Plasma5Support::DataEngine
#include <Plasma/DataEngine>
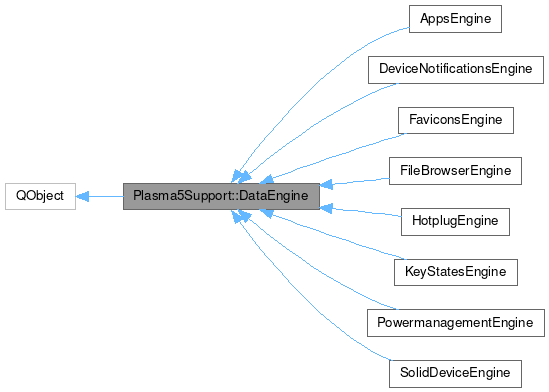
Public Types | |
typedef QMap< QString, QVariant > | Data |
typedef QMapIterator< QString, QVariant > | DataIterator |
typedef QHash< QString, DataEngine * > | Dict |
typedef QHash< QString, DataContainer * > | SourceDict |
Signals | |
void | sourceAdded (const QString &source) |
void | sourceRemoved (const QString &source) |
Public Member Functions | |
DataEngine (const KPluginMetaData &plugin, QObject *parent=nullptr) | |
DataEngine (QObject *parent=nullptr) | |
Q_INVOKABLE void | connectAllSources (QObject *visualization, uint pollingInterval=0, Plasma5Support::Types::IntervalAlignment intervalAlignment=Types::NoAlignment) const |
Q_INVOKABLE void | connectSource (const QString &source, QObject *visualization, uint pollingInterval=0, Plasma5Support::Types::IntervalAlignment intervalAlignment=Types::NoAlignment) const |
Q_INVOKABLE DataContainer * | containerForSource (const QString &source) |
Q_INVOKABLE void | disconnectSource (const QString &source, QObject *visualization) const |
bool | isEmpty () const |
bool | isValid () const |
KPluginMetaData | metadata () const |
QAbstractItemModel * | modelForSource (const QString &source) |
virtual Q_INVOKABLE Service * | serviceForSource (const QString &source) |
virtual QStringList | sources () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Slots | |
void | forceImmediateUpdateOfAllVisualizations () |
void | removeSource (const QString &source) |
void | updateAllSources () |
Protected Member Functions | |
void | addSource (DataContainer *source) |
QHash< QString, DataContainer * > | containerDict () const |
int | minimumPollingInterval () const |
void | removeAllData (const QString &source) |
void | removeAllSources () |
void | removeData (const QString &source, const QString &key) |
void | setData (const QString &source, const QString &key, const QVariant &value) |
void | setData (const QString &source, const QVariant &value) |
void | setData (const QString &source, const QVariantMap &data) |
void | setMinimumPollingInterval (int minimumMs) |
void | setModel (const QString &source, QAbstractItemModel *model) |
void | setPollingInterval (uint frequency) |
void | setStorageEnabled (const QString &source, bool store) |
void | setValid (bool valid) |
virtual bool | sourceRequestEvent (const QString &source) |
void | timerEvent (QTimerEvent *event) override |
virtual bool | updateSourceEvent (const QString &source) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Data provider for plasmoids (Plasma plugins)
This is the base class for DataEngines, which provide access to bodies of data via a common and consistent interface. The common use of a DataEngine is to provide data to a widget for display. This allows a user interface element to show all sorts of data: as long as there is a DataEngine, the data is retrievable.
DataEngines are loaded as plugins on demand and provide zero, one or more data sources which are identified by name. For instance, a network DataEngine might provide a data source for each network interface.
Definition at line 44 of file dataengine.h.
Member Typedef Documentation
◆ Data
typedef QMap<QString, QVariant> Plasma5Support::DataEngine::Data |
Definition at line 50 of file dataengine.h.
◆ DataIterator
Definition at line 51 of file dataengine.h.
◆ Dict
typedef QHash<QString, DataEngine *> Plasma5Support::DataEngine::Dict |
Definition at line 49 of file dataengine.h.
◆ SourceDict
Definition at line 52 of file dataengine.h.
Constructor & Destructor Documentation
◆ DataEngine() [1/2]
|
explicit |
Constructor.
- Parameters
-
parent The parent object. plugin metadata that describes the engine
- Since
- 5.67
Definition at line 34 of file dataengine.cpp.
◆ DataEngine() [2/2]
|
explicit |
Definition at line 42 of file dataengine.cpp.
◆ ~DataEngine()
|
override |
Definition at line 48 of file dataengine.cpp.
Member Function Documentation
◆ addSource()
|
protected |
Adds an already constructed data source.
The DataEngine takes ownership of the DataContainer object. The objectName of the source is used for the source name.
- Parameters
-
source the DataContainer to add to the DataEngine
Definition at line 210 of file dataengine.cpp.
◆ connectAllSources()
void Plasma5Support::DataEngine::connectAllSources | ( | QObject * | visualization, |
uint | pollingInterval = 0, | ||
Plasma5Support::Types::IntervalAlignment | intervalAlignment = Types::NoAlignment ) const |
Connects all currently existing sources to an object for data updates.
The object must have a slot with the following signature:
The data is a QHash of QVariants keyed by QString names, allowing one data source to provide sets of related data.
This method may be called multiple times for the same visualization without side-effects. This can be useful to change the pollingInterval.
Note that this method does not automatically connect sources that may appear later on. Connecting and responding to the sourceAdded signal is still required to achieve that.
- Parameters
-
visualization the object to connect the data source to pollingInterval the frequency, in milliseconds, with which to check for updates; a value of 0 (the default) means to update only when there is new data spontaneously generated (e.g. by the engine); any other value results in periodic updates from this source. This value is per-visualization and can be handy for items that require constant updates such as scrolling graphs or clocks. If the data has not changed, no update will be sent. intervalAlignment the number of ms to align the interval to
Definition at line 90 of file dataengine.cpp.
◆ connectSource()
void Plasma5Support::DataEngine::connectSource | ( | const QString & | source, |
QObject * | visualization, | ||
uint | pollingInterval = 0, | ||
Plasma5Support::Types::IntervalAlignment | intervalAlignment = Types::NoAlignment ) const |
Connects a source to an object for data updates.
The object must have a slot with the following signature:
The data is a QHash of QVariants keyed by QString names, allowing one data source to provide sets of related data.
- Parameters
-
source the name of the data source visualization the object to connect the data source to pollingInterval the frequency, in milliseconds, with which to check for updates; a value of 0 (the default) means to update only when there is new data spontaneously generated (e.g. by the engine); any other value results in periodic updates from this source. This value is per-visualization and can be handy for items that require constant updates such as scrolling graphs or clocks. If the data has not changed, no update will be sent. intervalAlignment the number of ms to align the interval to
Definition at line 68 of file dataengine.cpp.
◆ containerDict()
|
protected |
- Returns
- the list of active DataContainers.
Definition at line 296 of file dataengine.cpp.
◆ containerForSource()
DataContainer * Plasma5Support::DataEngine::containerForSource | ( | const QString & | source | ) |
Retrieves a pointer to the DataContainer for a given source.
This method should not be used if possible.
- Parameters
-
source the name of the source.
- Returns
- pointer to a DataContainer, or zero on failure
Definition at line 106 of file dataengine.cpp.
◆ disconnectSource()
void Plasma5Support::DataEngine::disconnectSource | ( | const QString & | source, |
QObject * | visualization ) const |
Disconnects a source from an object that was receiving data updates.
- Parameters
-
source the name of the data source visualization the object to connect the data source to
Definition at line 97 of file dataengine.cpp.
◆ forceImmediateUpdateOfAllVisualizations
|
protectedslot |
Forces an immediate update to all connected sources, even those with timeouts that haven't yet expired.
This should only be used when there was no data available, e.g. due to network non-availability, and then it becomes available. Normal changes in data values due to calls to updateSource or in the natural progression of the monitored object (e.g. CPU heat) should not result in a call to this method!
- Since
- 4.4
Definition at line 347 of file dataengine.cpp.
◆ isEmpty()
bool Plasma5Support::DataEngine::isEmpty | ( | ) | const |
Returns true if the data engine is empty, which is to say that it has no data sources currently.
- Returns
- true if the engine has no sources currently
Definition at line 286 of file dataengine.cpp.
◆ isValid()
bool Plasma5Support::DataEngine::isValid | ( | ) | const |
Returns true if this engine is valid, otherwise returns false.
- Returns
- true if the engine is valid
Definition at line 281 of file dataengine.cpp.
◆ metadata()
KPluginMetaData Plasma5Support::DataEngine::metadata | ( | ) | const |
- Returns
- description of the plugin that implements this DataEngine
- Since
- 5.67
Definition at line 63 of file dataengine.cpp.
◆ minimumPollingInterval()
|
protected |
- Returns
- the minimum time between updates.
- See also
- setMinimumPollingInterval
Definition at line 231 of file dataengine.cpp.
◆ modelForSource()
QAbstractItemModel * Plasma5Support::DataEngine::modelForSource | ( | const QString & | source | ) |
- Returns
- The model associated to a source if any. The ownership of the model stays with the DataContainer. Returns 0 if there isn't any model associated or if the source doesn't exists.
Definition at line 199 of file dataengine.cpp.
◆ removeAllData()
|
protected |
Removes all the data associated with a data source.
- Parameters
-
source the name of the data source
Definition at line 166 of file dataengine.cpp.
◆ removeAllSources()
|
protected |
Removes all data sources.
Definition at line 262 of file dataengine.cpp.
◆ removeData()
|
protected |
Removes a data entry from a source.
- Parameters
-
source the name of the data source key the data entry to remove
Definition at line 175 of file dataengine.cpp.
◆ removeSource
|
protectedslot |
Removes a data source.
- Parameters
-
source the name of the data source to remove
Definition at line 246 of file dataengine.cpp.
◆ serviceForSource()
- Parameters
-
source the source to target the Service at
- Returns
- a Service that has the source as a destination. The service is parented to the DataEngine, but should be deleted by the caller when finished with it
Reimplemented in AppsEngine, HotplugEngine, KeyStatesEngine, PowermanagementEngine, and SolidDeviceEngine.
Definition at line 58 of file dataengine.cpp.
◆ setData() [1/3]
|
protected |
Sets a value for a data source.
If the source doesn't exist then it is created.
- Parameters
-
source the name of the data source key the key to use for the data value the data to associated with the source
Definition at line 126 of file dataengine.cpp.
◆ setData() [2/3]
|
protected |
Sets a value for a data source.
If the source doesn't exist then it is created.
- Parameters
-
source the name of the data source value the data to associated with the source
Definition at line 121 of file dataengine.cpp.
◆ setData() [3/3]
|
protected |
Adds a set of data to a data source.
If the source doesn't exist then it is created.
- Parameters
-
source the name of the data source data the data to add to the source
Definition at line 144 of file dataengine.cpp.
◆ setMinimumPollingInterval()
|
protected |
Sets the minimum amount of time, in milliseconds, that must pass between successive updates of data.
This can help prevent too many updates happening due to multiple update requests coming in, which can be useful for expensive (time- or resource-wise) update mechanisms.
The default minimumPollingInterval is -1, or "never perform automatic updates"
- Parameters
-
minimumMs the minimum time lapse, in milliseconds, between updates. A value less than 0 means to never perform automatic updates, a value of 0 means update immediately on every update request, a value >0 will result in a minimum time lapse being enforced.
Definition at line 226 of file dataengine.cpp.
◆ setModel()
|
protected |
Associates a model to a data source.
If the source doesn't exist then it is created. The source will have the key "HasModel" to easily indicate there is a model present.
The ownership of the model is transferred to the DataContainer, so the model will be deleted when a new one is set or when the DataContainer itself is deleted. As the DataContainer, it will be deleted when there won't be any visualization associated to this source.
- Parameters
-
source the name of the data source model the model instance
Definition at line 184 of file dataengine.cpp.
◆ setPollingInterval()
|
protected |
Sets up an internal update tick for all data sources.
On every update, updateSourceEvent will be called for each applicable source.
- See also
- updateSourceEvent
- Parameters
-
frequency the time, in milliseconds, between updates. A value of 0 will stop internally triggered updates.
Definition at line 236 of file dataengine.cpp.
◆ setStorageEnabled()
|
protected |
Sets a source to be stored for easy retrieval when the real source of the data (usually a network connection) is unavailable.
- Parameters
-
source the name of the source store if source should be stored
- Since
- 4.6
Definition at line 356 of file dataengine.cpp.
◆ setValid()
|
protected |
Sets whether or not this engine is valid, e.g.
can be used. In practice, only the internal fall-back engine, the NullEngine should have need for this.
- Parameters
-
valid whether or not the engine is valid
Definition at line 291 of file dataengine.cpp.
◆ sourceAdded
|
signal |
Emitted when a new data source is created.
Note that you do not need to emit this yourself unless you are reimplementing sources() and want to advertise that a new source is available (but hasn't been created yet).
- Parameters
-
source the name of the new data source
◆ sourceRemoved
|
signal |
Emitted when a data source is removed.
Note that you do not need to emit this yourself unless you have reimplemented sources() and want to signal that a source that was available but was never created is no longer available.
- Parameters
-
source the name of the data source that was removed
◆ sourceRequestEvent()
|
protectedvirtual |
When a source that does not currently exist is requested by the consumer, this method is called to give the DataEngine the opportunity to create one.
The name of the data source (e.g. the source parameter passed into setData) must be the same as the name passed to sourceRequestEvent otherwise the requesting visualization may not receive notice of a data update.
If the source can not be populated with data immediately (e.g. due to an asynchronous data acquisition method such as an HTTP request) the source must still be created, even if it is empty. This can be accomplished in these cases with the follow line:
setData(name, DataEngine::Data());
- Parameters
-
source the name of the source that has been requested
- Returns
- true if a DataContainer was set up, false otherwise
Reimplemented in FaviconsEngine, FileBrowserEngine, PowermanagementEngine, and SolidDeviceEngine.
Definition at line 111 of file dataengine.cpp.
◆ sources()
|
virtual |
- Returns
- a list of all the data sources available via this DataEngine Whether these sources are currently available (which is what the default implementation provides) or not is up to the DataEngine to decide.
Reimplemented in PowermanagementEngine.
Definition at line 53 of file dataengine.cpp.
◆ timerEvent()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Reimplemented from QObject.
Definition at line 301 of file dataengine.cpp.
◆ updateAllSources
|
protectedslot |
Immediately updates all existing sources when called.
Definition at line 333 of file dataengine.cpp.
◆ updateSourceEvent()
|
protectedvirtual |
Called by internal updating mechanisms to trigger the engine to refresh the data contained in a given source.
Reimplement this method when using facilities such as setPollingInterval.
- See also
- setPollingInterval
- Parameters
-
source the name of the source that should be updated
- Returns
- true if the data was changed, or false if there was no change or if the change will occur later
Reimplemented in PowermanagementEngine, and SolidDeviceEngine.
Definition at line 116 of file dataengine.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:42 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.