MauiMan::ThemeManager
#include <thememanager.h>
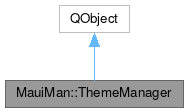
Classes | |
struct | DefaultValues |
Properties | |
QString | accentColor |
uint | borderRadius |
QString | customColorScheme |
QString | defaultFont |
bool | enableCSD |
bool | enableEffects |
uint | iconSize |
QString | iconTheme |
uint | marginSize |
QString | monospacedFont |
uint | paddingSize |
QString | smallFont |
uint | spacingSize |
int | styleType |
QString | windowControlsTheme |
![]() | |
objectName | |
Signals | |
void | accentColorChanged (QString accentColor) |
void | borderRadiusChanged (uint radius) |
void | customColorSchemeChanged (QString customColorScheme) |
void | defaultFontChanged (QString defaultFont) |
void | enableCSDChanged (bool enableCSD) |
void | enableEffectsChanged (bool enableEffects) |
void | iconSizeChanged (uint size) |
void | iconThemeChanged (QString iconTheme) |
void | marginSizeChanged (uint marginSize) |
void | monospacedFontChanged (QString monospacedFont) |
void | paddingSizeChanged (uint paddingSize) |
void | smallFontChanged (QString smallFont) |
void | spacingSizeChanged (uint spacingSize) |
void | styleTypeChanged (int styleType) |
void | windowControlsThemeChanged (QString windowControlsTheme) |
Public Member Functions | |
ThemeManager (QObject *parent=nullptr) | |
const QString & | accentColor () const |
uint | borderRadius () const |
QString | customColorScheme () const |
QString | defaultFont () const |
bool | enableCSD () const |
bool | enableEffects () const |
uint | iconSize () const |
const QString & | iconTheme () const |
uint | marginSize () const |
QString | monospacedFont () const |
uint | paddingSize () const |
void | resetAccentColor () |
void | resetBorderRadius () |
void | resetDefaultFont () |
void | resetIconSize () |
void | resetMarginSize () |
void | resetMonospacedFont () |
void | resetPaddingSize () |
void | resetSmallFont () |
void | resetSPacingSize () |
void | setAccentColor (const QString &newAccentColor) |
void | setBorderRadius (uint newBorderRadius) |
void | setCustomColorScheme (const QString &customColorScheme) |
void | setDefaultFont (const QString &defaultFont) |
void | setEnableCSD (bool enableCSD) |
void | setEnableEffects (bool enableEffects) |
void | setIconSize (uint newIconSize) |
void | setIconTheme (const QString &newIconTheme) |
void | setMarginSize (uint marginSize) |
void | setMonospacedFont (const QString &monospacedFont) |
void | setPaddingSize (uint paddingSize) |
void | setSmallFont (const QString &smallFont) |
void | setSpacingSize (uint spacingSize) |
void | setStyleType (int newStyleType) |
void | setWindowControlsTheme (const QString &newWindowControlsTheme) |
QString | smallFont () const |
uint | spacingSize () const |
int | styleType () const |
const QString & | windowControlsTheme () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The ThemeManager class Helpful for third parties to connect to property changes from the Theme module setting changes.
- Note
- Note that the properties can be reset back to their default values by using the
undefined
value from QML, or using the reset methods accordingly.
The preferences have a set of default values, however the values used will be the ones present in the conf file. When a property is reset, then the default value will be written to the conf file.
Definition at line 29 of file thememanager.h.
Property Documentation
◆ accentColor
|
readwrite |
The preferred accent color used for the highlighted and checked states.
Definition at line 46 of file thememanager.h.
◆ borderRadius
|
readwrite |
The corner border radius of the UI elements, also affects the radius of the CSD window corners.
Definition at line 67 of file thememanager.h.
◆ customColorScheme
|
readwrite |
The preferred color scheme to be used when the styleType
is set to Custom.
Definition at line 112 of file thememanager.h.
◆ defaultFont
|
readwrite |
The preferred default font, for most part of the UI.
Definition at line 97 of file thememanager.h.
◆ enableCSD
|
readwrite |
Whether to use client side decorations (CSD) for the applications.
By default this is set to true
Definition at line 62 of file thememanager.h.
◆ enableEffects
|
readwrite |
Whether the user prefers for the system to have visual effects, such as animations, blur, etc.
Definition at line 92 of file thememanager.h.
◆ iconSize
|
readwrite |
The preferred size of the icons in the buttons, menu entries and other elements.
Definition at line 72 of file thememanager.h.
◆ iconTheme
|
readwrite |
The preferred icon theme.
This preference is only valid when using the Maui Shell session.
Definition at line 51 of file thememanager.h.
◆ marginSize
|
readwrite |
The preferred margin size around views.
Definition at line 82 of file thememanager.h.
◆ monospacedFont
|
readwrite |
The preferred mono spaced font, for example the one used in console terminals, or in text editors.
Definition at line 107 of file thememanager.h.
◆ paddingSize
|
readwrite |
The preferred padding size for the UI elements, such as buttons, tool bars, menu entries, etc.
Definition at line 77 of file thememanager.h.
◆ smallFont
|
readwrite |
The preferred small font, for details.
Definition at line 102 of file thememanager.h.
◆ spacingSize
|
readwrite |
The preferred spacing size between elements in a row or column, such as lists or browsing elements.
Definition at line 87 of file thememanager.h.
◆ styleType
|
readwrite |
The style type for the color scheme.
The possible values are:
- 0 Light
- 1 Dark
- 2 Adaptive
- 3 Custom (from the plasma color-scheme file preferences)
- 4 True Black
- 5 Inverted (True White)
Definition at line 41 of file thememanager.h.
◆ windowControlsTheme
|
readwrite |
The preferred theme for the CSD window control buttons.
The list of available options depends on the installed themes. For more information on this refer to the MauiKit CSDControls documentation.
Definition at line 56 of file thememanager.h.
Constructor & Destructor Documentation
◆ ThemeManager()
|
explicit |
Definition at line 14 of file thememanager.cpp.
Member Function Documentation
◆ accentColor()
const QString & ThemeManager::accentColor | ( | ) | const |
Definition at line 153 of file thememanager.cpp.
◆ borderRadius()
uint ThemeManager::borderRadius | ( | ) | const |
Definition at line 358 of file thememanager.cpp.
◆ customColorScheme()
QString ThemeManager::customColorScheme | ( | ) | const |
Definition at line 535 of file thememanager.cpp.
◆ defaultFont()
QString ThemeManager::defaultFont | ( | ) | const |
Definition at line 472 of file thememanager.cpp.
◆ enableCSD()
bool ThemeManager::enableCSD | ( | ) | const |
Definition at line 208 of file thememanager.cpp.
◆ enableEffects()
bool ThemeManager::enableEffects | ( | ) | const |
Definition at line 393 of file thememanager.cpp.
◆ iconSize()
uint ThemeManager::iconSize | ( | ) | const |
Definition at line 378 of file thememanager.cpp.
◆ iconTheme()
const QString & ThemeManager::iconTheme | ( | ) | const |
Definition at line 176 of file thememanager.cpp.
◆ marginSize()
uint ThemeManager::marginSize | ( | ) | const |
Definition at line 414 of file thememanager.cpp.
◆ monospacedFont()
QString ThemeManager::monospacedFont | ( | ) | const |
Definition at line 482 of file thememanager.cpp.
◆ paddingSize()
uint ThemeManager::paddingSize | ( | ) | const |
Definition at line 409 of file thememanager.cpp.
◆ resetAccentColor()
void ThemeManager::resetAccentColor | ( | ) |
Definition at line 171 of file thememanager.cpp.
◆ resetBorderRadius()
void ThemeManager::resetBorderRadius | ( | ) |
Definition at line 373 of file thememanager.cpp.
◆ resetDefaultFont()
void ThemeManager::resetDefaultFont | ( | ) |
Definition at line 498 of file thememanager.cpp.
◆ resetIconSize()
void MauiMan::ThemeManager::resetIconSize | ( | ) |
Definition at line 551 of file thememanager.cpp.
◆ resetMarginSize()
void ThemeManager::resetMarginSize | ( | ) |
Definition at line 446 of file thememanager.cpp.
◆ resetMonospacedFont()
void ThemeManager::resetMonospacedFont | ( | ) |
Definition at line 530 of file thememanager.cpp.
◆ resetPaddingSize()
void ThemeManager::resetPaddingSize | ( | ) |
Definition at line 430 of file thememanager.cpp.
◆ resetSmallFont()
void ThemeManager::resetSmallFont | ( | ) |
Definition at line 514 of file thememanager.cpp.
◆ resetSPacingSize()
void ThemeManager::resetSPacingSize | ( | ) |
Definition at line 467 of file thememanager.cpp.
◆ setAccentColor()
void ThemeManager::setAccentColor | ( | const QString & | newAccentColor | ) |
Definition at line 158 of file thememanager.cpp.
◆ setBorderRadius()
void ThemeManager::setBorderRadius | ( | uint | newBorderRadius | ) |
Definition at line 363 of file thememanager.cpp.
◆ setCustomColorScheme()
void ThemeManager::setCustomColorScheme | ( | const QString & | customColorScheme | ) |
Definition at line 540 of file thememanager.cpp.
◆ setDefaultFont()
void ThemeManager::setDefaultFont | ( | const QString & | defaultFont | ) |
Definition at line 487 of file thememanager.cpp.
◆ setEnableCSD()
void ThemeManager::setEnableCSD | ( | bool | enableCSD | ) |
Definition at line 213 of file thememanager.cpp.
◆ setEnableEffects()
void ThemeManager::setEnableEffects | ( | bool | enableEffects | ) |
Definition at line 398 of file thememanager.cpp.
◆ setIconSize()
void ThemeManager::setIconSize | ( | uint | newIconSize | ) |
Definition at line 383 of file thememanager.cpp.
◆ setIconTheme()
void ThemeManager::setIconTheme | ( | const QString & | newIconTheme | ) |
Definition at line 181 of file thememanager.cpp.
◆ setMarginSize()
void ThemeManager::setMarginSize | ( | uint | marginSize | ) |
Definition at line 435 of file thememanager.cpp.
◆ setMonospacedFont()
void ThemeManager::setMonospacedFont | ( | const QString & | monospacedFont | ) |
Definition at line 519 of file thememanager.cpp.
◆ setPaddingSize()
void ThemeManager::setPaddingSize | ( | uint | paddingSize | ) |
Definition at line 419 of file thememanager.cpp.
◆ setSmallFont()
void ThemeManager::setSmallFont | ( | const QString & | smallFont | ) |
Definition at line 503 of file thememanager.cpp.
◆ setSpacingSize()
void ThemeManager::setSpacingSize | ( | uint | spacingSize | ) |
Definition at line 456 of file thememanager.cpp.
◆ setStyleType()
void ThemeManager::setStyleType | ( | int | newStyleType | ) |
Definition at line 142 of file thememanager.cpp.
◆ setWindowControlsTheme()
void ThemeManager::setWindowControlsTheme | ( | const QString & | newWindowControlsTheme | ) |
Definition at line 197 of file thememanager.cpp.
◆ smallFont()
QString ThemeManager::smallFont | ( | ) | const |
Definition at line 477 of file thememanager.cpp.
◆ spacingSize()
uint ThemeManager::spacingSize | ( | ) | const |
Definition at line 451 of file thememanager.cpp.
◆ styleType()
int ThemeManager::styleType | ( | ) | const |
Definition at line 137 of file thememanager.cpp.
◆ windowControlsTheme()
const QString & ThemeManager::windowControlsTheme | ( | ) | const |
Definition at line 192 of file thememanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 25 2025 11:54:49 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.