Phonon::AudioOutput
#include <phonon/AudioOutput>
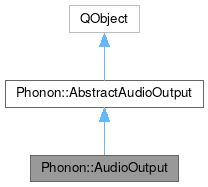
Properties | |
bool | muted |
QString | name |
AudioOutputDevice | outputDevice |
qreal | volume |
qreal | volumeDecibel |
![]() | |
objectName | |
Signals | |
void | mutedChanged (bool) |
void | outputDeviceChanged (const Phonon::AudioOutputDevice &newAudioOutputDevice) |
void | volumeChanged (qreal newVolume) |
Public Slots | |
void | setMuted (bool mute) |
void | setName (const QString &newName) |
bool | setOutputDevice (const Phonon::AudioOutputDevice &newAudioOutputDevice) |
void | setVolume (qreal newVolume) |
void | setVolumeDecibel (qreal newVolumeDecibel) |
Public Member Functions | |
AudioOutput (Phonon::Category category, QObject *parent=nullptr) | |
AudioOutput (QObject *parent=nullptr) | |
Phonon::Category | category () const |
bool | isMuted () const |
QString | name () const |
AudioOutputDevice | outputDevice () const |
qreal | volume () const |
qreal | volumeDecibel () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
AbstractAudioOutput (AbstractAudioOutputPrivate &dd, QObject *parent) | |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Class for audio output to the soundcard.
Use this class to define the audio output.
- See also
- Phonon::Ui::VolumeSlider
Definition at line 48 of file audiooutput.h.
Property Documentation
◆ muted
|
readwrite |
This property tells whether the output is muted.
Muting the output has the same effect as calling setVolume(0.0).
Definition at line 96 of file audiooutput.h.
◆ name
|
readwrite |
This is the name that appears in Mixer applications that control the volume of this output.
- See also
- category
Definition at line 60 of file audiooutput.h.
◆ outputDevice
|
readwrite |
This property holds the (hardware) destination for the output.
The default device is determined by the category and the global configuration for that category of outputs. Normally you don't need to override this setting - letting the user change the global configuration is the right choice. You can still override the device though, if you have good reasons to do so.
- See also
- outputDeviceChanged
Definition at line 89 of file audiooutput.h.
◆ volume
|
readwrite |
This is the current loudness of the output (it is using Stevens' law to calculate the change in voltage internally).
- See also
- volumeDecibel
Definition at line 67 of file audiooutput.h.
◆ volumeDecibel
|
readwrite |
This is the current volume of the output in decibel.
0 dB means no change in volume, -6dB means an attenuation of the voltage to 50% and an attenuation of the power to 25%, -inf dB means silence.
- See also
- volume
Definition at line 77 of file audiooutput.h.
Constructor & Destructor Documentation
◆ AudioOutput() [1/2]
|
explicit |
Creates a new AudioOutput that defines output to a physical device.
- Parameters
-
category The category can be used by mixer applications to group volume controls of applications into categories. That makes it easier for the user to identify the programs. The category is also used for the default output device that is configured centrally. As an example: often users want to have the audio signal of a VoIP application go to their USB headset while all other sounds should go to the internal soundcard. parent QObject parent
- See also
- Phonon::categoryToString
- outputDevice
Definition at line 68 of file audiooutput.cpp.
◆ AudioOutput() [2/2]
|
explicit |
Definition at line 75 of file audiooutput.cpp.
Member Function Documentation
◆ category()
Category Phonon::AudioOutput::category | ( | ) | const |
Returns the category of this output.
Definition at line 247 of file audiooutput.cpp.
◆ isMuted()
bool Phonon::AudioOutput::isMuted | ( | ) | const |
Definition at line 207 of file audiooutput.cpp.
◆ mutedChanged
|
signal |
This signal is emitted when the muted property has changed.
As this property can change by outside sources, a UI element showing the muted property should listen to this signal.
◆ name()
QString Phonon::AudioOutput::name | ( | ) | const |
Definition at line 134 of file audiooutput.cpp.
◆ outputDevice()
AudioOutputDevice Phonon::AudioOutput::outputDevice | ( | ) | const |
Definition at line 253 of file audiooutput.cpp.
◆ outputDeviceChanged
|
signal |
This signal is emitted when the (hardware) device for the output has changed.
The change can happen either through setOutputDevice or if the global configuration for the used category has changed.
- See also
- outputDevice
◆ setMuted
|
slot |
Definition at line 213 of file audiooutput.cpp.
◆ setName
|
slot |
Definition at line 140 of file audiooutput.cpp.
◆ setOutputDevice
|
slot |
Definition at line 259 of file audiooutput.cpp.
◆ setVolume
|
slot |
Definition at line 157 of file audiooutput.cpp.
◆ setVolumeDecibel
|
slot |
Definition at line 202 of file audiooutput.cpp.
◆ volume()
qreal Phonon::AudioOutput::volume | ( | ) | const |
Definition at line 181 of file audiooutput.cpp.
◆ volumeChanged
|
signal |
This signal is emitted whenever the volume has changed.
As the volume can change without a call to setVolume this is important to keep a widget showing the current volume up to date.
◆ volumeDecibel()
qreal Phonon::AudioOutput::volumeDecibel | ( | ) | const |
Definition at line 194 of file audiooutput.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:04:30 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.