NotificationManager::Notifications
#include <notifications.h>
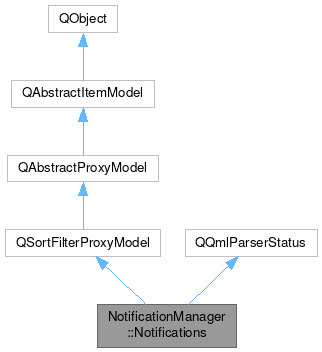
Properties | |
int | activeJobsCount |
int | activeNotificationsCount |
QStringList | blacklistedDesktopEntries |
QStringList | blacklistedNotifyRcNames |
int | count |
bool | expandUnread |
int | expiredNotificationsCount |
int | groupLimit |
GroupMode | groupMode |
int | jobsPercentage |
QDateTime | lastRead |
QML_ELEMENTint | limit |
bool | showDismissed |
bool | showExpired |
bool | showJobs |
bool | showNotifications |
SortMode | sortMode |
Qt::SortOrder | sortOrder |
int | unreadNotificationsCount |
Urgencies | urgencies |
QStringList | whitelistedDesktopEntries |
QStringList | whitelistedNotifyRcNames |
QWindow * | window |
![]() | |
autoAcceptChildRows | |
dynamicSortFilter | |
filterCaseSensitivity | |
filterKeyColumn | |
filterRegularExpression | |
filterRole | |
isSortLocaleAware | |
recursiveFilteringEnabled | |
sortCaseSensitivity | |
sortRole | |
![]() | |
sourceModel | |
![]() | |
objectName | |
Signals | |
void | activeJobsCountChanged () |
void | activeNotificationsCountChanged () |
void | blacklistedDesktopEntriesChanged () |
void | blacklistedNotifyRcNamesChanged () |
void | countChanged () |
void | expandUnreadChanged () |
void | expiredNotificationsCountChanged () |
void | groupLimitChanged () |
void | groupModeChanged () |
void | jobsPercentageChanged () |
void | lastReadChanged () |
void | limitChanged () |
void | showDismissedChanged () |
void | showExpiredChanged () |
void | showJobsChanged () |
void | showNotificationsChanged () |
void | sortModeChanged () |
void | sortOrderChanged () |
void | unreadNotificationsCountChanged () |
void | urgenciesChanged () |
void | whitelistedDesktopEntriesChanged () |
void | whitelistedNotifyRcNamesChanged () |
void | windowChanged (QWindow *window) |
Public Member Functions | |
Notifications (QObject *parent=nullptr) | |
int | activeJobsCount () const |
int | activeNotificationsCount () const |
QStringList | blacklistedDesktopEntries () const |
QStringList | blacklistedNotifyRcNames () const |
Q_INVOKABLE void | clear (ClearFlags flags) |
Q_INVOKABLE void | close (const QModelIndex &idx) |
Q_INVOKABLE void | collapseAllGroups () |
Q_INVOKABLE void | configure (const QModelIndex &idx) |
int | count () const |
QVariant | data (const QModelIndex &index, int role) const override |
bool | expandUnread () const |
Q_INVOKABLE void | expire (const QModelIndex &idx) |
int | expiredNotificationsCount () const |
bool | filterAcceptsRow (int source_row, const QModelIndex &source_parent) const override |
Q_INVOKABLE QModelIndex | groupIndex (const QModelIndex &idx) const |
int | groupLimit () const |
GroupMode | groupMode () const |
Q_INVOKABLE void | invokeAction (const QModelIndex &idx, const QString &actionId, InvokeBehavior=None) |
Q_INVOKABLE void | invokeDefaultAction (const QModelIndex &idx, InvokeBehavior behavior=None) |
int | jobsPercentage () const |
Q_INVOKABLE void | killJob (const QModelIndex &idx) |
QDateTime | lastRead () const |
bool | lessThan (const QModelIndex &source_left, const QModelIndex &source_right) const override |
int | limit () const |
Q_INVOKABLE QPersistentModelIndex | makePersistentModelIndex (const QModelIndex &idx) const |
Q_INVOKABLE void | reply (const QModelIndex &idx, const QString &text, InvokeBehavior behavior) |
void | resetLastRead () |
Q_INVOKABLE void | resumeJob (const QModelIndex &idx) |
QHash< int, QByteArray > | roleNames () const override |
int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
void | setBlacklistedDesktopEntries (const QStringList &blacklist) |
void | setBlacklistedNotifyRcNames (const QStringList &blacklist) |
bool | setData (const QModelIndex &index, const QVariant &value, int role) override |
void | setExpandUnread (bool expand) |
void | setGroupLimit (int limit) |
void | setGroupMode (GroupMode groupMode) |
void | setLastRead (const QDateTime &lastRead) |
void | setLimit (int limit) |
void | setShowDismissed (bool show) |
void | setShowExpired (bool show) |
void | setShowJobs (bool showJobs) |
void | setShowNotifications (bool showNotifications) |
void | setSortMode (SortMode sortMode) |
void | setSortOrder (Qt::SortOrder sortOrder) |
void | setUrgencies (Urgencies urgencies) |
void | setWhitelistedDesktopEntries (const QStringList &whitelist) |
void | setWhitelistedNotifyRcNames (const QStringList &whitelist) |
void | setWindow (QWindow *window) |
bool | showDismissed () const |
bool | showExpired () const |
bool | showJobs () const |
bool | showNotifications () const |
SortMode | sortMode () const |
Qt::SortOrder | sortOrder () const |
Q_INVOKABLE void | startTimeout (const QModelIndex &idx) |
Q_INVOKABLE void | startTimeout (uint notificationId) |
Q_INVOKABLE void | stopTimeout (const QModelIndex &idx) |
Q_INVOKABLE void | suspendJob (const QModelIndex &idx) |
int | unreadNotificationsCount () const |
Urgencies | urgencies () const |
QStringList | whitelistedDesktopEntries () const |
QStringList | whitelistedNotifyRcNames () const |
QWindow * | window () const |
![]() | |
QSortFilterProxyModel (QObject *parent) | |
bool | autoAcceptChildRows () const const |
void | autoAcceptChildRowsChanged (bool autoAcceptChildRows) |
QBindable< bool > | bindableAutoAcceptChildRows () |
QBindable< bool > | bindableDynamicSortFilter () |
QBindable< Qt::CaseSensitivity > | bindableFilterCaseSensitivity () |
QBindable< int > | bindableFilterKeyColumn () |
QBindable< QRegularExpression > | bindableFilterRegularExpression () |
QBindable< int > | bindableFilterRole () |
QBindable< bool > | bindableIsSortLocaleAware () |
QBindable< bool > | bindableRecursiveFilteringEnabled () |
QBindable< Qt::CaseSensitivity > | bindableSortCaseSensitivity () |
QBindable< int > | bindableSortRole () |
virtual QModelIndex | buddy (const QModelIndex &index) const const override |
virtual bool | canFetchMore (const QModelIndex &parent) const const override |
virtual int | columnCount (const QModelIndex &parent) const const override |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) override |
bool | dynamicSortFilter () const const |
virtual void | fetchMore (const QModelIndex &parent) override |
Qt::CaseSensitivity | filterCaseSensitivity () const const |
void | filterCaseSensitivityChanged (Qt::CaseSensitivity filterCaseSensitivity) |
int | filterKeyColumn () const const |
QRegularExpression | filterRegularExpression () const const |
int | filterRole () const const |
void | filterRoleChanged (int filterRole) |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const const override |
virtual bool | hasChildren (const QModelIndex &parent) const const override |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const const override |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent) const const override |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) override |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) override |
void | invalidate () |
bool | isRecursiveFilteringEnabled () const const |
bool | isSortLocaleAware () const const |
virtual QModelIndex | mapFromSource (const QModelIndex &sourceIndex) const const override |
virtual QItemSelection | mapSelectionFromSource (const QItemSelection &sourceSelection) const const override |
virtual QItemSelection | mapSelectionToSource (const QItemSelection &proxySelection) const const override |
virtual QModelIndex | mapToSource (const QModelIndex &proxyIndex) const const override |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, Qt::MatchFlags flags) const const override |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const const override |
virtual QStringList | mimeTypes () const const override |
virtual QModelIndex | parent (const QModelIndex &child) const const override |
void | recursiveFilteringEnabledChanged (bool recursiveFilteringEnabled) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) override |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) override |
void | setAutoAcceptChildRows (bool accept) |
void | setDynamicSortFilter (bool enable) |
void | setFilterCaseSensitivity (Qt::CaseSensitivity cs) |
void | setFilterFixedString (const QString &pattern) |
void | setFilterKeyColumn (int column) |
void | setFilterRegularExpression (const QRegularExpression ®ularExpression) |
void | setFilterRegularExpression (const QString &pattern) |
void | setFilterRole (int role) |
void | setFilterWildcard (const QString &pattern) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) override |
void | setRecursiveFilteringEnabled (bool recursive) |
void | setSortCaseSensitivity (Qt::CaseSensitivity cs) |
void | setSortLocaleAware (bool on) |
void | setSortRole (int role) |
virtual void | setSourceModel (QAbstractItemModel *sourceModel) override |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &idx) const const override |
virtual void | sort (int column, Qt::SortOrder order) override |
Qt::CaseSensitivity | sortCaseSensitivity () const const |
void | sortCaseSensitivityChanged (Qt::CaseSensitivity sortCaseSensitivity) |
int | sortColumn () const const |
void | sortLocaleAwareChanged (bool sortLocaleAware) |
Qt::SortOrder | sortOrder () const const |
int | sortRole () const const |
void | sortRoleChanged (int sortRole) |
virtual QSize | span (const QModelIndex &index) const const override |
virtual Qt::DropActions | supportedDropActions () const const override |
![]() | |
QAbstractProxyModel (QObject *parent) | |
QBindable< QAbstractItemModel * > | bindableSourceModel () |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const override |
virtual bool | clearItemData (const QModelIndex &index) override |
virtual QMap< int, QVariant > | itemData (const QModelIndex &proxyIndex) const const override |
virtual void | revert () override |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) override |
QAbstractItemModel * | sourceModel () const const |
void | sourceModelChanged () |
virtual bool | submit () override |
virtual Qt::DropActions | supportedDragActions () const const override |
![]() | |
QAbstractItemModel (QObject *parent) | |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
bool | removeColumn (int column, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Protected Member Functions | |
void | classBegin () override |
void | componentComplete () override |
![]() | |
virtual bool | filterAcceptsColumn (int source_column, const QModelIndex &source_parent) const const |
void | invalidateColumnsFilter () |
void | invalidateFilter () |
void | invalidateRowsFilter () |
![]() | |
QModelIndex | createSourceIndex (int row, int col, void *internalPtr) const const |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, const void *ptr) const const |
QModelIndex | createIndex (int row, int column, quintptr id) const const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const const |
virtual void | resetInternalData () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
DoNotUseParent | |
HorizontalSortHint | |
IndexIsValid | |
NoLayoutChangeHint | |
NoOption | |
ParentIsInvalid | |
VerticalSortHint | |
Detailed Description
A model with notifications and jobs.
This model contains application notifications as well as jobs and lets you apply fine-grained filter, sorting, and grouping rules.
Definition at line 29 of file notifications.h.
Member Typedef Documentation
◆ ClearFlags
Definition at line 324 of file notifications.h.
◆ InvokeBehaviors
QFlags< InvokeBehavior > NotificationManager::Notifications::InvokeBehaviors |
Definition at line 362 of file notifications.h.
◆ Urgencies
Definition at line 313 of file notifications.h.
Member Enumeration Documentation
◆ ClearFlag
Which items should be cleared in a call to clear
.
Definition at line 319 of file notifications.h.
◆ GroupMode
The group mode for the model.
Definition at line 350 of file notifications.h.
◆ InvokeBehavior
enum NotificationManager::Notifications::InvokeBehavior |
Definition at line 357 of file notifications.h.
◆ JobState
The state an application job is in.
Definition at line 330 of file notifications.h.
◆ Roles
Enumerator | |
---|---|
IdRole | A notification identifier. This can be uint notification ID or string application job source. |
SummaryRole | The notification summary. |
ImageRole | The notification main image, which is not the application icon. Only valid for pixmap icons. |
IsGroupRole | Whether the item is a group. |
GroupChildrenCountRole | The number of children in a group. |
ExpandedGroupChildrenCountRole | The number of children in a group that are expanded. |
IsGroupExpandedRole | Whether the group is expanded, this role is writable. |
IsInGroupRole | Whether the notification is currently inside a group. |
TypeRole | The type of model entry, either NotificationType or JobType. |
CreatedRole | When the notification was first created. |
UpdatedRole | When the notification was last updated, invalid when it hasn't been updated. |
BodyRole | The notification body text. |
IconNameRole | The notification main icon name, which is not the application icon. Only valid for icon names, if a URL supplied, it is loaded and exposed as ImageRole instead. |
DesktopEntryRole | The desktop entry (without .desktop suffix, e.g. org.kde.spectacle) of the application that sent the notification. |
NotifyRcNameRole | The notifyrc name (e.g. spectaclerc) of the application that sent the notification. |
ApplicationNameRole | The user-visible name of the application (e.g. Spectacle) |
ApplicationIconNameRole | The icon name of the application. |
OriginNameRole | The name of the device or account the notification originally came from, e.g. "My Phone" (in case of device sync) or "foo@example.com" (in case of an email notification) |
JobStateRole | The state of the job, either JobStateJopped, JobStateSuspended, or JobStateRunning. |
PercentageRole | The percentage of the job. Use |
JobErrorRole | The error id of the job, zero in case of no error. |
SuspendableRole | Whether the job can be suspended.
|
KillableRole | Whether the job can be killed/canceled.
|
JobDetailsRole | A pointer to a Job item itself containing more detailed information about the job. |
ActionNamesRole | The IDs of the actions, excluding the default and settings action, e.g. [action1, action2]. |
ActionLabelsRole | The user-visible labels of the actions, excluding the default and settings action, e.g. ["Accept", "Reject"]. |
HasDefaultActionRole | Whether the notification has a default action, which is one that is invoked when the popup itself is clicked. |
DefaultActionLabelRole | The user-visible label of the default action, typically not shown as the popup itself becomes clickable. |
UrlsRole | A list of URLs associated with the notification, e.g. a path to a screenshot that was just taken or image received. |
UrgencyRole | The notification urgency, either LowUrgency, NormalUrgency, or CriticalUrgency. Jobs do not have an urgency. |
TimeoutRole | The timeout for the notification in milliseconds. 0 means the notification should not timeout, -1 means a sensible default should be applied. |
ConfigurableRole | Whether the notification can be configured because a desktopEntry or notifyRcName is known, or the notification has a setting action.
|
ConfigureActionLabelRole | The user-visible label for the settings action. |
ClosableRole | Whether the item can be closed. Notifications are always closable, jobs are only when in JobStateStopped. |
ExpiredRole | The notification timed out and closed. Actions on it cannot be invoked anymore. |
DismissedRole | The notification got temporarily hidden by the user but could still be interacted with. |
ReadRole | Whether the notification got read by the user. If true, the notification isn't considered unread even if created after lastRead.
|
UserActionFeedbackRole | Whether this notification is a response/confirmation to an explicit user action.
|
HasReplyActionRole | Whether the notification has a reply action.
|
ReplyActionLabelRole | The user-visible label for the reply action.
|
ReplyPlaceholderTextRole | A custom placeholder text for the reply action, e.g. "Reply to Max...".
|
ReplySubmitButtonTextRole | A custom text for the reply submit button, e.g. "Submit Comment".
|
ReplySubmitButtonIconNameRole | A custom icon name for the reply submit button.
|
CategoryRole | The (optional) category of the notification. Notifications can optionally have a type indicator. Although neither client or nor server must support this, some may choose to. Those servers implementing categories may use them to intelligently display the notification in a certain way, or group notifications of similar types.
|
ResidentRole | Whether the notification should keep its actions even when they were invoked.
|
TransientRole | Whether the notification is transient and should not be kept in history.
|
Definition at line 220 of file notifications.h.
◆ SortMode
The sort mode for the model.
Definition at line 340 of file notifications.h.
◆ Type
The type of model item.
Enumerator | |
---|---|
NotificationType | This item represents a notification. |
JobType | This item represents an application job. |
Definition at line 294 of file notifications.h.
◆ Urgency
The notification urgency.
- Note
- jobs do not have an urgency, yet still might be above normal urgency notifications.
Definition at line 306 of file notifications.h.
Property Documentation
◆ activeJobsCount
|
read |
The number of active jobs.
Definition at line 201 of file notifications.h.
◆ activeNotificationsCount
|
read |
The number of active, i.e.
non-expired notifications
Definition at line 177 of file notifications.h.
◆ blacklistedDesktopEntries
|
readwrite |
A list of desktop entries for which no notifications should be shown.
If the same desktop entry is present in both blacklist and whitelist, the blacklist takes precedence, i.e. the notification is not shown.
Definition at line 70 of file notifications.h.
◆ blacklistedNotifyRcNames
|
readwrite |
A list of notifyrc names for which no notifications should be shown.
If the same notifyrc name is present in both blacklist and whitelist, the blacklist takes precedence, i.e. the notification is not shown.
Definition at line 78 of file notifications.h.
◆ count
|
read |
The number of notifications in the model.
Definition at line 172 of file notifications.h.
◆ expandUnread
|
readwrite |
Whether to automatically show notifications that are unread.
This is any notification that was created or updated after the value of lastRead
.
Definition at line 167 of file notifications.h.
◆ expiredNotificationsCount
|
read |
The number of inactive, i.e.
non-expired notifications
Definition at line 182 of file notifications.h.
◆ groupLimit
|
readwrite |
How many notifications are shown in each group.
You can expand a group by setting the IsGroupExpandedRole to true.
Default is 0, which means no limit.
Definition at line 160 of file notifications.h.
◆ groupMode
|
readwrite |
The group mode for notifications.
Default is ungrouped.
Definition at line 151 of file notifications.h.
◆ jobsPercentage
|
read |
The combined percentage of all jobs.
This is the average of all percentages and could can be used to show a global progress bar.
Definition at line 208 of file notifications.h.
◆ lastRead
|
readwrite |
The time when the user last could read the notifications.
This is typically reset whenever the list of notifications is opened and is used to determine the unreadNotificationsCount
Definition at line 189 of file notifications.h.
◆ limit
|
readwrite |
The number of notifications the model should at most contain.
Default is 0, which is no limit.
Definition at line 40 of file notifications.h.
◆ showDismissed
|
readwrite |
Whether to show dismissed notifications.
Dismissed notifications are those that are temporarily hidden by the user. This can e.g. be a copy job that has its popup closed but still continues in the background.
Default is false.
Definition at line 62 of file notifications.h.
◆ showExpired
|
readwrite |
Whether to show expired notifications.
Expired notifications are those that timed out, i.e. ones that were not explicitly closed or acted upon by the user, nor revoked by the issuing application.
An expired notification has its actions removed.
Default is false.
Definition at line 52 of file notifications.h.
◆ showJobs
|
readwrite |
◆ showNotifications
|
readwrite |
◆ sortMode
|
readwrite |
The sort mode for notifications.
Default is strictly by date created/updated.
Definition at line 130 of file notifications.h.
◆ sortOrder
|
readwrite |
The sort order for notifications.
This only affects the sort order by date. When sortMode
is set to SortByTypeAndUrgency the order of notification groups (e.g. high - jobs - normal - low) is unaffected, and only notifications within the same group are either sorted ascending or descending by their creation/update date.
Default is DescendingOrder, i.e. newest notifications come first.
- Since
- 5.19
Definition at line 144 of file notifications.h.
◆ unreadNotificationsCount
|
read |
The number of notifications added since lastRead.
This can be used to show a "n unread notifications" label
Definition at line 196 of file notifications.h.
◆ urgencies
|
readwrite |
The notification urgency types the model should contain.
Default is all urgencies: low, normal, critical.
Definition at line 123 of file notifications.h.
◆ whitelistedDesktopEntries
|
readwrite |
A list of desktop entries for which notifications should be shown.
This bypasses any filtering for urgency.
If the same desktop entry is present in both whitelist and blacklist, the blacklist takes precedence, i.e. the notification is not shown.
Default is empty list, which means normal filtering is applied.
Definition at line 90 of file notifications.h.
◆ whitelistedNotifyRcNames
|
readwrite |
A list of notifyrc names for which notifications should be shown.
This bypasses any filtering for urgency.
If the same notifyrc name is present in both whitelist and blacklist, the blacklist takes precedence, i.e. the notification is not shown.
Default is empty list, which means normal filtering is applied.
Definition at line 102 of file notifications.h.
◆ window
|
readwrite |
The window that will render the notifications.
This is used to tell the xdg_activation_v1 protocol who is requesting the activation.
Definition at line 215 of file notifications.h.
Constructor & Destructor Documentation
◆ Notifications()
|
explicit |
Definition at line 370 of file notifications.cpp.
Member Function Documentation
◆ activeJobsCount()
int Notifications::activeJobsCount | ( | ) | const |
Definition at line 640 of file notifications.cpp.
◆ activeNotificationsCount()
int Notifications::activeNotificationsCount | ( | ) | const |
Definition at line 601 of file notifications.cpp.
◆ blacklistedDesktopEntries()
QStringList Notifications::blacklistedDesktopEntries | ( | ) | const |
Definition at line 480 of file notifications.cpp.
◆ blacklistedNotifyRcNames()
QStringList Notifications::blacklistedNotifyRcNames | ( | ) | const |
Definition at line 490 of file notifications.cpp.
◆ classBegin()
|
overrideprotectedvirtual |
Implements QQmlParserStatus.
Definition at line 390 of file notifications.cpp.
◆ clear()
void Notifications::clear | ( | ClearFlags | flags | ) |
Clear notifications.
Removes the notifications matching th ClearFlags from the model. This can be used for e.g. a "Clear History" action.
Definition at line 783 of file notifications.cpp.
◆ close()
void Notifications::close | ( | const QModelIndex & | idx | ) |
Close a notification.
Closes the notification in response to the user explicitly closing it.
When the model index belongs to a group, the entire group is closed.
Definition at line 669 of file notifications.cpp.
◆ collapseAllGroups()
void Notifications::collapseAllGroups | ( | ) |
Definition at line 808 of file notifications.cpp.
◆ componentComplete()
|
overrideprotectedvirtual |
Implements QQmlParserStatus.
Definition at line 394 of file notifications.cpp.
◆ configure()
void Notifications::configure | ( | const QModelIndex & | idx | ) |
Configure a notification.
This will invoke the settings action, if available, otherwise open the kcm_notifications KCM for configuring the respective application and event.
Definition at line 704 of file notifications.cpp.
◆ count()
int Notifications::count | ( | ) | const |
Definition at line 596 of file notifications.cpp.
◆ data()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 815 of file notifications.cpp.
◆ expandUnread()
bool Notifications::expandUnread | ( | ) | const |
Definition at line 428 of file notifications.cpp.
◆ expire()
void Notifications::expire | ( | const QModelIndex & | idx | ) |
Expire a notification.
Closes the notification in response to its timeout running out.
Call this if you have an implementation that handles the timeout itself by having called stopTimeout
- See also
- stopTimeout
Definition at line 655 of file notifications.cpp.
◆ expiredNotificationsCount()
int Notifications::expiredNotificationsCount | ( | ) | const |
Definition at line 606 of file notifications.cpp.
◆ filterAcceptsRow()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 825 of file notifications.cpp.
◆ groupIndex()
QModelIndex Notifications::groupIndex | ( | const QModelIndex & | idx | ) | const |
Returns a model index pointing to the group of a notification.
Definition at line 793 of file notifications.cpp.
◆ groupLimit()
int Notifications::groupLimit | ( | ) | const |
Definition at line 410 of file notifications.cpp.
◆ groupMode()
Notifications::GroupMode Notifications::groupMode | ( | ) | const |
Definition at line 582 of file notifications.cpp.
◆ invokeAction()
void Notifications::invokeAction | ( | const QModelIndex & | idx, |
const QString & | actionId, | ||
InvokeBehavior | behavior = None ) |
Invoke a notification action.
Invokes the action with the given actionId on the notification. For invoking the default action, i.e. the one that is triggered when clicking the notification bubble, use invokeDefaultAction
Definition at line 729 of file notifications.cpp.
◆ invokeDefaultAction()
void Notifications::invokeDefaultAction | ( | const QModelIndex & | idx, |
InvokeBehavior | behavior = None ) |
Invoke the default notification action.
Invokes the action that should be triggered when clicking the notification bubble itself.
Definition at line 722 of file notifications.cpp.
◆ jobsPercentage()
int Notifications::jobsPercentage | ( | ) | const |
Definition at line 645 of file notifications.cpp.
◆ killJob()
void Notifications::killJob | ( | const QModelIndex & | idx | ) |
Kill a job.
Definition at line 776 of file notifications.cpp.
◆ lastRead()
QDateTime Notifications::lastRead | ( | ) | const |
Definition at line 611 of file notifications.cpp.
◆ lessThan()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 830 of file notifications.cpp.
◆ limit()
int Notifications::limit | ( | ) | const |
Definition at line 400 of file notifications.cpp.
◆ makePersistentModelIndex()
QPersistentModelIndex Notifications::makePersistentModelIndex | ( | const QModelIndex & | idx | ) | const |
Convert the given QModelIndex into a QPersistentModelIndex.
Definition at line 650 of file notifications.cpp.
◆ reply()
void Notifications::reply | ( | const QModelIndex & | idx, |
const QString & | text, | ||
InvokeBehavior | behavior ) |
Reply to a notification.
Replies to the given notification with the given text.
- Since
- 5.18
Definition at line 736 of file notifications.cpp.
◆ resetLastRead()
void Notifications::resetLastRead | ( | ) |
Definition at line 630 of file notifications.cpp.
◆ resumeJob()
void Notifications::resumeJob | ( | const QModelIndex & | idx | ) |
Resume a job.
Definition at line 769 of file notifications.cpp.
◆ roleNames()
|
overridevirtual |
Reimplemented from QAbstractProxyModel.
Definition at line 840 of file notifications.cpp.
◆ rowCount()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 835 of file notifications.cpp.
◆ setBlacklistedDesktopEntries()
void Notifications::setBlacklistedDesktopEntries | ( | const QStringList & | blacklist | ) |
Definition at line 485 of file notifications.cpp.
◆ setBlacklistedNotifyRcNames()
void Notifications::setBlacklistedNotifyRcNames | ( | const QStringList & | blacklist | ) |
Definition at line 495 of file notifications.cpp.
◆ setData()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 820 of file notifications.cpp.
◆ setExpandUnread()
void Notifications::setExpandUnread | ( | bool | expand | ) |
Definition at line 433 of file notifications.cpp.
◆ setGroupLimit()
void Notifications::setGroupLimit | ( | int | limit | ) |
Definition at line 415 of file notifications.cpp.
◆ setGroupMode()
void Notifications::setGroupMode | ( | GroupMode | groupMode | ) |
Definition at line 587 of file notifications.cpp.
◆ setLastRead()
void Notifications::setLastRead | ( | const QDateTime & | lastRead | ) |
Definition at line 619 of file notifications.cpp.
◆ setLimit()
void Notifications::setLimit | ( | int | limit | ) |
Definition at line 405 of file notifications.cpp.
◆ setShowDismissed()
void Notifications::setShowDismissed | ( | bool | show | ) |
Definition at line 475 of file notifications.cpp.
◆ setShowExpired()
void Notifications::setShowExpired | ( | bool | show | ) |
Definition at line 465 of file notifications.cpp.
◆ setShowJobs()
void Notifications::setShowJobs | ( | bool | showJobs | ) |
Definition at line 541 of file notifications.cpp.
◆ setShowNotifications()
void Notifications::setShowNotifications | ( | bool | showNotifications | ) |
Definition at line 525 of file notifications.cpp.
◆ setSortMode()
void Notifications::setSortMode | ( | SortMode | sortMode | ) |
Definition at line 567 of file notifications.cpp.
◆ setSortOrder()
void Notifications::setSortOrder | ( | Qt::SortOrder | sortOrder | ) |
Definition at line 577 of file notifications.cpp.
◆ setUrgencies()
void Notifications::setUrgencies | ( | Urgencies | urgencies | ) |
Definition at line 557 of file notifications.cpp.
◆ setWhitelistedDesktopEntries()
void Notifications::setWhitelistedDesktopEntries | ( | const QStringList & | whitelist | ) |
Definition at line 505 of file notifications.cpp.
◆ setWhitelistedNotifyRcNames()
void Notifications::setWhitelistedNotifyRcNames | ( | const QStringList & | whitelist | ) |
Definition at line 515 of file notifications.cpp.
◆ setWindow()
void Notifications::setWindow | ( | QWindow * | window | ) |
Definition at line 451 of file notifications.cpp.
◆ showDismissed()
bool Notifications::showDismissed | ( | ) | const |
Definition at line 470 of file notifications.cpp.
◆ showExpired()
bool Notifications::showExpired | ( | ) | const |
Definition at line 460 of file notifications.cpp.
◆ showJobs()
bool Notifications::showJobs | ( | ) | const |
Definition at line 536 of file notifications.cpp.
◆ showNotifications()
bool Notifications::showNotifications | ( | ) | const |
Definition at line 520 of file notifications.cpp.
◆ sortMode()
Notifications::SortMode Notifications::sortMode | ( | ) | const |
Definition at line 562 of file notifications.cpp.
◆ sortOrder()
Qt::SortOrder Notifications::sortOrder | ( | ) | const |
Definition at line 572 of file notifications.cpp.
◆ startTimeout() [1/2]
void Notifications::startTimeout | ( | const QModelIndex & | idx | ) |
Start automatic timeout of notifications.
Call this if you no longer handle the timeout yourself.
- See also
- stopTimeout
Definition at line 743 of file notifications.cpp.
◆ startTimeout() [2/2]
void Notifications::startTimeout | ( | uint | notificationId | ) |
Definition at line 748 of file notifications.cpp.
◆ stopTimeout()
void Notifications::stopTimeout | ( | const QModelIndex & | idx | ) |
Stop the automatic timeout of notifications.
Call this if you have an implementation that handles the timeout itself taking into account e.g. whether the user is currently interacting with the notification to not close it under their mouse. Call expire
once your custom timer has run out.
- See also
- expire
Definition at line 755 of file notifications.cpp.
◆ suspendJob()
void Notifications::suspendJob | ( | const QModelIndex & | idx | ) |
Suspend a job.
Definition at line 762 of file notifications.cpp.
◆ unreadNotificationsCount()
int Notifications::unreadNotificationsCount | ( | ) | const |
Definition at line 635 of file notifications.cpp.
◆ urgencies()
Notifications::Urgencies Notifications::urgencies | ( | ) | const |
Definition at line 552 of file notifications.cpp.
◆ whitelistedDesktopEntries()
QStringList Notifications::whitelistedDesktopEntries | ( | ) | const |
Definition at line 500 of file notifications.cpp.
◆ whitelistedNotifyRcNames()
QStringList Notifications::whitelistedNotifyRcNames | ( | ) | const |
Definition at line 510 of file notifications.cpp.
◆ window()
QWindow * Notifications::window | ( | ) | const |
Definition at line 446 of file notifications.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:55:13 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.