libkcal
KCal::Calendar Class Reference
This is the main "calendar" object class. More...
#include <calendar.h>
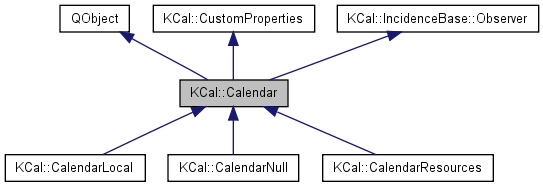
Classes | |
class | Observer |
The Observer class. More... | |
Signals | |
void | calendarChanged () |
void | calendarLoaded () |
void | calendarSaved () |
Public Member Functions | |
virtual bool | addEvent (Event *event)=0 |
virtual bool | addIncidence (Incidence *incidence) |
virtual bool | addJournal (Journal *journal)=0 |
virtual bool | addTodo (Todo *todo)=0 |
virtual Alarm::List | alarms (const QDateTime &from, const QDateTime &to)=0 |
virtual bool | beginChange (Incidence *incidence) |
Calendar (const QString &timeZoneId) | |
QStringList | categories () |
virtual void | close ()=0 |
virtual bool | deleteEvent (Event *event)=0 |
virtual bool | deleteIncidence (Incidence *incidence) |
virtual bool | deleteJournal (Journal *journal)=0 |
virtual bool | deleteTodo (Todo *todo)=0 |
Incidence * | dissociateOccurrence (Incidence *incidence, QDate date, bool single=true) |
virtual bool | endChange (Incidence *incidence) |
virtual Event * | event (const QString &uid)=0 |
Event::List | events (const QDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | events (const QDate &start, const QDate &end, bool inclusive=false) |
Event::List | events (const QDateTime &qdt) |
virtual Event::List | events (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
CalFilter * | filter () |
const Person & | getOwner () const |
Incidence * | incidence (const QString &uid) |
Incidence * | incidenceFromSchedulingID (const QString &sid) |
virtual Incidence::List | incidences (const QDate &date) |
virtual Incidence::List | incidences () |
Incidence::List | incidencesFromSchedulingID (const QString &UID) |
bool | isLocalTime () const |
bool | isModified () const |
virtual bool | isSaving () |
virtual Journal * | journal (const QString &uid)=0 |
virtual Journal::List | journals (const QDate &date) |
virtual Journal::List | journals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
QString | productId () |
virtual Event::List | rawEvents (const QDate &start, const QDate &end, bool inclusive=false)=0 |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const QDate &date, EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEventsForDate (const QDateTime &qdt)=0 |
virtual Incidence::List | rawIncidences () |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Journal::List | rawJournalsForDate (const QDate &date)=0 |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Todo::List | rawTodosForDate (const QDate &date)=0 |
void | registerObserver (Observer *observer) |
virtual bool | reload (const QString &tz)=0 |
virtual void | removeRelations (Incidence *incidence) |
virtual void | save ()=0 |
void | setFilter (CalFilter *filter) |
void | setLocalTime () |
void | setModified (bool modified) |
void | setOwner (const Person &owner) |
void | setProductId (const QString &productId) |
void | setTimeZoneId (const QString &timeZoneId) |
virtual void | setTimeZoneIdViewOnly (const QString &timeZoneId)=0 |
virtual void | setupRelations (Incidence *incidence) |
QString | timeZoneId () const |
virtual Todo * | todo (const QString &uid)=0 |
virtual Todo::List | todos (const QDate &date) |
virtual Todo::List | todos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
void | unregisterObserver (Observer *observer) |
virtual | ~Calendar () |
Static Public Member Functions | |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (Event::List *eventList, EventSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (Journal::List *journalList, JournalSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (Todo::List *todoList, TodoSortField sortField, SortDirection sortDirection) |
Protected Member Functions | |
virtual void | customPropertyUpdated () |
virtual void | doSetTimeZoneId (const QString &) |
void | incidenceUpdated (IncidenceBase *incidenceBase) |
void | notifyIncidenceAdded (Incidence *incidence) |
void | notifyIncidenceChanged (Incidence *incidence) |
void | notifyIncidenceDeleted (Incidence *incidence) |
void | setObserversEnabled (bool enabled) |
Detailed Description
This is the main "calendar" object class.It holds information like Incidences(Events, To-dos, Journals), user information, etc. etc.
This is an abstract base class defining the interface to a calendar. It is implemented by subclasses like CalendarLocal, which use different methods to store and access the data.
Ownership of Incidences:
Incidence ownership is handled by the following policy: As soon as an Incidence (or any other subclass of IncidenceBase) object is added to the Calendar by an add...() method it is owned by the Calendar object. The Calendar takes care of deleting it. All Incidences returned by the query functions are returned as pointers so that changes to the returned Incidences are immediately visible in the Calendar. Do Not delete any Incidence object you get from Calendar.
Time Zone Handling:
- Incidence Storing:
- By default, (when LocalTime is unset) Incidence dates will have the "UTC" time zone when stored into a calendar file.
- To store Incidence dates without a time zone (i.e, "floating time zone") LocalTime must be set using the setLocalTime() method.
- Incidence Viewing:
- By default, Incidence dates will have the "UTC" time zone when read from a calendar.
- To view Incidence dates using another time zone TimeZoneId must be set using the setTimeZoneId() method, or the TimeZoneId can be passed to the Calendar constructor.
- It is permitted to switch viewing time zones using setTimeZoneId() as desired after the Calendar object has been constructed.
- Note that:
- The Calendar class doesn't do anything with TimeZoneId: it simply saves it for later use by the ICalFormat class.
- The ICalFormat class takes TimeZoneId and applies it to loaded Incidences before returning them in ICalFormat::load().
- Each Incidence can have its own time zone (or have a floating time zone).
- Once an Incidence is loaded it is adjusted to use the viewing time zone, TimeZoneId.
- Depending on the LocalTime setting, all loaded Incidences are stored either in UTC or without a time zone (floating time zone).
Definition at line 167 of file calendar.h.
Constructor & Destructor Documentation
Calendar::Calendar | ( | const QString & | timeZoneId | ) |
Construct Calendar object using a Time Zone.
- Parameters:
-
timeZoneId is a string containing a Time Zone ID, which is assumed to be valid. The Time Zone Id is used to set the time zone for viewing Incidence dates.
On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning:
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Definition at line 43 of file calendar.cpp.
Calendar::~Calendar | ( | ) | [virtual] |
Member Function Documentation
virtual bool KCal::Calendar::addEvent | ( | Event * | event | ) | [pure virtual] |
Insert an Event into the Calendar.
- Parameters:
-
event is a pointer to the Event to insert.
- Returns:
- true if the Event was successfully inserted; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
bool Calendar::addIncidence | ( | Incidence * | incidence | ) | [virtual] |
Insert an Incidence into the Calendar.
- Parameters:
-
incidence is a pointer to the Incidence to insert.
- Returns:
- true if the Incidence was successfully inserted; false otherwise.
Reimplemented in KCal::CalendarResources.
Definition at line 283 of file calendar.cpp.
virtual bool KCal::Calendar::addJournal | ( | Journal * | journal | ) | [pure virtual] |
Insert a Journal into the Calendar.
- Parameters:
-
journal is a pointer to the Journal to insert.
- Returns:
- true if the Journal was successfully inserted; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual bool KCal::Calendar::addTodo | ( | Todo * | todo | ) | [pure virtual] |
Insert a Todo into the Calendar.
- Parameters:
-
todo is a pointer to the Todo to insert.
- Returns:
- true if the Todo was successfully inserted; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Alarm::List KCal::Calendar::alarms | ( | const QDateTime & | from, | |
const QDateTime & | to | |||
) | [pure virtual] |
Return a list of Alarms within a time range for this Calendar.
- Parameters:
-
from is the starting timestamp. to is the ending timestamp.
- Returns:
- the list of Alarms for the for the specified time range.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
bool Calendar::beginChange | ( | Incidence * | incidence | ) | [virtual] |
Flag that a change to a Calendar Incidence is starting.
- Parameters:
-
incidence is a pointer to the Incidence that will be changing.
Reimplemented in KCal::CalendarResources.
Definition at line 843 of file calendar.cpp.
void KCal::Calendar::calendarChanged | ( | ) | [signal] |
Signal that the Calendar has been modified.
void KCal::Calendar::calendarLoaded | ( | ) | [signal] |
Signal that the Calendar has been loaded into memory.
void KCal::Calendar::calendarSaved | ( | ) | [signal] |
Signal that the Calendar has been saved.
QStringList Calendar::categories | ( | ) |
Return a list of all categories used by Incidences in this Calendar.
- Returns:
- a QStringList containing all the categories.
Definition at line 125 of file calendar.cpp.
virtual void KCal::Calendar::close | ( | ) | [pure virtual] |
Clears out the current Calendar, freeing all used memory etc.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
void Calendar::customPropertyUpdated | ( | ) | [protected, virtual] |
Called when a custom property has been changed.
The default implementation does nothing: override in derived classes to perform change processing.
Reimplemented from KCal::CustomProperties.
Definition at line 807 of file calendar.cpp.
virtual bool KCal::Calendar::deleteEvent | ( | Event * | event | ) | [pure virtual] |
Remove an Event from the Calendar.
- Parameters:
-
event is a pointer to the Event to remove.
- Returns:
- true if the Event was successfully remove; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
bool Calendar::deleteIncidence | ( | Incidence * | incidence | ) | [virtual] |
virtual bool KCal::Calendar::deleteJournal | ( | Journal * | journal | ) | [pure virtual] |
Remove a Journal from the Calendar.
- Parameters:
-
journal is a pointer to the Journal to remove.
- Returns:
- true if the Journal was successfully removed; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual bool KCal::Calendar::deleteTodo | ( | Todo * | todo | ) | [pure virtual] |
Remove a Todo from the Calendar.
- Parameters:
-
todo is a pointer to the Todo to remove.
- Returns:
- true if the Todo was successfully removed; false otherwise.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
Incidence * Calendar::dissociateOccurrence | ( | Incidence * | incidence, | |
QDate | date, | |||
bool | single = true | |||
) |
Dissociate an Incidence from a recurring Incidence.
Dissociate a single occurrence or all future occurrences from a recurring sequence.
By default, only one single Incidence for the specified date will be dissociated and returned. If single is false, then the recurrence will be split at date, the old Incidence will have its recurrence ending at date and the new Incidence will have all recurrences past the date.
- Parameters:
-
incidence is a pointer to a recurring Incidence. date is the QDate within the recurring Incidence on which the dissociation will be performed. single is a flag meaning that a new Incidence should be created from the recurring Incidences after date.
- Returns:
- a pointer to a new recurring Incidence if single is false.
Definition at line 304 of file calendar.cpp.
virtual void KCal::Calendar::doSetTimeZoneId | ( | const QString & | ) | [inline, protected, virtual] |
Let Calendar subclasses set the Time Zone ID.
First parameter is a string containing a Time Zone ID, which is assumed to be valid. On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning:
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Reimplemented in KCal::CalendarResources.
Definition at line 887 of file calendar.h.
bool Calendar::endChange | ( | Incidence * | incidence | ) | [virtual] |
Flag that a change to a Calendar Incidence has completed.
- Parameters:
-
incidence is a pointer to the Incidence that was changed.
Reimplemented in KCal::CalendarResources.
Definition at line 848 of file calendar.cpp.
Returns the Event associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
Event::List Calendar::events | ( | const QDate & | date, | |
EventSortField | sortField = EventSortUnsorted , |
|||
SortDirection | sortDirection = SortDirectionAscending | |||
) |
Return a sorted, filtered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters:
-
date request filtered Event list for this QDate only. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of sorted, filtered Events occurring on date.
Definition at line 251 of file calendar.cpp.
Event::List Calendar::events | ( | const QDate & | start, | |
const QDate & | end, | |||
bool | inclusive = false | |||
) |
Return a filtered list of all Events occurring within a date range.
- Parameters:
-
start is the starting date. end is the ending date. inclusive if true only Events which are completely included within the date range are returned.
