Konsole::Pty
#include <Pty.h>
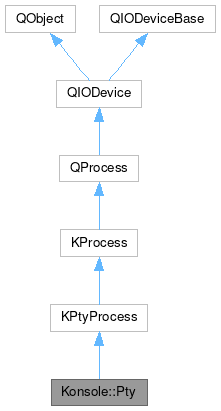
Signals | |
void | receivedData (const char *buffer, int length) |
Public Slots | |
void | sendData (const QByteArray &data) |
void | setUtf8Mode (bool on) |
Public Member Functions | |
Pty (int ptyMasterFd, QObject *parent=nullptr) | |
Pty (QObject *parent=nullptr) | |
void | closePty () |
char | eraseChar () const |
bool | flowControlEnabled () const |
int | foregroundProcessGroup () const |
QSize | pixelSize () const |
void | setEraseChar (char eraseChar) |
void | setFlowControlEnabled (bool on) |
void | setInitialWorkingDirectory (const QString &dir) |
void | setWindowSize (int columns, int lines, int width, int height) |
void | setWriteable (bool writeable) |
int | start (const QString &program, const QStringList &arguments, const QStringList &environment) |
QSize | windowSize () const |
![]() | |
KPtyProcess (int ptyMasterFd, QObject *parent=nullptr) | |
KPtyProcess (QObject *parent=nullptr) | |
bool | isUseUtmp () const |
KPtyDevice * | pty () const |
PtyChannels | ptyChannels () const |
void | setPtyChannels (PtyChannels channels) |
void | setUseUtmp (bool value) |
![]() | |
KProcess (QObject *parent=nullptr) | |
void | clearEnvironment () |
void | clearProgram () |
int | execute (int msecs=-1) |
KProcess & | operator<< (const QString &arg) |
KProcess & | operator<< (const QStringList &args) |
OutputChannelMode | outputChannelMode () const |
QStringList | program () const |
void | setEnv (const QString &name, const QString &value, bool overwrite=true) |
void | setNextOpenMode (QIODevice::OpenMode mode) |
void | setOutputChannelMode (OutputChannelMode mode) |
void | setProgram (const QString &exe, const QStringList &args=QStringList()) |
void | setProgram (const QStringList &argv) |
void | setShellCommand (const QString &cmd) |
void | start () |
int | startDetached () |
void | unsetEnv (const QString &name) |
![]() | |
QProcess (QObject *parent) | |
QStringList | arguments () const const |
virtual qint64 | bytesToWrite () const const override |
std::function< void()> | childProcessModifier ()> childProcessModifier() const const |
virtual void | close () override |
void | closeReadChannel (ProcessChannel channel) |
void | closeWriteChannel () |
CreateProcessArgumentModifier | createProcessArgumentsModifier () const const |
QStringList | environment () const const |
QProcess::ProcessError | error () const const |
void | errorOccurred (QProcess::ProcessError error) |
int | exitCode () const const |
QProcess::ExitStatus | exitStatus () const const |
void | finished (int exitCode, QProcess::ExitStatus exitStatus) |
InputChannelMode | inputChannelMode () const const |
virtual bool | isSequential () const const override |
void | kill () |
QString | nativeArguments () const const |
virtual bool | open (OpenMode mode) override |
ProcessChannelMode | processChannelMode () const const |
QProcessEnvironment | processEnvironment () const const |
qint64 | processId () const const |
QString | program () const const |
QByteArray | readAllStandardError () |
QByteArray | readAllStandardOutput () |
ProcessChannel | readChannel () const const |
void | readyReadStandardError () |
void | readyReadStandardOutput () |
void | setArguments (const QStringList &arguments) |
void | setChildProcessModifier (const std::function< void()> &modifier) |
void | setCreateProcessArgumentsModifier (CreateProcessArgumentModifier modifier) |
void | setEnvironment (const QStringList &environment) |
void | setInputChannelMode (InputChannelMode mode) |
void | setNativeArguments (const QString &arguments) |
void | setProcessChannelMode (ProcessChannelMode mode) |
void | setProcessEnvironment (const QProcessEnvironment &environment) |
void | setProgram (const QString &program) |
void | setReadChannel (ProcessChannel channel) |
void | setStandardErrorFile (const QString &fileName, OpenMode mode) |
void | setStandardInputFile (const QString &fileName) |
void | setStandardOutputFile (const QString &fileName, OpenMode mode) |
void | setStandardOutputProcess (QProcess *destination) |
void | setUnixProcessParameters (const UnixProcessParameters ¶ms) |
void | setUnixProcessParameters (UnixProcessFlags flagsOnly) |
void | setWorkingDirectory (const QString &dir) |
void | start (const QString &program, const QStringList &arguments, OpenMode mode) |
void | start (OpenMode mode) |
void | startCommand (const QString &command, OpenMode mode) |
bool | startDetached (qint64 *pid) |
void | started () |
QProcess::ProcessState | state () const const |
void | stateChanged (QProcess::ProcessState newState) |
void | terminate () |
UnixProcessParameters | unixProcessParameters () const const |
virtual bool | waitForBytesWritten (int msecs) override |
bool | waitForFinished (int msecs) |
virtual bool | waitForReadyRead (int msecs) override |
bool | waitForStarted (int msecs) |
QString | workingDirectory () const const |
![]() | |
QIODevice (QObject *parent) | |
void | aboutToClose () |
virtual bool | atEnd () const const |
virtual qint64 | bytesAvailable () const const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const const |
void | channelBytesWritten (int channel, qint64 bytes) |
void | channelReadyRead (int channel) |
void | commitTransaction () |
int | currentReadChannel () const const |
int | currentWriteChannel () const const |
QString | errorString () const const |
bool | getChar (char *c) |
bool | isOpen () const const |
bool | isReadable () const const |
bool | isTextModeEnabled () const const |
bool | isTransactionStarted () const const |
bool | isWritable () const const |
QIODeviceBase::OpenMode | openMode () const const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
virtual qint64 | pos () const const |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
int | readChannelCount () const const |
void | readChannelFinished () |
qint64 | readLine (char *data, qint64 maxSize) |
QByteArray | readLine (qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | rollbackTransaction () |
virtual bool | seek (qint64 pos) |
void | setCurrentReadChannel (int channel) |
void | setCurrentWriteChannel (int channel) |
void | setTextModeEnabled (bool enabled) |
virtual qint64 | size () const const |
qint64 | skip (qint64 maxSize) |
void | startTransaction () |
void | ungetChar (char c) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &data) |
int | writeChannelCount () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
enum | PtyChannelFlag |
typedef QFlags< PtyChannelFlag > | PtyChannels |
![]() | |
enum | OutputChannelMode |
![]() | |
typedef | CreateProcessArgumentModifier |
enum | ExitStatus |
enum | InputChannelMode |
enum | ProcessChannel |
enum | ProcessChannelMode |
enum | ProcessError |
enum | ProcessState |
enum | UnixProcessFlag |
typedef | UnixProcessFlags |
![]() | |
typedef | QObjectList |
![]() | |
typedef | OpenMode |
enum | OpenModeFlag |
![]() | |
objectName | |
![]() | |
static int | execute (const QString &exe, const QStringList &args=QStringList(), int msecs=-1) |
static int | execute (const QStringList &argv, int msecs=-1) |
static int | startDetached (const QString &exe, const QStringList &args=QStringList()) |
static int | startDetached (const QStringList &argv) |
![]() | |
int | execute (const QString &program, const QStringList &arguments) |
QString | nullDevice () |
QStringList | splitCommand (QStringView command) |
bool | startDetached (const QString &program, const QStringList &arguments, const QString &workingDirectory, qint64 *pid) |
QStringList | systemEnvironment () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
AllChannels | |
AllOutputChannels | |
NoChannels | |
StderrChannel | |
StdinChannel | |
StdoutChannel | |
![]() | |
ForwardedChannels | |
MergedChannels | |
OnlyStderrChannel | |
OnlyStdoutChannel | |
SeparateChannels | |
![]() | |
CloseFileDescriptors | |
Crashed | |
CrashExit | |
FailedToStart | |
ForwardedChannels | |
ForwardedErrorChannel | |
ForwardedInputChannel | |
ForwardedOutputChannel | |
IgnoreSigPipe | |
ManagedInputChannel | |
MergedChannels | |
NormalExit | |
NotRunning | |
ReadError | |
ResetSignalHandlers | |
Running | |
SeparateChannels | |
StandardError | |
StandardOutput | |
Starting | |
Timedout | |
UnknownError | |
UseVFork | |
WriteError | |
![]() | |
Append | |
ExistingOnly | |
NewOnly | |
NotOpen | |
ReadOnly | |
ReadWrite | |
Text | |
Truncate | |
Unbuffered | |
WriteOnly | |
![]() | |
KCOREADDONS_NO_EXPORT | KProcess (KProcessPrivate *d, QObject *parent) |
![]() | |
virtual qint64 | readData (char *data, qint64 maxlen) override |
void | setProcessState (ProcessState state) |
![]() | |
virtual qint64 | readLineData (char *data, qint64 maxSize) |
void | setErrorString (const QString &str) |
void | setOpenMode (QIODeviceBase::OpenMode openMode) |
virtual qint64 | skipData (qint64 maxSize) |
virtual qint64 | writeData (const char *data, qint64 maxSize)=0 |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
std::unique_ptr< KProcessPrivate > const | d_ptr |
Detailed Description
The Pty class is used to start the terminal process, send data to it, receive data from it and manipulate various properties of the pseudo-teletype interface used to communicate with the process.
To use this class, construct an instance and connect to the sendData slot and receivedData signal to send data to or receive data from the process.
To start the terminal process, call the start() method with the program name and appropriate arguments.
Constructor & Destructor Documentation
◆ Pty() [1/2]
|
explicit |
Constructs a new Pty.
Connect to the sendData() slot and receivedData() signal to prepare for sending and receiving data from the terminal process.
To start the terminal process, call the run() method with the name of the program to start and appropriate arguments.
◆ Pty() [2/2]
|
explicit |
Construct a process using an open pty master.
Member Function Documentation
◆ closePty()
void Pty::closePty | ( | ) |
◆ eraseChar()
◆ flowControlEnabled()
bool Pty::flowControlEnabled | ( | ) | const |
◆ foregroundProcessGroup()
int Pty::foregroundProcessGroup | ( | ) | const |
Returns the process id of the teletype's current foreground process.
This is the process which is currently reading input sent to the terminal via. sendData()
If there is a problem reading the foreground process group, 0 will be returned.
◆ pixelSize()
QSize Pty::pixelSize | ( | ) | const |
Returns the size of the window used by this teletype in pixels.
See setWindowSize()
◆ receivedData
|
signal |
Emitted when a new block of data is received from the teletype.
- Parameters
-
buffer Pointer to the data received. length Length of buffer
◆ sendData
|
slot |
Sends data to the process currently controlling the teletype ( whose id is returned by foregroundProcessGroup() )
- Parameters
-
data the data to send.
◆ setEraseChar()
void Pty::setEraseChar | ( | char | eraseChar | ) |
◆ setFlowControlEnabled()
void Pty::setFlowControlEnabled | ( | bool | on | ) |
Enables or disables Xon/Xoff flow control.
The flow control setting may be changed later by a terminal application, so flowControlEnabled() may not equal the value of on
in the previous call to setFlowControlEnabled()
◆ setInitialWorkingDirectory()
void Pty::setInitialWorkingDirectory | ( | const QString & | dir | ) |
◆ setUtf8Mode
|
slot |
◆ setWindowSize()
void Pty::setWindowSize | ( | int | columns, |
int | lines, | ||
int | width, | ||
int | height ) |
◆ setWriteable()
void Pty::setWriteable | ( | bool | writeable | ) |
◆ start()
int Pty::start | ( | const QString & | program, |
const QStringList & | arguments, | ||
const QStringList & | environment ) |
Starts the terminal process.
Returns 0 if the process was started successfully or non-zero otherwise.
- Parameters
-
program Path to the program to start arguments Arguments to pass to the program being started environment A list of key=value pairs which will be added to the environment for the new process. At the very least this should include an assignment for the TERM environment variable.
◆ windowSize()
QSize Pty::windowSize | ( | ) | const |
Returns the size of the window used by this teletype in characters.
See setWindowSize()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:55:13 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.