether the search should be strictly enforced. If strict is true then the tag should have been created by the app making the request, otherwise checks if the tag exists and could have been created by any other application.
- Returns
Definition at line 111 of file tagging.cpp.
◆ tagged
|
signal |
Emitted when a new tag has been created.
- Parameters
-
tag contains information about the new tag, such as color, add date and comment
◆ tagRemoved
|
signal |
Emitted when a tag has been removed.
- Parameters
-
tag
◆ tagUrl
|
slot |
Adds a tag to a given file URL, if the given tag doesn't exists then it gets created.
- Parameters
-
url the file URL to be tagged tag the tag to be added to the file URL color
- Deprecated
- Optional color
- Parameters
-
comment optional comment
- Returns
- whether the operation was successful
Definition at line 164 of file tagging.cpp.
◆ toggleFav
|
slot |
Toggle the fav tag of a given file, meaning, if a file is marked as fav then the tag gets removed and if it is not marked then the fav tag gets added.
- Parameters
-
url the file URL
- Returns
- whether the operation has been successful
Definition at line 410 of file tagging.cpp.
◆ unFav
|
slot |
If the given file has been marked as favorite then the tag is removed.
This works if the tagging component has been enabled, otherwise returns false as default.
- Parameters
-
url the file URL
- Returns
- whether the operation has been successful
Definition at line 423 of file tagging.cpp.
◆ updateUrl
Updates a file URL to a new URL, preserving all associated tags.
This is useful if a file is rename or moved to a new location
- Parameters
-
url previous file URL newUrl new file URL
- Returns
- whether the operation was successful
Definition at line 205 of file tagging.cpp.
◆ updateUrlTags
|
slot |
Updates the tags associated to a file URL.
If any of the given tags doesn't exists then they get created, if a tag associated to the current file URL is missing in the new passed tags then those get removed
- Parameters
-
url the file URL tags the new set of tags to be associated to the file URL
- Returns
- whether the operation was successful
Definition at line 193 of file tagging.cpp.
◆ urlRemoved
|
signal |
Emitted when a file has been removed a thus all associations to any tag.
- Parameters
-
url
◆ urlTagExists
Checks if a given tag is associated to a give file URL.
The check can be strictly enforced, meaning the given URL was tagged by the application making the request
- Parameters
-
url the file URL tag the tag to perform the check strict strictly enforced check
- Returns
Definition at line 118 of file tagging.cpp.
◆ urlTagged
Emitted when a tag has been assigened to a file URL.
- Parameters
-
url The file URL tagged tag the tag name
◆ urlTagRemoved
Emitted when a tag has been dissociated from a file URL.
- Parameters
-
tag url
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:55:53 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.