KBookmarkGroup
#include <kbookmark.h>
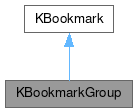
Protected Member Functions | |
QDomElement | nextKnownTag (const QDomElement &start, bool goNext) const |
Additional Inherited Members | |
![]() | |
enum | MetaDataOverwriteMode { OverwriteMetaData , DontOverwriteMetaData } |
![]() | |
static QString | commonParent (const QString &A, const QString &B) |
static QString | nextAddress (const QString &address) |
static QString | parentAddress (const QString &address) |
static uint | positionInParent (const QString &address) |
static QString | previousAddress (const QString &address) |
static KBookmark | standaloneBookmark (const QString &text, const QUrl &url, const QString &icon) |
![]() | |
QDomElement | element |
Detailed Description
A group of bookmarks.
Definition at line 315 of file kbookmark.h.
Constructor & Destructor Documentation
◆ KBookmarkGroup() [1/2]
KBookmarkGroup::KBookmarkGroup | ( | ) |
Create an invalid group.
This is mostly for use in QList, and other places where we need a null group. Also used as a parent for a bookmark that doesn't have one (e.g. Netscape bookmarks)
Definition at line 104 of file kbookmark.cpp.
◆ KBookmarkGroup() [2/2]
KBookmarkGroup::KBookmarkGroup | ( | const QDomElement & | elem | ) |
Create a bookmark group as specified by the given element.
Definition at line 109 of file kbookmark.cpp.
Member Function Documentation
◆ addBookmark() [1/2]
Create a new bookmark, as the last child of this group Don't forget to use KBookmarkManager::self()->emitChanged( parentBookmark );.
- Parameters
-
bm the bookmark to add
Definition at line 212 of file kbookmark.cpp.
◆ addBookmark() [2/2]
KBookmark KBookmarkGroup::addBookmark | ( | const QString & | text, |
const QUrl & | url, | ||
const QString & | icon ) |
Create a new bookmark, as the last child of this group Don't forget to use KBookmarkManager::self()->emitChanged( parentBookmark );.
- Parameters
-
text for the bookmark url the URL that the bookmark points to. It will be stored in its QUrl::FullyEncoded string format. icon the name of the icon to associate with the bookmark. A suitable default will be determined from the URL if not specified.
Definition at line 218 of file kbookmark.cpp.
◆ createNewFolder()
KBookmarkGroup KBookmarkGroup::createNewFolder | ( | const QString & | text | ) |
Create a new bookmark folder, as the last child of this group.
- Parameters
-
text for the folder. If you want an dialog use KBookmarkDialog
Definition at line 161 of file kbookmark.cpp.
◆ createNewSeparator()
KBookmark KBookmarkGroup::createNewSeparator | ( | ) |
Create a new bookmark separator Don't forget to use KBookmarkManager::self()->emitChanged( parentBookmark );.
Definition at line 175 of file kbookmark.cpp.
◆ deleteBookmark()
void KBookmarkGroup::deleteBookmark | ( | const KBookmark & | bk | ) |
Delete a bookmark - it has to be one of our children !
Don't forget to use KBookmarkManager::self()->emitChanged( parentBookmark );
Definition at line 238 of file kbookmark.cpp.
◆ findToolbar()
QDomElement KBookmarkGroup::findToolbar | ( | ) | const |
Definition at line 248 of file kbookmark.cpp.
◆ first()
KBookmark KBookmarkGroup::first | ( | ) | const |
Return the first child bookmark of this group.
Definition at line 119 of file kbookmark.cpp.
◆ groupUrlList()
- Returns
- the list of urls of bookmarks at top level of the group
Definition at line 262 of file kbookmark.cpp.
◆ indexOf()
int KBookmarkGroup::indexOf | ( | const KBookmark & | child | ) | const |
Return the index of a child bookmark, -1 if not found.
Definition at line 134 of file kbookmark.cpp.
◆ isOpen()
bool KBookmarkGroup::isOpen | ( | ) | const |
- Returns
- true if the bookmark folder is opened in the bookmark editor
Definition at line 114 of file kbookmark.cpp.
◆ isToolbarGroup()
bool KBookmarkGroup::isToolbarGroup | ( | ) | const |
- Returns
- true if this is the toolbar group
Definition at line 243 of file kbookmark.cpp.
◆ moveBookmark()
Moves bookmark
after after
(which should be a child of ours).
If after is null, bookmark
is moved as the first child. Don't forget to use KBookmarkManager::self()->emitChanged( parentBookmark );
Definition at line 187 of file kbookmark.cpp.
◆ next()
Return the next sibling of a child bookmark of this group.
- Parameters
-
current has to be one of our child bookmarks.
Definition at line 129 of file kbookmark.cpp.
◆ nextKnownTag()
|
protected |
Definition at line 145 of file kbookmark.cpp.
◆ previous()
Return the previous sibling of a child bookmark of this group.
- Parameters
-
current has to be one of our child bookmarks.
Definition at line 124 of file kbookmark.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:04:45 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.