- Returns:
- the list of filtered Events occurring within the specified date range.
Definition at line 267 of file calendar.cpp.
Event::List Calendar::events | ( | const QDateTime & | qdt | ) |
Return a filtered list of all Events which occur on the given timestamp.
- Returns:
- the list of filtered Events occurring on the specified timestamp.
Definition at line 260 of file calendar.cpp.
Event::List Calendar::events | ( | EventSortField | sortField = EventSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, filtered list of all Events for this Calendar.
- Parameters:
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all filtered Events sorted as specified.
Definition at line 275 of file calendar.cpp.
CalFilter * Calendar::filter | ( | ) |
const Person & Calendar::getOwner | ( | ) | const |
Get the owner of the Calendar.
- Returns:
- the owner Person object.
Definition at line 72 of file calendar.cpp.
Returns the Incidence associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Definition at line 370 of file calendar.cpp.
Returns the Incidence associated with the given scheduling identifier.
- Parameters:
-
sid is a unique scheduling identifier string.
Definition at line 393 of file calendar.cpp.
Incidence::List Calendar::incidences | ( | const QDate & | date | ) | [virtual] |
Return a filtered list of all Incidences which occur on the given date.
- Returns:
- the list of filtered Incidences occurring on the specified date.
Definition at line 144 of file calendar.cpp.
Incidence::List Calendar::incidences | ( | ) | [virtual] |
Return a filtered list of all Incidences for this Calendar.
- Returns:
- the list of all filtered Incidences.
Definition at line 149 of file calendar.cpp.
Incidence::List Calendar::incidencesFromSchedulingID | ( | const QString & | UID | ) |
Searches all events and todos for (an incidence with this scheduling ID.
Returns a list of matching results.
Definition at line 382 of file calendar.cpp.
void Calendar::incidenceUpdated | ( | IncidenceBase * | incidenceBase | ) | [protected, virtual] |
The Observer interface.
So far not implemented.
- Parameters:
-
incidenceBase is a pointer an IncidenceBase object.
Implements KCal::IncidenceBase::Observer.
Reimplemented in KCal::CalendarLocal, and KCal::CalendarNull.
Definition at line 757 of file calendar.cpp.
bool Calendar::isLocalTime | ( | ) | const |
Determine if Calendar Incidences are to be written without a time zone.
- Returns:
- true if the Calendar is set to write Incidences withoout a time zone; false otherwise.
Definition at line 106 of file calendar.cpp.
bool KCal::Calendar::isModified | ( | ) | const [inline] |
Determine the Calendar's modification status.
- Returns:
- true if the Calendar has been modified since open or last save.
Definition at line 280 of file calendar.h.
virtual bool KCal::Calendar::isSaving | ( | ) | [inline, virtual] |
Determine if the Calendar is currently being saved.
- Returns:
- true if the Calendar is currently being saved; false otherwise.
Reimplemented in KCal::CalendarResources.
Definition at line 304 of file calendar.h.
Returns the Journal associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
Journal::List Calendar::journals | ( | const QDate & | date | ) | [virtual] |
Return a filtered list of all Journals for on the specifed date.
- Parameters:
-
date request filtered Journals for this QDate only.
- Returns:
- the list of filtered Journals for the specified date.
Definition at line 625 of file calendar.cpp.
Journal::List Calendar::journals | ( | JournalSortField | sortField = JournalSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, filtered list of all Journals for this Calendar.
- Parameters:
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all filtered Journals sorted as specified.
Definition at line 617 of file calendar.cpp.
Incidence::List Calendar::mergeIncidenceList | ( | const Event::List & | events, | |
const Todo::List & | todos, | |||
const Journal::List & | journals | |||
) | [static] |
Create a merged list of Events, Todos, and Journals.
- Parameters:
-
events is an Event list to merge. todos is a Todo list to merge. journals is a Journal list to merge.
- Returns:
- a list of merged Incidences.
Definition at line 822 of file calendar.cpp.
void Calendar::notifyIncidenceAdded | ( | Incidence * | incidence | ) | [protected] |
Let Calendar subclasses notify that they inserted an Incidence.
- Parameters:
-
incidence is a pointer to the Incidence object that was inserted.
Definition at line 771 of file calendar.cpp.
void Calendar::notifyIncidenceChanged | ( | Incidence * | incidence | ) | [protected] |
Let Calendar subclasses notify that they modified an Incidence.
- Parameters:
-
incidence is a pointer to the Incidence object that was modified.
Definition at line 783 of file calendar.cpp.
void Calendar::notifyIncidenceDeleted | ( | Incidence * | incidence | ) | [protected] |
Let Calendar subclasses notify that they removed an Incidence.
- Parameters:
-
incidence is a pointer to the Incidence object that was removed.
Definition at line 795 of file calendar.cpp.
QString Calendar::productId | ( | ) |
Get the Calendar's Product ID.
- Returns:
- the string containing the Product ID
Definition at line 817 of file calendar.cpp.
virtual Event::List KCal::Calendar::rawEvents | ( | const QDate & | start, | |
const QDate & | end, | |||
bool | inclusive = false | |||
) | [pure virtual] |
Return an unfiltered list of all Events occurring within a date range.
- Parameters:
-
start is the starting date. end is the ending date. inclusive if true only Events which are completely included within the date range are returned.
- Returns:
- the list of unfiltered Events occurring within the specified date range.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Event::List KCal::Calendar::rawEvents | ( | EventSortField | sortField = EventSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [pure virtual] |
Return a sorted, unfiltered list of all Events for this Calendar.
- Parameters:
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all unfiltered Events sorted as specified.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Event::List KCal::Calendar::rawEventsForDate | ( | const QDate & | date, | |
EventSortField | sortField = EventSortUnsorted , |
|||
SortDirection | sortDirection = SortDirectionAscending | |||
) | [pure virtual] |
Return a sorted, unfiltered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters:
-
date request unfiltered Event list for this QDate only. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of sorted, unfiltered Events occurring on date.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Event::List KCal::Calendar::rawEventsForDate | ( | const QDateTime & | qdt | ) | [pure virtual] |
Return an unfiltered list of all Events which occur on the given timestamp.
- Returns:
- the list of unfiltered Events occurring on the specified timestamp.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
Incidence::List Calendar::rawIncidences | ( | ) | [virtual] |
Return an unfiltered list of all Incidences for this Calendar.
- Returns:
- the list of all unfiltered Incidences.
Definition at line 154 of file calendar.cpp.
virtual Journal::List KCal::Calendar::rawJournals | ( | JournalSortField | sortField = JournalSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [pure virtual] |
Return a sorted, unfiltered list of all Journals for this Calendar.
- Parameters:
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all unfiltered Journals sorted as specified.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Journal::List KCal::Calendar::rawJournalsForDate | ( | const QDate & | date | ) | [pure virtual] |
Return an unfiltered list of all Journals for on the specifed date.
- Parameters:
-
date request unfiltered Journals for this QDate only.
- Returns:
- the list of unfiltered Journals for the specified date.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Todo::List KCal::Calendar::rawTodos | ( | TodoSortField | sortField = TodoSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [pure virtual] |
Return a sorted, unfiltered list of all Todos for this Calendar.
- Parameters:
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all unfiltered Todos sorted as specified.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
virtual Todo::List KCal::Calendar::rawTodosForDate | ( | const QDate & | date | ) | [pure virtual] |
Return an unfiltered list of all Todos which due on the specified date.
- Parameters:
-
date request unfiltered Todos due on this QDate.
- Returns:
- the list of unfiltered Todos due on the specified date.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
void Calendar::registerObserver | ( | Observer * | observer | ) |
virtual bool KCal::Calendar::reload | ( | const QString & | tz | ) | [pure virtual] |
Load the calendar contents from storage.
This requires the calendar to have been loaded once before, in other words initialized.
- tz The timezone to use for loading.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
void Calendar::removeRelations | ( | Incidence * | incidence | ) | [virtual] |
Remove all Relations from an Incidence.
- Parameters:
-
incidence is a pointer to the Incidence to have a Relation removed.
Definition at line 668 of file calendar.cpp.
virtual void KCal::Calendar::save | ( | ) | [pure virtual] |
Sync changes in memory to persistant storage.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
void Calendar::setFilter | ( | CalFilter * | filter | ) |
Set the Calendar filter.
- Parameters:
-
filter a pointer to a CalFilter object which will be used to filter Calendar Incidences.
Definition at line 111 of file calendar.cpp.
void Calendar::setLocalTime | ( | ) |
void Calendar::setModified | ( | bool | modified | ) |
Set if the Calendar had been modified.
- Parameters:
-
modified is true if the Calendar has been modified since open or last save.
Definition at line 744 of file calendar.cpp.
void Calendar::setObserversEnabled | ( | bool | enabled | ) | [protected] |
void Calendar::setOwner | ( | const Person & | owner | ) |
Set the owner of the Calendar.
- Parameters:
-
owner is a Person object.
Definition at line 77 of file calendar.cpp.
void Calendar::setProductId | ( | const QString & | productId | ) |
Set the Calendar Product ID.
- Parameters:
-
productId is a QString containing the Product ID.
Definition at line 812 of file calendar.cpp.
void Calendar::setTimeZoneId | ( | const QString & | timeZoneId | ) |
Set the Time Zone Id for the Calendar.
- Parameters:
-
timeZoneId is a string containing a Time Zone ID, which is assumed to be valid. The Time Zone Id is used to set the time zone for viewing Incidence dates.
On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning:
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Definition at line 84 of file calendar.cpp.
virtual void KCal::Calendar::setTimeZoneIdViewOnly | ( | const QString & | timeZoneId | ) | [pure virtual] |
Set the timezone used for viewing the incidences in this calendar.
In case it differs from the current timezone, shift the events such that they retain their absolute time (in UTC). setTimeZoneId
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
void Calendar::setupRelations | ( | Incidence * | incidence | ) | [virtual] |
Setup Relations for an Incidence.
- Parameters:
-
incidence is a pointer to the Incidence to have a Relation setup.
Definition at line 634 of file calendar.cpp.
Event::List Calendar::sortEvents | ( | Event::List * | eventList, | |
EventSortField | sortField, | |||
SortDirection | sortDirection | |||
) | [static] |
Sort a list of Events.
- Parameters:
-
eventList is a pointer to a list of Events. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- a list of Events sorted as specified.
Definition at line 159 of file calendar.cpp.
Journal::List Calendar::sortJournals | ( | Journal::List * | journalList, | |
JournalSortField | sortField, | |||
SortDirection | sortDirection | |||
) | [static] |
Sort a list of Journals.
- Parameters:
-
journalList is a pointer to a list of Journals. sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns:
- a list of Journals sorted as specified.
Definition at line 564 of file calendar.cpp.
Todo::List Calendar::sortTodos | ( | Todo::List * | todoList, | |
TodoSortField | sortField, | |||
SortDirection | sortDirection | |||
) | [static] |
Sort a list of Todos.
- Parameters:
-
todoList is a pointer to a list of Todos. sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns:
- a list of Todos sorted as specified.
Definition at line 405 of file calendar.cpp.
QString Calendar::timeZoneId | ( | ) | const |
Get the Time Zone ID for the Calendar.
- Returns:
- the string containg the Time Zone ID.
Definition at line 93 of file calendar.cpp.
Returns the Todo associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Implemented in KCal::CalendarLocal, KCal::CalendarNull, and KCal::CalendarResources.
Todo::List Calendar::todos | ( | const QDate & | date | ) | [virtual] |
Return a filtered list of all Todos which are due on the specified date.
- Parameters:
-
date request filtered Todos due on this QDate.
- Returns:
- the list of filtered Todos due on the specified date.
Definition at line 557 of file calendar.cpp.
Todo::List Calendar::todos | ( | TodoSortField | sortField = TodoSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, filtered list of all Todos for this Calendar.
- Parameters:
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all filtered Todos sorted as specified.
Definition at line 549 of file calendar.cpp.
void Calendar::unregisterObserver | ( | Observer * | observer | ) |
The documentation for this class was generated from the following files: