Plasma5Support::Service
#include <Plasma5Support/Service>
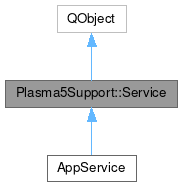
Properties | |
QString | destination |
QString | name |
QStringList | operationNames |
![]() | |
objectName | |
Signals | |
void | operationEnabledChanged (const QString &operation, bool enabled) |
void | serviceReady (Plasma5Support::Service *service) |
Public Member Functions | |
~Service () | |
Q_INVOKABLE QString | destination () const |
Q_INVOKABLE bool | isOperationEnabled (const QString &operation) const |
Q_INVOKABLE QString | name () const |
Q_INVOKABLE QVariantMap | operationDescription (const QString &operationName) |
Q_INVOKABLE QStringList | operationNames () const |
Q_INVOKABLE void | setDestination (const QString &destination) |
Q_INVOKABLE ServiceJob * | startOperationCall (const QVariantMap &description, QObject *parent=nullptr) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
Service (QObject *parent=nullptr) | |
virtual ServiceJob * | createJob (const QString &operation, QVariantMap ¶meters)=0 |
virtual void | registerOperationsScheme () |
void | setName (const QString &name) |
void | setOperationEnabled (const QString &operation, bool enable) |
void | setOperationsScheme (QIODevice *xml) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
This class provides a generic API for write access to settings or services.
Plasma5Support::Service allows interaction with a "destination", the definition of which depends on the Service itself. For a network settings Service this might be a profile name ("Home", "Office", "Road Warrior") while a web based Service this might be a username ("aseigo", "stranger65").
A Service provides one or more operations, each of which provides some sort of interaction with the destination. Operations are described using config XML which is used to create a KConfig object with one group per operation. The group names are used as the operation names, and the defined items in the group are the parameters available to be set when using that operation.
A service is started with a KConfigGroup (representing a ready to be serviced operation) and automatically deletes itself after completion and signaling success or failure. See KJob for more information on this part of the process.
Services may either be loaded "stand alone" from plugins, or from a DataEngine by passing in a source name to be used as the destination.
Sample use might look like:
Please remember, the service needs to be deleted when it will no longer be used. This can be done manually or by these (perhaps easier) alternatives:
If it is needed throughout the lifetime of the object:
If the service will not be used after just one operation call, use:
Property Documentation
◆ destination
◆ name
◆ operationNames
|
read |
Constructor & Destructor Documentation
◆ ~Service()
Plasma5Support::Service::~Service | ( | ) |
Destructor.
Definition at line 34 of file service.cpp.
◆ Service()
|
explicitprotected |
- Parameters
-
parent the parent object for this service
Definition at line 28 of file service.cpp.
Member Function Documentation
◆ createJob()
|
protectedpure virtual |
Called when a job should be created by the Service.
- Parameters
-
operation which operation to work on parameters the parameters set by the user for the operation
- Returns
- a ServiceJob that can be started and monitored by the consumer
◆ destination()
QString Plasma5Support::Service::destination | ( | ) | const |
- Returns
- the target destination, if any, that this service is associated with
Definition at line 44 of file service.cpp.
◆ isOperationEnabled()
bool Plasma5Support::Service::isOperationEnabled | ( | const QString & | operation | ) | const |
Query to find if an operation is enabled or not.
- Parameters
-
operation the name of the operation to check
- Returns
- true if the operation is enabled, false otherwise
Definition at line 141 of file service.cpp.
◆ name()
QString Plasma5Support::Service::name | ( | ) | const |
The name of this service.
Definition at line 109 of file service.cpp.
◆ operationDescription()
QVariantMap Plasma5Support::Service::operationDescription | ( | const QString & | operationName | ) |
Retrieves the parameters for a given operation.
- Parameters
-
operationName the operation to retrieve parameters for
- Returns
- QVariantMap containing the parameters
Definition at line 61 of file service.cpp.
◆ operationEnabledChanged
|
signal |
Emitted when an operation got enabled or disabled.
◆ operationNames()
QStringList Plasma5Support::Service::operationNames | ( | ) | const |
- Returns
- the possible operations for this profile
Definition at line 49 of file service.cpp.
◆ registerOperationsScheme()
|
protectedvirtual |
By default this is based on the file in plasma5support/services/name.operations, but can be reimplemented to use a different mechanism.
It should result in a call to setOperationsScheme(QIODevice *);
Definition at line 167 of file service.cpp.
◆ serviceReady
|
signal |
Emitted when this service is ready for use.
◆ setDestination()
void Plasma5Support::Service::setDestination | ( | const QString & | destination | ) |
Sets the destination for this Service to operate on.
- Parameters
-
destination specific to each Service, this sets which target or address for ServiceJobs to operate on
Definition at line 39 of file service.cpp.
◆ setName()
|
protected |
Sets the name of the Service; useful for Services not loaded from plugins, which use the plugin name for this.
- Parameters
-
name the name to use for this service
Definition at line 114 of file service.cpp.
◆ setOperationEnabled()
|
protected |
Enables a given service by name.
- Parameters
-
operation the name of the operation to enable or disable enable true if the operation should be enabled, false if disabled
Definition at line 126 of file service.cpp.
◆ setOperationsScheme()
|
protected |
Sets the XML used to define the operation schema for this Service.
Definition at line 146 of file service.cpp.
◆ startOperationCall()
ServiceJob * Plasma5Support::Service::startOperationCall | ( | const QVariantMap & | description, |
QObject * | parent = nullptr ) |
Called to create a ServiceJob which is associated with a given operation and parameter set.
- Returns
- a started ServiceJob; the consumer may connect to relevant signals before returning to the event loop
Definition at line 75 of file service.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 31 2025 12:04:52 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.