libkcal
KCal::CalendarResources Class Reference
This class provides a Calendar which is composed of other Calendars known as "Resources". More...
#include <calendarresources.h>
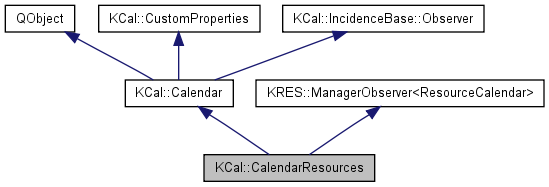
Detailed Description
This class provides a Calendar which is composed of other Calendars known as "Resources".Examples of Calendar Resources are:
- Calendars stored as local ICS formatted files
- a set of incidences (one-per-file) within a local directory
- birthdays and anniversaries contained in an addressbook
Definition at line 64 of file calendarresources.h.
Constructor & Destructor Documentation
CalendarResources::CalendarResources | ( | const QString & | timeZoneId, | |
const QString & | family = QString::fromLatin1( "calendar" ) | |||
) |
Construct CalendarResource object using a Time Zone and a Family name.
- Parameters:
-
timeZoneId is a string containing a Time Zone ID, which is assumed to be valid. The Time Zone Id is used to set the time zone for viewing Incidence dates.
On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning:
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
- Parameters:
-
family is any QString representing a unique name.
Definition at line 86 of file calendarresources.cpp.
CalendarResources::~CalendarResources | ( | ) |
Member Function Documentation
bool CalendarResources::addEvent | ( | Event * | event, | |
ResourceCalendar * | resource | |||
) |
Insert an Event into a Calendar Resource.
- Parameters:
-
event is a pointer to the Event to insert. resource is a pointer to the ResourceCalendar to be added to.
- Returns:
- true if the Event was successfully inserted; false otherwise.
- Note:
- In most cases use addIncidence( Incidence *incidence, ResourceCalendar *resource ) instead.
Definition at line 299 of file calendarresources.cpp.
bool CalendarResources::addEvent | ( | Event * | event | ) | [virtual] |
Insert an Event into the Calendar.
- Parameters:
-
event is a pointer to the Event to insert.
- Returns:
- true if the Event was successfully inserted; false otherwise.
- Note:
- In most cases use addIncidence( Incidence *incidence ) instead.
Implements KCal::Calendar.
Definition at line 293 of file calendarresources.cpp.
bool CalendarResources::addIncidence | ( | Incidence * | incidence, | |
ResourceCalendar * | resource | |||
) |
Insert an Incidence into a Calendar Resource.
- Parameters:
-
incidence is a pointer to the Incidence to insert. resource is a pointer to the ResourceCalendar to be added to.
- Returns:
- true if the Incidence was successfully inserted; false otherwise.
Definition at line 233 of file calendarresources.cpp.
bool CalendarResources::addIncidence | ( | Incidence * | incidence | ) | [virtual] |
Insert an Incidence into the Calendar.
- Parameters:
-
incidence is a pointer to the Incidence to insert.
- Returns:
- true if the Incidence was successfully inserted; false otherwise.
Reimplemented from KCal::Calendar.
Definition at line 266 of file calendarresources.cpp.
bool CalendarResources::addJournal | ( | Journal * | journal, | |
ResourceCalendar * | resource | |||
) |
Insert a Journal into a Calendar Resource.
- Parameters:
-
journal is a pointer to the Journal to insert. resource is a pointer to the ResourceCalendar to be added to.
- Returns:
- true if the Journal was successfully inserted; false otherwise.
- Note:
- In most cases use addIncidence( Incidence *incidence, ResourceCalendar *resource ) instead.
Definition at line 553 of file calendarresources.cpp.
bool CalendarResources::addJournal | ( | Journal * | journal | ) | [virtual] |
Insert a Journal into the Calendar.
- Parameters:
-
journal is a pointer to the Journal to insert.
- Returns:
- true if the Journal was successfully inserted; false otherwise.
- Note:
- In most cases use addIncidence( Incidence *incidence ) instead.
Implements KCal::Calendar.
Definition at line 526 of file calendarresources.cpp.
bool CalendarResources::addTodo | ( | Todo * | todo, | |
ResourceCalendar * | resource | |||
) |
Insert an Todo into a Calendar Resource.
- Parameters:
-
todo is a pointer to the Todo to insert. resource is a pointer to the ResourceCalendar to be added to.
- Returns:
- true if the Todo was successfully inserted; false otherwise.
- Note:
- In most cases use addIncidence( Incidence *incidence, ResourceCalendar *resource ) instead.
Definition at line 346 of file calendarresources.cpp.
bool CalendarResources::addTodo | ( | Todo * | todo | ) | [virtual] |
Insert a Todo into a Calendar Resource.
- Parameters:
-
todo is a pointer to the Todo to insert.
- Returns:
- true if the Todo was successfully inserted; false otherwise.
- Note:
- In most cases use addIncidence( Incidence *incidence ) instead.
Implements KCal::Calendar.
Definition at line 340 of file calendarresources.cpp.
Alarm::List CalendarResources::alarms | ( | const QDateTime & | from, | |
const QDateTime & | to | |||
) | [virtual] |
Return a list of Alarms within a time range for this Calendar.
- Parameters:
-
from is the starting timestamp. to is the ending timestamp.
- Returns:
- the list of Alarms for the for the specified time range.
Implements KCal::Calendar.
Definition at line 438 of file calendarresources.cpp.
Alarm::List CalendarResources::alarmsTo | ( | const QDateTime & | to | ) |
Return a list of Alarms that occur before the specified timestamp.
- Parameters:
-
to is the ending timestamp.
- Returns:
- the list of Alarms occurring before the specified QDateTime.
Definition at line 423 of file calendarresources.cpp.
bool CalendarResources::beginChange | ( | Incidence * | incidence | ) | [virtual] |
Flag that a change to a Calendar Incidence is starting.
- Parameters:
-
incidence is a pointer to the Incidence that will be changing.
Reimplemented from KCal::Calendar.
Definition at line 718 of file calendarresources.cpp.
void CalendarResources::close | ( | ) | [virtual] |
Clear out the current Calendar, freeing all used memory etc.
Implements KCal::Calendar.
Definition at line 192 of file calendarresources.cpp.
void CalendarResources::connectResource | ( | ResourceCalendar * | resource | ) | [protected] |
Definition at line 612 of file calendarresources.cpp.
int CalendarResources::decrementChangeCount | ( | ResourceCalendar * | resource | ) | [protected] |
Decrement the number of times this Resource has been changed by 1.
- Parameters:
-
resource is a pointer to the ResourceCalendar to be counted.
- Returns:
- the new number of times this Resource has been changed.
Definition at line 783 of file calendarresources.cpp.
bool CalendarResources::deleteEvent | ( | Event * | event | ) | [virtual] |
Remove an Event from the Calendar.
- Parameters:
-
event is a pointer to the Event to remove.
- Returns:
- true if the Event was successfully removed; false otherwise.
- Note:
- In most cases use deleteIncidence( Incidence *incidence) instead.
Implements KCal::Calendar.
Definition at line 304 of file calendarresources.cpp.
bool CalendarResources::deleteJournal | ( | Journal * | journal | ) | [virtual] |
Remove a Journal from the Calendar.
- Parameters:
-
journal is a pointer to the Journal to remove.
- Returns:
- true if the Journal was successfully removed; false otherwise.
- Note:
- In most cases use deleteIncidence( Incidence *incidence ) instead.
Implements KCal::Calendar.
Definition at line 532 of file calendarresources.cpp.
bool CalendarResources::deleteTodo | ( | Todo * | todo | ) | [virtual] |
Remove an Todo from the Calendar.
- Parameters:
-
todo is a pointer to the Todo to remove.
- Returns:
- true if the Todo was successfully removed; false otherwise.
- Note:
- In most cases use deleteIncidence( Incidence *incidence ) instead.
Implements KCal::Calendar.
Definition at line 351 of file calendarresources.cpp.
QWidget * CalendarResources::dialogParentWidget | ( | ) |
Returns the current parent for new dialogs.
This is a bad hack, but we need to properly set the parent for the resource selection dialog. Otherwise the dialog will not be modal to the editor dialog in korganizer and the user can still work in the editor dialog (and thus crash korganizer). Afterwards we need to reset it (to avoid pointers to widgets that are already deleted) so we also need the accessor
Definition at line 182 of file calendarresources.cpp.
void CalendarResources::doSetTimeZoneId | ( | const QString & | timeZoneId | ) | [protected, virtual] |
Let CalendarResource subclasses set the Time Zone ID.
First parameter is a string containing a Time Zone ID, which is assumed to be valid. On some systems, /usr/share/zoneinfo/zone.tab may be available for reference.
Example: "Europe/Berlin"
- Warning:
- Do Not pass an empty timeZoneId string as this may cause unintended consequences when storing Incidences into the Calendar.
Reimplemented from KCal::Calendar.
Definition at line 665 of file calendarresources.cpp.
bool CalendarResources::endChange | ( | Incidence * | incidence | ) | [virtual] |
Flag that a change to a Calendar Incidence has completed.
- Parameters:
-
incidence is a pointer to the Incidence that was changed.
Reimplemented from KCal::Calendar.
Definition at line 748 of file calendarresources.cpp.
Returns the Event associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Implements KCal::Calendar.
Definition at line 325 of file calendarresources.cpp.
int CalendarResources::incrementChangeCount | ( | ResourceCalendar * | resource | ) | [protected] |
Increment the number of times this Resource has been changed by 1.
- Parameters:
-
resource is a pointer to the ResourceCalendar to be counted.
- Returns:
- the new number of times this Resource has been changed.
Definition at line 770 of file calendarresources.cpp.
bool CalendarResources::isSaving | ( | ) | [virtual] |
Determine if the Calendar is currently being saved.
- Returns:
- true if the Calendar is currently being saved; false otherwise.
Reimplemented from KCal::Calendar.
Definition at line 221 of file calendarresources.cpp.
Returns the Journal associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Implements KCal::Calendar.
Definition at line 560 of file calendarresources.cpp.
void CalendarResources::load | ( | ) |
Loads all Incidences from the Resources.
The Resources must be added first using either readConfig(KConfig *config), which adds the system Resources, or manually using resourceAdded(ResourceCalendar *resource).
Definition at line 123 of file calendarresources.cpp.
Event::List CalendarResources::rawEvents | ( | const QDate & | start, | |
const QDate & | end, | |||
bool | inclusive = false | |||
) | [virtual] |
Return an unfiltered list of all Events occurring within a date range.
- Parameters:
-
start is the starting date. end is the ending date. inclusive if true only Events which are completely included within the date range are returned.
- Returns:
- the list of unfiltered Events occurring within the specified date range.
Implements KCal::Calendar.
Definition at line 471 of file calendarresources.cpp.
Event::List CalendarResources::rawEvents | ( | EventSortField | sortField = EventSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, unfiltered list of all Events.
- Parameters:
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all unfiltered Events sorted as specified.
Implements KCal::Calendar.
Definition at line 507 of file calendarresources.cpp.
Event::List CalendarResources::rawEventsForDate | ( | const QDate & | date, | |
EventSortField | sortField = EventSortUnsorted , |
|||
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, unfiltered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters:
-
date request unfiltered Event list for this QDate only. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of sorted, unfiltered Events occurring on date.
Implements KCal::Calendar.
Definition at line 454 of file calendarresources.cpp.
Event::List CalendarResources::rawEventsForDate | ( | const QDateTime & | qdt | ) | [virtual] |
Return an unfiltered list of all Events which occur on the given timestamp.
- Returns:
- the list of unfiltered Events occurring on the specified timestamp.
Implements KCal::Calendar.
Definition at line 489 of file calendarresources.cpp.
Journal::List CalendarResources::rawJournals | ( | JournalSortField | sortField = JournalSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, unfiltered list of all Journals for this Calendar.
- Parameters:
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all unfiltered Journals sorted as specified.
Implements KCal::Calendar.
Definition at line 577 of file calendarresources.cpp.
Journal::List CalendarResources::rawJournalsForDate | ( | const QDate & | date | ) | [virtual] |
Return an unfiltered list of all Journals for on the specifed date.
- Parameters:
-
date request unfiltered Journals for this QDate only.
- Returns:
- the list of unfiltered Journals for the specified date.
Implements KCal::Calendar.
Definition at line 595 of file calendarresources.cpp.
Todo::List CalendarResources::rawTodos | ( | TodoSortField | sortField = TodoSortUnsorted , |
|
SortDirection | sortDirection = SortDirectionAscending | |||
) | [virtual] |
Return a sorted, unfiltered list of all Todos for this Calendar.
- Parameters:
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns:
- the list of all unfiltered Todos sorted as specified.
Implements KCal::Calendar.
Definition at line 372 of file calendarresources.cpp.
Todo::List CalendarResources::rawTodosForDate | ( | const QDate & | date | ) | [virtual] |
Return an unfiltered list of all Todos which are due on the specified date.
- Parameters:
-
date request unfiltered Todos due on this QDate.
- Returns:
- the list of unfiltered Todos due on the specified date.
Implements KCal::Calendar.
Definition at line 406 of file calendarresources.cpp.
void CalendarResources::readConfig | ( | KConfig * | config = 0 |
) |
Read the Resources settings from a config file.
- Parameters:
-
config The KConfig object which points to the config file. If no object is given (null pointer) the standard config file is used.
- Note:
- Call this method before load().
Definition at line 113 of file calendarresources.cpp.
void CalendarResources::releaseSaveTicket | ( | Ticket * | ticket | ) | [virtual] |
Release the save Ticket.
The Calendar is unlocked without saving.
- Parameters:
-
ticket is a pointer to a Ticket object.
Definition at line 712 of file calendarresources.cpp.
bool CalendarResources::reload | ( | const QString & | tz | ) | [virtual] |
Reloads all incidences from all resources.
- tz The timezone to set.
- Returns:
- success or failure
Implements KCal::Calendar.
Definition at line 163 of file calendarresources.cpp.
CalendarResources::Ticket * CalendarResources::requestSaveTicket | ( | ResourceCalendar * | resource | ) |
Request ticket for saving the Calendar.
If a ticket is returned the Calendar is locked for write access until save() or releaseSaveTicket() is called.
- Parameters:
-
resource is a pointer to the ResourceCalendar object.
- Returns:
- a pointer to a Ticket object indicating that the Calendar is locked for write access; otherwise a null pointer.
Definition at line 681 of file calendarresources.cpp.
ResourceCalendar * CalendarResources::resource | ( | Incidence * | incidence | ) |
Get the Resource associated with a specified Incidence.
- Parameters:
-
incidence is a pointer to an Incidence whose Resource is to be located.
- Returns:
- a pointer to the Resource containing the Incidence.
Definition at line 627 of file calendarresources.cpp.
void CalendarResources::resourceAdded | ( | ResourceCalendar * | resource | ) |
Add a Resource to the Calendar.
This method must be public, because in-process added Resources do not emit the corresponding signal, so this methodd has to be called manually!
- Parameters:
-
resource is a pointer to the ResourceCalendar to add.
Definition at line 635 of file calendarresources.cpp.
void CalendarResources::resourceDeleted | ( | ResourceCalendar * | resource | ) | [protected] |
Definition at line 658 of file calendarresources.cpp.
CalendarResourceManager* KCal::CalendarResources::resourceManager | ( | ) | const [inline] |
Get the CalendarResourceManager used by this calendar.
- Returns:
- a pointer to the CalendarResourceManage.
Definition at line 222 of file calendarresources.h.
void CalendarResources::resourceModified | ( | ResourceCalendar * | resource | ) | [protected] |
Definition at line 651 of file calendarresources.cpp.
void CalendarResources::save | ( | ) | [virtual] |
Sync changes in memory to persistant storage.
Implements KCal::Calendar.
Definition at line 207 of file calendarresources.cpp.
Save this Calendar.
If the save is successfull, the Ticket is deleted. Otherwise, the caller must release the Ticket with releaseSaveTicket() to abandon the save operation or call save() to try the save again.
- Parameters:
-
ticket is a pointer to the Ticket object. incidence is a pointer to the Incidence object. If incidence is null, save the entire Calendar (all Resources) else only the specified Incidence is saved.
- Returns:
- true if the save was successful; false otherwise.
Definition at line 694 of file calendarresources.cpp.
void CalendarResources::setAskDestinationPolicy | ( | ) |
Set the destination policy such that Incidences are added to a Resource which is queried.
Definition at line 177 of file calendarresources.cpp.
void CalendarResources::setDialogParentWidget | ( | QWidget * | parent | ) |
Set the widget parent for new dialogs.
This is a bad hack, but we need to properly set the parent for the resource selection dialog. Otherwise the dialog will not be modal to the editor dialog in korganizer and the user can still work in the editor dialog (and thus crash korganizer).
Definition at line 187 of file calendarresources.cpp.
void CalendarResources::setStandardDestinationPolicy | ( | ) |
Set the destination policy such that Incidences are always added to the standard Resource.
Definition at line 172 of file calendarresources.cpp.
void CalendarResources::setTimeZoneIdViewOnly | ( | const QString & | tz | ) | [virtual] |
Set the viewing time zone, which requires that all resources are saved, and then reloaded such that the event times are re-interpreted in the new timezone.
Note that the absolute times of events do not change with this. If you want to change the times of the contents of the resources, use setTimeZoneId
Implements KCal::Calendar.
Definition at line 675 of file calendarresources.cpp.
void KCal::CalendarResources::signalErrorMessage | ( | const QString & | err | ) | [signal] |
Signal an error message.
void KCal::CalendarResources::signalResourceAdded | ( | ResourceCalendar * | resource | ) | [signal] |
Signal that an Incidence has been inserted to the Resource.
void KCal::CalendarResources::signalResourceDeleted | ( | ResourceCalendar * | resource | ) | [signal] |
Signal that an Incidence has been removed from the Resource.
void KCal::CalendarResources::signalResourceModified | ( | ResourceCalendar * | resource | ) | [signal] |
Signal that the Resource has been modified.
void CalendarResources::slotLoadError | ( | ResourceCalendar * | resource, | |
const QString & | err | |||
) | [protected, slot] |
Definition at line 801 of file calendarresources.cpp.
void CalendarResources::slotSaveError | ( | ResourceCalendar * | resource, | |
const QString & | err | |||
) | [protected, slot] |
Definition at line 806 of file calendarresources.cpp.
Returns the Todo associated with the given unique identifier.
- Parameters:
-
uid is a unique identifier string.
Implements KCal::Calendar.
Definition at line 389 of file calendarresources.cpp.
The documentation for this class was generated from the following files: