PlasmaQuick::SharedQmlEngine
#include <sharedqmlengine.h>
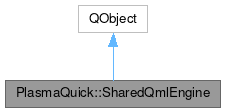
Properties | |
bool | initializationDelayed |
QObject * | rootObject |
QUrl | source |
QQmlComponent::Status | status |
QString | translationDomain |
![]() | |
objectName | |
Signals | |
void | finished () |
void | statusChanged (QQmlComponent::Status) |
Public Slots | |
void | completeInitialization (const QVariantHash &initialProperties=QVariantHash()) |
Public Member Functions | |
SharedQmlEngine (Plasma::Applet *applet, QObject *parent=nullptr) | |
SharedQmlEngine (QObject *parent=nullptr) | |
QObject * | createObjectFromComponent (QQmlComponent *component, QQmlContext *context=nullptr, const QVariantHash &initialProperties=QVariantHash()) |
QObject * | createObjectFromSource (const QUrl &source, QQmlContext *context=nullptr, const QVariantHash &initialProperties=QVariantHash()) |
std::shared_ptr< QQmlEngine > | engine () |
bool | isInitializationDelayed () const |
QQmlComponent * | mainComponent () const |
QQmlContext * | rootContext () const |
QObject * | rootObject () const |
void | setInitializationDelayed (const bool delay) |
void | setSource (const QUrl &source) |
void | setSourceFromModule (QAnyStringView module, QAnyStringView type) |
void | setTranslationDomain (const QString &translationDomain) |
QUrl | source () const |
QQmlComponent::Status | status () const |
QString | translationDomain () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An object that instantiates an entire QML context, with its own declarative engine.
PlasmaQuick::SharedQmlEngine provides a class to conveniently use QML based declarative user interfaces. A SharedQmlEngine corresponds to one QML file (which can include others). It will a shared QQmlEngine with a single root object, described in the QML file.
- Since
- 6.0
Definition at line 42 of file sharedqmlengine.h.
Property Documentation
◆ initializationDelayed
|
readwrite |
Definition at line 48 of file sharedqmlengine.h.
◆ rootObject
|
read |
Definition at line 49 of file sharedqmlengine.h.
◆ source
|
readwrite |
Definition at line 46 of file sharedqmlengine.h.
◆ status
|
read |
Definition at line 50 of file sharedqmlengine.h.
◆ translationDomain
|
readwrite |
Definition at line 47 of file sharedqmlengine.h.
Constructor & Destructor Documentation
◆ SharedQmlEngine() [1/2]
|
explicit |
Construct a new PlasmaQuick::SharedQmlEngine.
- Parameters
-
parent The QObject parent for this object.
Definition at line 153 of file sharedqmlengine.cpp.
◆ SharedQmlEngine() [2/2]
|
explicit |
Definition at line 164 of file sharedqmlengine.cpp.
◆ ~SharedQmlEngine()
|
override |
Definition at line 174 of file sharedqmlengine.cpp.
Member Function Documentation
◆ completeInitialization
|
slot |
Finishes the process of initialization.
If isInitializationDelayed() is false, calling this will have no effect.
- Parameters
-
initialProperties optional properties that will be set on the object when created (and before Component.onCompleted gets emitted
Definition at line 252 of file sharedqmlengine.cpp.
◆ createObjectFromComponent()
QObject * PlasmaQuick::SharedQmlEngine::createObjectFromComponent | ( | QQmlComponent * | component, |
QQmlContext * | context = nullptr, | ||
const QVariantHash & | initialProperties = QVariantHash() ) |
Creates and returns an object based on the provided QQmlComponent with the same QQmlEngine and the same root context as the admin object, that will be the parent of the newly created object.
- Parameters
-
component the component we want to instantiate context The QQmlContext in which we will create the object, if 0 it will use the engine's root context initialProperties optional properties that will be set on the object when created (and before Component.onCompleted gets emitted
Definition at line 282 of file sharedqmlengine.cpp.
◆ createObjectFromSource()
QObject * PlasmaQuick::SharedQmlEngine::createObjectFromSource | ( | const QUrl & | source, |
QQmlContext * | context = nullptr, | ||
const QVariantHash & | initialProperties = QVariantHash() ) |
Creates and returns an object based on the provided url to a Qml file with the same QQmlEngine and the same root context as the main object, that will be the parent of the newly created object.
- Parameters
-
source url where the QML file is located context The QQmlContext in which we will create the object, if 0 it will use the engine's root context initialProperties optional properties that will be set on the object when created (and before Component.onCompleted gets emitted
Definition at line 274 of file sharedqmlengine.cpp.
◆ engine()
std::shared_ptr< QQmlEngine > PlasmaQuick::SharedQmlEngine::engine | ( | ) |
- Returns
- the declarative engine that runs the qml file assigned to this widget.
Definition at line 219 of file sharedqmlengine.cpp.
◆ finished
|
signal |
Emitted when the parsing and execution of the QML file is terminated.
◆ isInitializationDelayed()
bool PlasmaQuick::SharedQmlEngine::isInitializationDelayed | ( | ) | const |
- Returns
- true if the initialization of the QML file will be delayed at the end of the event loop
Definition at line 214 of file sharedqmlengine.cpp.
◆ mainComponent()
QQmlComponent * PlasmaQuick::SharedQmlEngine::mainComponent | ( | ) | const |
- Returns
- the main QQmlComponent of the engine
Definition at line 229 of file sharedqmlengine.cpp.
◆ rootContext()
QQmlContext * PlasmaQuick::SharedQmlEngine::rootContext | ( | ) | const |
The components's creation context.
Definition at line 234 of file sharedqmlengine.cpp.
◆ rootObject()
QObject * PlasmaQuick::SharedQmlEngine::rootObject | ( | ) | const |
- Returns
- the root object of the declarative object tree
Definition at line 224 of file sharedqmlengine.cpp.
◆ setInitializationDelayed()
void PlasmaQuick::SharedQmlEngine::setInitializationDelayed | ( | const bool | delay | ) |
Sets whether the execution of the QML file has to be delayed later in the event loop.
It has to be called before setQmlPath(). In this case it will be possible to assign new objects in the main engine context before the main component gets initialized. In that case it will be possible to access it immediately from the QML code. The initialization will either be completed automatically asynchronously or explicitly by calling completeInitialization()
- Parameters
-
delay if true the initialization of the QML file will be delayed at the end of the event loop
Definition at line 209 of file sharedqmlengine.cpp.
◆ setSource()
void PlasmaQuick::SharedQmlEngine::setSource | ( | const QUrl & | source | ) |
Sets the path of the QML file to parse and execute.
- Parameters
-
path the absolute path of a QML file
Definition at line 191 of file sharedqmlengine.cpp.
◆ setSourceFromModule()
void PlasmaQuick::SharedQmlEngine::setSourceFromModule | ( | QAnyStringView | module, |
QAnyStringView | type ) |
Sets the QML source to execute from a type in a module.
- Parameters
-
module The module to load the type from. type The type to load from the module.
Definition at line 196 of file sharedqmlengine.cpp.
◆ setTranslationDomain()
void PlasmaQuick::SharedQmlEngine::setTranslationDomain | ( | const QString & | translationDomain | ) |
Call this method before calling setupBindings to install a translation domain for all i18n global functions.
If a translation domain is set all i18n calls delegate to the matching i18nd calls with the provided translation domain.
The translationDomain affects all i18n calls including those from imports. Because of that modules intended to be used as imports should prefer the i18nd variants and set the translation domain explicitly in each call.
This method is only required if your declarative usage is inside a library. If it's in an application there is no need to set the translation domain as the application's domain can be used.
- Parameters
-
translationDomain The translation domain to be used for i18n calls.
Definition at line 181 of file sharedqmlengine.cpp.
◆ source()
QUrl PlasmaQuick::SharedQmlEngine::source | ( | ) | const |
- Returns
- the absolute path of the current QML file
Definition at line 201 of file sharedqmlengine.cpp.
◆ status()
QQmlComponent::Status PlasmaQuick::SharedQmlEngine::status | ( | ) | const |
The component's current status.
Definition at line 239 of file sharedqmlengine.cpp.
◆ translationDomain()
QString PlasmaQuick::SharedQmlEngine::translationDomain | ( | ) | const |
- Returns
- the translation domain for the i18n calls done in this QML engine
Definition at line 186 of file sharedqmlengine.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:48:43 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.