libkcal
KCal::CalendarLocal Class Reference
This class provides a calendar stored as a local file. More...
#include <calendarlocal.h>
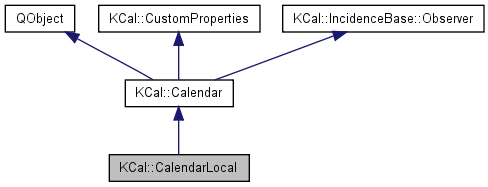
Public Member Functions | |
bool | addEvent (Event *event) |
bool | addJournal (Journal *) |
bool | addTodo (Todo *todo) |
Alarm::List | alarms (const QDateTime &from, const QDateTime &to) |
Alarm::List | alarmsTo (const QDateTime &to) |
CalendarLocal (const QString &timeZoneId) | |
void | close () |
void | deleteAllEvents () |
void | deleteAllJournals () |
void | deleteAllTodos () |
bool | deleteEvent (Event *event) |
bool | deleteJournal (Journal *) |
bool | deleteTodo (Todo *) |
Event * | event (const QString &uid) |
Journal * | journal (const QString &uid) |
bool | load (const QString &fileName, CalFormat *format=0) |
Event::List | rawEvents (const QDate &start, const QDate &end, bool inclusive=false) |
Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEventsForDate (const QDateTime &qdt) |
Event::List | rawEventsForDate (const QDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Journal::List | rawJournalsForDate (const QDate &date) |
Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Todo::List | rawTodosForDate (const QDate &date) |
bool | reload (const QString &tz) |
void | save () |
bool | save (const QString &fileName, CalFormat *format=0) |
void | setTimeZoneIdViewOnly (const QString &tz) |
Todo * | todo (const QString &uid) |
~CalendarLocal () | |
Protected Member Functions | |
void | appendAlarms (Alarm::List &alarms, Incidence *incidence, const QDateTime &from, const QDateTime &to) |
void | appendRecurringAlarms (Alarm::List &alarms, Incidence *incidence, const QDateTime &from, const QDateTime &to) |
void | incidenceUpdated (IncidenceBase *i) |
void | insertEvent (Event *event) |
Detailed Description
This class provides a calendar stored as a local file.Definition at line 36 of file calendarlocal.h.
Constructor & Destructor Documentation
CalendarLocal::CalendarLocal | ( | const QString & | timeZoneId | ) |
Constructs a new calendar, with variables initialized to sane values.
Definition at line 44 of file calendarlocal.cpp.
CalendarLocal::~CalendarLocal | ( | ) |
Definition at line 57 of file calendarlocal.cpp.
Member Function Documentation
bool CalendarLocal::addEvent | ( | Event * | event | ) | [virtual] |
bool CalendarLocal::addJournal | ( | Journal * | journal | ) | [virtual] |
Add a Journal entry to calendar.
Implements KCal::Calendar.
Definition at line 506 of file calendarlocal.cpp.
bool CalendarLocal::addTodo | ( | Todo * | todo | ) | [virtual] |
Add a todo to the todolist.
Implements KCal::Calendar.
Definition at line 156 of file calendarlocal.cpp.
Alarm::List CalendarLocal::alarms | ( | const QDateTime & | from, | |
const QDateTime & | to | |||
) | [virtual] |
Return all alarms, which ocur in the given time interval.
Implements KCal::Calendar.
Definition at line 237 of file calendarlocal.cpp.
Alarm::List CalendarLocal::alarmsTo | ( | const QDateTime & | to | ) |
void CalendarLocal::appendAlarms | ( | Alarm::List & | alarms, | |
Incidence * | incidence, | |||
const QDateTime & | from, | |||
const QDateTime & | to | |||
) | [protected] |
Append alarms of incidence in interval to list of alarms.
Definition at line 259 of file calendarlocal.cpp.
void CalendarLocal::appendRecurringAlarms | ( | Alarm::List & | alarms, | |
Incidence * | incidence, | |||
const QDateTime & | from, | |||
const QDateTime & | to | |||
) | [protected] |
Append alarms of recurring events in interval to list of alarms.
Definition at line 278 of file calendarlocal.cpp.
void CalendarLocal::close | ( | ) | [virtual] |
Clears out the current calendar, freeing all used memory etc.
etc.
Implements KCal::Calendar.
Definition at line 92 of file calendarlocal.cpp.
void CalendarLocal::deleteAllEvents | ( | ) |
void CalendarLocal::deleteAllJournals | ( | ) |
void CalendarLocal::deleteAllTodos | ( | ) |
bool CalendarLocal::deleteEvent | ( | Event * | event | ) | [virtual] |
Deletes an event from this calendar.
Implements KCal::Calendar.
Definition at line 121 of file calendarlocal.cpp.
bool CalendarLocal::deleteJournal | ( | Journal * | journal | ) | [virtual] |
Remove a Journal from the calendar.
Implements KCal::Calendar.
Definition at line 524 of file calendarlocal.cpp.
bool CalendarLocal::deleteTodo | ( | Todo * | todo | ) | [virtual] |
Remove a todo from the todolist.
Implements KCal::Calendar.
Definition at line 172 of file calendarlocal.cpp.
Retrieves an event on the basis of the unique string ID.
Implements KCal::Calendar.
Definition at line 150 of file calendarlocal.cpp.
void CalendarLocal::incidenceUpdated | ( | IncidenceBase * | i | ) | [protected, virtual] |
Notification function of IncidenceBase::Observer.
Reimplemented from KCal::Calendar.
Definition at line 353 of file calendarlocal.cpp.
void CalendarLocal::insertEvent | ( | Event * | event | ) | [protected] |
inserts an event into its "proper place" in the calendar.
Definition at line 367 of file calendarlocal.cpp.
Return Journal with given UID.
Implements KCal::Calendar.
Definition at line 549 of file calendarlocal.cpp.
Loads a calendar on disk in vCalendar or iCalendar format into the current calendar.
Incidences already present are preserved. If an event of the file to be loaded has the same unique id as an incidence already present the new incidence is ignored.
To load a CalendarLocal object from a file without preserving existing incidences call close() before load().
- Returns:
- true, if successful, false on error.
- Parameters:
-
fileName the name of the calendar on disk. format the format to use. If 0, iCalendar and vCalendar will be used
Definition at line 62 of file calendarlocal.cpp.
Event::List CalendarLocal::rawEvents | ( | const QDate & | start, | |
const QDate & | end, | |||
bool | inclusive = false | |||
) | [virtual] |
Get unfiltered events in a range of dates.
If inclusive is set to true, only events are returned, which are completely included in the range. If inclusive is set to false, all events which overlap the range are returned. An event's entire time span is considered in evaluating whether it should be returned. For a non-recurring event, its span is from its start to its end date. For a recurring event, its time span is from its first to its last recurrence.
Implements KCal::Calendar.
Definition at line 414 of file calendarlocal.cpp.
Event::List CalendarLocal::rawEvents | ( | EventSortField | sortField = EventSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return unfiltered list of all events in calendar.
Implements KCal::Calendar.
Definition at line 497 of file calendarlocal.cpp.
Event::List CalendarLocal::rawEventsForDate | ( | const QDateTime & | qdt | ) | [virtual] |
Get unfiltered events for date qdt.
Implements KCal::Calendar.
Definition at line 492 of file calendarlocal.cpp.
Event::List CalendarLocal::rawEventsForDate | ( | const QDate & | date, | |
EventSortField | sortField = EventSortUnsorted , |
|||
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Builds and then returns a list of all events that match for the date specified.
useful for dayView, etc. etc.
Implements KCal::Calendar.
Definition at line 380 of file calendarlocal.cpp.
Journal::List CalendarLocal::rawJournals | ( | JournalSortField | sortField = JournalSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return list of all journals.
Implements KCal::Calendar.
Definition at line 559 of file calendarlocal.cpp.
Journal::List CalendarLocal::rawJournalsForDate | ( | const QDate & | date | ) | [virtual] |
Get unfiltered journals for a given date.
Implements KCal::Calendar.
Definition at line 564 of file calendarlocal.cpp.
Todo::List CalendarLocal::rawTodos | ( | TodoSortField | sortField = TodoSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return list of all todos.
Implements KCal::Calendar.
Definition at line 201 of file calendarlocal.cpp.
Todo::List CalendarLocal::rawTodosForDate | ( | const QDate & | date | ) | [virtual] |
Returns list of todos due on the specified date.
Implements KCal::Calendar.
Definition at line 217 of file calendarlocal.cpp.
bool CalendarLocal::reload | ( | const QString & | tz | ) | [virtual] |
Reloads the contents of the storage into memory.
The associated file name must be known, in other words a previous load() must have been executed.
- Returns:
- success or failure
Implements KCal::Calendar.
Definition at line 69 of file calendarlocal.cpp.
void KCal::CalendarLocal::save | ( | ) | [inline, virtual] |
Sync changes in memory to persistant storage.
Implements KCal::Calendar.
Definition at line 81 of file calendarlocal.h.
Writes out the calendar to disk in the specified format.
CalendarLocal takes ownership of the CalFormat object.
- Parameters:
-
fileName the name of the file format the format to use
- Returns:
- true, if successful, false on error.
Definition at line 80 of file calendarlocal.cpp.
void CalendarLocal::setTimeZoneIdViewOnly | ( | const QString & | tz | ) | [virtual] |
Set the timezone of the calendar to be used for interpreting the events in the calendar.
This requires that the calendar is saved first, so the user is asked whether he wants to do that, or keep the timezone as is.
Implements KCal::Calendar.
Definition at line 579 of file calendarlocal.cpp.
Searches todolist for an event with this unique string identifier, returns a pointer or null.
Implements KCal::Calendar.
Definition at line 207 of file calendarlocal.cpp.
The documentation for this class was generated from the following files: