KBookmark
#include <KBookmark>
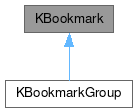
Classes | |
class | List |
Public Types | |
enum | MetaDataOverwriteMode { OverwriteMetaData , DontOverwriteMetaData } |
Public Member Functions | |
KBookmark () | |
KBookmark (const QDomElement &elem) | |
QString | address () const |
QString | description () const |
QString | fullText () const |
bool | hasParent () const |
QString | icon () const |
QDomElement | internalElement () const |
bool | isGroup () const |
bool | isNull () const |
bool | isSeparator () const |
QDomNode | metaData (const QString &owner, bool create) const |
QString | metaDataItem (const QString &key) const |
QString | mimeType () const |
bool | operator== (const KBookmark &rhs) const |
KBookmarkGroup | parentGroup () const |
void | populateMimeData (QMimeData *mimeData) const |
int | positionInParent () const |
void | setDescription (const QString &description) |
void | setFullText (const QString &fullText) |
void | setIcon (const QString &icon) |
void | setMetaDataItem (const QString &key, const QString &value, MetaDataOverwriteMode mode=OverwriteMetaData) |
void | setMimeType (const QString &mimeType) |
void | setShowInToolbar (bool show) |
void | setUrl (const QUrl &url) |
bool | showInToolbar () const |
QString | text () const |
KBookmarkGroup | toGroup () const |
void | updateAccessMetadata () |
QUrl | url () const |
Static Public Member Functions | |
static QString | commonParent (const QString &A, const QString &B) |
static QString | nextAddress (const QString &address) |
static QString | parentAddress (const QString &address) |
static uint | positionInParent (const QString &address) |
static QString | previousAddress (const QString &address) |
static KBookmark | standaloneBookmark (const QString &text, const QUrl &url, const QString &icon) |
Protected Attributes | |
QDomElement | element |
Detailed Description
A class representing a bookmark.
Definition at line 26 of file kbookmark.h.
Member Enumeration Documentation
◆ MetaDataOverwriteMode
enum KBookmark::MetaDataOverwriteMode |
Definition at line 31 of file kbookmark.h.
Constructor & Destructor Documentation
◆ KBookmark() [1/2]
KBookmark::KBookmark | ( | ) |
Constructs a null bookmark, i.e.
a bookmark for which isNull() returns true If you want to create a new bookmark use eitehr KBookmarkGroup.addBookmark or if you want an interactive dialog use KBookmarkDialog.
Definition at line 276 of file kbookmark.cpp.
◆ KBookmark() [2/2]
|
explicit |
Creates the KBookmark wrapper for.
- Parameters
-
elem Mostly for internal usage.
Definition at line 280 of file kbookmark.cpp.
Member Function Documentation
◆ address()
QString KBookmark::address | ( | ) | const |
Return the "address" of this bookmark in the whole tree.
This is used when telling other processes about a change in a given bookmark. The encoding of the address is "/4/2", for instance, to designate the 2nd child inside the 4th child of the root bookmark.
Definition at line 477 of file kbookmark.cpp.
◆ commonParent()
- Returns
- the common parent of both addresses which has the greatest depth
Definition at line 515 of file kbookmark.cpp.
◆ description()
QString KBookmark::description | ( | ) | const |
◆ fullText()
QString KBookmark::fullText | ( | ) | const |
Text shown for the bookmark, not truncated.
You should not use this - this is mainly for keditbookmarks.
Definition at line 313 of file kbookmark.cpp.
◆ hasParent()
bool KBookmark::hasParent | ( | ) | const |
- Returns
- true if bookmark is contained by a QDomDocument, if not it is most likely that it has become separated and is thus invalid and/or has been deleted from the bookmarks.
Definition at line 302 of file kbookmark.cpp.
◆ icon()
QString KBookmark::icon | ( | ) | const |
- Returns
- the pixmap file for this bookmark (i.e. the name of the icon)
Definition at line 351 of file kbookmark.cpp.
◆ internalElement()
QDomElement KBookmark::internalElement | ( | ) | const |
for KEditBookmarks
Definition at line 500 of file kbookmark.cpp.
◆ isGroup()
bool KBookmark::isGroup | ( | ) | const |
Whether the bookmark is a group or a normal bookmark.
Definition at line 285 of file kbookmark.cpp.
◆ isNull()
bool KBookmark::isNull | ( | ) | const |
- Returns
- true if this is a null bookmark. This will never be the case for a real bookmark (in a menu), but it's used for instance as the end condition for KBookmarkGroup::next()
Definition at line 297 of file kbookmark.cpp.
◆ isSeparator()
bool KBookmark::isSeparator | ( | ) | const |
Whether the bookmark is a separator.
Definition at line 292 of file kbookmark.cpp.
◆ metaData()
- Returns
- the metadata container node for a certain metadata owner
- Since
- 4.1
Definition at line 581 of file kbookmark.cpp.
◆ metaDataItem()
Get the value of a specific metadata item (owner = "http://www.kde.org").
- Parameters
-
key Name of the metadata item
- Returns
- Value of the metadata item. QString() is returned in case the specified key does not exist.
Definition at line 590 of file kbookmark.cpp.
◆ mimeType()
QString KBookmark::mimeType | ( | ) | const |
◆ nextAddress()
- Returns
- address of next sibling (e.g. /4/5/2 -> /4/5/3) This doesn't check whether it actually exists
Definition at line 576 of file kbookmark.cpp.
◆ operator==()
bool KBookmark::operator== | ( | const KBookmark & | rhs | ) | const |
Comparison operator.
Definition at line 613 of file kbookmark.cpp.
◆ parentAddress()
- Returns
- address of parent
Definition at line 560 of file kbookmark.cpp.
◆ parentGroup()
KBookmarkGroup KBookmark::parentGroup | ( | ) | const |
- Returns
- the group containing this bookmark
Definition at line 466 of file kbookmark.cpp.
◆ populateMimeData()
void KBookmark::populateMimeData | ( | QMimeData * | mimeData | ) | const |
Adds this bookmark into the given QMimeData.
WARNING: do not call this method multiple times, use KBookmark::List::populateMimeData instead.
- Parameters
-
mimeData the QMimeData instance used to drag or copy this bookmark
Definition at line 663 of file kbookmark.cpp.
◆ positionInParent() [1/2]
int KBookmark::positionInParent | ( | ) | const |
Return the position in the parent, i.e.
the last number in the address
Definition at line 495 of file kbookmark.cpp.
◆ positionInParent() [2/2]
|
static |
- Returns
- position in parent (e.g. /4/5/2 -> 2)
Definition at line 565 of file kbookmark.cpp.
◆ previousAddress()
- Returns
- address of previous sibling (e.g. /4/5/2 -> /4/5/1) Returns QString() for a first child
Definition at line 570 of file kbookmark.cpp.
◆ setDescription()
void KBookmark::setDescription | ( | const QString & | description | ) |
Set the description of the bookmark.
- Parameters
-
description
- Since
- 4.4
Definition at line 420 of file kbookmark.cpp.
◆ setFullText()
void KBookmark::setFullText | ( | const QString & | fullText | ) |
Set the text shown for the bookmark.
- Parameters
-
fullText the new bookmark title
Definition at line 324 of file kbookmark.cpp.
◆ setIcon()
void KBookmark::setIcon | ( | const QString & | icon | ) |
Set the icon name of the bookmark.
- Parameters
-
icon the new icon name for this bookmark
Definition at line 397 of file kbookmark.cpp.
◆ setMetaDataItem()
void KBookmark::setMetaDataItem | ( | const QString & | key, |
const QString & | value, | ||
MetaDataOverwriteMode | mode = OverwriteMetaData ) |
Change the value of a specific metadata item, or create the given item if it doesn't exist already (owner = "http://www.kde.org").
- Parameters
-
key Name of the metadata item to change value Value to use for the specified metadata item mode Whether to overwrite the item's value if it exists already or not.
Definition at line 601 of file kbookmark.cpp.
◆ setMimeType()
void KBookmark::setMimeType | ( | const QString & | mimeType | ) |
Set the Mime-Type of this item.
- Parameters
-
Mime-Type
- Since
- 4.1
Definition at line 444 of file kbookmark.cpp.
◆ setShowInToolbar()
void KBookmark::setShowInToolbar | ( | bool | show | ) |
Set whether this bookmark is show in a filterd toolbar.
Definition at line 461 of file kbookmark.cpp.
◆ setUrl()
void KBookmark::setUrl | ( | const QUrl & | url | ) |
Set the URL of the bookmark.
- Parameters
-
url the new bookmark URL
Definition at line 346 of file kbookmark.cpp.
◆ showInToolbar()
bool KBookmark::showInToolbar | ( | ) | const |
- Returns
- if the bookmark should be shown in the toolbar (used by the filtered toolbar)
Definition at line 451 of file kbookmark.cpp.
◆ standaloneBookmark()
|
static |
Creates a stand alone bookmark.
This is fairly expensive since a new QDom Tree is build.
Definition at line 505 of file kbookmark.cpp.
◆ text()
QString KBookmark::text | ( | ) | const |
Text shown for the bookmark If bigger than 40, the text is shortened by replacing middle characters with "..." (see KStringHandler::csqueeze)
Definition at line 308 of file kbookmark.cpp.
◆ toGroup()
KBookmarkGroup KBookmark::toGroup | ( | ) | const |
Convert this to a group - do this only if isGroup() returns true.
Definition at line 471 of file kbookmark.cpp.
◆ updateAccessMetadata()
void KBookmark::updateAccessMetadata | ( | ) |
Updates the bookmarks access metadata Call when a user accesses the bookmark.
Definition at line 540 of file kbookmark.cpp.
◆ url()
QUrl KBookmark::url | ( | ) | const |
URL contained by the bookmark.
Definition at line 341 of file kbookmark.cpp.
Member Data Documentation
◆ element
|
protected |
Definition at line 305 of file kbookmark.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:53:54 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.