Plasma5Support::DataSource
#include <datasource.h>
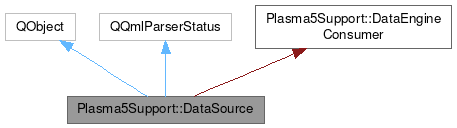
Public Types | |
enum | Change { NoChange = 0 , DataEngineChanged = 1 , SourcesChanged = 2 } |
typedef QFlags< Change > | Changes |
typedef QMap< QString, QVariant > | Data |
![]() | |
typedef | QObjectList |
Properties | |
QStringList | connectedSources |
QQmlPropertyMap * | data |
QString | dataEngine |
QString | engine |
int | interval |
Plasma5Support::Types::IntervalAlignment | intervalAlignment |
QQmlPropertyMap * | models |
QStringList | sources |
bool | valid |
![]() | |
objectName | |
Signals | |
void | connectedSourcesChanged () |
void | dataChanged () |
void | engineChanged () |
void | intervalAlignmentChanged () |
void | intervalChanged () |
void | newData (const QString &sourceName, const QVariantMap &data) |
void | sourceAdded (const QString &source) |
void | sourceConnected (const QString &source) |
void | sourceDisconnected (const QString &source) |
void | sourceRemoved (const QString &source) |
void | sourcesChanged () |
Public Slots | |
void | dataUpdated (const QString &sourceName, const Plasma5Support::DataEngine::Data &data) |
void | modelChanged (const QString &sourceName, QAbstractItemModel *model) |
Public Member Functions | |
DataSource (QObject *parent=nullptr) | |
void | classBegin () override |
void | componentComplete () override |
QStringList | connectedSources () const |
Q_INVOKABLE void | connectSource (const QString &source) |
QQmlPropertyMap * | data () const |
Q_INVOKABLE void | disconnectSource (const QString &source) |
QString | engine () const |
int | interval () const |
Plasma5Support::Types::IntervalAlignment | intervalAlignment () const |
QQmlPropertyMap * | models () const |
Q_INVOKABLE QObject * | serviceForSource (const QString &source) |
void | setConnectedSources (const QStringList &s) |
void | setEngine (const QString &e) |
void | setInterval (const int interval) |
void | setIntervalAlignment (Plasma5Support::Types::IntervalAlignment intervalAlignment) |
QStringList | sources () const |
bool | valid () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() |
Protected Slots | |
void | removeSource (const QString &source) |
void | setupData () |
void | updateSources () |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Provides data from a range of plugins.
Definition at line 31 of file datasource.h.
Member Typedef Documentation
◆ Changes
QFlags< Change > Plasma5Support::DataSource::Changes |
Definition at line 43 of file datasource.h.
◆ Data
Definition at line 45 of file datasource.h.
Member Enumeration Documentation
◆ Change
enum Plasma5Support::DataSource::Change |
Definition at line 38 of file datasource.h.
Property Documentation
◆ connectedSources
|
readwrite |
List of all the sources connected to the DataEngine.
Definition at line 95 of file datasource.h.
◆ data
|
read |
All the data fetched by this dataengine.
This is a map of maps.
- At the first level, there are the source names.
- At the second level, there are source-specific keys set by the DataEngine.
Refer to a particular DataEngine implementation for available sources, keys and expected value types.
Definition at line 121 of file datasource.h.
◆ dataEngine
|
readwrite |
Plugin name of the Plasma5Support DataEngine.
Definition at line 84 of file datasource.h.
◆ engine
|
readwrite |
Definition at line 85 of file datasource.h.
◆ interval
|
readwrite |
Polling interval in milliseconds when the data will be fetched again.
If 0, no polling will be done.
Definition at line 64 of file datasource.h.
◆ intervalAlignment
|
readwrite |
The interval to align polling to.
Definition at line 74 of file datasource.h.
◆ models
|
read |
All the models associated to this DataEngine, indexed by source.
In order for a model to be available, besides being implemented in the DataEngine, the user has to be connected to its source, i.e. the source name has to be present in connectedSources list.
Definition at line 134 of file datasource.h.
◆ sources
|
read |
Read-only list of all the sources available from the DataEngine (connected or not).
Definition at line 105 of file datasource.h.
◆ valid
|
read |
True if the connection to the Plasma DataEngine is valid.
Definition at line 55 of file datasource.h.
Constructor & Destructor Documentation
◆ DataSource()
|
explicit |
Definition at line 15 of file datasource.cpp.
Member Function Documentation
◆ classBegin()
|
overridevirtual |
Implements QQmlParserStatus.
Definition at line 26 of file datasource.cpp.
◆ componentComplete()
|
overridevirtual |
Implements QQmlParserStatus.
Definition at line 30 of file datasource.cpp.
◆ connectedSources()
|
inline |
Definition at line 96 of file datasource.h.
◆ connectSource()
void Plasma5Support::DataSource::connectSource | ( | const QString & | source | ) |
Connects a new source and adds it to the connectedSources list.
Definition at line 217 of file datasource.cpp.
◆ data()
|
inline |
Definition at line 122 of file datasource.h.
◆ dataUpdated
|
slot |
Definition at line 157 of file datasource.cpp.
◆ disconnectSource()
void Plasma5Support::DataSource::disconnectSource | ( | const QString & | source | ) |
Disconnects from a DataEngine source and removes it from the connectedSources list.
Definition at line 231 of file datasource.cpp.
◆ engine()
|
inline |
Definition at line 86 of file datasource.h.
◆ interval()
|
inline |
Definition at line 65 of file datasource.h.
◆ intervalAlignment()
|
inline |
Definition at line 75 of file datasource.h.
◆ modelChanged
|
slot |
Definition at line 169 of file datasource.cpp.
◆ models()
|
inline |
Definition at line 135 of file datasource.h.
◆ removeSource
|
protectedslot |
Definition at line 183 of file datasource.cpp.
◆ serviceForSource()
- Returns
- a Plasma5Support::Service given a source name
- Parameters
-
source source name we want a service of
Definition at line 204 of file datasource.cpp.
◆ setConnectedSources()
void Plasma5Support::DataSource::setConnectedSources | ( | const QStringList & | s | ) |
Definition at line 36 of file datasource.cpp.
◆ setEngine()
void Plasma5Support::DataSource::setEngine | ( | const QString & | e | ) |
Definition at line 67 of file datasource.cpp.
◆ setInterval()
void Plasma5Support::DataSource::setInterval | ( | const int | interval | ) |
Definition at line 118 of file datasource.cpp.
◆ setIntervalAlignment()
void Plasma5Support::DataSource::setIntervalAlignment | ( | Plasma5Support::Types::IntervalAlignment | intervalAlignment | ) |
Definition at line 129 of file datasource.cpp.
◆ setupData
|
protectedslot |
Definition at line 140 of file datasource.cpp.
◆ sources()
|
inline |
Definition at line 106 of file datasource.h.
◆ updateSources
|
protectedslot |
Definition at line 241 of file datasource.cpp.
◆ valid()
|
inline |
Definition at line 56 of file datasource.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:59:38 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.