TaskManager::TasksModel
#include <tasksmodel.h>
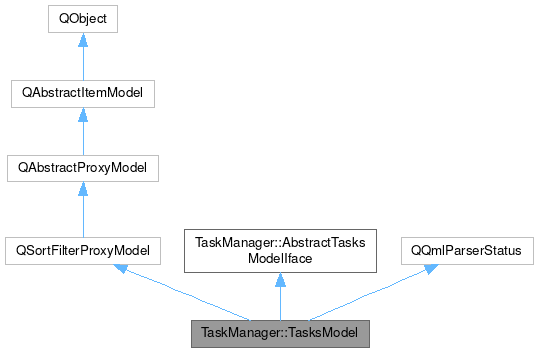
Public Types | |
enum | GroupMode { GroupDisabled = 0 , GroupApplications } |
enum | SortMode { SortDisabled = 0 , SortManual , SortAlpha , SortVirtualDesktop , SortActivity , SortLastActivated } |
![]() | |
enum | CheckIndexOption |
enum | LayoutChangeHint |
Public Member Functions | |
TasksModel (QObject *parent=nullptr) | |
QModelIndex | activeTask () const |
QString | activity () const |
bool | anyTaskDemandsAttention () const |
void | classBegin () override |
void | componentComplete () override |
QVariant | data (const QModelIndex &proxyIndex, int role) const override |
bool | filterByActivity () const |
RegionFilterMode::Mode | filterByRegion () const |
bool | filterByScreen () const |
bool | filterByVirtualDesktop () const |
bool | filterHidden () const |
bool | filterMinimized () const |
bool | filterNotMaximized () const |
bool | filterNotMinimized () const |
QStringList | groupingAppIdBlacklist () const |
QStringList | groupingLauncherUrlBlacklist () const |
int | groupingWindowTasksThreshold () const |
bool | groupInline () const |
TasksModel::GroupMode | groupMode () const |
bool | hideActivatedLaunchers () const |
Q_INVOKABLE QStringList | launcherActivities (const QUrl &url) |
int | launcherCount () const |
QStringList | launcherList () const |
Q_INVOKABLE int | launcherPosition (const QUrl &url) const |
bool | launchInPlace () const |
Q_INVOKABLE QModelIndex | makeModelIndex (int row, int childRow=-1) const |
Q_INVOKABLE QPersistentModelIndex | makePersistentModelIndex (int row, int childRow=-1) const |
Q_INVOKABLE bool | move (int row, int newPos, const QModelIndex &parent=QModelIndex()) |
QRect | regionGeometry () const |
Q_INVOKABLE void | requestActivate (const QModelIndex &index) override |
Q_INVOKABLE void | requestActivities (const QModelIndex &index, const QStringList &activities) override |
Q_INVOKABLE bool | requestAddLauncher (const QUrl &url) |
Q_INVOKABLE bool | requestAddLauncherToActivity (const QUrl &url, const QString &activity) |
Q_INVOKABLE void | requestClose (const QModelIndex &index) override |
Q_INVOKABLE void | requestMove (const QModelIndex &index) override |
Q_INVOKABLE void | requestNewInstance (const QModelIndex &index) override |
Q_INVOKABLE void | requestNewVirtualDesktop (const QModelIndex &index) override |
Q_INVOKABLE void | requestOpenUrls (const QModelIndex &index, const QList< QUrl > &urls) override |
Q_INVOKABLE void | requestPublishDelegateGeometry (const QModelIndex &index, const QRect &geometry, QObject *delegate=nullptr) override |
Q_INVOKABLE bool | requestRemoveLauncher (const QUrl &url) |
Q_INVOKABLE bool | requestRemoveLauncherFromActivity (const QUrl &url, const QString &activity) |
Q_INVOKABLE void | requestResize (const QModelIndex &index) override |
Q_INVOKABLE void | requestToggleFullScreen (const QModelIndex &index) override |
Q_INVOKABLE void | requestToggleGrouping (const QModelIndex &index) |
Q_INVOKABLE void | requestToggleKeepAbove (const QModelIndex &index) override |
Q_INVOKABLE void | requestToggleKeepBelow (const QModelIndex &index) override |
Q_INVOKABLE void | requestToggleMaximized (const QModelIndex &index) override |
Q_INVOKABLE void | requestToggleMinimized (const QModelIndex &index) override |
Q_INVOKABLE void | requestToggleShaded (const QModelIndex &index) override |
Q_INVOKABLE void | requestVirtualDesktops (const QModelIndex &index, const QVariantList &desktops) override |
QHash< int, QByteArray > | roleNames () const override |
Q_INVOKABLE int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
QRect | screenGeometry () const |
bool | separateLaunchers () const |
void | setActivity (const QString &activity) |
void | setFilterByActivity (bool filter) |
void | setFilterByRegion (RegionFilterMode::Mode mode) |
void | setFilterByScreen (bool filter) |
void | setFilterByVirtualDesktop (bool filter) |
void | setFilterHidden (bool filter) |
void | setFilterMinimized (bool filter) |
void | setFilterNotMaximized (bool filter) |
void | setFilterNotMinimized (bool filter) |
void | setGroupingAppIdBlacklist (const QStringList &list) |
void | setGroupingLauncherUrlBlacklist (const QStringList &list) |
void | setGroupingWindowTasksThreshold (int threshold) |
void | setGroupInline (bool groupInline) |
void | setGroupMode (TasksModel::GroupMode mode) |
void | setHideActivatedLaunchers (bool hideActivatedLaunchers) |
void | setLauncherList (const QStringList &launchers) |
void | setLaunchInPlace (bool launchInPlace) |
void | setRegionGeometry (const QRect &geometry) |
void | setScreenGeometry (const QRect &geometry) |
void | setSeparateLaunchers (bool separate) |
void | setSortMode (SortMode mode) |
void | setTaskReorderingEnabled (bool enabled) |
void | setVirtualDesktop (const QVariant &desktop=QVariant()) |
SortMode | sortMode () const |
Q_INVOKABLE void | syncLaunchers () |
bool | taskReorderingEnabled () const |
QVariant | virtualDesktop () const |
![]() | |
QSortFilterProxyModel (QObject *parent) | |
bool | autoAcceptChildRows () const const |
void | autoAcceptChildRowsChanged (bool autoAcceptChildRows) |
QBindable< bool > | bindableAutoAcceptChildRows () |
QBindable< bool > | bindableDynamicSortFilter () |
QBindable< Qt::CaseSensitivity > | bindableFilterCaseSensitivity () |
QBindable< int > | bindableFilterKeyColumn () |
QBindable< QRegularExpression > | bindableFilterRegularExpression () |
QBindable< int > | bindableFilterRole () |
QBindable< bool > | bindableIsSortLocaleAware () |
QBindable< bool > | bindableRecursiveFilteringEnabled () |
QBindable< Qt::CaseSensitivity > | bindableSortCaseSensitivity () |
QBindable< int > | bindableSortRole () |
virtual QModelIndex | buddy (const QModelIndex &index) const const override |
virtual bool | canFetchMore (const QModelIndex &parent) const const override |
virtual int | columnCount (const QModelIndex &parent) const const override |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) override |
bool | dynamicSortFilter () const const |
virtual void | fetchMore (const QModelIndex &parent) override |
Qt::CaseSensitivity | filterCaseSensitivity () const const |
void | filterCaseSensitivityChanged (Qt::CaseSensitivity filterCaseSensitivity) |
int | filterKeyColumn () const const |
QRegularExpression | filterRegularExpression () const const |
int | filterRole () const const |
void | filterRoleChanged (int filterRole) |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const const override |
virtual bool | hasChildren (const QModelIndex &parent) const const override |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const const override |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent) const const override |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) override |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) override |
void | invalidate () |
bool | isRecursiveFilteringEnabled () const const |
bool | isSortLocaleAware () const const |
virtual QModelIndex | mapFromSource (const QModelIndex &sourceIndex) const const override |
virtual QItemSelection | mapSelectionFromSource (const QItemSelection &sourceSelection) const const override |
virtual QItemSelection | mapSelectionToSource (const QItemSelection &proxySelection) const const override |
virtual QModelIndex | mapToSource (const QModelIndex &proxyIndex) const const override |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, Qt::MatchFlags flags) const const override |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const const override |
virtual QStringList | mimeTypes () const const override |
virtual QModelIndex | parent (const QModelIndex &child) const const override |
void | recursiveFilteringEnabledChanged (bool recursiveFilteringEnabled) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) override |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) override |
void | setAutoAcceptChildRows (bool accept) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role) override |
void | setDynamicSortFilter (bool enable) |
void | setFilterCaseSensitivity (Qt::CaseSensitivity cs) |
void | setFilterFixedString (const QString &pattern) |
void | setFilterKeyColumn (int column) |
void | setFilterRegularExpression (const QRegularExpression ®ularExpression) |
void | setFilterRegularExpression (const QString &pattern) |
void | setFilterRole (int role) |
void | setFilterWildcard (const QString &pattern) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) override |
void | setRecursiveFilteringEnabled (bool recursive) |
void | setSortCaseSensitivity (Qt::CaseSensitivity cs) |
void | setSortLocaleAware (bool on) |
void | setSortRole (int role) |
virtual void | setSourceModel (QAbstractItemModel *sourceModel) override |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &idx) const const override |
virtual void | sort (int column, Qt::SortOrder order) override |
Qt::CaseSensitivity | sortCaseSensitivity () const const |
void | sortCaseSensitivityChanged (Qt::CaseSensitivity sortCaseSensitivity) |
int | sortColumn () const const |
void | sortLocaleAwareChanged (bool sortLocaleAware) |
Qt::SortOrder | sortOrder () const const |
int | sortRole () const const |
void | sortRoleChanged (int sortRole) |
virtual QSize | span (const QModelIndex &index) const const override |
virtual Qt::DropActions | supportedDropActions () const const override |
![]() | |
QAbstractProxyModel (QObject *parent) | |
QBindable< QAbstractItemModel * > | bindableSourceModel () |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const override |
virtual bool | clearItemData (const QModelIndex &index) override |
virtual QMap< int, QVariant > | itemData (const QModelIndex &proxyIndex) const const override |
virtual void | revert () override |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) override |
QAbstractItemModel * | sourceModel () const const |
void | sourceModelChanged () |
virtual bool | submit () override |
virtual Qt::DropActions | supportedDragActions () const const override |
![]() | |
QAbstractItemModel (QObject *parent) | |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
bool | removeColumn (int column, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
std::shared_ptr< ActivityInfo > | activityInfo () const |
bool | filterAcceptsRow (int sourceRow, const QModelIndex &sourceParent) const override |
bool | lessThan (const QModelIndex &left, const QModelIndex &right) const override |
std::shared_ptr< VirtualDesktopInfo > | virtualDesktopInfo () const |
![]() | |
virtual bool | filterAcceptsColumn (int source_column, const QModelIndex &source_parent) const const |
void | invalidateColumnsFilter () |
void | invalidateFilter () |
void | invalidateRowsFilter () |
![]() | |
QModelIndex | createSourceIndex (int row, int col, void *internalPtr) const const |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, const void *ptr) const const |
QModelIndex | createIndex (int row, int column, quintptr id) const const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const const |
virtual void | resetInternalData () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | CheckIndexOptions |
DoNotUseParent | |
HorizontalSortHint | |
IndexIsValid | |
NoLayoutChangeHint | |
NoOption | |
ParentIsInvalid | |
VerticalSortHint | |
![]() | |
typedef | QObjectList |
Detailed Description
A unified tasks model.
This model presents tasks sourced from supplied launcher URLs, startup notification data and window data retrieved from the windowing server the host process is connected to. The underlying windowing system is abstracted away.
The source data is abstracted into a unified lifecycle for tasks suitable for presentation in a user interface.
Matching startup and window tasks replace launcher tasks. Startup tasks are omitted when matching window tasks exist. Tasks that desire not to be shown in a user interface are omitted.
Tasks may be filtered, sorted or grouped by setting properties on the model.
Tasks may be interacted with by calling methods on the model.
Definition at line 47 of file tasksmodel.h.
Member Enumeration Documentation
◆ GroupMode
Enumerator | |
---|---|
GroupDisabled | No grouping is done. |
GroupApplications | Tasks are grouped by the application backing them. |
Definition at line 100 of file tasksmodel.h.
◆ SortMode
Enumerator | |
---|---|
SortDisabled | No sorting is done. |
SortManual | Tasks can be moved with move() and syncLaunchers(). |
SortAlpha | Tasks are sorted alphabetically, by AbstractTasksModel::AppName and Qt::DisplayRole. |
SortVirtualDesktop | Tasks are sorted by the virtual desktop they are on. |
SortActivity | Tasks are sorted by the number of tasks on the activities they're on. |
SortLastActivated | Tasks are sorted by the last time they were active. |
Definition at line 90 of file tasksmodel.h.
Property Documentation
◆ activeTask
|
read |
Definition at line 87 of file tasksmodel.h.
◆ activity
|
readwrite |
Definition at line 63 of file tasksmodel.h.
◆ anyTaskDemandsAttention
|
read |
Definition at line 58 of file tasksmodel.h.
◆ count
|
read |
Definition at line 53 of file tasksmodel.h.
◆ filterByActivity
|
readwrite |
Definition at line 67 of file tasksmodel.h.
◆ filterByRegion
|
readwrite |
Definition at line 68 of file tasksmodel.h.
◆ filterByScreen
|
readwrite |
Definition at line 66 of file tasksmodel.h.
◆ filterByVirtualDesktop
|
readwrite |
Definition at line 65 of file tasksmodel.h.
◆ filterHidden
|
readwrite |
Definition at line 72 of file tasksmodel.h.
◆ filterMinimized
|
readwrite |
Definition at line 69 of file tasksmodel.h.
◆ filterNotMaximized
|
readwrite |
Definition at line 71 of file tasksmodel.h.
◆ filterNotMinimized
|
readwrite |
Definition at line 70 of file tasksmodel.h.
◆ groupingAppIdBlacklist
|
readwrite |
Definition at line 83 of file tasksmodel.h.
◆ groupingLauncherUrlBlacklist
|
readwrite |
Definition at line 84 of file tasksmodel.h.
◆ groupingWindowTasksThreshold
|
readwrite |
Definition at line 81 of file tasksmodel.h.
◆ groupInline
|
readwrite |
Definition at line 80 of file tasksmodel.h.
◆ groupMode
|
readwrite |
Definition at line 79 of file tasksmodel.h.
◆ hideActivatedLaunchers
|
readwrite |
Definition at line 77 of file tasksmodel.h.
◆ launcherCount
|
read |
Definition at line 54 of file tasksmodel.h.
◆ launcherList
|
readwrite |
Definition at line 56 of file tasksmodel.h.
◆ launchInPlace
|
readwrite |
Definition at line 76 of file tasksmodel.h.
◆ regionGeometry
|
readwrite |
Definition at line 62 of file tasksmodel.h.
◆ screenGeometry
|
readwrite |
Definition at line 61 of file tasksmodel.h.
◆ separateLaunchers
|
readwrite |
Definition at line 75 of file tasksmodel.h.
◆ sortMode
|
readwrite |
Definition at line 74 of file tasksmodel.h.
◆ taskReorderingEnabled
|
readwrite |
Definition at line 86 of file tasksmodel.h.
◆ virtualDesktop
|
readwrite |
Definition at line 60 of file tasksmodel.h.
Constructor & Destructor Documentation
◆ TasksModel()
|
explicit |
Definition at line 969 of file tasksmodel.cpp.
◆ ~TasksModel()
|
override |
Definition at line 993 of file tasksmodel.cpp.
Member Function Documentation
◆ activeTask()
QModelIndex TaskManager::TasksModel::activeTask | ( | ) | const |
Finds the first active (AbstractTasksModel::IsActive) task in the model and returns its QModelIndex, or a null QModelIndex if no active task is found.
- Returns
- the model index for the first active task, if any.
Definition at line 1916 of file tasksmodel.cpp.
◆ activity()
QString TaskManager::TasksModel::activity | ( | ) | const |
The id of the activity used in filtering by activity.
Usually set to the id of the current activity. Defaults to an empty id.
- See also
- setActivity
- Returns
- the id of the activity used in filtering.
Definition at line 1103 of file tasksmodel.cpp.
◆ activityInfo()
|
protected |
- Returns
- a shared pointer to ActivityInfo to access activity information
Definition at line 2050 of file tasksmodel.cpp.
◆ anyTaskDemandsAttention()
bool TaskManager::TasksModel::anyTaskDemandsAttention | ( | ) | const |
Returns whether any task in the model currently demands attention (AbstractTasksModel::IsDemandingAttention).
- Returns
- whether any task in the model currently demands attention.
Definition at line 1068 of file tasksmodel.cpp.
◆ classBegin()
|
overridevirtual |
Implements QQmlParserStatus.
Definition at line 1963 of file tasksmodel.cpp.
◆ componentComplete()
|
overridevirtual |
Implements QQmlParserStatus.
Definition at line 1968 of file tasksmodel.cpp.
◆ data()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 1011 of file tasksmodel.cpp.
◆ filterAcceptsRow()
|
overrideprotectedvirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 1976 of file tasksmodel.cpp.
◆ filterByActivity()
bool TaskManager::TasksModel::filterByActivity | ( | ) | const |
Whether tasks should be filtered by activity.
Defaults to false
.
Filtering by activity only happens if an activity id is set, even if this returns true
.
- See also
- setFilterByActivity
- setActivity
- Returns
true
if tasks should be filtered by activity.
Definition at line 1133 of file tasksmodel.cpp.
◆ filterByRegion()
RegionFilterMode::Mode TaskManager::TasksModel::filterByRegion | ( | ) | const |
Whether tasks should be filtered by region.
Defaults to RegionFilterMode::Disabled
.
Filtering by region only happens if a region is set, even if the filter is enabled.
- See also
- RegionFilterMode
- setFilterByRegion
- setRegionGeometry
- Since
- 6.0
- Returns
- Region filter mode.
Definition at line 1143 of file tasksmodel.cpp.
◆ filterByScreen()
bool TaskManager::TasksModel::filterByScreen | ( | ) | const |
Whether tasks should be filtered by screen.
Defaults to false
.
Filtering by screen only happens if a screen number is set, even if this returns true
.
- See also
- setFilterByScreen
- setScreenGeometry
- Returns
true
if tasks should be filtered by screen.
Definition at line 1123 of file tasksmodel.cpp.
◆ filterByVirtualDesktop()
bool TaskManager::TasksModel::filterByVirtualDesktop | ( | ) | const |
Whether tasks should be filtered by virtual desktop.
Defaults to false
.
Filtering by virtual desktop only happens if a virtual desktop number is set, even if this returns true
.
- Returns
true
if tasks should be filtered by virtual desktop.
Definition at line 1113 of file tasksmodel.cpp.
◆ filterHidden()
bool TaskManager::TasksModel::filterHidden | ( | ) | const |
Whether hidden tasks should be filtered.
Defaults to false
.
- See also
- setFilterHidden
- Returns
true
if hidden tasks should be filtered.
Definition at line 1183 of file tasksmodel.cpp.
◆ filterMinimized()
bool TaskManager::TasksModel::filterMinimized | ( | ) | const |
Whether minimized tasks should be filtered out.
Defaults to false
.
- Returns
true
if minimized tasks should be filtered out.
- See also
- setFilterMinimized
- Since
- 5.27
Definition at line 1153 of file tasksmodel.cpp.
◆ filterNotMaximized()
bool TaskManager::TasksModel::filterNotMaximized | ( | ) | const |
Whether non-maximized tasks should be filtered.
Defaults to false
.
- See also
- setFilterNotMaximized
- Returns
true
if non-maximized tasks should be filtered.
Definition at line 1173 of file tasksmodel.cpp.
◆ filterNotMinimized()
bool TaskManager::TasksModel::filterNotMinimized | ( | ) | const |
Whether non-minimized tasks should be filtered.
Defaults to false
.
- See also
- setFilterNotMinimized
- Returns
true
if non-minimized tasks should be filtered.
Definition at line 1163 of file tasksmodel.cpp.
◆ groupingAppIdBlacklist()
QStringList TaskManager::TasksModel::groupingAppIdBlacklist | ( | ) | const |
A blacklist of app ids (AbstractTasksModel::AppId) that is consulted before grouping a task.
If a task's app id is found on the blacklist, it is not grouped.
The default app id blacklist is empty.
- See also
- setGroupingAppIdBlacklist
- Returns
- the blacklist of app ids consulted before grouping a task.
Definition at line 1345 of file tasksmodel.cpp.
◆ groupingLauncherUrlBlacklist()
QStringList TaskManager::TasksModel::groupingLauncherUrlBlacklist | ( | ) | const |
A blacklist of launcher URLs (AbstractTasksModel::LauncherUrl) that is consulted before grouping a task.
If a task's launcher URL is found on the blacklist, it is not grouped.
The default launcher URL blacklist is empty.
- See also
- setGroupingLauncherUrlBlacklist
- Returns
- the blacklist of launcher URLs consulted before grouping a task.
Definition at line 1361 of file tasksmodel.cpp.
◆ groupingWindowTasksThreshold()
int TaskManager::TasksModel::groupingWindowTasksThreshold | ( | ) | const |
As window tasks (AbstractTasksModel::IsWindow) come and go, groups will be formed when this threshold value is exceeded, and broken apart when it matches or falls below.
Defaults to -1
, which means grouping is done regardless of the number of window tasks.
When the groupInline property is set to true
, the threshold is ignored: Grouping is always done.
- See also
- setGroupingWindowTasksThreshold
- groupInline
- Returns
- the threshold number of window tasks used in grouping decisions.
Definition at line 1327 of file tasksmodel.cpp.
◆ groupInline()
bool TaskManager::TasksModel::groupInline | ( | ) | const |
Returns whether grouping is done "inline" or not, i.e.
whether groups are maintained inside the flat, top-level list, or by forming a tree. In inline grouping mode, move() on a group member will move all siblings as well, and sorting is first done among groups, then group members.
Further, in inline grouping mode, the groupingWindowTasksThreshold setting is ignored: Grouping is always done.
- See also
- setGroupInline
- move
- groupingWindowTasksThreshold
- Returns
- whether grouping is done inline or not.
Definition at line 1311 of file tasksmodel.cpp.
◆ groupMode()
TasksModel::GroupMode TaskManager::TasksModel::groupMode | ( | ) | const |
Returns the current group mode, i.e.
the criteria by which tasks should be grouped.
Defaults to TasksModel::GroupApplication, which groups tasks backed by the same application.
If the group mode is TasksModel::GroupDisabled, no grouping is done.
- See also
- setGroupMode
- Returns
- the current group mode.
Definition at line 1272 of file tasksmodel.cpp.
◆ hideActivatedLaunchers()
bool TaskManager::TasksModel::hideActivatedLaunchers | ( | ) | const |
Whether launchers should be hidden after they have been activated.
Defaults to true
.
- See also
- hideActivatedLaunchers
- Returns
- whether launchers will be hidden after they have been activated.
Definition at line 1281 of file tasksmodel.cpp.
◆ launcherActivities()
QStringList TaskManager::TasksModel::launcherActivities | ( | const QUrl & | url | ) |
Return the list of activities the launcher belongs to.
If there is no launcher with that url, the list will be empty, while if the launcher is on all activities, it will contain a null uuid.
URLs are compared for equality after removing the query string used to hold metadata.
Definition at line 1477 of file tasksmodel.cpp.
◆ launcherCount()
int TaskManager::TasksModel::launcherCount | ( | ) | const |
The number of launcher tasks in the tast list.
- Returns
- the number of launcher tasks in the task list.
Definition at line 1063 of file tasksmodel.cpp.
◆ launcherList()
QStringList TaskManager::TasksModel::launcherList | ( | ) | const |
The list of launcher URLs serialized to strings along with the activities they belong to.
- See also
- setLauncherList
- Returns
- the list of launcher URLs serialized to strings.
Definition at line 1389 of file tasksmodel.cpp.
◆ launcherPosition()
int TaskManager::TasksModel::launcherPosition | ( | const QUrl & | url | ) | const |
Return the position of the launcher with the given URL.
URLs are compared for equality after removing the query string used to hold metadata.
- See also
- launcherUrlsMatch
- Parameters
-
url A launcher URL.
- Returns
-1
if no launcher exists for the given URL.
Definition at line 1486 of file tasksmodel.cpp.
◆ launchInPlace()
bool TaskManager::TasksModel::launchInPlace | ( | ) | const |
Whether window tasks should be sorted as their associated launcher tasks or separately.
Defaults to false
.
- See also
- setLaunchInPlace
- Returns
- whether window tasks should be sorted as their associated launcher tasks.
Definition at line 1256 of file tasksmodel.cpp.
◆ lessThan()
|
overrideprotectedvirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 2029 of file tasksmodel.cpp.
◆ makeModelIndex()
QModelIndex TaskManager::TasksModel::makeModelIndex | ( | int | row, |
int | childRow = -1 ) const |
Given a row in the model, returns a QModelIndex for it.
To get an index for a child in a task group, an optional child row may be passed as well.
This easier to use from Qt Quick views than QAbstractItemModel::index is.
- Parameters
-
row A row index in the model. childRow A row index for a child of the task group at the given row.
- Returns
- a model index for the task at the given row, or for one of its child tasks.
Definition at line 1939 of file tasksmodel.cpp.
◆ makePersistentModelIndex()
QPersistentModelIndex TaskManager::TasksModel::makePersistentModelIndex | ( | int | row, |
int | childRow = -1 ) const |
Given a row in the model, returns a QPersistentModelIndex for it.
To get an index for a child in a task group, an optional child row may be passed as well.
- Parameters
-
row A row index in the model. childRow A row index for a child of the task group at the given row.
- Returns
- a model index for the task at the given row, or for one of its child tasks.
Definition at line 1958 of file tasksmodel.cpp.
◆ move()
bool TaskManager::TasksModel::move | ( | int | row, |
int | newPos, | ||
const QModelIndex & | parent = QModelIndex() ) |
Moves a task to a new position in the list.
The insert position is is bounded to the list start and end.
syncLaunchers() should be called after a set of move operations to update the launcherList property to reflect the new order.
When the groupInline property is set to true
, a move request for a group member will bring all siblings along.
- See also
- syncLaunchers
- launcherList
- setGroupInline
- Parameters
-
index An index in this tasks model. newPos The new list position to move the task to.
Definition at line 1616 of file tasksmodel.cpp.
◆ regionGeometry()
QRect TaskManager::TasksModel::regionGeometry | ( | ) | const |
The geometry of the region used in filtering by region.
Defaults to a null QRect.
- See also
- setRegionGeometry
- Since
- 6.0
- Returns
- the geometry of the region used in filtering.
Definition at line 1093 of file tasksmodel.cpp.
◆ requestActivate()
|
overridevirtual |
Request activation of the task at the given index.
Derived classes are free to interpret the meaning of "activate" themselves depending on the nature and state of the task, e.g. launch or raise a window task.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1495 of file tasksmodel.cpp.
◆ requestActivities()
|
overridevirtual |
Request moving the task at the given index to the specified activities.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
This base implementation does nothing.
- Parameters
-
index An index in this tasks model. activities The new list of activities.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1593 of file tasksmodel.cpp.
◆ requestAddLauncher()
bool TaskManager::TasksModel::requestAddLauncher | ( | const QUrl & | url | ) |
Request adding a launcher with the given URL.
If this URL is already in the list, the request will fail. URLs are compared for equality after removing the query string used to hold metadata.
- See also
- launcherUrlsMatch
- Parameters
-
url A launcher URL.
- Returns
true
if a launcher was added.
Definition at line 1405 of file tasksmodel.cpp.
◆ requestAddLauncherToActivity()
bool TaskManager::TasksModel::requestAddLauncherToActivity | ( | const QUrl & | url, |
const QString & | activity ) |
Request adding a launcher with the given URL to current activity.
If this URL is already in the list, the request will fail. URLs are compared for equality after removing the query string used to hold metadata.
- See also
- launcherUrlsMatch
- Parameters
-
url A launcher URL.
- Returns
true
if a launcher was added.
Definition at line 1441 of file tasksmodel.cpp.
◆ requestClose()
|
overridevirtual |
Request the task at the given index be closed.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1516 of file tasksmodel.cpp.
◆ requestMove()
|
overridevirtual |
Request starting an interactive move for the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1523 of file tasksmodel.cpp.
◆ requestNewInstance()
|
overridevirtual |
Request an additional instance of the application backing the task at the given index.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1502 of file tasksmodel.cpp.
◆ requestNewVirtualDesktop()
|
overridevirtual |
Request entering the window at the given index on a new virtual desktop, which is created in response to this request.
- Parameters
-
index An index in this window tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1586 of file tasksmodel.cpp.
◆ requestOpenUrls()
|
overridevirtual |
Requests to open the given URLs with the application backing the task at the given index.
- Parameters
-
index An index in this tasks model. urls The URLs to be passed to the application.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1509 of file tasksmodel.cpp.
◆ requestPublishDelegateGeometry()
|
overridevirtual |
Request informing the window manager of new geometry for a visual delegate for the task at the given index.
The geometry should be in screen coordinates.
If the task at the given index is a group parent, the geometry is set for all of its children. If the task at the given index is a group member, the geometry is set for all of its siblings.
- Parameters
-
index An index in this tasks model. geometry Visual delegate geometry in screen coordinates. delegate The delegate. Implementations are on their own with regard to extracting information from this, and should take care to reject invalid objects.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1600 of file tasksmodel.cpp.
◆ requestRemoveLauncher()
bool TaskManager::TasksModel::requestRemoveLauncher | ( | const QUrl & | url | ) |
Request removing the launcher with the given URL.
If this URL is already in the list, the request will fail. URLs are compared for equality after removing the query string used to hold metadata.
- See also
- launcherUrlsMatch
- Parameters
-
url A launcher URL.
- Returns
true
if the launcher was removed.
Definition at line 1422 of file tasksmodel.cpp.
◆ requestRemoveLauncherFromActivity()
bool TaskManager::TasksModel::requestRemoveLauncherFromActivity | ( | const QUrl & | url, |
const QString & | activity ) |
Request removing the launcher with the given URL from the current activity.
If this URL is already in the list, the request will fail. URLs are compared for equality after removing the query string used to hold metadata.
- See also
- launcherUrlsMatch
- Parameters
-
url A launcher URL.
- Returns
true
if the launcher was removed.
Definition at line 1458 of file tasksmodel.cpp.
◆ requestResize()
|
overridevirtual |
Request starting an interactive resize for the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1530 of file tasksmodel.cpp.
◆ requestToggleFullScreen()
|
overridevirtual |
Request toggling the fullscreen state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1565 of file tasksmodel.cpp.
◆ requestToggleGrouping()
void TaskManager::TasksModel::requestToggleGrouping | ( | const QModelIndex & | index | ) |
Request toggling whether the task at the given index, along with any tasks matching its kind, should be grouped or not.
Task groups will be formed or broken apart as needed, along with affecting future grouping decisions as new tasks appear.
As grouping is toggled for a task, updates are made to the grouping*Blacklist properties of the model instance.
- See also
- groupingAppIdBlacklist
- groupingLauncherUrlBlacklist
- Parameters
-
index An index in this tasks model.
Definition at line 1608 of file tasksmodel.cpp.
◆ requestToggleKeepAbove()
|
overridevirtual |
Request toggling the keep-above state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1551 of file tasksmodel.cpp.
◆ requestToggleKeepBelow()
|
overridevirtual |
Request toggling the keep-below state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1558 of file tasksmodel.cpp.
◆ requestToggleMaximized()
|
overridevirtual |
Request toggling the maximized state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1544 of file tasksmodel.cpp.
◆ requestToggleMinimized()
|
overridevirtual |
Request toggling the minimized state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1537 of file tasksmodel.cpp.
◆ requestToggleShaded()
|
overridevirtual |
Request toggling the shaded state of the task at the given index.
This is meant for tasks that have an associated window, and may be a no-op when there is no window.
- Parameters
-
index An index in this tasks model.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1572 of file tasksmodel.cpp.
◆ requestVirtualDesktops()
|
overridevirtual |
Request entering the window at the given index on the specified virtual desktops.
On Wayland, virtual desktop ids are QStrings. On X11, they are uint >0.
An empty list has a special meaning: The window is entered on all virtual desktops in the session.
On X11, a window can only be on one or all virtual desktops. Therefore, only the first list entry is actually used.
On X11, the id 0 has a special meaning: The window is entered on all virtual desktops in the session.
- Parameters
-
index An index in this window tasks model. desktops A list of virtual desktop ids.
Implements TaskManager::AbstractTasksModelIface.
Definition at line 1579 of file tasksmodel.cpp.
◆ roleNames()
|
overridevirtual |
Reimplemented from QAbstractProxyModel.
Definition at line 997 of file tasksmodel.cpp.
◆ rowCount()
|
overridevirtual |
Reimplemented from QSortFilterProxyModel.
Definition at line 1006 of file tasksmodel.cpp.
◆ screenGeometry()
QRect TaskManager::TasksModel::screenGeometry | ( | ) | const |
The geometry of the screen used in filtering by screen.
Defaults to a null QRect.
- See also
- setGeometryScreen
- Returns
- the geometry of the screen used in filtering.
Definition at line 1083 of file tasksmodel.cpp.
◆ separateLaunchers()
bool TaskManager::TasksModel::separateLaunchers | ( | ) | const |
Whether launchers are kept separate from other kinds of tasks.
Defaults to true
.
When enabled, launcher tasks are sorted first in the tasks model and move() disallows moving them below the last launcher task, or moving a different kind of task above the first launcher. New launcher tasks are inserted after the last launcher task. When disabled, move() allows mixing, and new launcher tasks are appended to the model.
Further, when disabled, the model always behaves as if launchInPlace is enabled: A window task takes the place of the first matching launcher task.
- See also
- LauncherTasksModel
- move
- launchInPlace
- setSeparateLaunchers
- Returns
- whether launcher tasks are kept separate.
Definition at line 1239 of file tasksmodel.cpp.
◆ setActivity()
void TaskManager::TasksModel::setActivity | ( | const QString & | activity | ) |
Set the id of the activity to use in filtering by activity.
- See also
- activity
- Parameters
-
activity An activity id.
Definition at line 1108 of file tasksmodel.cpp.
◆ setFilterByActivity()
void TaskManager::TasksModel::setFilterByActivity | ( | bool | filter | ) |
Set whether tasks should be filtered by activity.
Defaults to false
.
Filtering by activity only happens if an activity id is set, even if this is set to true
.
- See also
- filterByActivity
- setActivity
- Parameters
-
filter Whether tasks should be filtered by activity.
Definition at line 1138 of file tasksmodel.cpp.
◆ setFilterByRegion()
void TaskManager::TasksModel::setFilterByRegion | ( | RegionFilterMode::Mode | mode | ) |
Set whether tasks should be filtered by region.
Defaults to RegionFilterMode::Disabled
.
Filtering by region only happens if a region is set, even if the filter is enabled.
- See also
- RegionFilterMode
- filterByRegion
- setRegionGeometry
- Since
- 6.0
- Parameters
-
mode Region filter mode.
Definition at line 1148 of file tasksmodel.cpp.
◆ setFilterByScreen()
void TaskManager::TasksModel::setFilterByScreen | ( | bool | filter | ) |
Set whether tasks should be filtered by screen.
Filtering by screen only happens if a screen number is set, even if this is set to true
.
- See also
- filterByScreen
- setScreenGeometry
- Parameters
-
filter Whether tasks should be filtered by screen.
Definition at line 1128 of file tasksmodel.cpp.
◆ setFilterByVirtualDesktop()
void TaskManager::TasksModel::setFilterByVirtualDesktop | ( | bool | filter | ) |
Set whether tasks should be filtered by virtual desktop.
Filtering by virtual desktop only happens if a virtual desktop number is set, even if this is set to true
.
- See also
- filterByVirtualDesktop
- setVirtualDesktop
- Parameters
-
filter Whether tasks should be filtered by virtual desktop.
Definition at line 1118 of file tasksmodel.cpp.
◆ setFilterHidden()
void TaskManager::TasksModel::setFilterHidden | ( | bool | filter | ) |
Set whether hidden tasks should be filtered.
- See also
- filterHidden
- Parameters
-
filter Whether hidden tasks should be filtered.
Definition at line 1188 of file tasksmodel.cpp.
◆ setFilterMinimized()
void TaskManager::TasksModel::setFilterMinimized | ( | bool | filter | ) |
Sets whether non-minimized tasks should be filtered out.
- Parameters
-
filter Whether minimized tasks should be filtered out.
- See also
- filterMinimized
- Since
- 5.27
Definition at line 1158 of file tasksmodel.cpp.
◆ setFilterNotMaximized()
void TaskManager::TasksModel::setFilterNotMaximized | ( | bool | filter | ) |
Set whether non-maximized tasks should be filtered.
- See also
- filterNotMaximized
- Parameters
-
filter Whether non-maximized tasks should be filtered.
Definition at line 1178 of file tasksmodel.cpp.
◆ setFilterNotMinimized()
void TaskManager::TasksModel::setFilterNotMinimized | ( | bool | filter | ) |
Set whether non-minimized tasks should be filtered.
- See also
- filterNotMinimized
- Parameters
-
filter Whether non-minimized tasks should be filtered.
Definition at line 1168 of file tasksmodel.cpp.
◆ setGroupingAppIdBlacklist()
void TaskManager::TasksModel::setGroupingAppIdBlacklist | ( | const QStringList & | list | ) |
Sets the blacklist of app ids (AbstractTasksModel::AppId) that is consulted before grouping a task.
If a task's app id is found on the blacklist, it is not grouped.
When set, groups will be formed and broken apart as necessary.
- See also
- groupingAppIdBlacklist
- Parameters
-
list a blacklist of app ids to be consulted before grouping a task.
Definition at line 1354 of file tasksmodel.cpp.
◆ setGroupingLauncherUrlBlacklist()
void TaskManager::TasksModel::setGroupingLauncherUrlBlacklist | ( | const QStringList & | list | ) |
Sets the blacklist of launcher URLs (AbstractTasksModel::LauncherUrl) that is consulted before grouping a task.
If a task's launcher URL is found on the blacklist, it is not grouped.
When set, groups will be formed and broken apart as necessary.
- See also
- groupingLauncherUrlBlacklist
- Parameters
-
list a blacklist of launcher URLs to be consulted before grouping a task.
Definition at line 1370 of file tasksmodel.cpp.
◆ setGroupingWindowTasksThreshold()
void TaskManager::TasksModel::setGroupingWindowTasksThreshold | ( | int | threshold | ) |
Sets the number of window tasks (AbstractTasksModel::IsWindow) above which groups will be formed, and at or below which groups will be broken apart.
If set to -1, grouping will be done regardless of the number of window tasks in the source model.
When the groupInline property is set to true
, the threshold is ignored: Grouping is always done.
- See also
- groupingWindowTasksThreshold
- groupInline
- Parameters
-
threshold A threshold number of window tasks used in grouping decisions.
Definition at line 1332 of file tasksmodel.cpp.
◆ setGroupInline()
void TaskManager::TasksModel::setGroupInline | ( | bool | groupInline | ) |
Sets whether grouping is done "inline" or not, i.e.
whether groups are maintained inside the flat, top-level list, or by forming a tree. In inline grouping mode, move() on a group member will move all siblings as well, and sorting is first done among groups, then group members.
- See also
- groupInline
- move
- groupingWindowTasksThreshold
- Parameters
-
inline Whether to do grouping inline or not.
Definition at line 1316 of file tasksmodel.cpp.
◆ setGroupMode()
void TaskManager::TasksModel::setGroupMode | ( | TasksModel::GroupMode | mode | ) |
Sets the group mode, i.e.
the criteria by which tasks should be grouped.
The group mode can be set to TasksModel::GroupDisabled to disable grouping entirely, breaking apart any existing groups.
- See also
- groupMode
- Parameters
-
mode A group mode.
Definition at line 1299 of file tasksmodel.cpp.
◆ setHideActivatedLaunchers()
void TaskManager::TasksModel::setHideActivatedLaunchers | ( | bool | hideActivatedLaunchers | ) |
Sets whether launchers should be hidden after they have been activated.
Defaults to true
.
- See also
- hideActivatedLaunchers
- Parameters
-
hideActivatedLaunchers Whether launchers should be hidden after they have been activated.
Definition at line 1286 of file tasksmodel.cpp.
◆ setLauncherList()
void TaskManager::TasksModel::setLauncherList | ( | const QStringList & | launchers | ) |
Replace the list of launcher URL strings.
Invalid or empty URLs will be rejected. Duplicate URLs will be collapsed.
- See also
- launcherList
- Parameters
-
launchers A list of launcher URL strings.
Definition at line 1398 of file tasksmodel.cpp.
◆ setLaunchInPlace()
void TaskManager::TasksModel::setLaunchInPlace | ( | bool | launchInPlace | ) |
Sets whether window tasks should be sorted as their associated launcher tasks or separately.
- See also
- launchInPlace
- Parameters
-
launchInPlace Whether window tasks should be sorted as their associated launcher tasks.
Definition at line 1261 of file tasksmodel.cpp.
◆ setRegionGeometry()
void TaskManager::TasksModel::setRegionGeometry | ( | const QRect & | geometry | ) |
Set the geometry of the screen to use in filtering by region.
If set to an invalid QRect, filtering by region is disabled.
- See also
- regionGeometry
- Since
- 6.0
- Parameters
-
geometry A region geometry.
Definition at line 1098 of file tasksmodel.cpp.
◆ setScreenGeometry()
void TaskManager::TasksModel::setScreenGeometry | ( | const QRect & | geometry | ) |
Set the geometry of the screen to use in filtering by screen.
If set to an invalid QRect, filtering by screen is disabled.
- See also
- screenGeometry
- Parameters
-
geometry A screen geometry.
Definition at line 1088 of file tasksmodel.cpp.
◆ setSeparateLaunchers()
void TaskManager::TasksModel::setSeparateLaunchers | ( | bool | separate | ) |
Sets whether launchers are kept separate from other kinds of tasks.
When enabled, launcher tasks are sorted first in the tasks model and move() disallows moving them below the last launcher task, or moving a different kind of task above the first launcher. New launcher tasks are inserted after the last launcher task. When disabled, move() allows mixing, and new launcher tasks are appended to the model.
Further, when disabled, the model always behaves as if launchInPlace is enabled: A window task takes the place of the first matching launcher task.
- See also
- LauncherTasksModel
- move
- launchInPlace
- separateLaunchers
- Parameters
-
separate Whether to keep launcher tasks separate.
Definition at line 1244 of file tasksmodel.cpp.
◆ setSortMode()
void TaskManager::TasksModel::setSortMode | ( | SortMode | mode | ) |
Sets the sort mode used in sorting tasks.
- See also
- sortMode
- Parameters
-
mode A sort mode.
Definition at line 1198 of file tasksmodel.cpp.
◆ setTaskReorderingEnabled()
void TaskManager::TasksModel::setTaskReorderingEnabled | ( | bool | enabled | ) |
Enables or disables tasks reordering.
- Parameters
-
enabled enables tasks reordering if true
; disables it otherwise.
Definition at line 1382 of file tasksmodel.cpp.
◆ setVirtualDesktop()
Set the id of the virtual desktop to use in filtering by virtual desktop.
If set to an empty id, filtering by virtual desktop is disabled.
- See also
- virtualDesktop
- Parameters
-
desktop A virtual desktop id (QString on Wayland; uint >0 on X11).
Definition at line 1078 of file tasksmodel.cpp.
◆ sortMode()
TasksModel::SortMode TaskManager::TasksModel::sortMode | ( | ) | const |
The sort mode used in sorting tasks.
Defaults to SortAlpha.
- See also
- setSortMode
- Returns
- the current sort mode.
Definition at line 1193 of file tasksmodel.cpp.
◆ syncLaunchers()
void TaskManager::TasksModel::syncLaunchers | ( | ) |
Updates the launcher list to reflect the new order after calls to move(), if needed.
- See also
- move
- launcherList
Definition at line 1841 of file tasksmodel.cpp.
◆ taskReorderingEnabled()
bool TaskManager::TasksModel::taskReorderingEnabled | ( | ) | const |
Returns whether tasks reordering is enabled or not.
- Returns
- whether tasks reordering is enabled or not.
Definition at line 1377 of file tasksmodel.cpp.
◆ virtualDesktop()
QVariant TaskManager::TasksModel::virtualDesktop | ( | ) | const |
The id of the virtual desktop used in filtering by virtual desktop.
Usually set to the id of the current virtual desktop. Defaults to empty.
- See also
- setVirtualDesktop
- Returns
- the number of the virtual desktop used in filtering.
Definition at line 1073 of file tasksmodel.cpp.
◆ virtualDesktopInfo()
|
protected |
- Returns
- a shared pointer to VirtualDesktopInfo to access virtual desktop information
Definition at line 2039 of file tasksmodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:51:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.