PulseAudioQt::Device
#include <device.h>
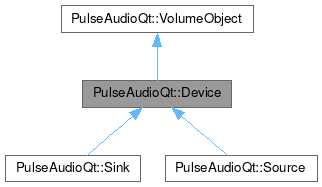
Public Types | |
enum | State { InvalidState = 0 , RunningState , IdleState , SuspendedState , UnknownState } |
Properties | |
quint32 | activePortIndex |
qint64 | baseVolume |
quint32 | cardIndex |
bool | default |
QString | description |
QString | formFactor |
QList< Port * > | ports |
QVariantMap | pulseProperties |
State | state |
bool | virtualDevice |
![]() | |
QStringList | channels |
QList< qint64 > | channelVolumes |
bool | muted |
QStringList | rawChannels |
qint64 | volume |
bool | volumeWritable |
Signals | |
void | activePortIndexChanged () |
void | baseVolumeChanged () |
void | cardIndexChanged () |
void | defaultChanged () |
void | descriptionChanged () |
void | formFactorChanged () |
void | portsChanged () |
void | pulsePropertiesChanged () |
void | stateChanged () |
void | virtualDeviceChanged () |
![]() | |
void | channelsChanged () |
void | channelVolumesChanged () |
void | isVolumeWritableChanged () |
void | mutedChanged () |
void | rawChannelsChanged () |
void | volumeChanged () |
Public Member Functions | |
quint32 | activePortIndex () const |
qint64 | baseVolume () const |
quint32 | cardIndex () const |
QString | description () const |
QString | formFactor () const |
virtual bool | isDefault () const =0 |
bool | isVirtualDevice () const |
QList< Port * > | ports () const |
QVariantMap | pulseProperties () const |
Q_ENUM (State) | |
virtual void | setActivePortIndex (quint32 port_index)=0 |
virtual void | setDefault (bool enable)=0 |
State | state () const |
virtual Q_INVOKABLE void | switchStreams ()=0 |
![]() | |
QStringList | channels () const |
QList< qint64 > | channelVolumes () const |
bool | isMuted () const |
bool | isVolumeWritable () const |
QStringList | rawChannels () const |
virtual Q_INVOKABLE void | setChannelVolume (int channel, qint64 volume)=0 |
virtual void | setChannelVolumes (const QList< qint64 > &channelVolumes)=0 |
virtual void | setMuted (bool muted)=0 |
virtual void | setVolume (qint64 volume)=0 |
qint64 | volume () const |
Detailed Description
Member Enumeration Documentation
◆ State
Property Documentation
◆ activePortIndex
◆ baseVolume
◆ cardIndex
◆ default
◆ description
◆ formFactor
◆ ports
◆ pulseProperties
◆ state
◆ virtualDevice
Constructor & Destructor Documentation
◆ ~Device()
|
override |
Definition at line 84 of file device.cpp.
Member Function Documentation
◆ activePortIndex()
quint32 PulseAudioQt::Device::activePortIndex | ( | ) | const |
The currently active port, by index.
Definition at line 37 of file device.cpp.
◆ baseVolume()
qint64 PulseAudioQt::Device::baseVolume | ( | ) | const |
The base volume of this device, generally useful as a good default volume.
Definition at line 42 of file device.cpp.
◆ cardIndex()
quint32 PulseAudioQt::Device::cardIndex | ( | ) | const |
Index of the card that owns this device.
Definition at line 27 of file device.cpp.
◆ description()
QString PulseAudioQt::Device::description | ( | ) | const |
A human readable description of this device.
Definition at line 17 of file device.cpp.
◆ formFactor()
QString PulseAudioQt::Device::formFactor | ( | ) | const |
The device's form factor.
One of "internal", "speaker", "handset", "tv", "webcam", "microphone", "headset", "headphone", "hands-free", "car", "hifi", "computer", "portable". This is based on PA_PROP_DEVICE_FORM_FACTOR.
Definition at line 22 of file device.cpp.
◆ isDefault()
|
pure virtual |
Whether this is the default device.
Implemented in PulseAudioQt::Sink, and PulseAudioQt::Source.
◆ isVirtualDevice()
bool PulseAudioQt::Device::isVirtualDevice | ( | ) | const |
- Returns
- true when the device is a virtual device (e.g. a software-only sink)
Definition at line 52 of file device.cpp.
◆ ports()
The ports associated with this device.
Definition at line 32 of file device.cpp.
◆ pulseProperties()
|
nodiscard |
- Returns
- QVariantMap the pulseaudio properties of this device (e.g. media.class, device.api, ...)
Definition at line 47 of file device.cpp.
◆ setActivePortIndex()
|
pure virtual |
Set the currently active port, by index.
Implemented in PulseAudioQt::Sink, and PulseAudioQt::Source.
◆ setDefault()
|
pure virtual |
Set whether this is the default device.
Implemented in PulseAudioQt::Sink, and PulseAudioQt::Source.
◆ state()
Device::State PulseAudioQt::Device::state | ( | ) | const |
The state of this device.
Definition at line 12 of file device.cpp.
◆ switchStreams()
|
pure virtual |
Switch all streams onto this Device Iterates through all relevant streams for the Device type and assigns them to this Device.
For example for a Sink device all SinkInputs known to the daemon will be explicitly switched onto this Sink. Useful for mass-rerouting of streams from different devices onto a specific target device.
Implemented in PulseAudioQt::Sink, and PulseAudioQt::Source.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:08:58 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.